WebSocket MQTT: Bridging Web Technologies and IoT Communication
In the rapidly evolving landscape of real-time communication and IoT, integrating web technologies with messaging protocols like MQTT is becoming increasingly crucial. This article explores the synergy of WebSockets and MQTT, delving into their individual strengths, how they work together, implementation details, security considerations, and best practices for leveraging them effectively.
WebSocket vs MQTT: A Comprehensive Comparison
Introduction to MQTT and WebSockets
MQTT (Message Queuing Telemetry Transport) is a lightweight, publish-subscribe network protocol that transports messages between devices. It's ideal for IoT applications due to its low bandwidth requirements and efficient message delivery. WebSockets, on the other hand, provide full-duplex communication channels over a single TCP connection, enabling real-time data transfer between a client and a server.
Key Differences: Protocol Design, Use Cases, and Performance
MQTT is designed for machine-to-machine (M2M) communication, particularly in constrained environments. It offers features like quality of service (QoS) levels to ensure reliable message delivery. WebSockets are primarily designed for real-time web applications, providing a persistent connection for bidirectional data flow. In terms of performance, MQTT excels in scenarios with limited bandwidth, while WebSockets are optimized for low-latency, real-time interactions.
The core differences lie in their design philosophies. MQTT prioritizes efficient message delivery and resource usage, while WebSockets focus on enabling real-time communication in web browsers. MQTT uses a publish-subscribe model, which decouples message senders (publishers) from receivers (subscribers), while WebSockets facilitate direct two-way communication between a client and a server. This difference impacts use cases. MQTT excels in IoT, where devices communicate sensor data and control signals. WebSockets shine in chat applications, online gaming, and live data dashboards.
When to Use MQTT Over WebSockets and Vice Versa
Use MQTT when dealing with resource-constrained devices or unreliable networks. WebSockets are preferable when real-time bidirectional communication is required in a web browser environment. Using MQTT over WebSockets allows you to leverage the strengths of both protocols, enabling MQTT communication from web browsers.
MQTT Over WebSockets: A Deep Dive
What is MQTT Over WebSockets?
MQTT over WebSockets enables MQTT communication through a WebSocket connection. This allows web browsers and other WebSocket-enabled clients to interact with MQTT brokers, providing a seamless bridge between web technologies and the MQTT protocol.
How MQTT and WebSockets Work Together
When using MQTT over WebSockets, the MQTT messages are encapsulated within WebSocket frames. The client initiates a WebSocket connection to the MQTT broker, which is configured to listen for WebSocket connections. Once the connection is established, the client can send and receive MQTT messages as payloads within the WebSocket frames. This enables MQTT functionality from within a web browser, where direct TCP connections are typically restricted.
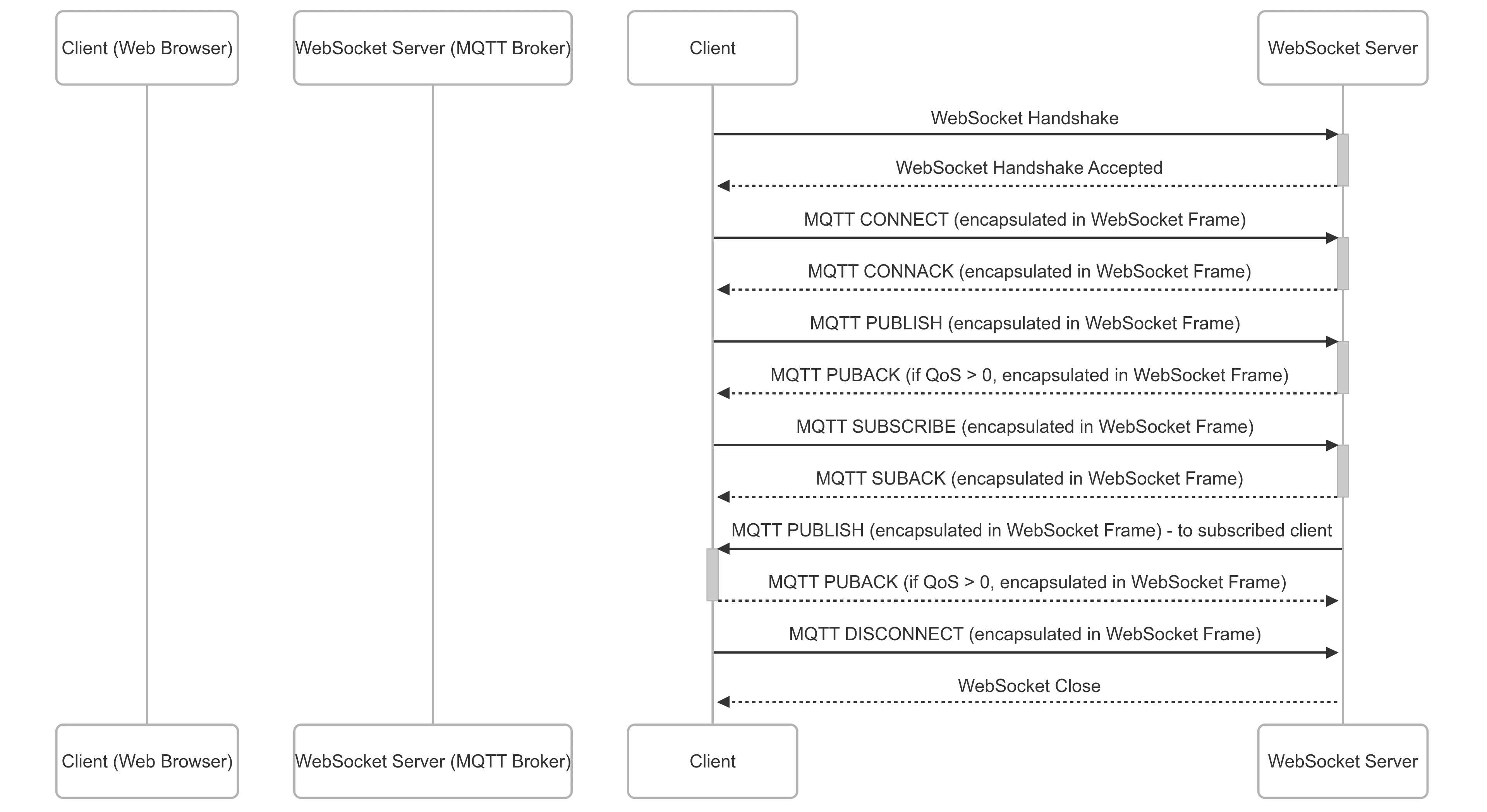
Here's a simplified illustration of the MQTT over WebSockets handshake:
1Client (Web Browser) -> WebSocket Server (MQTT Broker): WebSocket Handshake
2WebSocket Server -> Client (Web Browser): WebSocket Handshake Accepted
3Client (Web Browser) -> WebSocket Server (MQTT Broker): MQTT CONNECT (encapsulated in WebSocket Frame)
4WebSocket Server -> Client (Web Browser): MQTT CONNACK (encapsulated in WebSocket Frame)
5...
6
Benefits of Using MQTT Over WebSockets
- Web Browser Integration: Enables MQTT communication from web browsers without requiring browser plugins or extensions.
- Firewall Compatibility: WebSockets typically use port 80 or 443, which are commonly open in firewalls, facilitating easier communication.
- Simplified Architecture: Reduces the need for separate MQTT clients and web servers, simplifying the overall system architecture.
- Real-time Data Streaming: Facilitates real-time data streaming from IoT devices to web-based dashboards and applications.
Choosing the Right MQTT Broker for WebSockets
Several MQTT brokers support WebSockets, including:
- Mosquitto: A popular open-source MQTT broker that supports WebSockets.
- EMQX: A scalable and reliable MQTT broker designed for enterprise use, also supporting WebSockets.
- HiveMQ: A commercial MQTT broker with extensive features, including WebSocket support.
When choosing an MQTT broker, consider factors such as scalability, reliability, security features, and ease of configuration for WebSockets.
Implementing MQTT Over WebSockets
Setting up Your MQTT Broker
First, you need to configure your MQTT broker to listen for WebSocket connections. For example, with Mosquitto, you can modify the
mosquitto.conf
file to include the following:1listener 1883
2
3listener 9001
4protocol websockets
5
6
7#persistence true
8#persistence_location /var/lib/mosquitto/
9
10#log_dest file /var/log/mosquitto/mosquitto.log
11
12#include_dir /etc/mosquitto/conf.d
13
This configuration sets up Mosquitto to listen on port 1883 for standard MQTT connections and port 9001 for WebSocket connections.
Client-Side Implementation: JavaScript Example
The Paho MQTT JavaScript client library is a popular choice for implementing MQTT over WebSockets in web browsers. Here's a basic example:
1// Create a client instance
2var client = new Paho.MQTT.Client("broker.example.com", 9001, "clientId");
3
4// Set callback handlers
5client.onConnectionLost = onConnectionLost;
6client.onMessageArrived = onMessageArrived;
7
8// Connect the client
9client.connect({onSuccess:onConnect});
10
11// Called when the client connects
12function onConnect() {
13 console.log("onConnect");
14 client.subscribe("topic/test");
15}
16
17// Called when the client loses its connection
18function onConnectionLost(responseObject) {
19 if (responseObject.errorCode !== 0) {
20 console.log("onConnectionLost:" + responseObject.errorMessage);
21 }
22}
23
24// Called when a message arrives
25function onMessageArrived(message) {
26 console.log("onMessageArrived:" + message.payloadString);
27}
28
29// Send a message
30message = new Paho.MQTT.Message("Hello");
31message.destinationName = "topic/test";
32client.send(message);
33
This code snippet demonstrates how to connect to an MQTT broker over WebSockets, subscribe to a topic, and publish a message.
Client-Side Implementation: Python Example
For Python-based applications, the
paho-mqtt
library can be used to connect to an MQTT broker over WebSockets. Here's an example:1import paho.mqtt.client as mqtt
2
3def on_connect(client, userdata, flags, rc):
4 print("Connected with result code " + str(rc))
5 client.subscribe("topic/test")
6
7def on_message(client, userdata, msg):
8 print(msg.topic + " " + str(msg.payload.decode()))
9
10client = mqtt.Client(transport="websockets")
11client.on_connect = on_connect
12client.on_message = on_message
13
14client.ws_set_options(path="/mqtt")
15client.connect("broker.example.com", 9001, 60)
16
17client.loop_forever()
18
This example shows how to establish a WebSocket connection to an MQTT broker using Python and the
paho-mqtt
library.Handling Connection Issues and Reconnection
Robust applications should handle connection losses and implement reconnection mechanisms. The Paho MQTT client libraries provide callbacks for connection lost events, allowing you to trigger reconnection attempts.
Security Considerations for MQTT Over WebSockets
Transport Layer Security (TLS/SSL)
Always use TLS/SSL to encrypt the WebSocket connection and protect the MQTT messages from eavesdropping. Configure your MQTT broker to require TLS/SSL for WebSocket connections.
Authentication and Authorization
Implement robust authentication mechanisms to verify the identity of clients connecting to the MQTT broker. Use authorization to control which topics clients can subscribe to and publish to.
Data Encryption and Integrity
While TLS/SSL encrypts the transport layer, consider encrypting the MQTT message payloads for end-to-end security. Use message digests or digital signatures to ensure message integrity.
Advanced Topics and Best Practices
Scalability and Performance Optimization
- Load Balancing: Distribute WebSocket connections across multiple MQTT broker instances using a load balancer.
- Connection Pooling: Use connection pooling to reduce the overhead of establishing new WebSocket connections.
- Message Batching: Batch multiple MQTT messages into a single WebSocket frame to reduce network overhead.
- Optimize Message Size: Keep message payloads as small as possible to minimize bandwidth usage.
Monitoring and Logging
Implement comprehensive monitoring and logging to track connection status, message throughput, and error rates. Use monitoring tools to identify and resolve performance bottlenecks.
Integrating with Existing Systems
Integrate MQTT over WebSockets with existing web applications and IoT platforms using APIs and libraries. Use message queues and data processing pipelines to handle large volumes of data.
Conclusion
MQTT over WebSockets offers a powerful solution for bridging web technologies and IoT communication. By understanding the strengths of both protocols and implementing appropriate security measures, developers can create robust and scalable real-time applications. This fusion of technologies unlocks new possibilities for data visualization, remote control, and seamless integration between the web and the world of connected devices.
Further Reading:
MQTT Specification
: "Learn more about the MQTT protocol."WebSockets RFC
: "Understand the WebSockets protocol in detail."Paho MQTT Client Library
: "Explore a popular MQTT client library."
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ