Video Conferencing API: A Developer's Guide to Integration and Best Practices
In today's interconnected world, video conferencing has become an essential communication tool for businesses, education, and healthcare. Integrating video conferencing capabilities directly into your application can significantly enhance user experience and streamline workflows. This comprehensive guide will walk you through the world of video conferencing APIs, helping you understand their benefits, choose the right one for your needs, and implement it effectively.
What is a Video Conferencing API? (Approx. 200 words)
Defining Video Conferencing APIs
A Video Conferencing API (Application Programming Interface) is a set of tools, protocols, and code that allows developers to embed video conferencing functionality into their own applications. Instead of building a video conferencing platform from scratch, developers can leverage existing APIs to quickly and easily add features like video calls, screen sharing, and recording to their software.
How Video Conferencing APIs Work
Video Conferencing APIs act as intermediaries between your application and the underlying video conferencing infrastructure. When a user initiates a video call within your application, the API handles the complex tasks of establishing a connection, encoding and decoding video and audio streams, and managing data transfer. It abstracts away the technical details, allowing you to focus on the user interface and overall application logic.
Here's a visual representation of how a video conferencing API works:
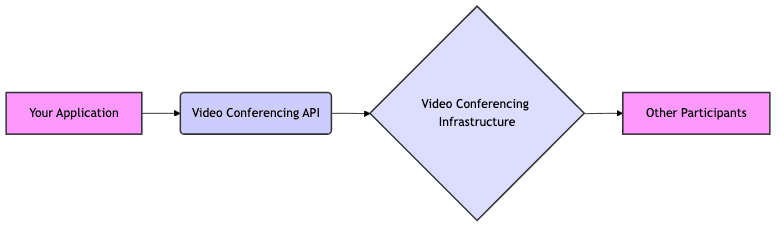
Key Benefits of Using a Video Conferencing API
- Faster Development: Save time and resources by leveraging pre-built functionality.
- Reduced Costs: Avoid the expense of building a video conferencing platform from scratch.
- Enhanced User Experience: Integrate video conferencing seamlessly into your application's existing workflows.
- Scalability: Easily scale your video conferencing capabilities as your user base grows.
- Customization: Tailor the video conferencing experience to your specific needs.
Choosing the Right Video Conferencing API (Approx. 300 words)
Factors to Consider When Selecting an API
Choosing the right video conferencing API is crucial for the success of your project. Consider the following factors:
- Features: Does the API offer the features you need, such as screen sharing, recording, and live streaming?
- Scalability: Can the API handle your expected user load?
- Reliability: Does the API have a proven track record of uptime and stability?
- Security: Does the API offer robust security features to protect user data?
- Ease of Use: Is the API well-documented and easy to integrate?
- Pricing: Does the API's pricing model fit your budget?
- Support: Does the API provider offer good customer support?
Key Features to Look For
When evaluating video conferencing APIs, prioritize features that align with your application's requirements. Some key features to look for include:
- High-Quality Video and Audio: Ensure clear and reliable communication.
- Screen Sharing: Enable users to share their screens for presentations and collaboration.
- Recording: Allow users to record video conferences for later viewing.
- Live Streaming: Broadcast video conferences to a wider audience.
- Chat: Provide a text-based chat feature for communication during video conferences.
- Whiteboarding: Enable collaborative whiteboarding for brainstorming and visual communication.
- Breakout Rooms: Divide participants into smaller groups for focused discussions.
- Background Blur/Virtual Backgrounds: Offer options for privacy and personalization.
Pricing Models and Costs
Video conferencing APIs typically offer various pricing models, including:
- Pay-as-you-go: Pay only for the minutes or data you use.
- Subscription-based: Pay a fixed monthly or annual fee for a set amount of usage.
- Usage-based with tiers: Pay different rates based on your usage volume.
Consider your expected usage patterns and budget when choosing a pricing model. Be sure to factor in potential overage charges and hidden fees.
Scalability and Reliability
Scalability and reliability are paramount for video conferencing APIs. Ensure that the API you choose can handle your expected user load and maintain consistent performance under varying conditions. Look for APIs with a Service Level Agreement (SLA) that guarantees a certain level of uptime.
Top Video Conferencing APIs Compared (Approx. 400 words)
Here's a comparison of some popular video conferencing APIs:
API #1: Twilio Video – Features, Strengths, Weaknesses
Twilio Video is a flexible and powerful video conferencing API that offers a wide range of features, including screen sharing, recording, and live streaming. It's known for its robust infrastructure and global reach.
- Features: High-quality video and audio, screen sharing, recording, live streaming, chat, programmable video.
- Strengths: Highly customizable, scalable, reliable, global infrastructure.
- Weaknesses: Can be complex to set up, pricing can be unpredictable.
python
1from twilio.jwt.access_token import AccessToken
2from twilio.jwt.access_token.grants import VideoGrant
3
4# Your Account SID and Auth Token from twilio.com/console
5# Set the environment variables for security
6account_sid = "ACxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
7api_key_sid = "SKxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"
8api_key_secret = "your_api_key_secret"
9
10# Required for all Twilio access tokens
11identity = "user123"
12
13# Create access token with Video grant
14token = AccessToken(account_sid, api_key_sid, api_key_secret, identity=identity)
15
16# Create a Video grant and add to token
17video_grant = VideoGrant(room="MyRoom")
18token.add_grant(video_grant)
19
20# Serialize the token to a string
21print(token.to_jwt())
22
API #2: Zoom Video SDK – Features, Strengths, Weaknesses
Zoom Video SDK provides the core Zoom video conferencing experience as a set of APIs and SDKs, allowing developers to integrate Zoom's proven technology into their applications.
- Features: HD video and audio, screen sharing, recording, chat, breakout rooms, virtual backgrounds.
- Strengths: Easy to use, reliable, feature-rich, integrates with existing Zoom infrastructure.
- Weaknesses: Less customizable than some other APIs, tighter integration with the Zoom ecosystem.
javascript
1// Initialize the Zoom Video SDK
2const zoomClient = ZoomVideo.init({
3 zoomJssdkKey: 'YOUR_ZOOM_JSSDK_KEY',
4 zoomJssdkSecret: 'YOUR_ZOOM_JSSDK_SECRET'
5});
6
7// Join a Zoom meeting
8zoomClient.join({
9 meetingNumber: 'YOUR_MEETING_NUMBER',
10 userName: 'YOUR_USERNAME',
11 signature: 'YOUR_MEETING_SIGNATURE',
12 sdkKey: 'YOUR_ZOOM_JSSDK_KEY'
13}).then(() => {
14 console.log('Successfully joined the Zoom meeting!');
15}).catch((error) => {
16 console.error('Failed to join the Zoom meeting:', error);
17});
18
API #3: Agora.io – Features, Strengths, Weaknesses
Agora.io offers a comprehensive suite of real-time communication APIs, including video conferencing, voice calling, and interactive live streaming. It's known for its low latency and high-quality audio and video.
- Features: High-quality video and audio, low latency, global network, interactive live streaming, voice calling.
- Strengths: Excellent performance, global reach, versatile API, robust SDKs.
- Weaknesses: Can be more expensive than some other APIs, complex pricing structure.
java
1// Initialize the Agora Engine
2private RtcEngine mRtcEngine;
3
4try {
5 mRtcEngine = RtcEngine.create(getBaseContext(), "YOUR_APP_ID", mRtcEventHandler);
6} catch (Exception e) {
7 Log.e(TAG, Log.getStackTraceString(e));
8 throw new RuntimeException("NEED TO check rtc sdk init fatal error
9" + Log.getStackTraceString(e));
10}
11
12// Join a channel
13mRtcEngine.joinChannel(null, "testChannel", "", 0);
14
API #4: Jitsi Meet – Features, Strengths, Weaknesses
Jitsi Meet is an open-source video conferencing platform that offers a free and customizable video conferencing API. It's a good option for developers who want more control over their video conferencing infrastructure.
- Features: Free and open-source, screen sharing, recording, chat, integration with other services.
- Strengths: Highly customizable, free to use, privacy-focused.
- Weaknesses: Requires more technical expertise to set up and maintain, may not be as scalable as commercial APIs.
html
1<!DOCTYPE html>
2<html>
3<head>
4 <meta charset="UTF-8">
5 <title>Jitsi Meet Iframe Example</title>
6</head>
7<body>
8 <iframe src="https://meet.jit.si/YourRoomName" width="800" height="600"></iframe>
9</body>
10</html>
11
Integrating a Video Conferencing API into Your Application (Approx. 500 words)
Step-by-Step Integration Guide (General Approach)
Integrating a video conferencing API typically involves the following steps:
- Sign up for an account: Create an account with the video conferencing API provider.
- Obtain API keys: Obtain API keys or credentials from the provider's developer portal.
- Install the SDK: Install the API's SDK (Software Development Kit) for your programming language or platform.
- Initialize the API: Initialize the API with your API keys or credentials.
- Implement video conferencing features: Use the API's functions and methods to implement the desired video conferencing features, such as joining a room, starting a call, and sharing the screen.
- Handle events: Implement event handlers to respond to events such as user joins, user leaves, and error messages.
- Test and deploy: Test your integration thoroughly and deploy your application to production.
Common Integration Challenges and Solutions
Integrating a video conferencing API can present several challenges, including:
- Complexity: Video conferencing APIs can be complex and require a good understanding of networking and real-time communication protocols.
- Solution: Start with the provider's sample code and tutorials. Break down the integration into smaller, manageable tasks.
- Compatibility: Ensuring compatibility with different browsers, devices, and operating systems can be challenging.
- Solution: Use the API's recommended SDKs and libraries. Test your integration on a variety of devices and browsers.
- Performance: Optimizing video and audio quality for different network conditions can be difficult.
- Solution: Use the API's quality settings and adaptive bitrate streaming features. Implement error handling to gracefully handle network disruptions.
- Security: Protecting user data and preventing unauthorized access is crucial.
- Solution: Use the API's security features, such as encryption and authentication. Follow security best practices for web development.
Handling User Authentication and Authorization
Securely authenticating and authorizing users is essential for protecting your video conferencing application. Implement a robust authentication system that verifies users' identities before granting them access to video conferencing features. Use the API's authentication and authorization mechanisms to control access to specific rooms or features.
Integrating with Existing Systems
Integrating a video conferencing API with your existing systems, such as CRM or project management software, can streamline workflows and enhance collaboration. Use the API's webhooks or events to trigger actions in other systems. For example, you can automatically create a meeting in your CRM when a video conference is scheduled.
Testing and Deployment
Thorough testing is crucial before deploying your video conferencing integration to production. Test on different devices, browsers, and network conditions. Use automated testing tools to ensure that your integration is working correctly. Monitor your application's performance after deployment and address any issues that arise.
Advanced Features and Use Cases (Approx. 300 words)
Screen Sharing and Recording
Screen sharing and recording are essential features for many video conferencing applications. Use the API's screen sharing capabilities to allow users to share their screens for presentations and collaboration. Implement recording functionality to allow users to record video conferences for later viewing or archiving.
Live Streaming Capabilities
Live streaming allows you to broadcast video conferences to a wider audience. Use the API's live streaming capabilities to stream video conferences to platforms like YouTube or Facebook Live. This can be useful for webinars, online events, and virtual conferences.
Real-time Transcription and Translation
Real-time transcription and translation can make video conferences more accessible and inclusive. Use AI-powered transcription and translation services to provide real-time captions and translations of spoken words. This can be especially helpful for participants who are deaf or hard of hearing, or who speak different languages.
Integration with AI-powered Features
Video conferencing APIs can be integrated with AI-powered features to enhance the user experience. For example, you can use AI to automatically blur backgrounds, detect and remove noise, or provide real-time facial recognition.
Use Cases: Telehealth, Education, Business
Video conferencing APIs are used in a wide range of industries and applications, including:
- Telehealth: Providing remote healthcare services to patients.
- Education: Delivering online classes and virtual training programs.
- Business: Conducting virtual meetings, webinars, and conferences.
Security Considerations for Video Conferencing APIs (Approx. 200 words)
Data Encryption and Security Protocols
Security is paramount when using video conferencing APIs. Ensure that the API you choose uses strong data encryption to protect user data in transit and at rest. Look for APIs that support industry-standard security protocols, such as TLS and AES.
User Authentication and Access Control
Implement robust user authentication and access control mechanisms to prevent unauthorized access to video conferences. Use strong passwords, multi-factor authentication, and role-based access control to protect user accounts and data.
Compliance with Data Privacy Regulations (e.g., GDPR, HIPAA)
If you are handling sensitive data, such as personal health information (PHI), you must comply with relevant data privacy regulations, such as GDPR and HIPAA. Choose a video conferencing API that is compliant with these regulations and implement appropriate security measures to protect user data.
Future Trends in Video Conferencing APIs (Approx. 100 words)
WebRTC Advancements
WebRTC (Web Real-Time Communication) is a free, open-source technology that enables real-time communication in web browsers and mobile applications. Future advancements in WebRTC will likely lead to improved video and audio quality, lower latency, and enhanced security for video conferencing APIs. Learn more about WebRTC:
Understand the underlying technology powering many video conferencing APIs.
AI and Machine Learning Integration
AI and machine learning are increasingly being integrated into video conferencing APIs to enhance the user experience. Expect to see more AI-powered features, such as automatic background blur, noise reduction, and real-time translation, in future video conferencing APIs.
Enhanced Security Measures
As cyber threats become more sophisticated, video conferencing API providers will continue to enhance their security measures. This will likely include stronger encryption, multi-factor authentication, and advanced threat detection capabilities. Read about API security best practices:
Improve the security of your video conferencing API integration.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ