Introduction to LiveKit WebRTC Technology
Real-time audio and video communication have become crucial components in many modern applications, enabling seamless interaction regardless of geographical boundaries. One powerful and flexible solution in this space is LiveKit. Built using the Go programming language and leveraging the Pion WebRTC library, LiveKit offers a robust platform for creating real-time communication applications. Additionally, LiveKit utilizes a Selective Forwarding Unit (SFU) architecture, which significantly enhances performance and scalability.
What is LiveKit WebRTC?
LiveKit is an open-source project designed to facilitate the development of real-time audio and video applications. Its core strength lies in its use of the Go programming language combined with Pion, a Go library for WebRTC. This combination ensures high performance and efficient handling of real-time data.
The SFU architecture used by LiveKit plays a pivotal role in optimizing bandwidth usage. Unlike traditional peer-to-peer (P2P) setups, where each participant's device must send and receive streams to every other participant, SFU centralizes this process. The server selectively forwards streams to participants based on their capabilities and network conditions, thereby reducing overall bandwidth consumption and improving the user experience.
Moreover, LiveKit offers SDKs for multiple platforms, including web, iOS, and Android, making it a versatile choice for developers aiming to build cross-platform real-time communication applications. Its comprehensive documentation and active community support further ease the development process, allowing developers to focus on creating innovative features rather than wrestling with the complexities of real-time communication.
In the following sections, we will delve into the specifics of setting up a LiveKit WebRTC application, providing step-by-step instructions and practical code snippets to help you get started quickly and efficiently. Whether you're building a simple video chat app or a complex real-time collaboration tool, LiveKit offers the flexibility and power needed to bring your vision to life.
Getting Started with the Code
Building a LiveKit WebRTC application involves a series of straightforward steps. In this section, we’ll walk you through creating a new LiveKit WebRTC app, installing necessary dependencies, understanding the project structure, and exploring the app architecture.
Create a New LiveKit WebRTC App
To get started, you'll need to set up a new project for your LiveKit WebRTC application. Ensure you have Go installed on your system, as LiveKit is built using the Go programming language.
[a] Install LiveKit
First, you need to install the LiveKit server package. Open your terminal and run the following command:
bash
1go get github.com/livekit/livekit-server
2
This command will download the LiveKit server package and add it to your project’s dependencies.
[b] Structure of the Project
Understanding the structure of your project is crucial for effective development. A typical LiveKit WebRTC project will have the following directory structure:
Go
1my-livekit-app/
2├── main.go
3├── go.mod
4├── go.sum
5└── internal/
6 ├── handlers/
7 ├── services/
8 └── utils/
9
- main.go: The entry point of your application.
- go.mod: The Go module file.
- go.sum: The checksum file for dependencies.
- internal/: Contains your application’s internal packages.
- handlers/: Handles incoming requests and responses.
- services/: Contains the core logic of your application.
- utils/: Utility functions and helpers.
App Architecture
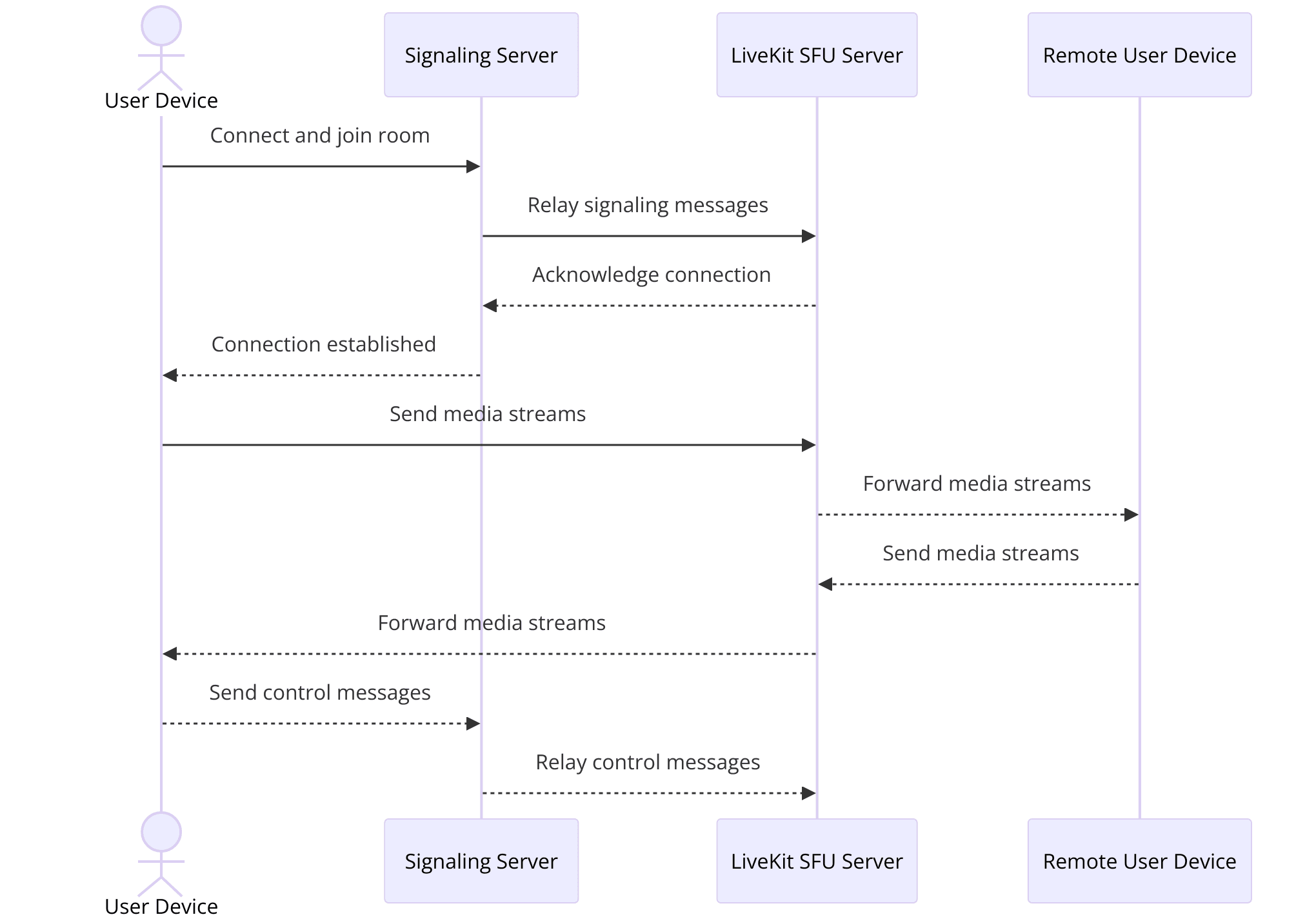
- Selective Forwarding Unit (SFU): The core component that manages media streams.
- Signaling Server: Manages signaling messages used to establish peer connections.
- Media Servers: Handle the actual media streams (audio, video, data).
By utilizing this architecture, LiveKit can handle a large number of participants efficiently, making it ideal for applications such as video conferencing, online classrooms, and live broadcasting.
In the next sections, we will delve deeper into the coding aspects, starting with setting up the main.go file and building the essential components of your LiveKit WebRTC application. Stay tuned as we guide you through each step with practical examples and detailed explanations.
Step 1: Get Started with Main.go
To kick off your LiveKit WebRTC application, you need to set up the main.go file. This file serves as the entry point for your Go application, initializing the LiveKit server and setting up the necessary configurations.
Setting Up Main.go
Start by creating a file named
main.go
in the root directory of your project. This file will contain the code to initialize and start the LiveKit server.[a] Initialize the main.go File
Begin by importing the necessary packages and initializing the LiveKit server. Below is a basic example to help you get started:
Go
1package main
2
3import (
4 "github.com/livekit/livekit-server"
5 "log"
6)
7
8func main() {
9 // Initialize the LiveKit server
10 server, err := livekit.NewServer()
11 if err != nil {
12 log.Fatal(err)
13 }
14
15 // Start the server
16 if err := server.Start(); err != nil {
17 log.Fatal(err)
18 }
19
20 log.Println("LiveKit server started successfully")
21}
22
Explanation of the Code
- Package Declaration: The
main
package indicates that this file is an executable program. - Imports: Importing the
livekit-server
package from LiveKit and thelog
package for logging errors and messages. - Main Function: The
main
function is the entry point of the application. - Server Initialization:
livekit.NewServer()
initializes a new LiveKit server instance. - Error Handling: Check for errors during server initialization and log them.
- Start the Server: Call
server.Start()
to start the server and log a message indicating the server has started successfully.
With this basic setup, your LiveKit server is ready to run. In the next steps, we will wireframe all the necessary components, implement the user interface, and add essential features to your application. Stay tuned as we build a fully functional LiveKit WebRTC application.
Step 2: Wireframe All the Components
After setting up your main.go file and initializing the LiveKit server, the next step is to wireframe the key components of your LiveKit WebRTC application. This step involves planning the essential parts of your application and setting up the basic structure for the UI and backend logic.
Creating the Basic Components
To create a robust and user-friendly LiveKit WebRTC application, you need to define and organize your components effectively. The main components typically include the join screen, user controls, and the participant view.
Wireframing the Components
- Join Screen: This component allows users to enter a room ID and join a session. It includes input fields and a join button.
- User Controls: This component provides controls for muting, toggling video, and other user actions.
- Participant View: This component displays the video and audio streams of all participants in the session.
Example Code Structure
Below is an example of how to organize these components in your project:
Go
1package main
2
3import (
4 "github.com/livekit/livekit-server"
5 "log"
6)
7
8func main() {
9 // Initialize the LiveKit server
10 server, err := livekit.NewServer()
11 if err != nil {
12 log.Fatal(err)
13 }
14
15 // Setup components
16 setupComponents()
17
18 // Start the server
19 if err := server.Start(); err != nil {
20 log.Fatal(err)
21 }
22
23 log.Println("LiveKit server started successfully")
24}
25
26func setupComponents() {
27 // Set up the join screen
28 setupJoinScreen()
29 // Set up user controls
30 setupUserControls()
31 // Set up participant view
32 setupParticipantView()
33}
34
35func setupJoinScreen() {
36 // Code to set up the join screen
37}
38
39func setupUserControls() {
40 // Code to set up user controls
41}
42
43func setupParticipantView() {
44 // Code to set up participant view
45}
46
Explanation of the Code
- Main Function: Initializes the LiveKit server and calls the
setupComponents
function. - setupComponents Function: Calls functions to set up each main component (join screen, user controls, and participant view).
- Component Setup Functions: Placeholder functions (
setupJoinScreen
,setupUserControls
,setupParticipantView
) where you will add the specific code to configure each component.
By organizing your project with these components, you ensure a modular and maintainable codebase. In the following sections, we will dive deeper into implementing each of these components, starting with the join screen. Stay tuned for detailed instructions and code examples to build a fully functional LiveKit WebRTC application.
Step 3: Implement Join Screen
The join screen is a crucial component of your LiveKit WebRTC application. It provides the interface for users to enter a room ID and join a session. Implementing a user-friendly and functional join screen is essential for a smooth user experience.
Creating the Join Screen
The join screen typically includes an input field for the room ID and a button to join the session. Here’s how you can implement it using HTML and JavaScript:
[a] HTML Code for Join Screen
Create an
index.html
file in your project’s public
directory (or equivalent) and add the following code:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>LiveKit Join Screen</title>
7 <link rel="stylesheet" href="styles.css">
8</head>
9<body>
10 <div id="join-screen">
11 <h1>Join a Room</h1>
12 <input type="text" id="room-id" placeholder="Enter Room ID" required>
13 <button id="join-button">Join Room</button>
14 </div>
15 <script src="app.js"></script>
16</body>
17</html>
18
[b] CSS for Styling (Optional)
Create a
styles.css
file in the same directory and add basic styling:CSS
1body {
2 font-family: Arial, sans-serif;
3 display: flex;
4 justify-content: center;
5 align-items: center;
6 height: 100vh;
7 margin: 0;
8 background-color: #f0f0f0;
9}
10
11#join-screen {
12 background-color: white;
13 padding: 20px;
14 border-radius: 5px;
15 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
16}
17
18#join-screen input, #join-screen button {
19 width: 100%;
20 padding: 10px;
21 margin: 5px 0;
22}
23
[c] JavaScript for Handling Join Action
Create an
app.js
file in the same directory and add the following code to handle the join button click event:JavaScript
1document.getElementById('join-button').addEventListener('click', joinRoom);
2
3function joinRoom() {
4 const roomId = document.getElementById('room-id').value;
5 if (roomId) {
6 // Redirect to the room page or initialize WebRTC connection
7 window.location.href = `/room.html?roomId=${roomId}`;
8 } else {
9 alert('Please enter a Room ID');
10 }
11}
12
Explanation of the Code
- HTML Structure: The
index.html
file sets up the basic structure with an input field and a button. - CSS Styling: The
styles.css
file adds simple styling to center the join screen and make it visually appealing. - JavaScript Logic: The
app.js
file contains the logic to capture the room ID input and handle the join action.
With this setup, users can enter a room ID and join a session by clicking the join button. The next steps will cover adding more functionalities and enhancing the user experience in your LiveKit WebRTC application.
Step 4: Implement Controls
Implementing user controls is a key step in developing your LiveKit WebRTC application. Controls allow users to manage their audio and video streams, providing functionality such as muting, unmuting, and toggling the video. These features enhance the user experience and make the application more interactive and user-friendly.
Adding User Controls
To implement the user controls, you need to create buttons for each control and write the corresponding JavaScript functions to handle the actions. Here’s how you can do it:
[a] HTML Code for User Controls
Add the following HTML code to your
room.html
file, which is loaded after the user joins a room:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>LiveKit Room</title>
7 <link rel="stylesheet" href="styles.css">
8</head>
9<body>
10 <div id="controls">
11 <button id="mute-button">Mute</button>
12 <button id="video-button">Stop Video</button>
13 <button id="leave-button">Leave Room</button>
14 </div>
15 <div id="participants"></div>
16 <script src="app.js"></script>
17</body>
18</html>
19
[b] CSS for Styling Controls
Update your
styles.css
file to style the controls:CSS
1#controls {
2 position: fixed;
3 bottom: 20px;
4 left: 50%;
5 transform: translateX(-50%);
6 background: rgba(0, 0, 0, 0.7);
7 padding: 10px;
8 border-radius: 5px;
9}
10
11#controls button {
12 margin: 0 5px;
13 padding: 10px;
14 background: white;
15 border: none;
16 border-radius: 5px;
17 cursor: pointer;
18}
19
[c] JavaScript for Handling Controls
Add the following JavaScript code to your
app.js
file to handle the control actions:JavaScript
1document.getElementById('mute-button').addEventListener('click', toggleMute);
2document.getElementById('video-button').addEventListener('click', toggleVideo);
3document.getElementById('leave-button').addEventListener('click', leaveRoom);
4
5function toggleMute() {
6 const isMuted = localStream.getAudioTracks()[0].enabled;
7 localStream.getAudioTracks()[0].enabled = !isMuted;
8 document.getElementById('mute-button').textContent = isMuted ? 'Unmute' : 'Mute';
9}
10
11function toggleVideo() {
12 const isVideoOn = localStream.getVideoTracks()[0].enabled;
13 localStream.getVideoTracks()[0].enabled = !isVideoOn;
14 document.getElementById('video-button').textContent = isVideoOn ? 'Start Video' : 'Stop Video';
15}
16
17function leaveRoom() {
18 // Code to leave the room and disconnect
19 window.location.href = '/';
20}
21
Explanation of the Code
- HTML Structure: The
room.html
file includes buttons for muting, stopping video, and leaving the room. - CSS Styling: The
styles.css
file styles the controls to be fixed at the bottom of the screen, centered, and visually appealing. - JavaScript Logic: The
app.js
file contains the logic for toggling the mute and video states and leaving the room. ThetoggleMute
andtoggleVideo
functions update the text content of the buttons based on the current state.
By implementing these controls, you provide users with essential functionalities to manage their audio and video streams, making your LiveKit WebRTC application more interactive and user-friendly. In the next step, we will focus on displaying participants in the room.
Step 5: Implement Participant View
The participant view is a crucial part of your LiveKit WebRTC application, allowing users to see and interact with other participants in the room. This involves dynamically displaying video and audio streams for each participant and updating the view as participants join or leave.
Displaying Participants
To implement the participant view, you need to dynamically create video elements for each participant and manage their streams. Here's how to do it:
[a] HTML Code for Participant View
Ensure your
room.html
file has a container to hold the participant video elements:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>LiveKit Room</title>
7 <link rel="stylesheet" href="styles.css">
8</head>
9<body>
10 <div id="controls">
11 <button id="mute-button">Mute</button>
12 <button id="video-button">Stop Video</button>
13 <button id="leave-button">Leave Room</button>
14 </div>
15 <div id="participants"></div>
16 <script src="app.js"></script>
17</body>
18</html>
19
[b] CSS for Styling Participant View
Update your
styles.css
file to style the participant video elements:CSS
1#participants {
2 display: flex;
3 flex-wrap: wrap;
4 justify-content: center;
5 margin-top: 20px;
6}
7
8#participants video {
9 margin: 10px;
10 border: 1px solid #ddd;
11 border-radius: 5px;
12 width: 200px;
13 height: 150px;
14}
15
[c] JavaScript for Handling Participant Streams
Add the following JavaScript code to your
app.js
file to manage participant streams:JavaScript
1let localStream;
2const participants = {};
3
4document.addEventListener('DOMContentLoaded', () => {
5 joinRoom();
6});
7
8function joinRoom() {
9 const roomId = new URLSearchParams(window.location.search).get('roomId');
10 if (roomId) {
11 // Initialize the WebRTC connection and get the local stream
12 navigator.mediaDevices.getUserMedia({ video: true, audio: true }).then(stream => {
13 localStream = stream;
14 displayParticipant('local', stream);
15
16 // Simulate adding remote participants (replace this with actual WebRTC implementation)
17 addRemoteParticipant('remote1');
18 addRemoteParticipant('remote2');
19 });
20 }
21}
22
23function displayParticipant(id, stream) {
24 const videoElement = document.createElement('video');
25 videoElement.id = id;
26 videoElement.srcObject = stream;
27 videoElement.autoplay = true;
28 videoElement.playsInline = true;
29
30 const participantsContainer = document.getElementById('participants');
31 participantsContainer.appendChild(videoElement);
32}
33
34function addRemoteParticipant(id) {
35 // Simulate remote participant stream
36 navigator.mediaDevices.getUserMedia({ video: true, audio: true }).then(stream => {
37 participants[id] = stream;
38 displayParticipant(id, stream);
39 });
40}
41
Explanation of the Code
- HTML Structure: The
room.html
file includes adiv
with the IDparticipants
to hold video elements for each participant. - CSS Styling: The
styles.css
file styles the participant video elements to be displayed in a flexible, wrapping layout with some margin and border styling. - JavaScript Logic: The
app.js
file manages the WebRTC streams and dynamically adds video elements for each participant. ThejoinRoom
function initializes the local stream and simulates adding remote participants. ThedisplayParticipant
function creates video elements for each participant and appends them to theparticipants
container.
With this setup, your LiveKit WebRTC application will dynamically display video streams for all participants in the room. In the next and final step, we will cover running your application and provide a conclusion with FAQs to wrap up the article.
Step 6: Run Your Code Now
With all the components set up, it's time to run your LiveKit WebRTC application. Ensure you have Go installed on your system and the necessary dependencies installed. Open your terminal in the project directory and run the following command:
bash
1go run main.go
2
This command will start the LiveKit server, allowing you to access your application. Open your browser and navigate to
http://localhost:8080
(or the appropriate URL based on your server configuration). Enter a Room ID on the join screen, and you should see the participant view with your video stream.Conclusion
Building a LiveKit WebRTC application involves several steps, from setting up the main Go server file to implementing the join screen, user controls, and participant view. By following this guide, you have created a functional real-time communication app using LiveKit's powerful and flexible platform. This setup provides a solid foundation for further customization and enhancement to suit your specific needs.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ