Twilio WebRTC
Twilio WebRTC goes further to extend the standard core features, ensuring the provision of advanced business- and developer-focused communication solutions. That is, developers can use Twilio APIs to add scalable, secure video and voice calling features to applications with the least time possible. Twilio takes the pain out of managing the complexities of browser-based communication in real-time, dealing with the signaling, session management, and media exchange.
- Twilio implementation: End-to-end features for developers to ensure they have the best developer experience, delivering peak performance to your end-users.
- Global Low Latency: Twilio performs real-time optimization of the path connection, guaranteeing that the world experiences the least latency for clear quality communication even if great geographical distance exists.
- Scalability: Developers are able to scale their applications with the growing number of users using Twilio's cloud infrastructure without compromising performance or stability.
- Security: The Twilio WebRTC comes with robust industry-standard encryption that covers secure communication against interception and access by unauthorized persons
- Cross-platform Support: Twilio makes it simple to have browsing from every platform through its WebRTC solutions.
Twilio WebRTC not only enhances your real-time communication abilities but also integrates smoothly with other Twilio services like Programmable SMS and Voice to make all this possible. They can leverage Twilio's WebRTC capabilities so that software application developers can provide full-spectrum solutions for communication by being in a position to put voice, video, text, and even sophisticated IVR systems in the applications they develop. "Twilio sees its approach to WebRTC as that which removes barriers for developers by giving them tools and support to most effectively build advanced, real-time communication solutions.".
No matter if you are developing an app for video calls or an enterprise-level communication platform, Twilio has every tool and the documentation you might need—from day one to a successful deployment.
In the following sections, we are going to dig deeper into the capabilities and use cases of Twilio WebRTC in JavaScript, zeroing in on how businesses in diverse industries leverage the technology to enhance their operation and provide users with great experiences.
Setting Up the Development Environment
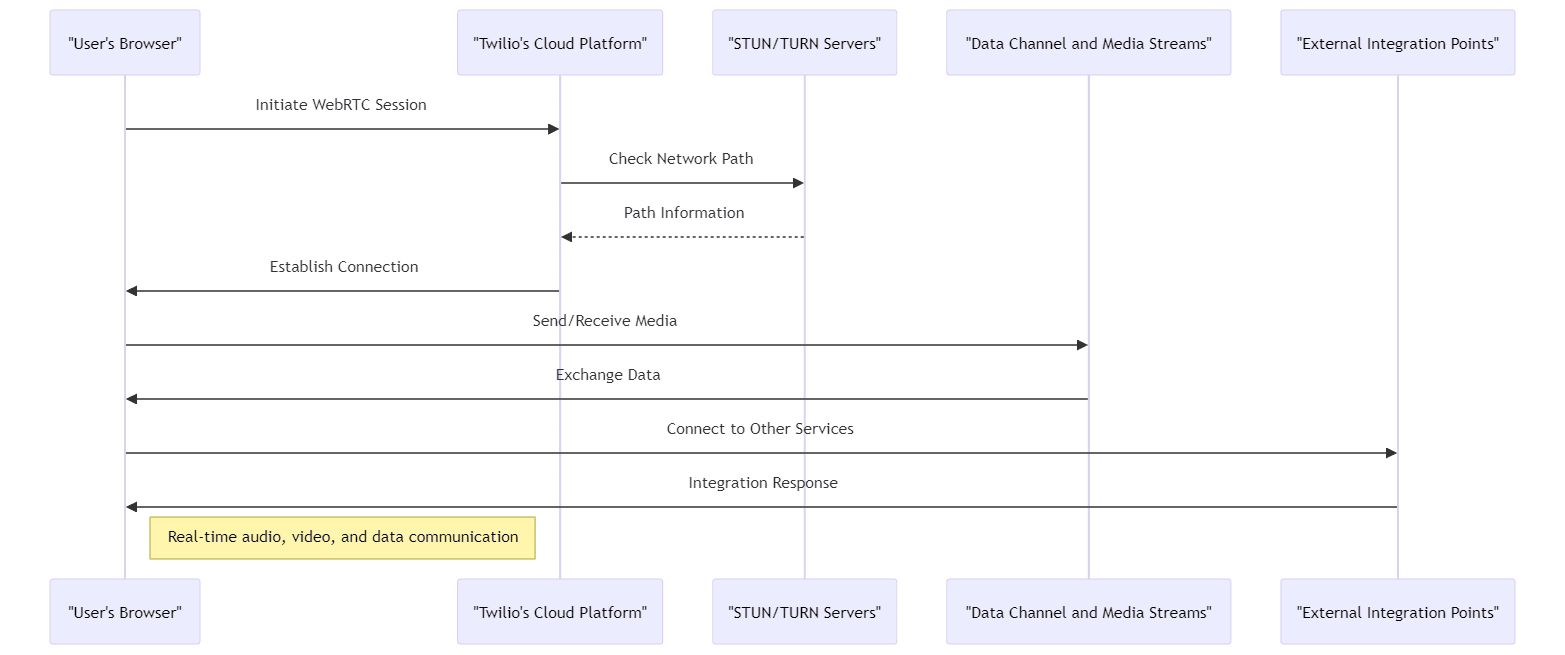
Before diving into the exciting world of building applications with Twilio WebRTC, it's crucial to set up a JS development environment that includes all the necessary tools and configurations. To begin, developers will need to ensure they have Node.js installed—a fundamental requirement for managing server-side scripting and dependencies.
- Installation of Node.js and npm: Start by downloading and installing Node.js from
nodejs.org
. This installation will also include npm (Node Package Manager), essential for managing JavaScript packages. - Creating a Project Directory: Organize your project by creating a new directory specifically for your WebRTC application. This can be done through simple commands in your command line interface (CLI).
- Integrating Twilio SDK: Utilize npm to install the Twilio SDK, which simplifies the use of Twilio APIs within your JavaScript code. This SDK is vital for implementing the Twilio-specific features of your WebRTC application.
bash
1mkdir my-twilio-webrtc-app
2cd my-twilio-webrtc-app
3npm install twilio
4
With your environment ready, you can begin crafting your application, focusing on integrating Twilio’s robust WebRTC capabilities.
Building a Basic Video Chat Application
Creating a basic video chat application is an excellent way to understand the fundamentals of Twilio WebRTC. This section outlines the steps and code snippets required to build a functioning video communication tool.
Setting Up the Front-End
- HTML: Start with a simple HTML template to host your video streams. Use video tags to display the local and remote video feeds.
- CSS/JavaScript:Enhance the interface with CSS for styling and JavaScript for handling the Twilio WebRTC functionalities.
HTML
1<html>
2<head>
3 <meta charset="UTF-8">
4 <title>Simple Twilio WebRTC Video Chat</title>
5</head>
6<body>
7 <video id="local-video" autoplay></video>
8 <video id="remote-video" autoplay></video>
9 <script src="app.js"></script>
10</body>
11</html>
12
Back-End Setup and Token Management
- Utilize Node.js to create a server that generates access tokens, which are crucial for authentication with the Twilio API.
- Implement routes that serve the necessary HTML and JavaScript files and handle token generation.
JavaScript
1const express = require('express');
2const { twilio } = require('twilio');
3
4const app = express();
5const AccessToken = twilio.jwt.AccessToken;
6const VideoGrant = AccessToken.VideoGrant;
7
8app.get('/', (req, res) => {
9 res.sendFile('index.html', { root: __dirname });
10});
11
12app.get('/token', (req, res) => {
13 const token = new AccessToken(
14 process.env.TWILIO_ACCOUNT_SID,
15 process.env.TWILIO_API_KEY_SID,
16 process.env.TWILIO_API_KEY_SECRET
17 );
18 token.addGrant(new VideoGrant());
19 res.send({ token: token.toJwt() });
20});
21
22const server = app.listen(3000, () => {
23 console.log('Server is running on port 3000');
24 });
25
Connecting to Twilio WebRTC
1- Use the Twilio JavaScript library to connect to the WebRTC services, handle media devices, and establish peer connections.
2- Implement event handlers to manage video stream attachments and call setup or disconnection.
JavaScript
1const { connect } = require('twilio-video');
2document.getElementById('join-call').addEventListener('click', async () => {
3 const response = await fetch('/token');
4 const { token } = await response.json();
5 const room = await connect(token, { name: 'my-first-twilio-room' });
6
7 room.participants.forEach(participantConnected);
8 room.on('participantConnected', participantConnected);
9
10 room.on('participantDisconnected', participantDisconnected);
11 window.addEventListener('beforeunload', () => room.disconnect());
12 function participantConnected(participant) {
13 console.log(`Participant ${participant.identity} connected.`);
14 participant.tracks.forEach(publication => {
15 if (publication.isSubscribed) {
16 const track = publication.track;
17 document.getElementById('remote-video').appendChild(track.attach());
18 }
19 });
20 }
21});
22
This step-by-step guide not only introduces you to the basics of setting up a Twilio WebRTC application but also dives into practical implementation, providing a solid foundation for more complex projects. As you expand your application, consider integrating additional Twilio services to enhance its capabilities and exploring advanced features discussed in the next section.
Advanced Features and Capabilities of Twilio WebRTC
Twilio WebRTC doesn't stop with the limit of simple real-time communication, but it goes a step ahead to provide a wider suite that comprises advanced features for sophisticated development needs. Here are the details of some of the advanced capabilities from Twilio WebRTC:
Data Channel for Real-Time Data Exchange
WebRTC, through Twilio, supports the building of data channels that transmit all types of data bidirectionally and in real time between peers. This is very key when dealing with applications that require voice and video as well, and to be able to allow exchange of textual data, files, or even gaming elements between the same two peers.
That implies, from now on, developers will be able to expand the interactivity scope of applications by these data channels. For example, in a video conference, they would be able to incorporate facilities for file sharing or chat messages.
JavaScript
1// Example: Establishing a data channel
2const dataChannel = peerConnection.createDataChannel("myDataChannel");
3dataChannel.onmessage = function(event) {
4 console.log("Data received: " + event.data);
5};
6dataChannel.send("Hello, peer!");
7
Utilizing STUN/TURN Servers for Network Traversal
Handling network constraints is a major challenge in real-time communications. Twilio WebRTC utilizes STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers to facilitate communication across different types of networks and firewalls.
These servers help in discovering the public IP addresses and managing the connections needed for direct peer-to-peer communication, thus ensuring connectivity stability and reliability.
Scalability and Session Management
Twilio WebRTC provides scalable solutions that adapt to varying loads, which is crucial for applications expecting a high number of users.
Session management is streamlined, with robust APIs that handle sessions efficiently, ensuring smooth user experiences even during high-traffic periods.
Integrating with Other Twilio Services
Twilio's ecosystem offers various services that can be seamlessly integrated with WebRTC to create more dynamic and versatile applications:
Programmable SMS and Voice
Integrate Twilio Programmable SMS to send alerts or notifications directly from your WebRTC app, enhancing the communication capabilities beyond the browser.
Twilio’s Programmable Voice can be incorporated to allow WebRTC applications to connect with traditional phone systems, bridging internet-based and standard telephony communication.
JavaScript
1// Example: Sending an SMS from a WebRTC app
2const client = require('twilio')(accountSid, authToken);
3client.messages
4 .create({
5 body: 'Meeting starts in 5 minutes',
6 from: '+12345678901',
7 to: '+10987654321'
8 })
9 .then(message => console.log(message.sid));
10
Twilio Studio
Twilio Studio can be used to build visual workflows that enhance the WebRTC application’s logic, enabling non-developers to design complex call flows and interaction sequences.
This integration allows for the addition of interactive voice responses (IVRs) and automated workflows directly within the WebRTC interface, making it incredibly powerful for customer service applications.
These advanced features and integrations highlight Twilio WebRTC’s capabilities not just as a tool for simple video or voice calls but as a comprehensive platform for building complex, interactive, and highly scalable communication solutions.
In the next section, we will explore real-world applications and case studies to see how various industries leverage these technologies to enhance their operations and provide exceptional user experiences.
Real-world Applications and Case Studies of Twilio WebRTC
Twilio WebRTC has that flexibility, making its use across many industries enhance better communication and operational effectiveness. The following illustrative case studies show how Twilio WebRTC is applied across various industries.
Health services
Telemedicine has transformed the provision of health services, especially in patient outreach services. With Twilio WebRTC, clinics can provide live video consultation from the patient to the health service provider. This kind of integration allows making both the prescription management with immediate medical advice and follow-up visits without the actual necessity to travel physically, therefore ensuring the chance for continued care even from a distance.
Education
The educational platforms use Twilio WebRTC in the powering of their virtual classroom. This enables students taking their lessons and teachers offering their services to feel in one room as the educational lesson sessions take place. It includes features like real-time data channels to allow sharing educational materials and getting instant feedback on assignments to make learning even better.
Customer Service
Many businesses use Twilio WebRTC to enable interactions for the customer service face to face. This human touch can make a big difference in the obtained increased level of customer satisfaction and loyalty by faster problem resolution and service delivery.
Financial Services
Banks and financial institutions use Twilio WebRTC to make easier and secure the remote interaction process in customer-related activities, like opening new accounts or availing themselves of financial advice through video calling. This technology offers customers the convenience and security standard compliances.
Conclusion
A technology that can easily befit the organizational systems and favorite-rated by most organizations ready to improve their effectiveness in digital interaction. Businesses and organizations continue to explore effective, reliable, and scalable communication solutions. Twilio WebRTC is proving to be a game-changing technology, rather universal in its adaptation across industries for their different needs and challenges.
Whether it's frictionless patient experiences in healthcare, interactive classroom environments in education, or world-class customer service in retail, Twilio WebRTC puts the capability in business' hands to win in a digital-first world.
In adopting Twilio WebRTC, companies are deploying not just the new technology but, in essence, investing in a future where communications are dynamic, real-time, and central to the digital experience. It's these kinds of technologies that are sure to be at the center stage in shaping the next generation of digital communication.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ