What is RecordRTC?
RecordRTC is an open-source library that simplifies the process of recording audio, video, and screen activities directly from the browser. Built on top of WebRTC, RecordRTC provides a robust solution for developers seeking to integrate recording functionality into their web applications with minimal effort.
Importance of WebRTC in Modern Web Applications
WebRTC (Web Real-Time Communication) is a powerful technology that enables real-time audio, video, and data sharing between browsers without the need for plugins. It has revolutionized the way web applications handle live communication, making it a vital component for any modern web app that requires real-time interaction, such as video conferencing, live streaming, and online collaboration tools.
Overview of JS and P2P Recording
JavaScript (JS) is the backbone of web development, allowing for dynamic and interactive user experiences. When combined with WebRTC, JS can leverage peer-to-peer (P2P) recording, which means media can be recorded directly from the user’s device and transmitted over the network without needing a central server. This decentralized approach not only improves performance and reduces latency but also enhances privacy and security by minimizing the number of intermediaries involved in the communication process. RecordRTC, by utilizing these technologies, offers a seamless way to capture and manage media streams in real time.
Let`s Build RecordRTC WebRTC App with the help of JavaScript
To start with RecordRTC, you first need to set up your development environment. Here are the steps:
Setting Up the Project Environment
Begin by ensuring you have Node.js and npm (Node Package Manager) installed on your machine. These tools are essential for managing dependencies and running your application.
Installation
Install the necessary dependencies for your project. Open your terminal and run the following commands:
bash
1npm install recordrtc webrtc-adapter
2
These commands will install RecordRTC and WebRTC adapter, which helps in handling browser-specific WebRTC issues.
Structure of the Project
Organize your project directory for better management and scalability. Here is a simple structure:
1/recordrtc-webrtc-app
2 /css
3 - styles.css
4 /js
5 - app.js
6 - index.html
7 - package.json
8
This structure includes directories for CSS and JavaScript files and the main HTML file.
App Architecture
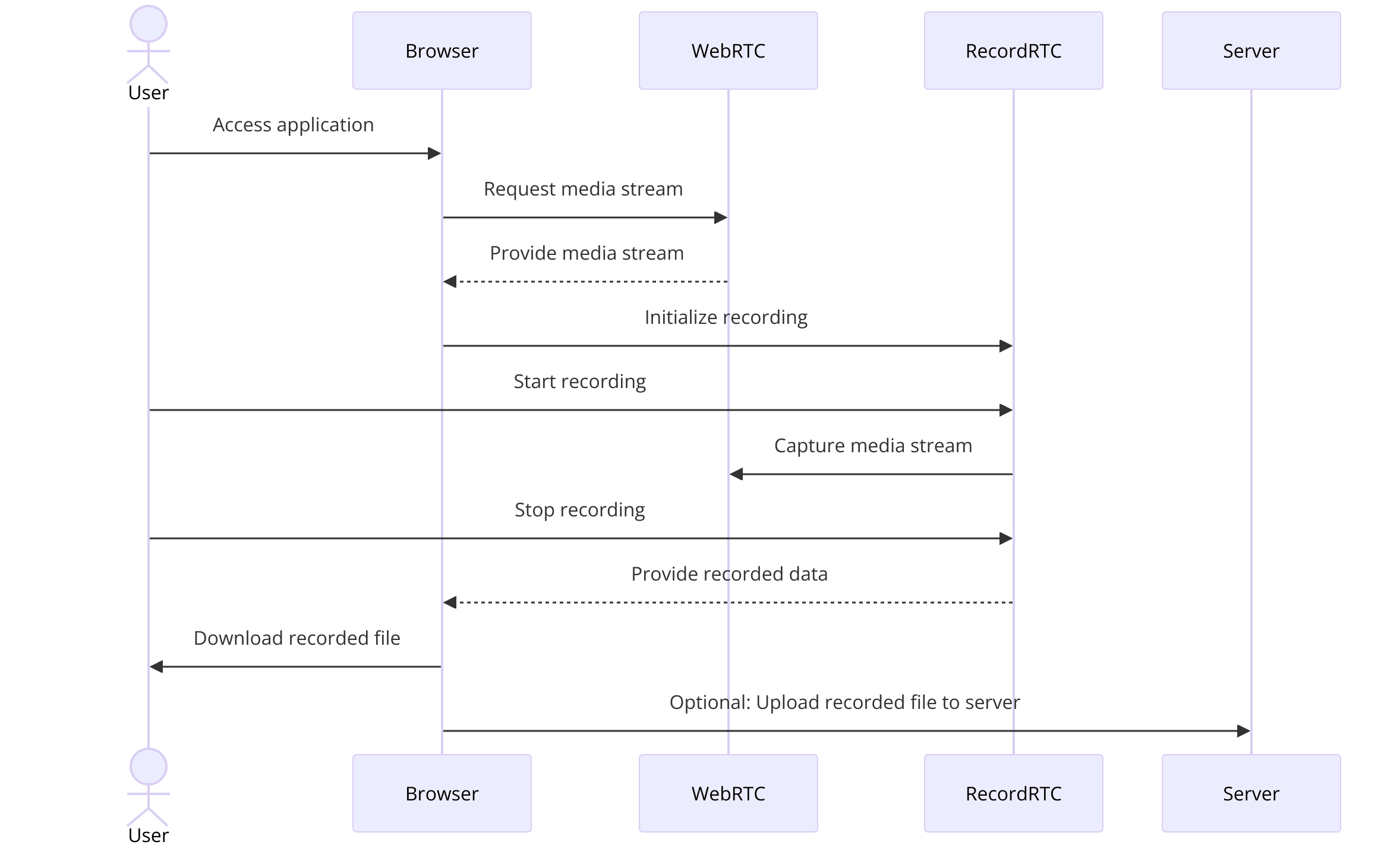
- HTML: The structure of your web page.
- CSS: Styling for a better user interface.
- JavaScript: The logic to handle WebRTC and RecordRTC functionalities.
This setup ensures a clean and manageable project, ready for further development with RecordRTC and WebRTC. In the next sections, we'll delve into the specifics of each step, starting with creating the basic HTML file and integrating RecordRTC.
Step 1: Get Started with index.html
In this section, we will create the foundational HTML file for our RecordRTC WebRTC application. This file will serve as the main entry point for our app, setting up the basic structure and integrating the necessary scripts.
Creating index.html
Basic HTML Structure
Start by creating a file named
index.html
in your project directory. This file will contain the HTML skeleton needed for our application. Here is a basic example:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RecordRTC WebRTC App</title>
7 <link rel="stylesheet" href="css/styles.css">
8</head>
9<body>
10 <h1>RecordRTC WebRTC Application</h1>
11 <video id="video" autoplay></video>
12 <div>
13 <button id="start-record">Start Recording</button>
14 <button id="stop-record" disabled>Stop Recording</button>
15 </div>
16 <script src="js/app.js"></script>
17</body>
18</html>
19
This basic structure includes a video element to display the video stream and buttons to control the recording process.
Integrating RecordRTC
Next, we need to integrate RecordRTC into our HTML file. Add the following script tags inside the
<head>
section to include the RecordRTC and WebRTC adapter scripts:HTML
1<script src="https://cdn.webrtc-experiment.com/RecordRTC.js"></script>
2<script src="https://webrtc.github.io/adapter/adapter-latest.js"></script>
3
Now, we need to initialize RecordRTC in our JavaScript file. In
app.js
, add the following code to set up the video stream and recording functionality:JavaScript
1navigator.mediaDevices.getUserMedia({ video: true, audio: true })
2 .then(function(stream) {
3 const video = document.getElementById('video');
4 video.srcObject = stream;
5 const recorder = RecordRTC(stream, {
6 type: 'video'
7 });
8
9 document.getElementById('start-record').onclick = function() {
10 recorder.startRecording();
11 this.disabled = true;
12 document.getElementById('stop-record').disabled = false;
13 };
14
15 document.getElementById('stop-record').onclick = function() {
16 recorder.stopRecording(function() {
17 const blob = recorder.getBlob();
18 const url = URL.createObjectURL(blob);
19 const a = document.createElement('a');
20 a.style.display = 'none';
21 a.href = url;
22 a.download = 'recording.webm';
23 document.body.appendChild(a);
24 a.click();
25 window.URL.revokeObjectURL(url);
26 });
27 this.disabled = true;
28 document.getElementById('start-record').disabled = false;
29 };
30 })
31 .catch(function(error) {
32 console.error('Error accessing media devices.', error);
33 });
34
With these steps, you have set up a basic HTML file and integrated RecordRTC into your application. You can now start and stop video recordings directly from your browser. In the next section, we will wireframe all the components to enhance the user interface and functionality.
Step 2: Wireframe All the Components
In this section, we will design the user interface (UI) for our RecordRTC WebRTC application. The UI will include elements for displaying video streams and controlling the recording process. We'll provide the HTML and CSS needed to create a functional and aesthetically pleasing interface.
Creating the Wireframe
Designing the User Interface
A good UI is crucial for a smooth user experience. Our application will have a video display area and control buttons for starting and stopping the recording. Here's the enhanced HTML structure:
HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RecordRTC WebRTC App</title>
7 <link rel="stylesheet" href="css/styles.css">
8</head>
9<body>
10 <div class="container">
11 <h1>RecordRTC WebRTC Application</h1>
12 <div class="video-container">
13 <video id="video" autoplay></video>
14 </div>
15 <div class="controls">
16 <button id="start-record">Start Recording</button>
17 <button id="stop-record" disabled>Stop Recording</button>
18 </div>
19 </div>
20 <script src="https://cdn.webrtc-experiment.com/RecordRTC.js"></script>
21 <script src="https://webrtc.github.io/adapter/adapter-latest.js"></script>
22 <script src="js/app.js"></script>
23</body>
24</html>
25
Code Snippets for UI Elements
To style the UI, create a CSS file named
styles.css
in the css
directory. Add the following CSS code to style the video element, buttons, and overall layout:CSS
1body {
2 font-family: Arial, sans-serif;
3 background-color: #f4f4f4;
4 margin: 0;
5 padding: 0;
6 display: flex;
7 justify-content: center;
8 align-items: center;
9 height: 100vh;
10}
11
12.container {
13 text-align: center;
14 background: #fff;
15 padding: 20px;
16 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
17 border-radius: 8px;
18}
19
20.video-container {
21 margin: 20px 0;
22}
23
24video {
25 width: 100%;
26 max-width: 600px;
27 border: 1px solid #ddd;
28 border-radius: 8px;
29}
30
31.controls {
32 margin-top: 20px;
33}
34
35button {
36 padding: 10px 20px;
37 font-size: 16px;
38 margin: 5px;
39 border: none;
40 border-radius: 5px;
41 cursor: pointer;
42 transition: background-color 0.3s;
43}
44
45button:disabled {
46 background-color: #ccc;
47 cursor: not-allowed;
48}
49
50button#start-record {
51 background-color: #28a745;
52 color: white;
53}
54
55button#start-record:hover {
56 background-color: #218838;
57}
58
59button#stop-record {
60 background-color: #dc3545;
61 color: white;
62}
63
64button#stop-record:hover {
65 background-color: #c82333;
66}
67
This CSS will ensure that your application looks clean and professional, with responsive design elements that adjust to different screen sizes.
With these steps, you have created a user-friendly interface for your RecordRTC WebRTC application. The next sections will focus on implementing the join screen, adding more functionalities, and enhancing the overall user experience.
Step 3: Implement Join Screen
In this section, we will build a join screen for our RecordRTC WebRTC application. This screen will allow users to input their name or room ID before entering the main recording interface. The join screen will enhance the user experience by providing a clear entry point to the application.
Building the Join Screen
Setting Up the Join Screen
First, let's create the join screen layout. Update your
index.html
to include a join screen and the main recording screen, which will be shown conditionally. Here’s how to set it up:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RecordRTC WebRTC App</title>
7 <link rel="stylesheet" href="css/styles.css">
8</head>
9<body>
10 <div id="join-screen" class="container">
11 <h1>Join Room</h1>
12 <input type="text" id="room-id" placeholder="Enter Room ID">
13 <button id="join-room">Join</button>
14 </div>
15 <div id="main-screen" class="container" style="display:none;">
16 <h1>RecordRTC WebRTC Application</h1>
17 <div class="video-container">
18 <video id="video" autoplay></video>
19 </div>
20 <div class="controls">
21 <button id="start-record">Start Recording</button>
22 <button id="stop-record" disabled>Stop Recording</button>
23 </div>
24 </div>
25 <script src="https://cdn.webrtc-experiment.com/RecordRTC.js"></script>
26 <script src="https://webrtc.github.io/adapter/adapter-latest.js"></script>
27 <script src="js/app.js"></script>
28</body>
29</html>
30
Handling User Inputs
Next, we need to handle the user input for the room ID and transition from the join screen to the main screen. Update your
app.js
to include the following JavaScript code:JavaScript
1document.getElementById('join-room').onclick = function() {
2 const roomId = document.getElementById('room-id').value;
3 if (roomId) {
4 document.getElementById('join-screen').style.display = 'none';
5 document.getElementById('main-screen').style.display = 'block';
6 startRecording(roomId);
7 } else {
8 alert('Please enter a room ID.');
9 }
10};
11
12function startRecording(roomId) {
13 navigator.mediaDevices.getUserMedia({ video: true, audio: true })
14 .then(function(stream) {
15 const video = document.getElementById('video');
16 video.srcObject = stream;
17 const recorder = RecordRTC(stream, {
18 type: 'video'
19 });
20
21 document.getElementById('start-record').onclick = function() {
22 recorder.startRecording();
23 this.disabled = true;
24 document.getElementById('stop-record').disabled = false;
25 };
26
27 document.getElementById('stop-record').onclick = function() {
28 recorder.stopRecording(function() {
29 const blob = recorder.getBlob();
30 const url = URL.createObjectURL(blob);
31 const a = document.createElement('a');
32 a.style.display = 'none';
33 a.href = url;
34 a.download = 'recording.webm';
35 document.body.appendChild(a);
36 a.click();
37 window.URL.revokeObjectURL(url);
38 });
39 this.disabled = true;
40 document.getElementById('start-record').disabled = false;
41 };
42 })
43 .catch(function(error) {
44 console.error('Error accessing media devices.', error);
45 });
46}
47
By implementing these changes, you have added a join screen that allows users to enter a room ID before starting the recording session. This step enhances the usability of your application by providing a structured entry point. In the next sections, we will continue building out the functionality and refining the user experience.
Step 4: Implement Controls
In this section, we will add recording controls to our RecordRTC WebRTC application. These controls will allow users to start, stop, and manage the recording process effectively. Implementing these controls is crucial for a seamless user experience.
Adding Recording Controls
Start, Stop, and Pause Recording
To manage the recording process, we need buttons that users can interact with. We have already included start and stop buttons in our HTML structure. Now, let's add functionality to these buttons in our
app.js
file.First, ensure the HTML structure for the buttons is as follows:
HTML
1<div class="controls">
2 <button id="start-record">Start Recording</button>
3 <button id="stop-record" disabled>Stop Recording</button>
4</div>
5
Event Handlers for Controls
Now, update the
app.js
file to add event handlers for these buttons. Here’s the complete JavaScript code to handle the start and stop recording actions:JavaScript
1function startRecording(roomId) {
2 navigator.mediaDevices.getUserMedia({ video: true, audio: true })
3 .then(function(stream) {
4 const video = document.getElementById('video');
5 video.srcObject = stream;
6 const recorder = RecordRTC(stream, {
7 type: 'video'
8 });
9
10 document.getElementById('start-record').onclick = function() {
11 recorder.startRecording();
12 this.disabled = true;
13 document.getElementById('stop-record').disabled = false;
14 };
15
16 document.getElementById('stop-record').onclick = function() {
17 recorder.stopRecording(function() {
18 const blob = recorder.getBlob();
19 const url = URL.createObjectURL(blob);
20 const a = document.createElement('a');
21 a.style.display = 'none';
22 a.href = url;
23 a.download = 'recording.webm';
24 document.body.appendChild(a);
25 a.click();
26 window.URL.revokeObjectURL(url);
27 });
28 this.disabled = true;
29 document.getElementById('start-record').disabled = false;
30 };
31 })
32 .catch(function(error) {
33 console.error('Error accessing media devices.', error);
34 });
35}
36
Enhancing the User Interface
To further improve the user interface, let's ensure our buttons provide immediate visual feedback to users. Update your
styles.css
with the following CSS to style the buttons:CSS
1button {
2 padding: 10px 20px;
3 font-size: 16px;
4 margin: 5px;
5 border: none;
6 border-radius: 5px;
7 cursor: pointer;
8 transition: background-color 0.3s;
9}
10
11button:disabled {
12 background-color: #ccc;
13 cursor: not-allowed;
14}
15
16button#start-record {
17 background-color: #28a745;
18 color: white;
19}
20
21button#start-record:hover {
22 background-color: #218838;
23}
24
25button#stop-record {
26 background-color: #dc3545;
27 color: white;
28}
29
30button#stop-record:hover {
31 background-color: #c82333;
32}
33
By implementing these controls and enhancing the UI, you have made it easier for users to start and stop recordings seamlessly. In the next section, we will build out the participant view, which will allow users to see and manage multiple video streams.
Step 5: Implement Participant View
In this section, we will build the participant view for our RecordRTC WebRTC application. This view will allow users to see their own video stream and, potentially, those of other participants. We’ll focus on displaying video streams effectively and managing multiple participants.
Building Participant View
Displaying Video Streams
To display video streams, we need to update our HTML structure and JavaScript code. We'll add a container for the video elements in our HTML file:
HTML
1<div class="video-container">
2 <video id="local-video" autoplay muted></video>
3</div>
4
In the
app.js
file, we need to ensure the local video stream is displayed correctly:JavaScript
1navigator.mediaDevices.getUserMedia({ video: true, audio: true })
2 .then(function(stream) {
3 const localVideo = document.getElementById('local-video');
4 localVideo.srcObject = stream;
5
6 const recorder = RecordRTC(stream, {
7 type: 'video'
8 });
9
10 document.getElementById('start-record').onclick = function() {
11 recorder.startRecording();
12 this.disabled = true;
13 document.getElementById('stop-record').disabled = false;
14 };
15
16 document.getElementById('stop-record').onclick = function() {
17 recorder.stopRecording(function() {
18 const blob = recorder.getBlob();
19 const url = URL.createObjectURL(blob);
20 const a = document.createElement('a');
21 a.style.display = 'none';
22 a.href = url;
23 a.download = 'recording.webm';
24 document.body.appendChild(a);
25 a.click();
26 window.URL.revokeObjectURL(url);
27 });
28 this.disabled = true;
29 document.getElementById('start-record').disabled = false;
30 };
31 })
32 .catch(function(error) {
33 console.error('Error accessing media devices.', error);
34 });
35
Managing Multiple Participants
To handle multiple participants, we need to dynamically create video elements and append them to the video container. Here's how you can modify the
app.js
file to manage multiple video streams:JavaScript
1function addParticipantVideo(stream, participantId) {
2 const videoContainer = document.querySelector('.video-container');
3 const videoElement = document.createElement('video');
4 videoElement.id = `participant-${participantId}`;
5 videoElement.srcObject = stream;
6 videoElement.autoplay = true;
7 videoContainer.appendChild(videoElement);
8}
9
10// Simulate adding a participant (in a real scenario, this would be triggered by a peer connection event)
11navigator.mediaDevices.getUserMedia({ video: true, audio: true })
12 .then(function(stream) {
13 addParticipantVideo(stream, 'local');
14 })
15 .catch(function(error) {
16 console.error('Error accessing media devices.', error);
17 });
18
19// Example to add another participant
20navigator.mediaDevices.getUserMedia({ video: true, audio: true })
21 .then(function(stream) {
22 addParticipantVideo(stream, 'remote');
23 })
24 .catch(function(error) {
25 console.error('Error accessing media devices.', error);
26 });
27
Enhancing the Participant View
To make the participant view more user-friendly, let's update the CSS to ensure all video elements are displayed neatly:
CSS
1.video-container {
2 display: flex;
3 flex-wrap: wrap;
4 gap: 10px;
5 justify-content: center;
6}
7
8video {
9 width: 45%;
10 max-width: 300px;
11 border: 1px solid #ddd;
12 border-radius: 8px;
13 background-color: black;
14}
15
This CSS ensures that video elements are displayed in a flexible, responsive grid, making it easy to manage multiple video streams.
By implementing these steps, you have created a participant view that can display multiple video streams and handle various participants in your RecordRTC WebRTC application. In the next section, we will run and test the entire application to ensure everything works seamlessly.
Step 6: Run Your Code Now
In this section, we will guide you through running and testing your RecordRTC WebRTC application. By the end of this step, you will be able to see your application in action, recording and displaying video streams.
Running and Testing the Application
How to Run the App Locally
To run your RecordRTC WebRTC application locally, you need to serve your files using a local web server. You can use a simple tool like
http-server
for this purpose. First, install http-server
globally if you haven't already:bash
1npm install -g http-server
2
Then, navigate to your project directory and start the server:
bash
1http-server
2
Open your browser and go to
http://localhost:8080
(or the port number provided by http-server
). You should see your application running, ready to record and display video streams.Debugging Tips
While testing your application, you might encounter some common issues. Here are a few tips to help you troubleshoot:
- Permission Issues: Ensure your browser has permission to access the camera and microphone.
- Console Errors: Check the browser console for any error messages that can give clues about what might be going wrong.
- Network Issues: If you're testing with multiple participants, ensure all participants are on the same network or properly connected via WebRTC signaling.
Conclusion
By following this guide, you've successfully built a RecordRTC WebRTC application that allows users to join a room, start and stop recording, and manage multiple video streams. This application demonstrates the powerful capabilities of WebRTC and RecordRTC for real-time media recording and streaming.
As you continue to develop your application, consider adding more features like chat, file sharing, and advanced video processing. The possibilities with WebRTC and RecordRTC are vast, and with a solid foundation, you can build robust real-time communication applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ