What is WebRTC and Signaling Servers?
Signaling is a crucial component of WebRTC, responsible for managing the control information exchanged between peers to establish, maintain, and terminate peer-to-peer communication. Signaling servers act as intermediaries to relay signaling messages between clients.
What is WebRTC and Signaling Servers?
Signaling is a crucial component of
WebRTC
, responsible for managing the control information exchanged between peers to establish, maintain, and terminate peer-to-peer communication. Think of it as the behind-the-scenes negotiation that allows your audio, video, and data streams to flow smoothly. Unlike these media streams, which travel directly between peers, signaling messages don't adhere to a standardized protocol within WebRTC itself. Instead, developers need to implement their own signaling mechanism, often leveraging existing communication protocols such asSIP
(Session Initiation Protocol), XMPP (Extensible Messaging and Presence Protocol), orWebSockets
. These protocols facilitate the vital exchange of information that makes WebRTC possible. The primary functions of signaling include:- Session Initiation: Establishing the initial connection by exchanging offer and answer messages. These messages contain session descriptions (SDP), which define the media capabilities of each peer.
- Network Information Exchange: Sharing ICE (Interactive Connectivity Establishment) candidates. ICE candidates provide network addresses, allowing for NAT traversal and helping peers find the best route for direct communication.
- Session Management: Handling changes to the session, such as adding or removing media streams or adjusting bandwidth. This also includes error handling and renegotiation.
Without a robust signaling process, peers wouldn't be able to locate each other or agree on the parameters for the communication session. Therefore, signaling servers play a critical role in WebRTC, acting as intermediaries to relay signaling messages between clients. To further illustrate, imagine User A wants to call User B. The signaling server facilitates this by relaying the connection request and the negotiation of session details between them.
What is WebRTC Signaling Servers?
A WebRTC signaling server is a server-side application that manages the exchange of signaling messages between clients. Its primary purpose is to ensure that peers can discover each other and negotiate the connection parameters required to establish a peer-to-peer communication channel. While the specific implementation of a signaling server can vary, the core function remains the same: facilitating the setup and management of WebRTC sessions.
How do WebRTC Signaling Servers Work? They act as a temporary meeting point, allowing peers to exchange information necessary to establish a direct connection. Once the connection is established, the signaling server is no longer directly involved in the media stream, enabling efficient peer-to-peer communication.
Common protocols used for signaling in WebRTC include:
- SIP (Session Initiation Protocol): Originally designed for initiating, maintaining, and terminating real-time sessions across IP networks. SIP is often used in VoIP applications. An advantage of SIP is its widespread adoption and feature-richness. A disadvantage can be its complexity compared to other protocols.
- XMPP (Extensible Messaging and Presence Protocol): An XML-based protocol used for instant messaging and presence information. XMPP's strengths lie in its extensibility and decentralized nature, making it suitable for various applications. A potential drawback is its XML-based format, which can lead to larger message sizes.
- WebSockets: A communication protocol providing full-duplex communication channels over a single TCP connection, often used for real-time web applications. WebSockets offer low latency and efficient communication, making them a popular choice for WebRTC signaling.
Setting Up a WebRTC Signaling Server
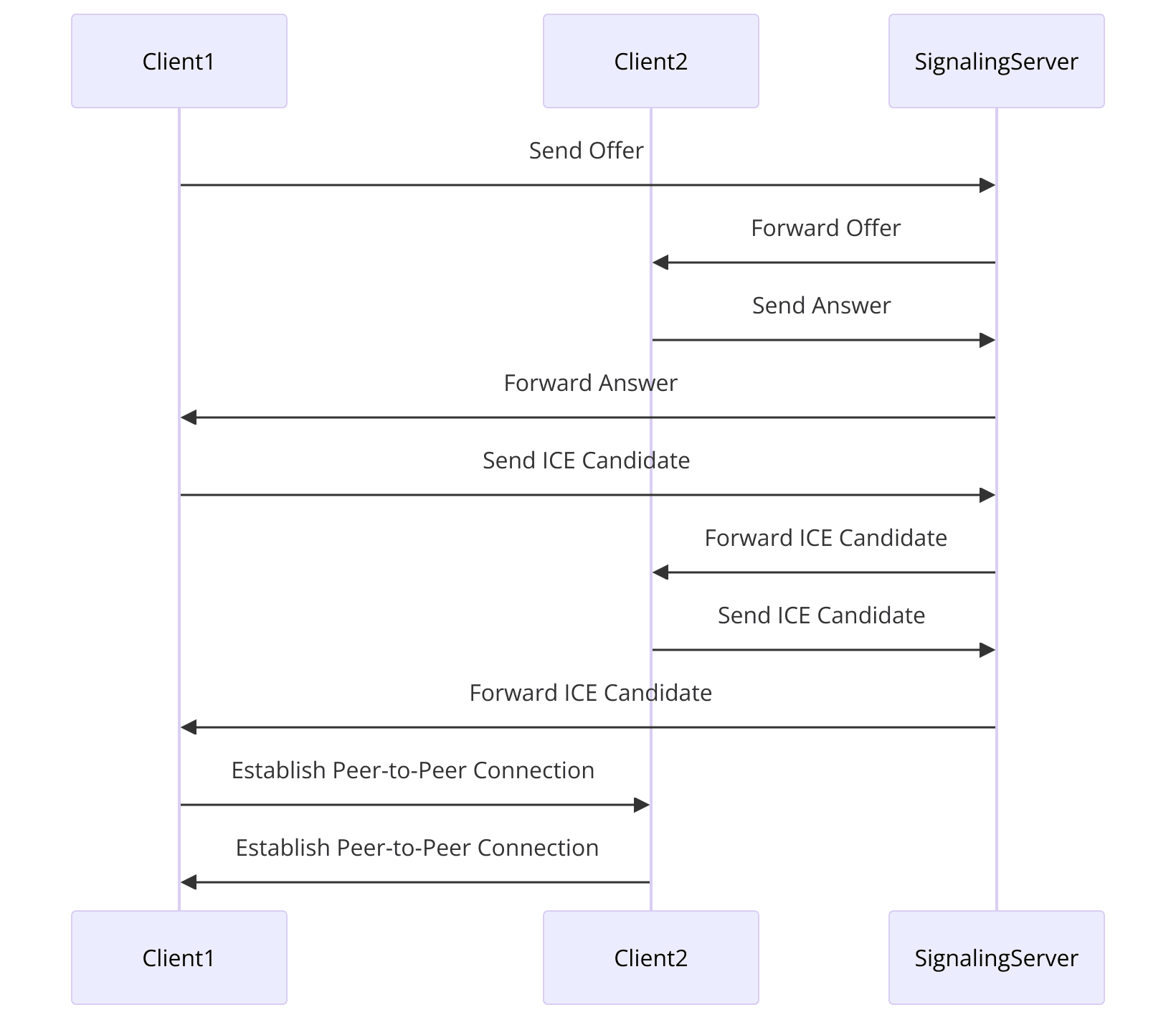
The workflow of a WebRTC signaling server typically involves:
- Client Registration: Clients connect to the signaling server and register their presence, often providing information like a unique identifier or username.
- Session Initiation: A client (the caller) sends an offer to the signaling server, which then forwards it to the intended recipient (the callee).
- Session Negotiation: The callee responds with an answer, and both clients exchange ICE candidates to find the optimal communication path, handling NAT traversal challenges.
- Session Management: The signaling server relays any additional messages required to manage the session, such as re-negotiation requests or end-of-call notifications.
Below is a simple example of a WebRTC signaling server implemented using WebSockets in Node.js:
1const WebSocket = require('ws');
2const wss = new WebSocket.Server({ port: 8080 });
3
4wss.on('connection', function connection(ws) {
5 ws.on('message', function incoming(message) {
6 // Broadcast to everyone else.
7 wss.clients.forEach(function each(client) {
8 if (client !== ws && client.readyState === WebSocket.OPEN) {
9 client.send(message);
10 }
11 });
12 });
13});
14
15console.log('WebSocket server is running on ws://localhost:8080');
16
This simple server listens for incoming WebSocket connections, and when a message is received, it broadcasts the message to all other connected clients. This basic setup can be expanded with additional logic to handle different types of signaling messages and improve security.
Choosing the Right Signaling Server
When setting up a WebRTC signaling server, it's essential to choose the right solution that fits your project's requirements. There are several factors to consider:
- Scalability: The server should handle multiple concurrent connections efficiently. Consider architectures like centralized (simple to manage but single point of failure) versus distributed (more complex, but higher reliability and scalability).
- Security: Ensure that the signaling server supports robust security measures, including encryption and authentication. Encryption of signaling messages is crucial to protect sensitive data.
- Compatibility: The server should be compatible with various WebRTC clients and protocols.
- Ease of Use: Look for servers with comprehensive documentation and active community support.
WebRTC Signaling Server Setup and Configuration
Setting up a WebRTC signaling server involves installing the necessary software and configuring it to handle signaling messages. Below is a step-by-step guide to get started. For this example, we'll use Node.js and the
ws
library to set up a basic WebRTC signaling server with WebSockets.Step-1: Install Node.js:
Ensure that you have Node.js installed. You can download it from
Node.js
.Step-2: Create a New Project:
Initialize a new Node.js project.
1mkdir webrtc-signaling-server
2cd webrtc-signaling-server
3npm init -y
4
Step-3: Install WebSocket Library:
Install the
ws
library for WebSocket support.1npm install ws
2
Initial Configuration Steps
Step-1: Create Server File:
Create a file named
server.js
and add the following code to set up a basic WebSocket server.1 const WebSocket = require('ws');
2 const wss = new WebSocket.Server({ port: 8080 });
3
4 wss.on('connection', function connection(ws) {
5 ws.on('message', function incoming(message) {
6 // Broadcast to everyone else.
7 wss.clients.forEach(function each(client) {
8 if (client !== ws && client.readyState === WebSocket.OPEN) {
9 client.send(message);
10 }
11 });
12 });
13 });
14
15 console.log('WebSocket server is running on ws://localhost:8080');
16
Step-2: Run the Server:
Start the WebSocket server by running:
bash
node server.js
Here's the complete code for a basic WebSocket signaling server:
1const WebSocket = require('ws');
2const wss = new WebSocket.Server({ port: 8080 });
3
4wss.on('connection', function connection(ws) {
5 ws.on('message', function incoming(message) {
6 // Broadcast to everyone else.
7 wss.clients.forEach(function each(client) {
8 if (client !== ws && client.readyState === WebSocket.OPEN) {
9 client.send(message);
10 }
11 });
12 });
13});
14
15console.log('WebSocket server is running on ws://localhost:8080');
16
This simple WebSocket server listens for connections on port 8080 and broadcasts any received messages to all connected clients, facilitating the exchange of signaling messages necessary for WebRTC connections.
Implementing WebRTC Signaling with WebSockets
WebSockets are a popular choice for implementing WebRTC signaling due to their low latency and full-duplex communication capabilities. This section provides a detailed example of how to implement signaling with WebSockets.
Why Use WebSockets?
WebSockets provide a persistent connection between the client and server, allowing for real-time communication with minimal latency. This is ideal for signaling, where timely exchange of messages is crucial for establishing and maintaining WebRTC connections.
Below is a more detailed example of a WebRTC signaling server using WebSockets, with added functionality to handle different types of signaling messages.
1const WebSocket = require('ws');
2const wss = new WebSocket.Server({ port: 8080 });
3
4wss.on('connection', function connection(ws) {
5 ws.on('message', function incoming(message) {
6 const data = JSON.parse(message);
7
8 switch (data.type) {
9 case 'offer':
10 handleOffer(data, ws);
11 break;
12 case 'answer':
13 handleAnswer(data, ws);
14 break;
15 case 'candidate':
16 handleCandidate(data, ws);
17 break;
18 default:
19 console.log('Unknown message type:', data.type);
20 }
21 });
22});
23
24function handleOffer(data, ws) {
25 wss.clients.forEach(function each(client) {
26 if (client !== ws && client.readyState === WebSocket.OPEN) {
27 client.send(JSON.stringify({
28 type: 'offer',
29 offer: data.offer,
30 from: data.from,
31 to: data.to
32 }));
33 }
34 });
35}
36
37function handleAnswer(data, ws) {
38 wss.clients.forEach(function each(client) {
39 if (client !== ws && client.readyState === WebSocket.OPEN) {
40 client.send(JSON.stringify({
41 type: 'answer',
42 answer: data.answer,
43 from: data.from,
44 to: data.to
45 }));
46 }
47 });
48}
49
50function handleCandidate(data, ws) {
51 wss.clients.forEach(function each(client) {
52 if (client !== ws && client.readyState === WebSocket.OPEN) {
53 client.send(JSON.stringify({
54 type: 'candidate',
55 candidate: data.candidate,
56 from: data.from,
57 to: data.to
58 }));
59 }
60 });
61}
62
63console.log('WebSocket signaling server is running on ws://localhost:8080');
64
Advanced Signaling Server Concepts
A robust WebRTC signaling server must efficiently handle various types of signaling messages. The primary message types are offers, answers, and ICE candidates. Understanding how to manage these messages is crucial for establishing and maintaining WebRTC peer-to-peer communication.
Types of Messages
- Offer: A session description sent by the caller to initiate a connection.
- Answer: A session description sent by the callee in response to an offer.
- ICE Candidate: Network information that helps peers establish a direct connection.
Parsing and Responding to Messages
Each type of message has a specific format and purpose. Properly parsing and responding to these messages ensures that peers can establish a reliable connection.
Handling Offer Messages
When a client sends an offer, the signaling server needs to forward it to the intended recipient. Here’s an example of how to handle an offer message:
1function handleOffer(data, ws) {
2 wss.clients.forEach(function each(client) {
3 if (client !== ws && client.readyState === WebSocket.OPEN) {
4 client.send(JSON.stringify({
5 type: 'offer',
6 offer: data.offer,
7 from: data.from,
8 to: data.to
9 }));
10 }
11 });
12}
13
Handling Answer Messages
Answer messages are handled similarly to offer messages. The server forwards the answer to the original offer sender:
1function handleAnswer(data, ws) {
2 wss.clients.forEach(function each(client) {
3 if (client !== ws && client.readyState === WebSocket.OPEN) {
4 client.send(JSON.stringify({
5 type: 'answer',
6 answer: data.answer,
7 from: data.from,
8 to: data.to
9 }));
10 }
11 });
12}
13
Handling ICE Candidate Messages
ICE candidates need to be exchanged between peers to facilitate network traversal. Here’s how to handle ICE candidate messages:
1function handleCandidate(data, ws) {
2 wss.clients.forEach(function each(client) {
3 if (client !== ws && client.readyState === WebSocket.OPEN) {
4 client.send(JSON.stringify({
5 type: 'candidate',
6 candidate: data.candidate,
7 from: data.from,
8 to: data.to
9 }));
10 }
11 });
12}
13
Security Considerations
Security is a critical aspect of any WebRTC application. Ensuring that your signaling server and the WebRTC connections it facilitates are secure is vital to protect user data and maintain privacy.
Authentication and Authorization
Implementing authentication and authorization mechanisms ensures that only legitimate users can connect to your signaling server.
Token-based Authentication
1const jwt = require('jsonwebtoken');
2const secretKey = 'your_secret_key';
3
4wss.on('connection', function connection(ws, req) {
5 const token = req.url.split('?token=')[1];
6 jwt.verify(token, secretKey, (err, decoded) => {
7 if (err) {
8 ws.close();
9 } else {
10 // Proceed with connection
11 }
12 });
13});
14
Encrypting Signaling Data
Using secure WebSocket connections (wss://) ensures that signaling data is encrypted in transit.
1const https = require('https');
2const fs = require('fs');
3const WebSocket = require('ws');
4
5const server = https.createServer({
6 cert: fs.readFileSync('path/to/cert.pem'),
7 key: fs.readFileSync('path/to/key.pem')
8});
9
10const wss = new WebSocket.Server({ server });
11
12server.listen(8080);
13
Scalability and Performance Optimization
As your WebRTC application grows, ensuring that your signaling server can handle increased load becomes crucial. Scalability and performance optimization techniques are essential to maintain service quality. What are the different options for building a signaling server that scales?
Load Balancing Techniques
Distributing the load across multiple servers helps manage high traffic and ensures redundancy. We can use NGINX for Load Balancing.
1http {
2 upstream signaling_servers {
3 server signaling1.example.com;
4 server signaling2.example.com;
5 }
6
7 server {
8 listen 80;
9
10 location / {
11 proxy_pass http://signaling_servers;
12 proxy_set_header Host $host;
13 proxy_set_header X-Real-IP $remote_addr;
14 proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
15 proxy_set_header X-Forwarded-Proto $scheme;
16 }
17 }
18}
19
Explore our other resources to learn more about WebRTC and how to enhance your communication solutions!
Future Trends in WebRTC Signaling
As WebRTC continues to evolve, several trends are emerging that will shape the future of real-time communication.
Emerging Technologies
- 5G Networks: The deployment of 5G networks will significantly enhance the performance of WebRTC applications, providing lower latency and higher bandwidth.
- Edge Computing: By processing data closer to the source, edge computing can reduce latency and improve the performance of WebRTC applications.
Potential Innovations
- AI and Machine Learning: AI and machine learning can enhance WebRTC applications by providing advanced features like real-time speech recognition, noise suppression, and video enhancement.
- Enhanced Security Protocols: The development of new security protocols will help protect WebRTC applications from emerging threats.
Industry Predictions
- Increased Adoption: The adoption of WebRTC will continue to grow across various industries, including healthcare, education, and entertainment.
- Standardization Efforts: Ongoing efforts to standardize WebRTC protocols and practices will lead to more robust and interoperable solutions.
Conclusion
By exploring these additional resources and external links, developers can gain a deeper understanding of WebRTC signaling servers and best practices for implementation. These resources are essential for building robust, secure, and scalable real-time communication applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ