Introduction to Websocket
In the realm of modern web development, real-time communication is becoming increasingly vital. This is where Websockets come into play. Websockets provide a full-duplex communication channel over a single, long-lived connection, which allows for interactive and real-time communication between a client and a server. Unlike the traditional HTTP protocol, which follows a request-response model, Websockets enable a persistent connection where data can flow freely in both directions without the overhead of establishing multiple HTTP connections.
Websockets are particularly useful in applications that require real-time data updates, such as chat applications, online gaming, live notifications, and collaborative tools. This technology bridges the gap between traditional web communication methods and the need for real-time data exchange, offering a more efficient and responsive user experience. In this article, we will delve into what Websockets are, how they work, and how you can implement them in your web applications.
What is a Websocket?
A Websocket is a communication protocol that provides a full-duplex channel over a single, long-lived connection. Unlike HTTP, which is a stateless request-response protocol, Websockets allow for continuous, bidirectional communication between a client and a server. This means that once a Websocket connection is established, data can be sent and received simultaneously, without the need for repeated handshakes.
The key features of Websockets include low latency, reduced overhead, and the ability to push updates to clients instantly. These benefits make Websockets ideal for applications requiring real-time updates, such as live chat applications, online gaming, live sports updates, and collaborative online tools. By maintaining an open connection, Websockets significantly improve the efficiency and responsiveness of web applications.
How Websockets Work?
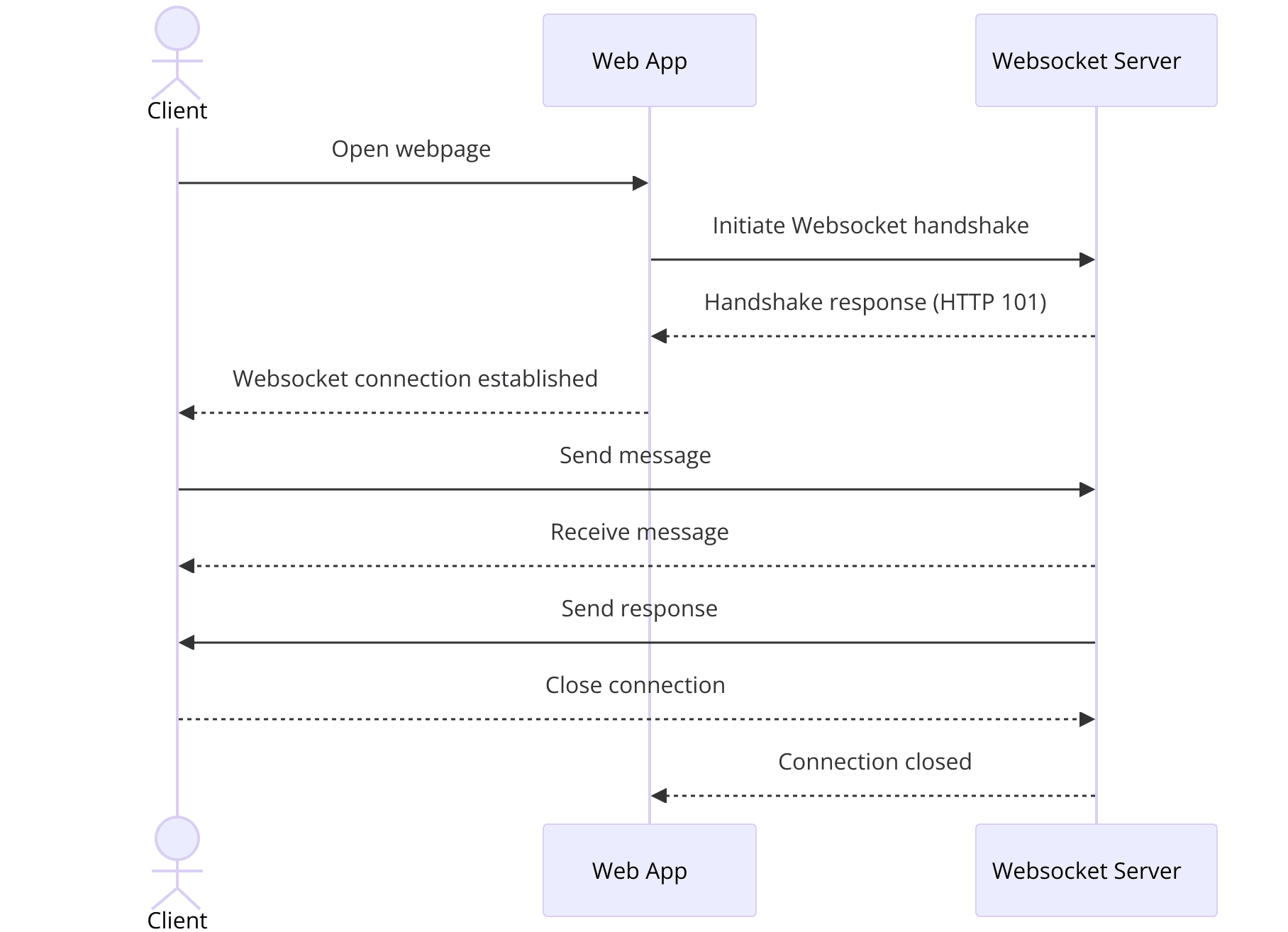
Once the handshake is complete, a persistent Websocket connection is established. Communication then occurs through a series of data frames, which can be text or binary messages. This full-duplex connection allows for real-time data exchange, with messages flowing freely in both directions until the connection is closed by either party.
Setting Up a Websocket Server
Setting up a Websocket server requires a suitable environment and tools. For this example, we’ll use Node.js and the popular
ws
library. First, ensure you have Node.js installed. Then, create a new project directory and initialize it with npm init -y
. Install the ws
library using npm install ws
.Here’s a basic example of a Websocket server in Node.js:
JavaScript
1const WebSocket = require('ws');
2
3const wss = new WebSocket.Server({ port: 8080 });
4
5wss.on('connection', ws => {
6 console.log('New client connected');
7
8 ws.on('message', message => {
9 console.log(`Received: ${message}`);
10 ws.send(`You said: ${message}`);
11 });
12
13 ws.on('close', () => {
14 console.log('Client disconnected');
15 });
16});
17
18console.log('WebSocket server is running on ws://localhost:8080');
19
Establishing a Websocket Client Connection
To connect to a Websocket server from a client, you can use JavaScript in the browser. The
WebSocket
API provides an easy-to-use interface for this purpose. Here’s an example of how to establish a connection to the Websocket server we set up earlier:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>WebSocket Client</title>
6</head>
7<body>
8 <script>
9 const socket = new WebSocket('ws://localhost:8080');
10
11 socket.addEventListener('open', event => {
12 console.log('Connected to WebSocket server');
13 socket.send('Hello Server!');
14 });
15
16 socket.addEventListener('message', event => {
17 console.log(`Message from server: ${event.data}`);
18 });
19
20 socket.addEventListener('close', event => {
21 console.log('Disconnected from WebSocket server');
22 });
23 </script>
24</body>
25</html>
26
Implementing Websocket Communication
Once the connection is established, you can send and receive messages between the client and server. Here’s how you can implement basic Websocket communication:
On the server side (continuation from the previous example):
JavaScript
1wss.on('connection', ws => {
2 ws.on('message', message => {
3 console.log(`Received: ${message}`);
4 ws.send(`Server received: ${message}`);
5 });
6});
7
On the client side:
JavaScript
1socket.addEventListener('open', event => {
2 socket.send('Hello Server!');
3});
4
5socket.addEventListener('message', event => {
6 console.log(`Message from server: ${event.data}`);
7});
8
This simple setup allows the client to send messages to the server and receive responses, demonstrating the full-duplex communication capability of Websockets.
Security Considerations
While Websockets provide powerful real-time communication capabilities, they also come with security challenges. Common threats include Cross-Site WebSocket Hijacking, Denial of Service (DoS) attacks, and man-in-the-middle attacks. To mitigate these risks, consider implementing the following security measures:
- Authentication and Authorization: Ensure that only authenticated users can establish Websocket connections.
- TLS/SSL Encryption: Use secure Websockets (
wss://
) to encrypt data in transit. - Rate Limiting: Implement rate limiting to prevent DoS attacks.
- Origin Checking: Validate the origin header to prevent Cross-Site WebSocket Hijacking.
By addressing these security considerations, you can create a more secure Websocket implementation.
Use Cases and Examples
Websockets are widely used in scenarios requiring real-time data exchange. Some common applications include:
- Chat Applications: Real-time messaging and notifications.
- Online Gaming: Instant game state updates and interactions.
- Live Sports Updates: Real-time scores and event notifications.
- Collaborative Tools: Real-time collaboration on documents and projects.
Example Case Study
A popular online game uses Websockets to provide real-time interactions between players. The game's server sends instant updates on player actions, ensuring a seamless and interactive gaming experience.
Troubleshooting and Optimization
To ensure optimal performance and reliability of your Websocket implementation, consider the following troubleshooting and optimization tips:
- Connection Issues: Verify the server and client URLs, and check network configurations.
- Message Handling: Implement proper error handling for message parsing and processing.
- Resource Management: Close unused Websocket connections to free up resources.
- Performance Monitoring: Use monitoring tools to track and analyze Websocket performance metrics.
By proactively addressing these issues, you can enhance the efficiency and robustness of your Websocket-based applications.
Conclusion
Websockets have revolutionized real-time communication in web applications, providing a powerful tool for creating responsive and interactive experiences. By understanding and implementing Websockets, developers can build applications that deliver instant updates and seamless communication, enhancing user engagement and satisfaction.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ