Introduction to WebRTC and React
What is WebRTC?
WebRTC, or Web Real-Time Communication, is a revolutionary open-source project that has transformed the landscape of digital communication. It enables peer-to-peer communication directly in the web browser without the need for any plugins or third-party software. This technology supports video, audio, and data transmission, making it a backbone for applications like video conferencing, live streaming, and instant messaging. The core appeal of WebRTC lies in its ability to facilitate real-time media sharing across diverse platforms with minimal latency
DEV Community
.What is React?
React, a JavaScript library developed by Facebook, is renowned for its efficiency in building dynamic user interfaces. Its component-based architecture allows developers to construct complex applications with reusable, maintainable code components. For developers aiming to integrate WebRTC into web applications, React provides a robust framework to manage the stateful logic required for real-time communications. This is particularly advantageous in WebRTC applications, where the state of connections, streams, and data transfers needs to be efficiently synced with the user interface to provide a seamless experience.
Integrating WebRTC with React
Combining WebRTC with React allows developers to leverage React's state management and component lifecycle methods to handle real-time data effectively. This integration enables the development of robust applications that can handle real-time audio and video communication seamlessly, providing users with high-quality interaction experiences. As real-time communication becomes increasingly integral to digital experiences, understanding how to integrate WebRTC with React not only enhances the performance but also the scalability of applications.
By the end of this section, developers will have a clear understanding of how WebRTC works and why React is an excellent choice for building applications that require real-time communication capabilities. The following sections will dive deeper into setting up a development environment, building basic components, and implementing WebRTC in a React application, providing a comprehensive guide for developers looking to explore this powerful combination.
Setting Up the Development Environment
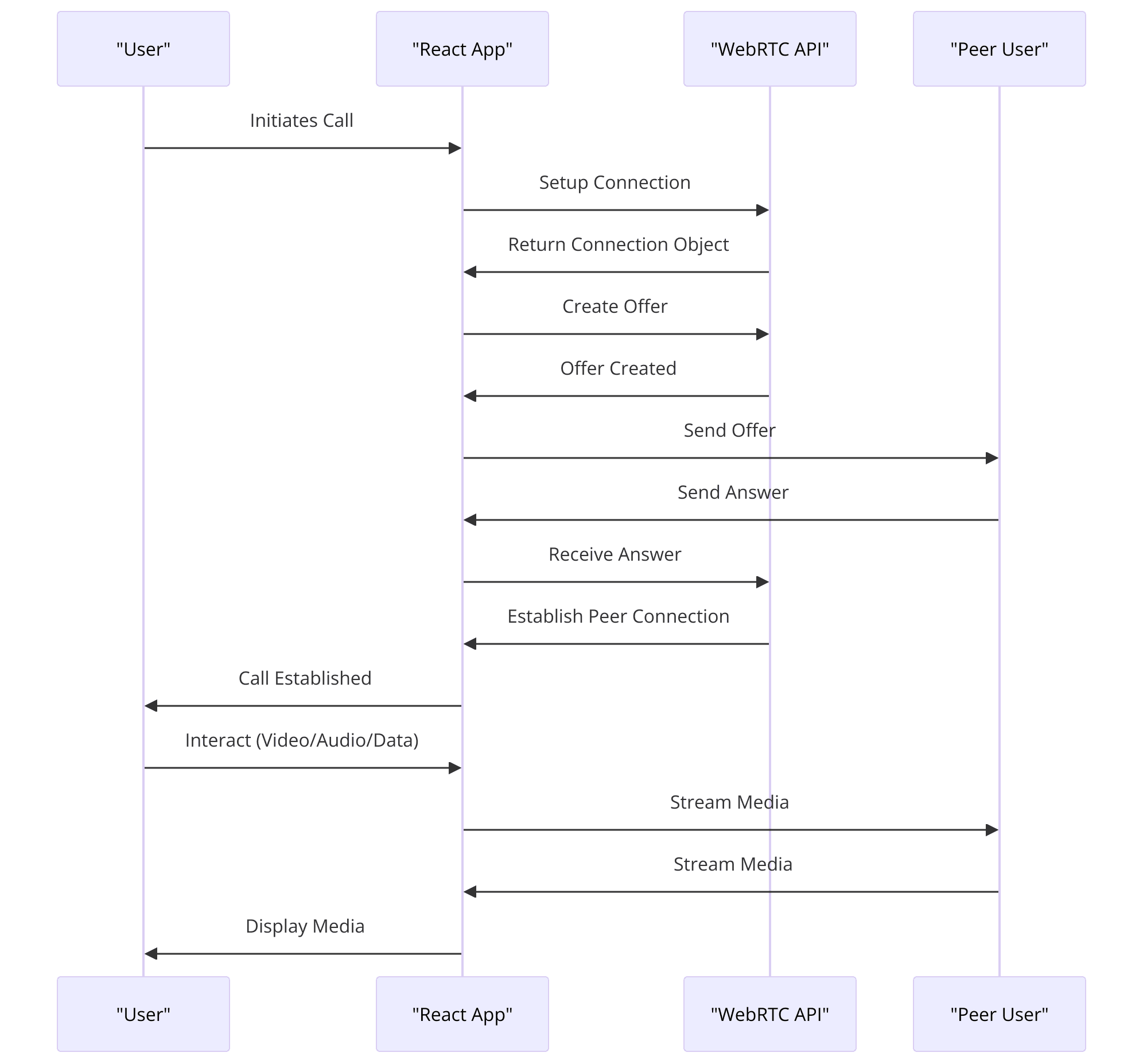
Before diving into the intricacies of WebRTC with React, it's essential to properly set up a development environment that can handle the demands of real-time communication applications. This section will guide you through the steps required to prepare your system, from creating a new React project to installing the necessary dependencies to support WebRTC.
Creating a New React Project
The first step in setting up your development environment is to create a new React project. This can be done easily with the Create React App (CRA) tool, which sets up the initial structure of your project with sensible defaults, including configurations for Babel and Webpack, which are essential for modern web development.
To create a new project, run the following command in your terminal:
1npx create-react-app my-webrtc-app
This command pulls the latest version of Create React App and sets up a new project named "my-webrtc-app". Once the setup process is complete, navigate into your project directory:
1cd my-webrtc-app
At this point, you can start the development server to see the initial React app by running:
1npm start
This command will open your default web browser to
http://localhost:3000
, where you can view your new React application running (Web and Mobile Dev Platform).Installing Necessary Dependencies
After setting up the basic React application, you need to add specific libraries that are crucial for integrating WebRTC. These libraries include
socket.io-client
for managing WebSocket connections, which is essential for the signaling process in WebRTC communications, and potentially a WebRTC adapter to ensure compatibility across all browsers.To install these dependencies, run the following commands in your project directory:
1npm install socket.io-client
2npm install webrtc-adapter
The
socket.io-client
library facilitates real-time, bidirectional event-based communication, which is necessary for signaling in WebRTC to exchange information like media metadata, network configuration, and control messages. The webrtc-adapter
is a shim to insulate apps from spec changes and prefix differences.Understanding Basic React and WebRTC Concepts
Before proceeding with the coding, make sure you have a solid understanding of React fundamentals, such as components, state, and props, as well as the lifecycle methods that are critical for managing the real-time aspects of WebRTC. Familiarity with JavaScript promises and async functions is also beneficial because much of the WebRTC functionality is based on asynchronous operations.
Additionally, a basic grasp of WebRTC's core components is helpful:
- RTCPeerConnection: Manages the connection between two peers.
- RTCDataChannel: Allows bidirectional data exchange between peers.
- MediaStream: Handles streams of audio or video.
This foundational knowledge will significantly ease the development process as you begin to integrate these technologies into your React application (
MDN Web Docs
).With your development environment set up and a basic understanding of the necessary technologies, you're now ready to start building the core components of your WebRTC-enabled React application. The following section will delve into structuring your project and setting up the main features needed for real-time communication.
Basic Components and Structure
After setting up the development environment and understanding the foundational concepts of WebRTC and React, the next step involves creating the basic components of your application. This section will outline the component structure and provide code examples for setting up a simple WebRTC application using React.
Component Structure
In a basic WebRTC application built with React, you typically need several components to manage different aspects of the application:
- App Component: Serves as the root component that encapsulates other components.
- VideoPlayer Component: Manages the display of video streams.
- Options Component: Provides controls for the user to manage the call (e.g., buttons to call, hang up, and mute).
- Notifications Component: Displays alerts and notifications to the user about call events.
Here is an overview of how these components interact within the application:
- App Component: Hosts the primary application logic and state, including the setup and management of the WebRTC connection.
- VideoPlayer Component: Handles the rendering of both local and remote video streams.
- Options Component: Includes user interface elements that allow users to perform actions like starting a call, ending a call, or switching their audio/video settings.
- Notifications Component: Alerts the user to incoming calls or other significant events within the application.
Code Example: Initial Setup
To start, you'll create these components within the React application. Below is an example of how you might structure your
App.js
to include these components:1import React from 'react';
2import VideoPlayer from './components/VideoPlayer';
3import Options from './components/Options';
4import Notifications from './components/Notifications';
5
6function App() {
7 return (
8 <div className="App">
9 <h1>WebRTC and React</h1>
10 <VideoPlayer />
11 <Options />
12 <Notifications />
13 </div>
14 );
15}
16
17export default App;
Each component will be developed to handle its specific tasks:
- VideoPlayer.jsx: This component will use the
useRef
hook to reference the video HTML elements that will display the media streams. - Options.jsx: This will manage the call actions and might include buttons that use callbacks to control the call state.
- Notifications.jsx: Used for displaying call-related notifications to the user, such as an incoming call alert.
VideoPlayer Component Example
Here is a simplified version of what the
VideoPlayer.jsx
component might look like:1import React, { useRef, useEffect } from 'react';
2
3const VideoPlayer = ({ localStream, remoteStream }) => {
4 const localVideoRef = useRef(null);
5 const remoteVideoRef = useRef(null);
6
7 useEffect(() => {
8 if (localStream && localVideoRef.current) {
9 localVideoRef.current.srcObject = localStream;
10 }
11 if (remoteStream && remoteVideoRef.current) {
12 remoteVideoRef.current.srcObject = remoteStream;
13 }
14 }, [localStream, remoteStream]);
15
16 return (
17 <div>
18 <video ref={localVideoRef} autoPlay muted />
19 <video ref={remoteVideoRef} autoPlay />
20 </div>
21 );
22};
23
24export default VideoPlayer;
In this example, the
VideoPlayer
component receives localStream
and remoteStream
as props, which are attached to video elements defined within the component. The useEffect
hook updates these video elements whenever the stream objects change.With the basic components set up, the next step is to implement the WebRTC functionality, including establishing peer connections and handling the signaling server communication. This will be covered in the next part of the article, where we'll delve deeper into integrating WebRTC into these components for real-time communication capabilities.
Implementing WebRTC in React App
Now that the basic structure and components of our WebRTC application are in place, it’s time to focus on the core functionality that will enable real-time communication. This involves setting up the WebRTC peer connections, handling the signaling process to coordinate these connections, and managing the media streams. We'll break down each of these tasks and provide code snippets to illustrate the implementation.
Setting Up the Peer Connection
The
RTCPeerConnection
interface is the backbone of any WebRTC application, enabling audio and video communication between peers. Here’s how to integrate it into your React application:Initialization
You need to create a new peer connection instance when a user intends to start a call. This instance is configured with ICE servers (STUN and TURN) to facilitate the connection between peers behind NATs and firewalls.
1const configuration = {
2 iceServers: [
3 { urls: 'stun:stun.l.google.com:19302' },
4 { urls: 'turn:numb.viagenie.ca', credential: 'muazkh', username: 'webrtc@live.com' }
5 ]
6};
7const peerConnection = new RTCPeerConnection(configuration);
Stream Handling
After establishing the peer connection, the next step is to add the local media stream to it, which involves capturing media from the user’s device.
1navigator.mediaDevices.getUserMedia({ video: true, audio: true })
2 .then(stream => {
3 localStream.current = stream;
4 // Add stream to the peer connection
5 stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
6 })
7 .catch(error => console.log('Error accessing media devices.', error));
Signaling and Managing Connections
Signaling is the process by which peers coordinate the communication prior to and during the connection. This includes exchanging metadata about media and any data required to coordinate the connection and control the communication session.
Signal Handling
Set up event listeners for handling signaling messages like 'offer', 'answer', and 'icecandidate', which are essential for establishing the peer-to-peer connection.
1socket.on('signal', data => {
2 switch(data.type) {
3 case 'offer':
4 handleReceiveOffer(data.offer, peerConnection);
5 break;
6 case 'answer':
7 handleReceiveAnswer(data.answer, peerConnection);
8 break;
9 case 'icecandidate':
10 handleNewICECandidateMsg(data.candidate, peerConnection);
11 break;
12 default:
13 break;
14 }
15});
Creating and Sending Offers/Answers
Implement functions to create and send offers and answers. When you receive an offer, you should create an answer in response, and vice versa.
1function handleReceiveOffer(offer, peerConnection) {
2 peerConnection.setRemoteDescription(new RTCSessionDescription(offer))
3 .then(() => peerConnection.createAnswer())
4 .then(answer => peerConnection.setLocalDescription(answer))
5 .then(() => socket.emit('signal', { type: 'answer', answer: peerConnection.localDescription }));
6}
7
8function handleReceiveAnswer(answer, peerConnection) {
9 const remoteDesc = new RTCSessionDescription(answer);
10 peerConnection.setRemoteDescription(remoteDesc);
11}
Handling ICE Candidates
ICE candidates are necessary to enable the peer connection to establish the most efficient path for the media stream.
1peerConnection.onicecandidate = event => {
2 if (event.candidate) {
3 socket.emit('signal', { type: 'icecandidate', candidate: event.candidate });
4 }
5};
6
7function handleNewICECandidateMsg(candidate, peerConnection) {
8 const iceCandidate = new RTCIceCandidate(candidate);
9 peerConnection.addIceCandidate(iceCandidate);
10}
Final Integration
Finally, integrate these functionalities into your React components, making sure that they react appropriately to user actions and events within the application. This involves updating the state based on events like receiving a call, ending a call, or encountering errors.
With the implementation of these features, your application will be able to facilitate real-time audio and video communication. The next part of the article will explore more advanced features and troubleshooting techniques to enhance the application’s capabilities and stability.
Advanced Features and Troubleshooting
After establishing the core functionalities of a WebRTC-enabled React application, enhancing its capabilities with advanced features and implementing effective troubleshooting are crucial next steps. This section delves into these aspects to help you refine your application and ensure its robustness and reliability in handling real-time communication.
Advanced Features
Data Channels
Beyond audio and video, WebRTC supports bidirectional data channels that can be used for text chat, file transfer, or even gaming. Integrating data channels involves creating a channel during the peer connection setup and handling the onmessage event.
1const dataChannel = peerConnection.createDataChannel("myLabel", dataChannelOptions);
2
3dataChannel.onmessage = event => {
4 console.log("Data received: " + event.data);
5};
6
7dataChannel.onerror = error => {
8 console.error("Data Channel Error:", error);
9};
Data channels add versatility to your applications, enabling a wide range of interactive features beyond standard voice and video communications
Screen Sharing
This can be implemented by changing the media stream provided to the peer connection. Instead of the usual webcam feed, you can capture and send the contents of a screen or a particular window.
1navigator.mediaDevices.getDisplayMedia(screenCaptureConstraints)
2 .then(screenStream => {
3 // Replace the current video track with the screen capture track
4 const screenTrack = screenStream.getTracks()[0];
5 const sender = peerConnection.getSenders().find(s => s.track.kind === screenTrack.kind);
6 sender.replaceTrack(screenTrack);
7 });
Screen sharing is especially useful for collaborative and educational applications where sharing real-time visuals is crucial.
Troubleshooting Common Issues
Handling errors and troubleshooting common issues are vital for maintaining a seamless user experience:
Connectivity Issues
Problems such as failed connections or interrupted streams are often related to network conditions or ICE candidate processing. Ensure your ICE server configuration is robust and includes both STUN and TURN servers to maximize connection success rates across different network types.
Error Handling
Proper error handling mechanisms should be implemented throughout the application to manage unexpected issues during signaling, media capture, or data transmission.
1peerConnection.oniceconnectionstatechange = () => {
2 if (peerConnection.iceConnectionState === 'failed') {
3 /* Handle failed connection */
4 }
5};
Providing fallbacks or retries can enhance resilience, especially in varying network conditions.
Performance Optimization
Monitor performance issues related to video quality and transmission delays. Adjusting codec settings, resolution, or bitrate dynamically based on the network conditions can significantly improve the user experience.
1peerConnection.getSenders().forEach(sender => {
2 const parameters = sender.getParameters();
3 if (parameters && parameters.encodings) {
4 parameters.encodings.forEach(encoding => encoding.maxBitrate = newMaxBitrate);
5 sender.setParameters(parameters);
6 }
7});
Conclusion
Incorporating advanced features and effective troubleshooting mechanisms can significantly enhance the capability and reliability of your WebRTC application. By understanding and implementing these techniques, developers can ensure their applications deliver a high-quality, robust user experience, even under challenging conditions. Moving forward, continuous testing and optimization based on real-world usage feedback will be key to maintaining and improving the application's performance.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ