Introduction to Ant Media Server WebRTC
What is Ant Media Server?
Ant Media Server is a powerful and scalable streaming engine meticulously designed to deliver low-latency live streams using various protocols such as WebRTC, RTMP, RTSP, and HLS. This robust media server supports adaptive bitrate streaming, ensuring optimal viewing experiences across different network conditions, and provides comprehensive tools for recording, broadcasting, and transcoding live streams. With its extensive feature set, Ant Media Server is an ideal solution for developers looking to implement robust and scalable live streaming applications. It serves as a powerful streaming engine for a variety of use cases.
Overview of WebRTC Technology
WebRTC (Web Real-Time Communication) is an open-source project that enables web applications and websites to capture and stream audio and video, and share data directly between browsers and devices without requiring an intermediary server. This technology is crucial for real-time communications like video conferencing, live streaming, and peer-to-peer file sharing. Its ability to provide high-quality, low-latency streaming makes it an essential tool for modern web and mobile applications demanding real-time interaction. A key benefit of WebRTC is its peer-to-peer nature, reducing server load in many applications.
Why Use Ant Media Server for WebRTC?
Combining the strengths of Ant Media Server with WebRTC technology offers a powerful and versatile platform for live streaming, particularly for applications requiring low-latency streaming. Ant Media Server enhances WebRTC by providing a scalable infrastructure capable of handling numerous simultaneous connections, adaptive bitrate streaming to optimize stream quality dynamically, and support for various codecs and protocols, including those optimized for WebRTC. This makes it an excellent choice for developers seeking to build high-performance, real-time communication applications. Furthermore, Ant Media Server can address WebRTC scalability challenges through load balancing and server clustering. Whether you're developing a video conferencing app, live broadcasting platform, or an interactive streaming service, Ant Media Server with WebRTC provides the tools and reliability you need. Ant Media Server also helps manage the complexities of WebRTC setup.
Getting Started with the Code!
Create a New Ant Media Server WebRTC App
To start with Ant Media Server and WebRTC, you'll need to set up your development environment. Ensure Java is installed on your system, as Ant Media Server is built using Java. You can download the latest version from the
official Java website
.[a] Clone the Ant Media Server repository
bash
1git clone https://github.com/ant-media/Ant-Media-Server.git
2
[b] Navigate to the project directory
bash
1cd Ant-Media-Server
2
Install Ant Media Server
To install Ant Media Server, follow these steps:
[a] Download the latest release
Visit the
Ant Media Server releases page
and download the appropriate version for your operating system.[b] Install the server
For Linux
bash
1sudo unzip ant-media-server-*.zip -d /usr/local/antmedia
2cd /usr/local/antmedia
3sudo ./start.sh
4
For Windows
- Unzip the downloaded file to a directory of your choice.
- Open a command prompt, navigate to the unzipped directory, and run
start.bat
.
Structure of the Project
Understanding the project structure is crucial for efficient development. The main components include:
- webapps: Contains the web applications and their configurations.
- conf: Holds configuration files for the server.
- logs: Directory where log files are stored.
- plugins: Directory for server plugins.
App Architecture
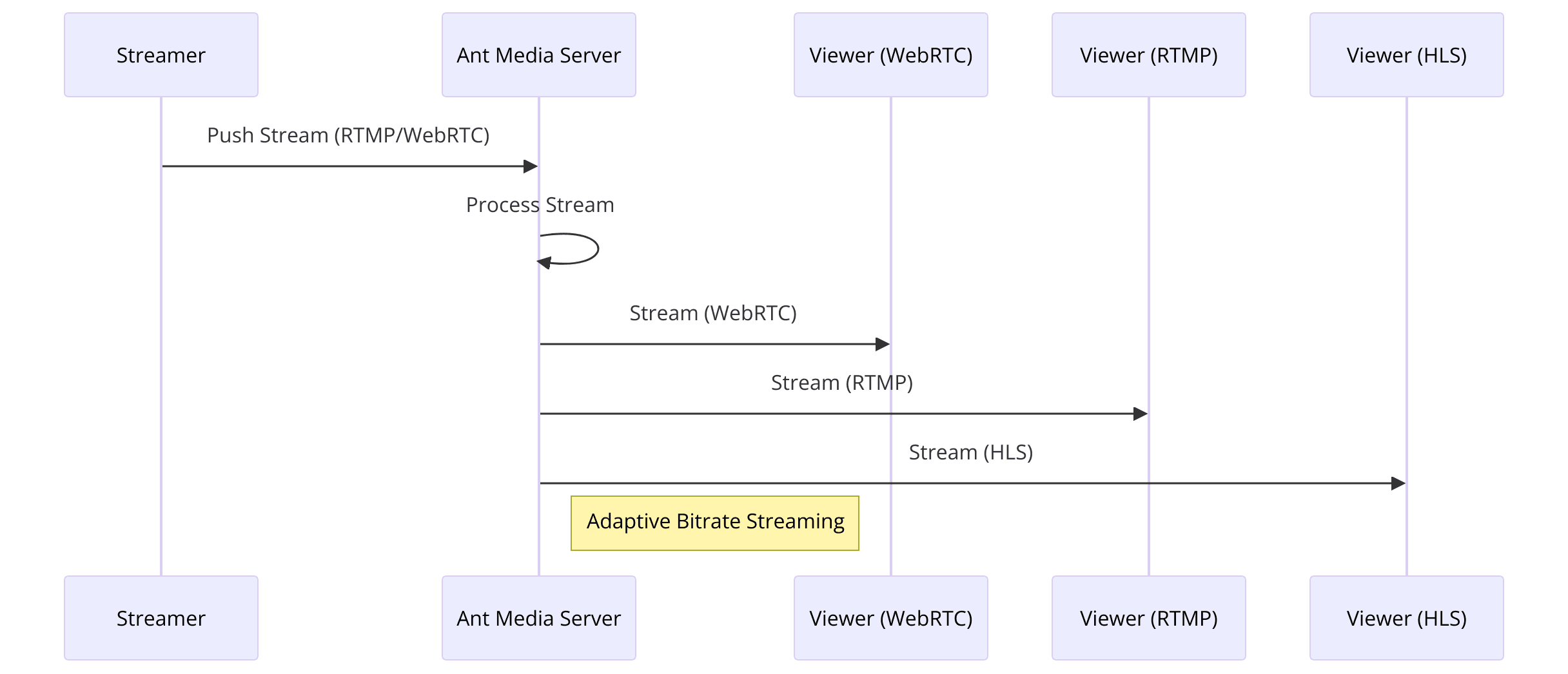
- Java-based backend: Manages streaming sessions, user authentication, and data storage.
- WebRTC integration: Ensures real-time communication with minimal latency.
- Adaptive bitrate streaming: Adjusts the stream quality based on the user's network conditions.
By following these steps, you can set up and start developing your Ant Media Server WebRTC application, leveraging its powerful features for your streaming needs.
Step 1: Setting Up the Environment
Initial Setup and Configuration
To get started with Ant Media Server and WebRTC, you need to set up your development environment. Ensure you have the necessary tools and dependencies installed. Here's a step-by-step guide to help you with the Ant Media Server WebRTC setup:
[a] Install Java
Ant Media Server is built using Java. Ensure you have the latest version of Java Development Kit (JDK) installed. You can download it from the official Java website.
bash
1 sudo apt-get update
2 sudo apt-get install openjdk-11-jdk
3
[b] Clone the Ant Media Server Repository
Use Git to clone the Ant Media Server repository from GitHub.
bash
1 git clone https://github.com/ant-media/Ant-Media-Server.git
2 cd Ant-Media-Server
3
Build the Project
Navigate to the project directory and build the project using Maven. Maven is a build automation tool used primarily for Java projects.
bash
1 ./gradlew build
2
Configuring Ant Media Server
After setting up the environment, configure Ant Media Server to enable WebRTC functionalities. This involves editing configuration files and ensuring the server is properly started.
[a] Edit Configuration Files
Navigate to the configuration directory and edit the necessary configuration files to suit your needs.
bash
1 cd conf
2 nano red5.properties
3
[b] Enable WebRTC
Ensure WebRTC is enabled in the configuration. You can find the relevant settings in the
red5.properties
file. Set webrtc_enabled
to true
.1 webrtc_enabled=true
2
[c] Start the Server
Finally, start the Ant Media Server to apply the configurations.
bash
1 ./start.sh
2
Now your environment is set up, and the Ant Media Server is configured to support WebRTC. It's important to consult the Ant Media Server documentation for specific configuration options related to WebRTC.
Step 2: Wireframing All Components
Designing the User Interface
Creating a functional and intuitive user interface (UI) is essential for a successful WebRTC application. Start by wireframing the UI to visualize the layout and interactions. The key components for a WebRTC application using Ant Media Server include a join screen, video display area, controls (mute, unmute, etc.), and participant list. These components are crucial for a seamless user experience.
Join Screen
1- **Elements:** Username input, Join button.
2- **Purpose:** Allows users to enter a username and join the video session.
Video Display Area
1- **Elements:** Video streams of participants.
2- **Purpose:** Displays the live video feeds of the connected participants.
Controls
1- **Elements:** Mute/Unmute button, Start/Stop video button, Screen sharing button.
2- **Purpose:** Provides essential functionalities for managing the video and audio streams.
Participant List
1- **Elements:** List of participants, their status (muted/unmuted).
2- **Purpose:** Shows the current participants in the session and their status.
Component Breakdown
Let's break down the components further to understand their roles and interactions:
[a] Join Screen Implementation
HTML
1 <div id="join-screen">
2 <input type="text" id="username" placeholder="Enter your username">
3 <button id="join-btn">Join</button>
4 </div>
5
1
[b] Video Display Area Implementation
HTML
1 <div id="video-container">
2 <!-- Video streams will be dynamically added here -->
3 </div>
4
1
[c] Controls Implementation
HTML
1 <div id="controls">
2 <button id="mute-btn">Mute</button>
3 <button id="video-btn">Stop Video</button>
4 <button id="share-btn">Share Screen</button>
5 </div>
6
1
[d] Participant List Implementation
HTML
1 <div id="participant-list">
2 <!-- Participant list will be dynamically updated here -->
3 </div>
4
By wireframing these components, you create a clear blueprint for the UI, ensuring all necessary elements are included and properly arranged. This approach helps streamline the development process and provides a solid foundation for implementing the WebRTC application with Ant Media Server. This initial design phase is crucial for a successful project.
Step 3: Implementing the Join Screen
Creating the Join Screen UI
The join screen is the entry point for users to enter their names and join the video session. Implementing a simple yet effective join screen ensures a smooth user experience. Here's how to set up the join screen using HTML, CSS, and JavaScript.
[a] HTML Structure
HTML
1 <div id="join-screen">
2 <input type="text" id="username" placeholder="Enter your username">
3 <button id="join-btn">Join</button>
4 </div>
5 <div id="video-container" style="display:none;">
6 <!-- Video streams will be dynamically added here -->
7 </div>
8
[b] CSS Styling
CSS
1 #join-screen {
2 display: flex;
3 flex-direction: column;
4 align-items: center;
5 margin-top: 50px;
6 }
7 #username {
8 padding: 10px;
9 margin-bottom: 10px;
10 width: 200px;
11 }
12 #join-btn {
13 padding: 10px 20px;
14 cursor: pointer;
15 }
16
[c] JavaScript for Handling User Inputs
JavaScript
1 document.getElementById('join-btn').addEventListener('click', function() {
2 const username = document.getElementById('username').value;
3 if (username) {
4 joinSession(username);
5 } else {
6 alert('Please enter a username.');
7 }
8 });
9
10 function joinSession(username) {
11 // Logic to join the WebRTC session
12 document.getElementById('join-screen').style.display = 'none';
13 document.getElementById('video-container').style.display = 'block';
14 // Initialize WebRTC connection here
15 }
16
Handling User Inputs
The join screen collects the username input from the user and initiates the process to join the WebRTC session. The JavaScript code handles the click event on the "Join" button, retrieves the username, and calls the
joinSession
function.[a] Validating Username Input
Ensure the username input is not empty before proceeding. Display an alert if the input is invalid.
JavaScript
1 if (username) {
2 joinSession(username);
3 } else {
4 alert('Please enter a username.');
5 }
6
[b] Joining the WebRTC Session
The
joinSession
function hides the join screen and displays the video container. This is where you will initialize the WebRTC connection using Ant Media Server's SDK.JavaScript
1 function joinSession(username) {
2 // Logic to join the WebRTC session
3 document.getElementById('join-screen').style.display = 'none';
4 document.getElementById('video-container').style.display = 'block';
5 // Initialize WebRTC connection here
6 }
7
, you create a user-friendly entry point for users to join your WebRTC session. This setup lays the groundwork for further development of the video streaming application using Ant Media Server.
Step 4: Implementing Container and Controls
Setting Up the Main Container
The main container is crucial for organizing video streams and control elements. It ensures that the UI remains structured and user-friendly, especially as new participants join or leave the session. Here's how to set up the main container:
[a] HTML Structure
HTML
1 <div id="main-container">
2 <div id="video-container">
3 <!-- Video streams will be dynamically added here -->
4 </div>
5 <div id="controls">
6 <button id="mute-btn">Mute</button>
7 <button id="video-btn">Stop Video</button>
8 <button id="share-btn">Share Screen</button>
9 </div>
10 </div>
11
[b] CSS Styling
CSS
1 #main-container {
2 display: flex;
3 flex-direction: column;
4 align-items: center;
5 margin-top: 20px;
6 }
7 #video-container {
8 display: flex;
9 flex-wrap: wrap;
10 justify-content: center;
11 width: 80%;
12 margin-bottom: 20px;
13 }
14 #controls {
15 display: flex;
16 justify-content: center;
17 }
18 #controls button {
19 margin: 0 10px;
20 padding: 10px 20px;
21 cursor: pointer;
22 }
23
Adding Controls and Features
Adding controls allows users to manage their audio and video streams, enhancing the interaction and usability of the application. Below is the JavaScript implementation for these controls:
[a] JavaScript for Controls
JavaScript
1 document.getElementById('mute-btn').addEventListener('click', function() {
2 const isMuted = toggleMute();
3 this.textContent = isMuted ? 'Unmute' : 'Mute';
4 });
5
6 document.getElementById('video-btn').addEventListener('click', function() {
7 const isVideoStopped = toggleVideo();
8 this.textContent = isVideoStopped ? 'Start Video' : 'Stop Video';
9 });
10
11 document.getElementById('share-btn').addEventListener('click', function() {
12 shareScreen();
13 });
14
15 function toggleMute() {
16 // Logic to mute/unmute audio
17 let isMuted = false;
18 // Implement mute/unmute functionality
19 return isMuted;
20 }
21
22 function toggleVideo() {
23 // Logic to stop/start video
24 let isVideoStopped = false;
25 // Implement stop/start video functionality
26 return isVideoStopped;
27 }
28
29 function shareScreen() {
30 // Logic to share screen
31 // Implement screen sharing functionality
32 }
33
[b] Handling Audio and Video Controls
- Mute/Unmute:
JavaScript
1 function toggleMute() {
2 let isMuted = false;
3 // Access the local stream and toggle audio track
4 localStream.getAudioTracks()[0].enabled = !localStream.getAudioTracks()[0].enabled;
5 isMuted = !localStream.getAudioTracks()[0].enabled;
6 return isMuted;
7 }
8
1
- Stop/Start Video:
JavaScript
1 function toggleVideo() {
2 let isVideoStopped = false;
3 // Access the local stream and toggle video track
4 localStream.getVideoTracks()[0].enabled = !localStream.getVideoTracks()[0].enabled;
5 isVideoStopped = !localStream.getVideoTracks()[0].enabled;
6 return isVideoStopped;
7 }
8
- Share Screen:
JavaScript
1 async function shareScreen() {
2 try {
3 const screenStream = await navigator.mediaDevices.getDisplayMedia({ video: true });
4 // Replace the current video track with the screen stream track
5 localStream.getVideoTracks()[0].stop();
6 localStream.removeTrack(localStream.getVideoTracks()[0]);
7 localStream.addTrack(screenStream.getVideoTracks()[0]);
8 } catch (error) {
9 console.error("Error sharing screen: ", error);
10 }
11 }
12
By setting up the main container and adding essential controls, you provide users with the ability to manage their participation in the WebRTC session effectively. These features enhance the interactivity and user experience of your application, making it more engaging and functional.
Security Considerations
When implementing WebRTC applications with Ant Media Server, it's vital to consider security best practices. Encrypting your streams using DTLS (Datagram Transport Layer Security) is crucial for protecting the confidentiality of your communication. Implement robust authentication methods to verify the identity of users joining the session, preventing unauthorized access. Regularly update Ant Media Server to patch any security vulnerabilities. Input validation is also essential to prevent injection attacks.
Troubleshooting Common Issues
Encountering issues when using Ant Media Server with WebRTC is not uncommon. Connectivity problems might arise due to firewall restrictions or network configurations. Codec compatibility issues can occur if the browser and server do not support the same codecs. To address performance bottlenecks, monitor CPU and bandwidth usage, and consider optimizing the video resolution and bitrate. Consulting the Ant Media Server documentation and community forums can provide valuable troubleshooting tips.
Step 5: Implementing Participant View
Displaying Participants
To create an engaging and interactive WebRTC application, displaying the video streams of all participants is essential. Implementing a dynamic participant view ensures that each user's video feed is shown and updated in real-time as they join or leave the session.
[a] HTML Structure for Participant View
HTML
1 <div id="participant-view">
2 <!-- Participant video elements will be dynamically added here -->
3 </div>
4
[b] CSS Styling for Participant View
1 #participant-view {
2 display: flex;
3 flex-wrap: wrap;
4 justify-content: center;
5 }
6 .participant {
7 margin: 10px;
8 border: 1px solid #ccc;
9 }
10 video {
11 width: 300px;
12 height: auto;
13 }
14
Managing Participant Data
JavaScript will handle the addition and removal of participant video elements dynamically. Use WebRTC to manage the streams and Ant Media Server to handle the signaling and connection setup.
[a] JavaScript for Managing Participants
JavaScript
1 const participantView = document.getElementById('participant-view');
2
3 function addParticipant(participantId, stream) {
4 const videoElement = document.createElement('video');
5 videoElement.id = participantId;
6 videoElement.srcObject = stream;
7 videoElement.autoplay = true;
8 videoElement.className = 'participant';
9 participantView.appendChild(videoElement);
10 }
11
12 function removeParticipant(participantId) {
13 const videoElement = document.getElementById(participantId);
14 if (videoElement) {
15 videoElement.srcObject.getTracks().forEach(track => track.stop());
16 videoElement.remove();
17 }
18 }
19
[b] Handling Participant Events
Adding a New Participant:
JavaScript
1 // Example: When a new participant joins
2 signalingServer.on('newParticipant', (participantId, stream) => {
3 addParticipant(participantId, stream);
4 });
5
Removing a Participant:
JavaScript
1 // Example: When a participant leaves
2 signalingServer.on('participantLeft', participantId => {
3 removeParticipant(participantId);
4 });
5
Implementing the WebRTC Stream Handling
To manage the streams, you need to handle the WebRTC connection setup and stream events using Ant Media Server's WebRTC functionalities.
JavaScript for WebRTC Stream Handling
JavaScript
1 const localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
2 addParticipant('local', localStream);
3
4 const peerConnection = new RTCPeerConnection();
5
6 localStream.getTracks().forEach(track => {
7 peerConnection.addTrack(track, localStream);
8 });
9
10 peerConnection.ontrack = (event) => {
11 const [stream] = event.streams;
12 addParticipant(event.track.id, stream);
13 };
14
15 // Signaling logic to connect peers using Ant Media Server's signaling API
16 signalingServer.on('offer', async (offer) => {
17 await peerConnection.setRemoteDescription(new RTCSessionDescription(offer));
18 const answer = await peerConnection.createAnswer();
19 await peerConnection.setLocalDescription(answer);
20 signalingServer.emit('answer', answer);
21 });
22
23 signalingServer.on('answer', async (answer) => {
24 await peerConnection.setRemoteDescription(new RTCSessionDescription(answer));
25 });
26
27 signalingServer.on('iceCandidate', async (candidate) => {
28 await peerConnection.addIceCandidate(new RTCIceCandidate(candidate));
29 });
30
31 peerConnection.onicecandidate = (event) => {
32 if (event.candidate) {
33 signalingServer.emit('iceCandidate', event.candidate);
34 }
35 };
36
By implementing the participant view and managing participant data dynamically, you ensure that your WebRTC application provides a seamless and interactive experience. This setup allows users to see and interact with each other in real-time, making the application more engaging and functional.
Step 6: Running Your Code Now
With all components in place, it’s time to run your WebRTC application using Ant Media Server. Ensure your server is up and running and that all configurations are correctly set.
Start Ant Media Server
bash
1 ./start.sh
2
Open Your Application
Open your web browser and navigate to the URL where your application is hosted. For example:
1 http://localhost:5080/YourAppName
2
Join the Session
Enter your username on the join screen and click "Join". You should now be able to see your video stream and those of other participants in real-time.
Conclusion
Ant Media Server with WebRTC offers a powerful solution for real-time communication applications. By following the steps outlined in this guide, you can set up, configure, and run a robust WebRTC application, enabling low-latency video streaming and interactive features.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ