Understanding WebRTC Latency: Causes, Solutions, and Optimization Techniques
Learn all about WebRTC latency, its causes, and how to optimize real-time communication for better performance. Discover techniques to reduce latency, measure performance, and implement best practices for WebRTC applications.
Introduction to WebRTC and Latency
WebRTC
(Web Real-Time Communication) is an open-source project that provides web applications and websites with real-time communication capabilities via simple application programming interfaces (APIs). This technology enables peer-to-peer connections, allowing audio, video, and data sharing between users directly in their web browsers without needing plugins or additional software. WebRTC consists of several core components:- GetUserMedia API: Captures media (audio and video) from the user's device.
- RTCPeerConnection API: Manages the peer-to-peer connection, handling the setup, maintenance, and closure of connections.
- RTCDataChannel API: Facilitates the direct exchange of data between peers, such as text messages or files.
How Latency Affects WebRTC Performance
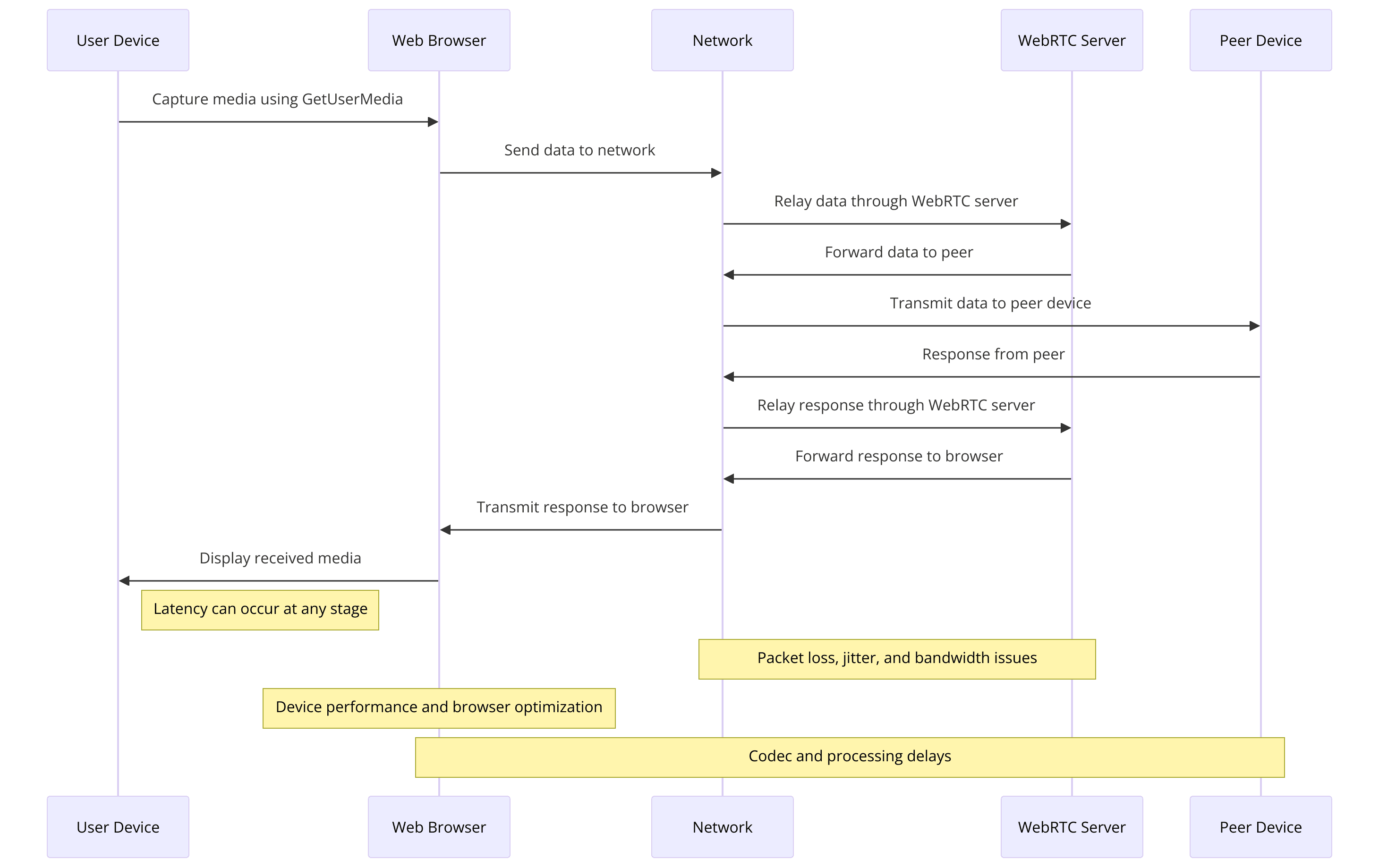
Latency refers to the time delay between the transmission of data and its reception. In the context of WebRTC, latency is the delay experienced during real-time communication, such as the lag between speaking and the other person hearing you in a
video call
.Latency is a critical factor in WebRTC performance. High latency can disrupt the natural flow of conversation, making it difficult for participants to communicate effectively. In applications like gaming and live streaming, high latency can lead to poor user experiences, with delayed responses and interruptions. There are several factors that contribute to latency in WebRTC applications.
Network-Related Latency Issues
Network conditions play a significant role in WebRTC latency. Several network-related factors can contribute to increased delays:
Packet Loss
Packet loss occurs when data packets traveling over the network fail to reach their destination. In WebRTC, packet loss can lead to interruptions in audio and video streams, causing noticeable delays and degradation in communication quality.
Jitter
Jitter refers to the variability in packet arrival times. High jitter can cause packets to arrive out of order, leading to delays as the system attempts to reassemble the data correctly.
Bandwidth Limitations
Insufficient bandwidth can cause congestion and slow down the transmission of audio and video streams. Ensuring adequate bandwidth is crucial for minimizing WebRTC latency and maintaining high-quality communication.
Device and Browser-Specific Latency
The performance of the devices and browsers used for WebRTC applications can also impact latency. Factors include:
Device Performance
Older or less powerful devices may struggle with the computational demands of
real-time audio and video
processing. This can introduce delays as the device attempts to keep up with the data flow.Browser Optimization
Different web browsers may handle WebRTC APIs differently. Some browsers may have optimizations that reduce latency, while others might introduce additional delays. Keeping browsers updated to the latest versions can help mitigate these issues.
Codec and Processing Delays
The choice of codecs and the processing power required to encode and decode audio and video streams are significant contributors to WebRTC latency:
Audio and Video Codecs
Different codecs have different processing requirements and efficiency levels. For instance, the VP8 and H.264 video codecs are commonly used in WebRTC, but their performance can vary based on the implementation and the hardware used.
Buffer Management
Buffers are used to smooth out variations in packet arrival times. However, large buffer sizes can introduce additional delays as data is queued before being processed. Balancing buffer size to minimize latency while maintaining stream quality is essential.
Measuring WebRTC Latency
Accurately measuring WebRTC latency is crucial for identifying performance bottlenecks and implementing effective optimizations. Several tools and techniques can be used to measure latency:
WebRTC Internals
Most modern browsers provide built-in tools, such as Chrome’s WebRTC Internals, that allow developers to inspect the details of WebRTC connections. These tools can provide insights into latency, jitter, and packet loss.
Third-Party Tools
There are various third-party tools available that can help measure WebRTC latency. Tools like TestRTC, Callstats.io, and Wireshark offer comprehensive analysis capabilities to monitor and troubleshoot WebRTC performance.
Real-World Measurement Examples
To provide practical guidance, here are examples of measuring WebRTC latency using common tools:
Using WebRTC Internals in Chrome
- Open a WebRTC application in Chrome.
- Access WebRTC Internals by navigating to
chrome://webrtc-internals/
. - Start a call and observe the various metrics displayed, including latency, jitter, and packet loss.
Using TestRTC
- Set up a test scenario in TestRTC.
- Run the test and analyze the results, focusing on the latency metrics provided.
By regularly measuring and analyzing WebRTC latency, developers can identify areas for improvement and implement targeted optimizations to reduce delays and enhance communication quality.
Techniques to Reduce WebRTC Latency
Network Optimization Techniques
Optimizing the network is one of the most effective ways to reduce WebRTC latency. Here are some key strategies:
Reducing Packet Loss
Implement techniques such as Forward Error Correction (FEC) and Automatic Repeat reQuest (ARQ) to mitigate packet loss. These methods help recover lost packets and ensure the integrity of the data being transmitted.
Managing Bandwidth
Allocate sufficient bandwidth for WebRTC applications. Use traffic shaping and Quality of Service (QoS) settings to prioritize real-time communication traffic over less critical data.
Minimizing Jitter
Use jitter buffers to smooth out the variability in packet arrival times. Although buffers can introduce some delay, they are essential for maintaining stream quality. Tuning the size of jitter buffers helps balance latency and quality.
Browser and Device Optimization
Optimizing the devices and browsers used for WebRTC can significantly reduce latency:
Device Optimization
Ensure devices used for WebRTC are powerful enough to handle real-time communication demands. Using devices with better processors and more memory can help reduce latency.
Browser Updates
Keep browsers updated to the latest versions to take advantage of performance improvements and bug fixes related to WebRTC. Different browsers may have varying levels of optimization for WebRTC, so testing across multiple browsers can identify the best options for low latency.
Codec and Buffer Management
Effective management of codecs and buffers is crucial for minimizing WebRTC latency:
Selecting the Right Codecs
Choose codecs that offer a good balance between quality and processing efficiency. For instance, the VP8 codec is widely used for video due to its efficiency, while Opus is a popular choice for audio due to its low latency.
Optimizing Buffer Sizes
Adjust the size of audio and video buffers to minimize latency. Smaller buffers reduce delay but may increase the risk of jitter, while larger buffers smooth out jitter but add delay. Finding the optimal buffer size is key to reducing latency while maintaining stream quality.
Here's a configuring a WebRTC connection to optimize for low latency:
JavaScript
1const configuration = {
2 iceServers: [{ urls: 'stun:stun.l.google.com:19302' }],
3 iceTransportPolicy: 'relay',
4 bundlePolicy: 'max-bundle',
5 rtcpMuxPolicy: 'require',
6 sdpSemantics: 'unified-plan'
7};
8
9const pc = new RTCPeerConnection(configuration);
10
11// Setting low latency preferences
12const transceiver = pc.addTransceiver('video');
13transceiver.setCodecPreferences([
14 { mimeType: 'video/VP8' },
15 { mimeType: 'video/H264' }
16]);
17
18// Configuring jitter buffer size
19pc.getSenders().forEach(sender => {
20 const params = sender.getParameters();
21 params.encodings.forEach(encoding => {
22 encoding.maxBitrate = 500000; // Set max bitrate to ensure quality
23 encoding.minBitrate = 300000; // Set min bitrate to ensure stability
24 encoding.bitratePriority = 1; // High priority for bitrate
25 encoding.networkPriority = 1; // High priority for network
26 });
27 sender.setParameters(params);
28});
29
By selecting efficient codecs and adjusting encoding parameters, developers can achieve a balance between quality and responsiveness.
Advanced Use Cases of WebRTC with Low Latency
Real-Time Gaming
WebRTC’s low latency capabilities make it ideal for real-time gaming applications. In multiplayer online games, players' actions need to be synchronized in real-time to ensure a seamless and competitive experience. High latency can result in lag, which can be detrimental to gameplay. By leveraging WebRTC, developers can create immersive and responsive gaming environments where players interact with minimal delays.
Interactive Broadcasting
Interactive broadcasting is another advanced use case where WebRTC's low latency is crucial. In live streaming scenarios, such as online education, live sports commentary, and virtual events, minimizing latency is essential for maintaining engagement and interaction with the audience. Delays can disrupt the flow of communication and reduce the effectiveness of the broadcast.
Remote Collaboration Tools
Remote collaboration tools have become increasingly important, especially with the rise of remote work. WebRTC powers many of these tools, providing the real-time audio and video communication needed for effective collaboration. Low latency is critical in these applications to ensure that conversations and interactions are smooth and natural.
Conclusion
WebRTC latency is a crucial factor in the performance and quality of real-time communication applications. Understanding and addressing the causes of latency can lead to significant improvements in user experience. By understanding these aspects and applying the recommended techniques, developers can optimize their WebRTC applications to deliver seamless and responsive real-time communication experiences.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ