Introduction
Web Real-Time Communication
(WebRTC) is a project that aims to provide web browsers with the ability to communicate in real-time using simple APIs. It enables the transmission of video, voice, and data between peers without the need for external plugins or third-party software. This technology not only facilitates peer-to-peer communication on web pages but also enhances applications with features like video conferencing and file sharing. WebRTC is particularly beneficial for developers looking to incorporate real-time communication functionalities without the complexity of specialized server setups.Basics of Screen Sharing with WebRTC
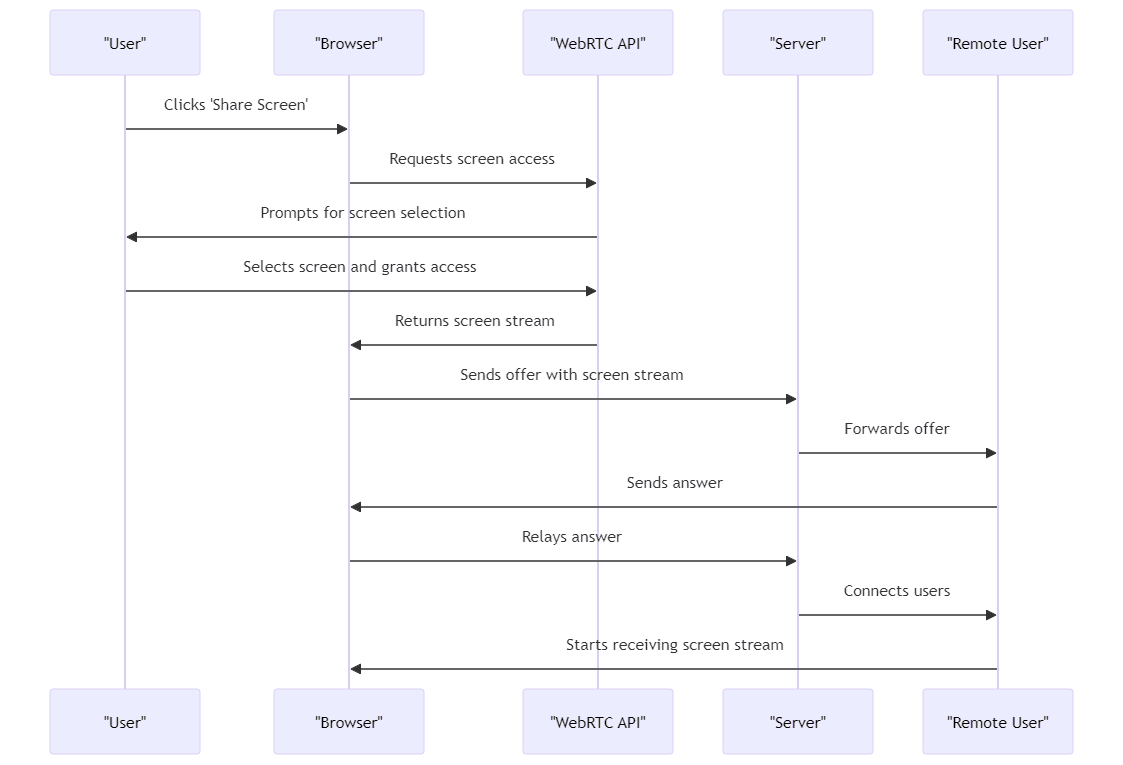
Screen sharing is a crucial aspect of WebRTC that allows users to share their screen content with others during video calls or streaming sessions. By utilizing the getDisplayMedia() method, WebRTC enables the capture of video streams from the host device's screen, which can then be shared with remote peers over the internet. This feature is essential for presentations, collaborative work, and online education, making it a valuable tool across various platforms.
Developers initiating screen sharing must configure media constraints to specify the type of media to capture. Here is a simple example:
JSX
1async function startScreenSharing() {
2 try {
3 const screenStream = await navigator.mediaDevices.getDisplayMedia({ video: true });
4 // Handle the captured stream
5 } catch (error) {
6 console.error('Error: Unable to access display media.', error);
7 }
8}
9
10
This code snippet exemplifies the process of requesting access to the video display and managing the media stream. This functionality can then be incorporated into web applications to enable live sharing.
Preparing the Environment for Screen Sharing
In order to effectively utilize WebRTC for screen sharing, developers must ensure that their environment fulfills certain prerequisites. Firstly, the application must be served over HTTPS, as modern browsers mandate a secure context for accessing powerful features such as media capture.
Additionally, it is important to note that browser support may vary, and developers should take into account the specific requirements and limitations of each browser. For example, while Chrome and Firefox actively support WebRTC APIs, including
getDisplayMedia()
, other browsers may have different levels of compatibility or feature support.Ensuring that the development environment aligns with these requirements is essential for successfully implementing WebRTC-based screen sharing. The following are the key points to verify:
- HTTPS Deployment: Secure the server to handle SSL/TLS for WebRTC.
- Browser Compatibility: Verify and test functionality across different browsers.
By adhering to these guidelines, developers can create robust applications that utilize WebRTC for real-time screen sharing, thereby enhancing user engagement and collaboration across various platforms.
Step-by-Step Guide to Implement Screen Sharing
Implementing screen sharing in a web application using WebRTC involves several crucial steps, from initializing the peer connection to handling the media stream efficiently. Here's a comprehensive guide to setting up WebRTC screen sharing:
Initialize the Peer Connection: Start by setting up a
RTCPeerConnection
, which is essential for handling the transmission of audio and video data.JSX
1const peerConnection = new RTCPeerConnection(configuration);
2
3
Capture the Screen: Utilize the
getDisplayMedia()
method to capture the screen. This API is part of the MediaDevices interface and is crucial for obtaining a media stream that contains the contents of the screen.JSX
1async function captureScreen() {
2 const displayMediaOptions = {
3 video: {
4 cursor: "always"
5 },
6 audio: false
7 };
8 return await navigator.mediaDevices.getDisplayMedia(displayMediaOptions);
9}
10
11
Add Screen Stream to Peer Connection: Once the screen is captured, the resulting stream must be added to the peer connection to be shared with other participants.
JSX
1const stream = await captureScreen();
2stream.getTracks().forEach(track => {
3 peerConnection.addTrack(track, stream);
4});
5
6
Handle Incoming and Outgoing Offers: Create and handle offers and answers to establish the connection between peers. This involves signaling, where SDP (Session Description Protocol) offers and answers are exchanged.
JSX
1peerConnection.onicecandidate = event => {
2 if (event.candidate) {
3 sendCandidateToPeer(event.candidate);
4 }
5};
6
7async function createAndSendOffer() {
8 const offer = await peerConnection.createOffer();
9 await peerConnection.setLocalDescription(offer);
10 sendOfferToPeer(offer);
11}
12
13
Establish the Connection: Complete the connection setup by handling the signaling data exchanged between peers, which includes handling ICE candidates and setting remote descriptions.
Stream Management: Manage the screen sharing stream effectively, allowing users to stop and start sharing as needed.
JSX
1function stopSharing() {
2 stream.getTracks().forEach(track => track.stop());
3}
4
5
Advanced Features and Customization
Expanding the basic functionality of WebRTC screen sharing can significantly enhance user experience, particularly in environments where collaboration and quality are critical.
Below are some advanced features and customization options that can be integrated:
Handling Multiple Participants: To support multiple participants viewing the shared screen, manage multiple peer connections or utilize a server to relay streams.
Private Rooms: Implement functionality for users to create and join private rooms, using unique identifiers for room names. This can be facilitated through URL parameters or room tokens.
JSX
1const roomName = "privateRoom123";
2const roomURL = `https://yourapp.com/${roomName}`;
3
4
Adjusting Video Quality: Control the quality of the video stream to optimize performance across different network conditions. Use constraints in the
getDisplayMedia()
call to specify resolution, frame rate, and more.JSX
1const highQualityOptions = {
2 video: {
3 width: { ideal: 1920 },
4 height: { ideal: 1080 },
5 frameRate: { ideal: 30 }
6 }
7};
8
9
Troubleshooting Common Issues
Implementing WebRTC screen sharing can come with challenges such as browser compatibility issues, network constraints, and API limitations. Here are common issues and their solutions:
- Browser Compatibility: Ensure that your application handles different levels of API support across browsers. Provide fallbacks or user instructions when certain features are unsupported.
- Network Issues: Handle poor network conditions by adjusting media stream quality dynamically based on connection speed.
- API Limitations: Understand the limitations of the
getDisplayMedia()
API, such as not being able to capture certain applications or system-level audio. Provide clear documentation to users about what can and cannot be shared.
Including these detailed steps and considerations ensures that developers can implement robust and flexible WebRTC screen sharing features in their applications, enhancing the interactivity and functionality of real-time communication platforms.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ