Introduction to AIOQUIC and WebRTC Technology
In today's digital landscape, seamless and efficient real-time communication is paramount. WebRTC (Web Real-Time Communication) is a powerful technology that enables peer-to-peer audio, video, and data sharing directly between browsers and mobile applications. However, achieving optimal performance requires robust transport protocols, which is where QUIC (Quick UDP Internet Connections) comes into play. QUIC, a modern transport protocol designed by Google, enhances connection speed and reliability by using UDP for multiplexed connections.
What is AIOQUIC WebRTC Technology?
AIOQUIC is a Python library that implements the QUIC and HTTP/3 protocols, making it an ideal choice for developers looking to integrate QUIC with WebRTC. The combination of AIOQUIC and WebRTC offers a high-performance, low-latency communication solution that is both secure and scalable. This article will guide you through the process of setting up a WebRTC application using the AIOQUIC library, leveraging the power of Python and the latest advancements in internet protocols. For more details, you can explore the
AIOQUIC GitHub repository
.Getting Started with AIOQUIC WebRTC Integration
Create a New AIOQUIC WebRTC App
To begin integrating AIOQUIC with WebRTC, the first step is to set up a new project. This involves creating a project directory and initializing it with necessary files and configurations.
[a] Create Project Directory
bash
1 mkdir aioquic-webrtc-app
2 cd aioquic-webrtc-app
[b] Initialize a Python Virtual Environment
bash
1 python -m venv venv
2 source venv/bin/activate # On Windows use `venv\Scripts\activate`
Install Required Dependencies
With your project directory set up, the next step is to install AIOQUIC and other dependencies necessary for your WebRTC application.
[a] Install aioquic and Related Libraries
bash
1 pip install aioquic aiortc
[b] Install Additional Dependencies
You might need additional packages for handling asynchronous operations and other WebRTC functionalities:
bash
1 pip install asyncio
2 pip install websockets
Structure of the Project
Creating a well-organized project structure is crucial for maintainability and scalability. Here’s a recommended structure for your AIOQUIC WebRTC application:
Python
1aioquic-webrtc-app/
2├── app/
3│ ├── __init__.py
4│ ├── signaling.py
5│ ├── webrtc.py
6├── static/
7│ ├── index.html
8│ ├── style.css
9│ ├── app.js
10├── tests/
11│ ├── test_signaling.py
12│ ├── test_webrtc.py
13├── main.py
14├── requirements.txt
App Architecture
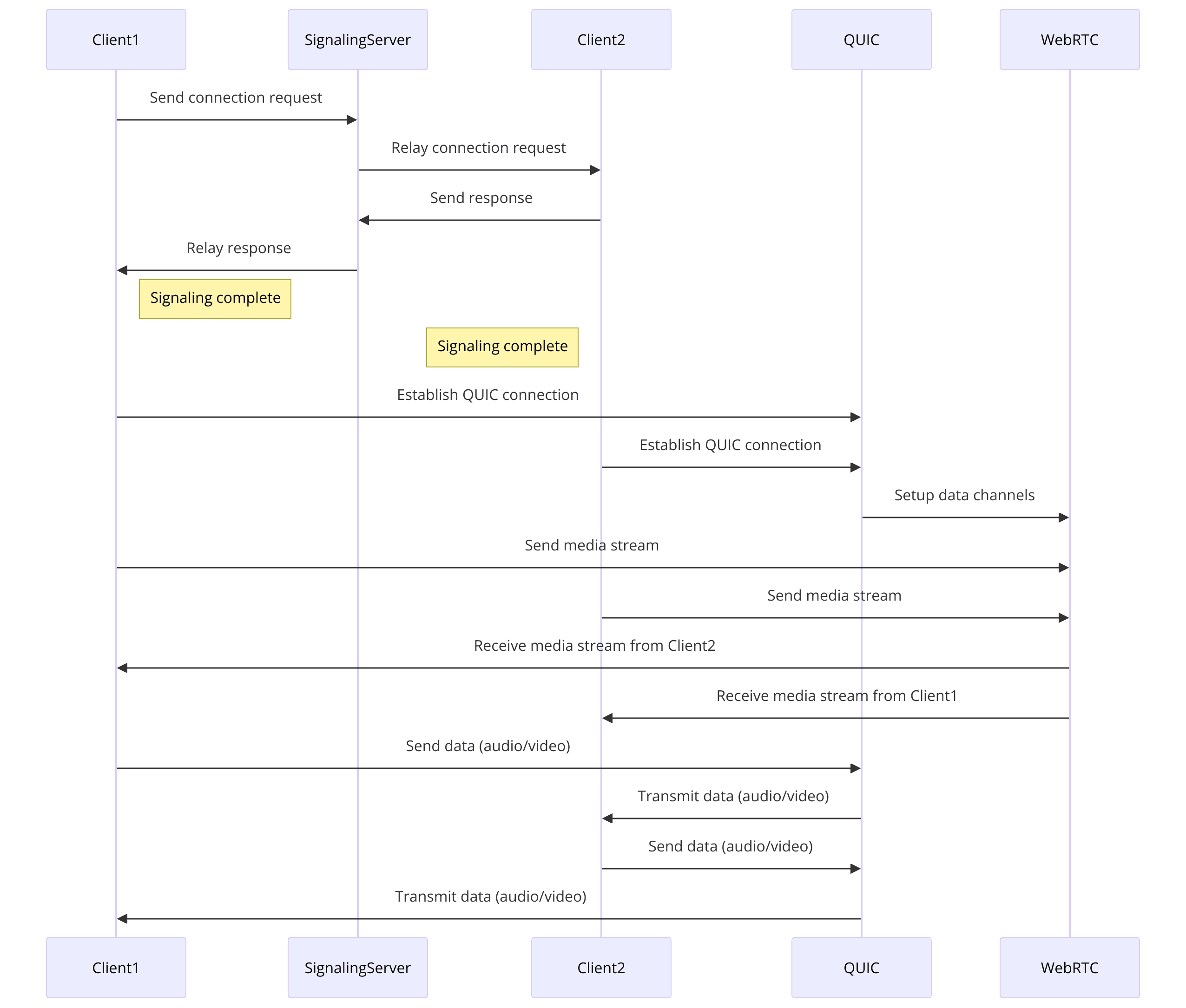
- Signaling: Handles the exchange of connection information between peers.
- WebRTC: Manages the real-time communication aspects, such as media stream handling and peer connections.
- Static Files: Contains HTML, CSS, and JavaScript files for the client-side application.
- Tests: Includes unit tests to ensure each component works correctly.
With these steps, you have laid the foundation for your AIOQUIC WebRTC application. In the next sections, we will dive deeper into implementing each part of the application.
Step 1: Setting Up the Initial File Structure
To start building your AIOQUIC WebRTC application, you need to set up the initial file structure and create the necessary files. This setup will serve as the backbone of your project, allowing you to organize and manage your code efficiently.
Creating Initial Files and Folders
[a] Project Directory Structure
Begin by creating the directories and files as outlined in the project structure.
bash
1 mkdir -p aioquic-webrtc-app/{app,static,tests}
2 touch aioquic-webrtc-app/{app/__init__.py,app/signaling.py,app/webrtc.py,static/index.html,static/style.css,static/app.js,tests/test_signaling.py,tests/test_webrtc.py,main.py,requirements.txt}
[b] Basic Setup Script
In the
main.py
file, set up a basic script to initialize the application.Python
1 # main.py
2 import asyncio
3 from aioquic.asyncio import QuicConnectionProtocol, connect
4 from aioquic.asyncio.protocol import QuicServer
5
6 async def main():
7 print("Starting AIOQUIC WebRTC application")
8
9 if __name__ == "__main__":
10 asyncio.run(main())
Writing the Basic Setup Script
This basic setup script initializes your application and sets the stage for further development. The
main()
function will eventually handle the initialization of your signaling server, WebRTC connections, and other necessary components.[c] Installing Dependencies
Ensure all dependencies are listed in the
requirements.txt
file.1 aioquic
2 aiortc
3 asyncio
4 websockets
[d] Installing Dependencies
Run the following command to install the listed dependencies.
bash
1 pip install -r requirements.txt
Sample Code Snippets
The initial script is simple but essential. It provides a foundation upon which you will build more complex functionalities. Here’s a closer look at the
main.py
setup:Python
1import asyncio
2
3async def main():
4 print("Starting AIOQUIC WebRTC application")
5
6if __name__ == "__main__":
7 asyncio.run(main())
With this setup, you have created the foundational structure of your AIOQUIC WebRTC application. In the following steps, you will add more functionality to build a fully operational WebRTC application using AIOQUIC.
Step 2: Wireframe All Components
With the initial setup in place, the next step is to wireframe all the key components of your AIOQUIC WebRTC application. This involves designing the core modules and ensuring they can interact seamlessly. The primary components include signaling, WebRTC functionality, and the client-side interface.
Designing the Core Components
[a] Signaling Component
The signaling server facilitates the exchange of connection information between peers. It is essential for establishing the initial WebRTC connections.
Python
1 # app/signaling.py
2 import asyncio
3 import websockets
4
5 async def signaling_server(websocket, path):
6 async for message in websocket:
7 print(f"Received message: {message}")
8 await websocket.send(f"Echo: {message}")
9
10 start_server = websockets.serve(signaling_server, "localhost", 8765)
11
12 asyncio.get_event_loop().run_until_complete(start_server)
13 asyncio.get_event_loop().run_forever()
[b] WebRTC Component
This module handles the real-time communication aspects, such as media stream handling and peer connections.
Python
1 # app/webrtc.py
2 from aiortc import RTCPeerConnection, RTCSessionDescription
3 from aiortc.contrib.signaling import BYE
4
5 async def run(pc, signaling):
6 await signaling.connect()
7
8 @pc.on("datachannel")
9 def on_datachannel(channel):
10 @channel.on("message")
11 def on_message(message):
12 print(f"Received message: {message}")
13 if message == BYE:
14 print("Received BYE, ending session")
15 signaling.close()
16
17 # Continue with additional WebRTC setup...
[c] Client-Side Interface
HTML, CSS, and JavaScript files to provide a user interface for interacting with the WebRTC application.
HTML
1 <!-- static/index.html -->
2 <!DOCTYPE html>
3 <html lang="en">
4 <head>
5 <meta charset="UTF-8">
6 <title>AIOQUIC WebRTC App</title>
7 <link rel="stylesheet" href="style.css">
8 </head>
9 <body>
10 <h1>Welcome to AIOQUIC WebRTC App</h1>
11 <button id="join">Join</button>
12 <script src="app.js"></script>
13 </body>
14 </html>
CSS
1 /* static/style.css */
2 body {
3 font-family: Arial, sans-serif;
4 display: flex;
5 justify-content: center;
6 align-items: center;
7 height: 100vh;
8 background-color: #f0f0f0;
9 }
10
11 button {
12 padding: 10px 20px;
13 font-size: 16px;
14 }
JavaScript
1 // static/app.js
2 document.getElementById('join').addEventListener('click', () => {
3 const ws = new WebSocket('ws://localhost:8765');
4 ws.onopen = () => {
5 ws.send('Hello Server');
6 };
7 ws.onmessage = (event) => {
8 console.log(`Received: ${event.data}`);
9 };
10 });
Example Code Snippets for Each Component
The example code snippets above illustrate the basic implementation of each component. These components will form the backbone of your AIOQUIC WebRTC application, enabling real-time communication and interaction.
By wireframing these components, you establish a clear structure for your application, making it easier to develop, debug, and scale. The next steps will involve fleshing out these components with more detailed functionality and integration.
Step 3: Implementing the Join Screen
In this step, we'll create a user-friendly interface for users to join the WebRTC session. The join screen is crucial as it serves as the entry point for participants to connect and start interacting.
Creating the Join Screen
[a] HTML Structure
The HTML file will contain the basic structure for the join screen, including a button for users to click and join the session.
HTML
1 <!-- static/index.html -->
2 <!DOCTYPE html>
3 <html lang="en">
4 <head>
5 <meta charset="UTF-8">
6 <title>AIOQUIC WebRTC App</title>
7 <link rel="stylesheet" href="style.css">
8 </head>
9 <body>
10 <div class="container">
11 <h1>Join the AIOQUIC WebRTC Session</h1>
12 <button id="join">Join</button>
13 </div>
14 <script src="app.js"></script>
15 </body>
16 </html>
[b] Styling the Join Screen
Use CSS to style the join screen for a better user experience.
CSS
1 /* static/style.css */
2 body {
3 font-family: Arial, sans-serif;
4 display: flex;
5 justify-content: center;
6 align-items: center;
7 height: 100vh;
8 background-color: #f0f0f0;
9 margin: 0;
10 }
11
12 .container {
13 text-align: center;
14 }
15
16 button {
17 padding: 10px 20px;
18 font-size: 16px;
19 cursor: pointer;
20 background-color: #4CAF50;
21 color: white;
22 border: none;
23 border-radius: 5px;
24 margin-top: 20px;
25 }
26
27 button:hover {
28 background-color: #45a049;
29 }
[c] JavaScript for Join Functionality
Implement the JavaScript to handle the join button click event and establish a WebSocket connection.
JavaScript
1 // static/app.js
2 document.getElementById('join').addEventListener('click', () => {
3 const ws = new WebSocket('ws://localhost:8765');
4 ws.onopen = () => {
5 console.log('WebSocket connection opened');
6 ws.send('Joining the WebRTC session');
7 };
8 ws.onmessage = (event) => {
9 console.log(`Received: ${event.data}`);
10 };
11 ws.onclose = () => {
12 console.log('WebSocket connection closed');
13 };
14 ws.onerror = (error) => {
15 console.error(`WebSocket error: ${error}`);
16 };
17 });
Step-by-Step Instructions
- HTML: Create a
div
container with a heading and a button for joining the session. - CSS: Style the page to center the content and style the button with hover effects.
- JavaScript: Add an event listener to the join button to initiate a WebSocket connection when clicked.
This implementation provides a simple and intuitive interface for users to join the WebRTC session, setting the stage for real-time communication. In the next step, we will add control functionalities to enhance the user experience further.
Step 4: Implementing Controls
Implementing control functionalities in your WebRTC application enhances user experience by allowing users to manage their communication effectively. Common controls include muting/unmuting the microphone, starting/stopping the video, and disconnecting from the session.
Adding Control Functionalities
[a] HTML for Controls
Update the HTML to include buttons for controlling the audio and video.
HTML
1 <!-- static/index.html -->
2 <!DOCTYPE html>
3 <html lang="en">
4 <head>
5 <meta charset="UTF-8">
6 <title>AIOQUIC WebRTC App</title>
7 <link rel="stylesheet" href="style.css">
8 </head>
9 <body>
10 <div class="container">
11 <h1>Join the AIOQUIC WebRTC Session</h1>
12 <button id="join">Join</button>
13 <div id="controls" style="display: none;">
14 <button id="mute">Mute</button>
15 <button id="video">Stop Video</button>
16 <button id="leave">Leave</button>
17 </div>
18 </div>
19 <script src="app.js"></script>
20 </body>
21 </html>
[b] CSS for Controls
Style the control buttons for a consistent look.
CSS
1 /* static/style.css */
2 body {
3 font-family: Arial, sans-serif;
4 display: flex;
5 justify-content: center;
6 align-items: center;
7 height: 100vh;
8 background-color: #f0f0f0;
9 margin: 0;
10 }
11
12 .container {
13 text-align: center;
14 }
15
16 button {
17 padding: 10px 20px;
18 font-size: 16px;
19 cursor: pointer;
20 background-color: #4CAF50;
21 color: white;
22 border: none;
23 border-radius: 5px;
24 margin-top: 20px;
25 }
26
27 button:hover {
28 background-color: #45a049;
29 }
30
31 #controls button {
32 margin: 10px;
33 }
[c] JavaScript for Control Functionalities
Implement the JavaScript to manage the audio and video streams.
JavaScript
1 // static/app.js
2 let localStream;
3 let isMuted = false;
4 let isVideoStopped = false;
5
6 document.getElementById('join').addEventListener('click', async () => {
7 const ws = new WebSocket('ws://localhost:8765');
8 ws.onopen = async () => {
9 console.log('WebSocket connection opened');
10 ws.send('Joining the WebRTC session');
11 document.getElementById('controls').style.display = 'block';
12
13 // Get user media
14 localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
15 };
16
17 ws.onmessage = (event) => {
18 console.log(`Received: ${event.data}`);
19 };
20
21 ws.onclose = () => {
22 console.log('WebSocket connection closed');
23 };
24
25 ws.onerror = (error) => {
26 console.error(`WebSocket error: ${error}`);
27 };
28 });
29
30 document.getElementById('mute').addEventListener('click', () => {
31 isMuted = !isMuted;
32 localStream.getAudioTracks()[0].enabled = !isMuted;
33 document.getElementById('mute').innerText = isMuted ? 'Unmute' : 'Mute';
34 });
35
36 document.getElementById('video').addEventListener('click', () => {
37 isVideoStopped = !isVideoStopped;
38 localStream.getVideoTracks()[0].enabled = !isVideoStopped;
39 document.getElementById('video').innerText = isVideoStopped ? 'Start Video' : 'Stop Video';
40 });
41
42 document.getElementById('leave').addEventListener('click', () => {
43 ws.close();
44 document.getElementById('controls').style.display = 'none';
45 localStream.getTracks().forEach(track => track.stop());
46 });
Step-by-Step Instructions
- HTML: Add buttons for muting/unmuting the microphone, stopping/starting the video, and leaving the session.
- CSS: Style the new buttons to match the existing UI.
- JavaScript: Implement event listeners for the control buttons to handle media stream tracks and WebSocket connections.
This implementation equips users with the necessary controls to manage their WebRTC session effectively. In the next step, we will focus on implementing the participant view to handle multiple participants in the session.
Step 5: Implementing Participant View
To create a comprehensive WebRTC application, it's essential to handle multiple participants effectively. This involves managing the display of video and audio streams from all participants in the session.
Displaying Participants in the WebRTC Session
HTML for Participant Views
Update the HTML to include a section for displaying participant video elements.
HTML
1 <!-- static/index.html -->
2 <!DOCTYPE html>
3 <html lang="en">
4 <head>
5 <meta charset="UTF-8">
6 <title>AIOQUIC WebRTC App</title>
7 <link rel="stylesheet" href="style.css">
8 </head>
9 <body>
10 <div class="container">
11 <h1>Join the AIOQUIC WebRTC Session</h1>
12 <button id="join">Join</button>
13 <div id="controls" style="display: none;">
14 <button id="mute">Mute</button>
15 <button id="video">Stop Video</button>
16 <button id="leave">Leave</button>
17 </div>
18 <div id="participants"></div>
19 </div>
20 <script src="app.js"></script>
21 </body>
22 </html>
[b] CSS for Participant Views
Style the participant video elements.
CSS
1 /* static/style.css */
2 body {
3 font-family: Arial, sans-serif;
4 display: flex;
5 justify-content: center;
6 align-items: center;
7 flex-direction: column;
8 height: 100vh;
9 background-color: #f0f0f0;
10 margin: 0;
11 }
12
13 .container {
14 text-align: center;
15 }
16
17 button {
18 padding: 10px 20px;
19 font-size: 16px;
20 cursor: pointer;
21 background-color: #4CAF50;
22 color: white;
23 border: none;
24 border-radius: 5px;
25 margin-top: 20px;
26 }
27
28 button:hover {
29 background-color: #45a049;
30 }
31
32 #controls button {
33 margin: 10px;
34 }
35
36 #participants {
37 display: flex;
38 flex-wrap: wrap;
39 justify-content: center;
40 margin-top: 20px;
41 }
42
43 video {
44 width: 300px;
45 margin: 10px;
46 border: 2px solid #4CAF50;
47 border-radius: 5px;
48 }
[c] JavaScript for Managing Participant Streams
Implement the JavaScript to handle adding and displaying participant streams.
JavaScript
1 // static/app.js
2 let localStream;
3 let isMuted = false;
4 let isVideoStopped = false;
5 const pc = new RTCPeerConnection();
6 const participants = {};
7
8 document.getElementById('join').addEventListener('click', async () => {
9 const ws = new WebSocket('ws://localhost:8765');
10 ws.onopen = async () => {
11 console.log('WebSocket connection opened');
12 ws.send('Joining the WebRTC session');
13 document.getElementById('controls').style.display = 'block';
14
15 // Get user media
16 localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
17 localStream.getTracks().forEach(track => pc.addTrack(track, localStream));
18
19 // Display local video
20 addVideoStream('local', localStream);
21
22 pc.ontrack = (event) => {
23 const [remoteStream] = event.streams;
24 const remoteId = event.track.id;
25 if (!participants[remoteId]) {
26 addVideoStream(remoteId, remoteStream);
27 participants[remoteId] = remoteStream;
28 }
29 };
30 };
31
32 ws.onmessage = async (event) => {
33 const message = JSON.parse(event.data);
34 if (message.offer) {
35 await pc.setRemoteDescription(new RTCSessionDescription(message.offer));
36 const answer = await pc.createAnswer();
37 await pc.setLocalDescription(answer);
38 ws.send(JSON.stringify({ answer: pc.localDescription }));
39 } else if (message.answer) {
40 await pc.setRemoteDescription(new RTCSessionDescription(message.answer));
41 } else if (message.candidate) {
42 await pc.addIceCandidate(new RTCIceCandidate(message.candidate));
43 }
44 };
45
46 ws.onclose = () => {
47 console.log('WebSocket connection closed');
48 };
49
50 ws.onerror = (error) => {
51 console.error(`WebSocket error: ${error}`);
52 };
53
54 pc.onicecandidate = ({ candidate }) => {
55 if (candidate) {
56 ws.send(JSON.stringify({ candidate }));
57 }
58 };
59 });
60
61 document.getElementById('mute').addEventListener('click', () => {
62 isMuted = !isMuted;
63 localStream.getAudioTracks()[0].enabled = !isMuted;
64 document.getElementById('mute').innerText = isMuted ? 'Unmute' : 'Mute';
65 });
66
67 document.getElementById('video').addEventListener('click', () => {
68 isVideoStopped = !isVideoStopped;
69 localStream.getVideoTracks()[0].enabled = !isVideoStopped;
70 document.getElementById('video').innerText = isVideoStopped ? 'Start Video' : 'Stop Video';
71 });
72
73 document.getElementById('leave').addEventListener('click', () => {
74 ws.close();
75 document.getElementById('controls').style.display = 'none';
76 localStream.getTracks().forEach(track => track.stop());
77 Object.keys(participants).forEach(id => {
78 document.getElementById(id).remove();
79 delete participants[id];
80 });
81 });
82
83 function addVideoStream(id, stream) {
84 const video = document.createElement('video');
85 video.id = id;
86 video.srcObject = stream;
87 video.autoplay = true;
88 document.getElementById('participants').appendChild(video);
89 }
Step-by-Step Instructions
- HTML: Add a
div
element for displaying participant videos and update the control buttons. - CSS: Style the participant video elements for a neat display.
- JavaScript: Implement event listeners to handle WebRTC peer connections and dynamically add video elements for each participant stream.
This implementation ensures that your application can manage and display multiple participant streams, enhancing the real-time communication experience. In the next step, we will finalize the application by running and testing the complete setup.
Step 6: Running Your Code
With all components in place, it's time to run and test your AIOQUIC WebRTC application. This step involves starting the signaling server, launching the WebRTC application, and verifying that all functionalities work as expected.
Final Steps to Run the Application
[a] Start the Signaling Server
Ensure your signaling server is running. Open a terminal and navigate to your project directory. Then, run the signaling server script.
bash
1 python app/signaling.py
[b] Launch the WebRTC Application
Open another terminal, navigate to the project directory, and start the main application script.
bash
1 python main.py
[c] Open the Web Application
Open your web browser and navigate to
http://localhost:8000
to load the WebRTC application. You should see the join screen and control buttons.Debugging Tips and Common Issues
- WebSocket Connection Issues: Ensure that the WebSocket server URL in your JavaScript matches the server address and port.
- Media Device Permissions: Make sure your browser has permissions to access the camera and microphone.
- Console Errors: Check the browser console for any JavaScript errors and resolve them as needed.
- Network Configuration: Verify that your network configuration allows WebRTC and WebSocket traffic.
Sample Output
When you run the application, you should see a join screen with a button to join the WebRTC session. Upon clicking join, the control buttons for muting, stopping the video, and leaving the session should appear. You will also see your video stream and any additional participant streams displayed on the page.
Conclusion
Integrating AIOQUIC with WebRTC offers a powerful and efficient solution for real-time communication applications. By leveraging the QUIC protocol and the flexibility of Python, developers can create high-performance, low-latency communication systems. This guide has walked you through the setup, implementation, and management of a WebRTC application using AIOQUIC, ensuring a smooth development process.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ