Mastering WebRTC in React Native
Introduction to WebRTC and React Native
WebRTC (Web Real-Time Communication) is an open-source project that provides real-time communication capabilities to web browsers and mobile applications via simple APIs. It enables audio and video communication directly between browsers or devices without requiring an intermediary server (in some cases, a signaling server is still required to negotiate the connection).
What is React Native?
React Native is a JavaScript framework for building native mobile applications. It allows developers to use their existing JavaScript knowledge to create cross-platform apps for iOS and Android from a single codebase.
Why Combine WebRTC and React Native?
Combining WebRTC and React Native enables you to build cross-platform, real-time communication applications with native performance. You can leverage the power of WebRTC for peer-to-peer audio and video streaming and the flexibility of React Native to create a user-friendly mobile interface. This combination is ideal for building applications such as video conferencing apps, live streaming platforms, and real-time collaboration tools. The ability to write code once and deploy to both iOS and Android significantly reduces development time and costs. Furthermore, it allows developers familiar with Javascript to rapidly deploy high performant communication applications. This also unlocks possibilities for features such as screen sharing and secure peer to peer communication.
Setting up your React Native Environment for WebRTC
Before diving into code, you'll need to set up your React Native environment to work with WebRTC.
Choosing the Right WebRTC Library (react-native-webrtc
)
For React Native,
react-native-webrtc
is the most popular and well-maintained library for WebRTC integration. It provides the necessary native modules and JavaScript APIs to access WebRTC functionalities. It handles the complexities of interacting with the native WebRTC implementations on both iOS and Android.Installation and Configuration
Install the
react-native-webrtc
library using npm or yarn:npm
1npm install react-native-webrtc
2
yarn
1yarn add react-native-webrtc
2
Next, you'll need to link the native modules. For React Native versions 0.60 and above, this is typically handled automatically with autolinking. However, it's good to verify the installation.
After installing the package, you might need to rebuild your project:
bash
1react-native run-android
2react-native run-ios
3
Basic project setup with
react-native-webrtc
involves importing the necessary components:javascript
1import {mediaDevices} from 'react-native-webrtc';
2import {RTCPeerConnection, RTCIceCandidate, RTCSessionDescription} from 'react-native-webrtc';
3import {RTCView} from 'react-native-webrtc';
4
Android Setup
For Android, ensure that you have the necessary permissions in your
AndroidManifest.xml
file. These permissions are crucial for accessing the camera and microphone.AndroidManifest.xml
1<uses-permission android:name="android.permission.CAMERA" />
2<uses-permission android:name="android.permission.RECORD_AUDIO" />
3<uses-permission android:name="android.permission.INTERNET" />
4<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
5<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
6<uses-permission android:name="android.permission.BLUETOOTH" />
7<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
8<uses-feature android:name="android.hardware.camera" />
9<uses-feature android:name="android.hardware.camera.autofocus" />
10
Also, you may need to configure the
build.gradle
file to ensure compatibility with the WebRTC library.iOS Setup
For iOS, you'll need to add permissions in your
Info.plist
file. This is required for accessing the camera and microphone on iOS devices. These privacy settings are essential for user trust and compliance with Apple's guidelines.Info.plist
1<key>NSCameraUsageDescription</key>
2<string>This app needs access to your camera for video calls.</string>
3<key>NSMicrophoneUsageDescription</key>
4<string>This app needs access to your microphone for audio calls.</string>
5
Additionally, verify that your Podfile is correctly configured and run
pod install
in the ios
directory.Building a Simple Video Chat Application with WebRTC and React Native
Now, let's create a basic video chat application using WebRTC and React Native.
Project Structure
A typical project structure might look like this:
1YourProject/
2 ├── App.js
3 ├── components/
4 │ ├── Video.js
5 │ └── ...
6 ├── services/
7 │ └── WebRTCService.js
8 └── ...
9
Setting up the User Interface
Create basic UI components for displaying video streams and handling user interactions.
javascript
1import React, {useState, useEffect, useRef} from 'react';
2import {View, Text, StyleSheet, TouchableOpacity} from 'react-native';
3import {RTCView} from 'react-native-webrtc';
4
5const VideoComponent = () => {
6 const [localStream, setLocalStream] = useState(null);
7 const [remoteStream, setRemoteStream] = useState(null);
8
9 return (
10 <View style={styles.container}>
11 <Text>Local Video</Text>
12 <RTCView streamURL={localStream ? localStream.toURL() : null} style={styles.video} objectFit="cover"/>
13 <Text>Remote Video</Text>
14 <RTCView streamURL={remoteStream ? remoteStream.toURL() : null} style={styles.video} objectFit="cover"/>
15 </View>
16 );
17};
18
19const styles = StyleSheet.create({
20 container: {
21 flex: 1,
22 justifyContent: 'center',
23 alignItems: 'center',
24 },
25 video: {
26 width: 200,
27 height: 200,
28 borderColor: 'black',
29 borderWidth: 1,
30 },
31});
32
33export default VideoComponent;
34
Implementing the WebRTC Logic
Implement the core WebRTC functionality, including peer connection setup, media stream handling, and signaling. Consider using a
WebRTCService.js
to keep things modular.First, establishing a peer connection involves creating an
RTCPeerConnection
instance and configuring ICE servers.javascript
1const configuration = {
2 iceServers: [
3 { urls: 'stun:stun.l.google.com:19302' },
4 { urls: 'stun:stun1.l.google.com:19302' },
5 ],
6};
7
8const pc = new RTCPeerConnection(configuration);
9
Next, handle media streams by accessing the user's camera and microphone.
javascript
1mediaDevices.getUserMedia({ audio: true, video: true })
2 .then(stream => {
3 // Got stream!
4 })
5 .catch(error => {
6 // Handle error
7 });
8
To add video and audio tracks to the peer connection, use the
addTrack
method.javascript
1stream.getTracks().forEach(track => pc.addTrack(track, stream));
2
Error handling and connection management are crucial for a robust application. Listen for ICE candidate events and handle connection state changes.
javascript
1pc.onicecandidate = event => {
2 if (event.candidate) {
3 // Send candidate to remote peer via signaling
4 }
5};
6
7pc.onconnectionstatechange = event => {
8 switch (pc.connectionState) {
9 case 'disconnected':
10 case 'failed':
11 // Handle disconnection
12 break;
13 }
14};
15
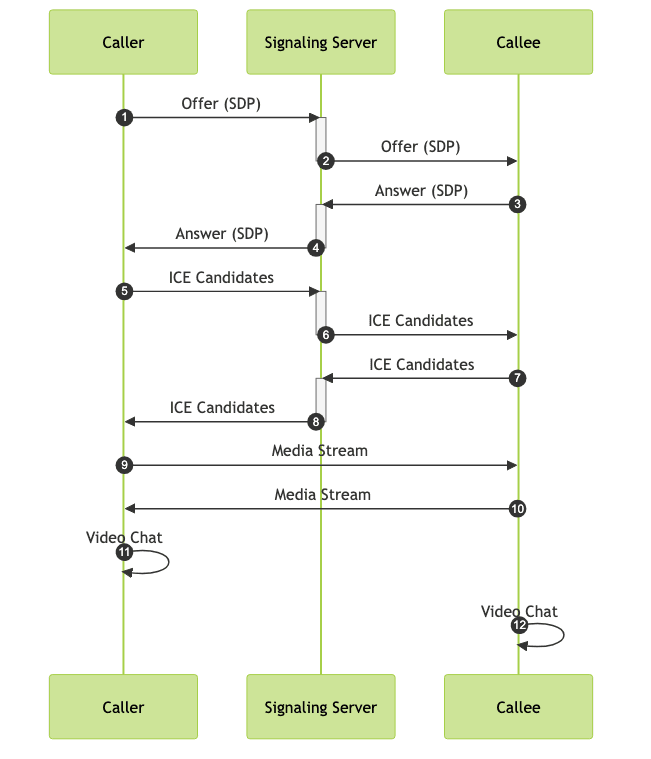
Testing the Application
Test your application on both iOS and Android devices to ensure cross-platform compatibility and proper functionality. Use tools like Reactotron or the built-in React Native debugger for debugging.
Advanced WebRTC Techniques in React Native
Explore advanced techniques to enhance your WebRTC applications.
Handling Signaling
Signaling is the process of exchanging metadata between peers before a WebRTC connection can be established. This metadata includes session descriptions (SDP) and ICE candidates. Choosing a signaling server is critical for enabling peers to discover each other and negotiate a connection. Common options include WebSockets and Socket.IO. These technologies provide a persistent, bidirectional communication channel between clients and the server.
Implementing Data Channels
WebRTC data channels allow you to send arbitrary data between peers. This is useful for implementing features like file sharing, text chat, or game data synchronization.
javascript
1const dataChannel = pc.createDataChannel('myChannel');
2
3dataChannel.onopen = () => {
4 dataChannel.send('Hello, world!');
5};
6
7dataChannel.onmessage = event => {
8 console.log('Received message:', event.data);
9};
10
Optimizing Performance
Optimize video quality and reduce latency by adjusting WebRTC parameters. Consider strategies like using simulcast for adaptive bitrate streaming and implementing congestion control mechanisms. Bandwidth management is also crucial for ensuring a smooth user experience, especially in environments with limited network resources.
Screen Sharing
Screen sharing functionality can be added using platform-specific APIs to capture the screen content and stream it to the remote peer.
Troubleshooting Common WebRTC and React Native Issues
Address common issues that may arise during development.
Connection Errors
Troubleshoot connection errors by examining ICE candidate exchange, NAT traversal issues, and firewall configurations. Ensure that your signaling server is functioning correctly and that peers can successfully exchange SDP and ICE candidates.
Media Stream Issues
Resolve media stream issues by verifying camera and microphone permissions, checking device compatibility, and handling codec negotiation failures. Ensure that the correct media constraints are being used and that the user has granted the necessary permissions.
Platform-Specific Problems
Address platform-specific problems by consulting the React Native and
react-native-webrtc
documentation, reviewing error logs, and seeking community support. Common issues include native module linking problems, permission errors, and codec compatibility issues.Conclusion and Future Trends
WebRTC and React Native provide a powerful combination for building real-time communication applications. As WebRTC technology continues to evolve, expect to see advancements in areas like AI-powered video enhancement, improved security, and more seamless integration with other web technologies. WebRTC in React Native opens the door to cross-platform, native-performing communication experiences.
- Learn more about WebRTC:
Official WebRTC website
- React Native Documentation:
Comprehensive guide to React Native development
- react-native-webrtc NPM package:
NPM page for the main library
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ