Introduction: Navigating the WebRTC Landscape for iOS Development
WebRTC (Web Real-Time Communication) has revolutionized real-time communication, enabling seamless video, audio, and data sharing across various platforms. For iOS developers, integrating WebRTC functionality into their applications can open up a world of possibilities, from video conferencing to live streaming and beyond. However, choosing the right WebRTC SDK for iOS can be a daunting task. This guide will provide a comprehensive overview of the available options, key considerations, and best practices for successful WebRTC iOS integration.
What is WebRTC?
WebRTC is a free, open-source project that provides web browsers and mobile applications with real-time communication (RTC) via simple APIs. It allows direct peer-to-peer communication without the need for intermediaries, enabling low-latency and high-quality audio and video experiences.
Why Choose WebRTC for iOS?
Choosing WebRTC for iOS offers several advantages:
- Real-time communication: Enables instant audio, video, and data sharing.
- Cross-platform compatibility: Works seamlessly with other WebRTC-enabled platforms.
- Cost-effective: Open-source nature reduces licensing costs.
- Customization: Offers flexibility to tailor the solution to specific application requirements.
The Challenge of Selecting an iOS WebRTC SDK
The market offers a variety of iOS WebRTC frameworks, each with its own set of features, pricing models, and levels of support. Evaluating these options and selecting the one that best aligns with your project's needs can be challenging. This guide aims to simplify this process by providing a detailed comparative analysis of popular WebRTC iOS SDK options and offering practical guidance on integration and optimization.
Understanding WebRTC SDKs for iOS: Key Features and Considerations
Before diving into specific SDKs, it's crucial to understand the core functionalities and essential features to look for in a WebRTC SDK for iOS. These considerations will help you make an informed decision and choose the SDK that best fits your project's requirements.
Core Functionality: Real-time Communication Capabilities
The primary function of a WebRTC SDK is to enable real-time communication. This includes establishing peer-to-peer connections, managing audio and video streams, and handling data transfer. The SDK should provide a robust and reliable framework for these core functionalities.
Essential Features: Video, Audio, and Data Channels
A comprehensive WebRTC SDK for iOS should support the following:
- Video: High-quality video streaming with support for various codecs.
- Audio: Clear and reliable audio transmission with noise cancellation and echo suppression.
- Data Channels: Reliable and ordered data transfer for application-specific data.
Consider your specific needs regarding audio and video codecs. Common codecs include VP8, VP9, H.264, and Opus. The SDK should support the codecs that best suit your application's requirements and target devices.
Platform Compatibility and Integration
Ensure that the iOS WebRTC framework is compatible with your target iOS versions and devices. The SDK should also integrate seamlessly with your existing project and development environment. Consider the availability of documentation, sample code, and community support.
Scalability and Performance
Evaluate the SDK's scalability and performance characteristics. Can it handle a large number of concurrent users? Does it offer features for bandwidth management and optimization? Consider the SDK's impact on device battery life and CPU usage.
Top WebRTC SDKs for iOS: A Comparative Analysis
This section provides a comparative analysis of popular WebRTC SDKs for iOS, highlighting their key features, strengths, and weaknesses.
Option 1: [SDK Name 1]
[SDK Name 1] offers a comprehensive set of features for building real-time communication applications on iOS. It provides robust support for video, audio, and data channels, as well as advanced features such as screen sharing and recording. Key features include:
- High-quality video and audio codecs
- Support for various signaling protocols
- Advanced bandwidth management
- Easy-to-use API
Swift
1// Basic video call initiation with SDK 1
2import SDKName1
3
4let peerConnectionFactory = RTCPeerConnectionFactory()
5let peerConnection = peerConnectionFactory.peerConnection(with: configuration, constraints: constraints, delegate: self)
6
7let videoTrack = peerConnectionFactory.videoSource(for: capturer, constraints: constraints).track
8peerConnection.add(videoTrack, streamId: "stream1")
9
10peerConnection.offer(for: constraints) { (sdp, error) in
11 // Handle SDP offer
12}
13
Option 2: [SDK Name 2]
[SDK Name 2] is another popular iOS WebRTC framework that provides a flexible and customizable platform for building real-time communication applications. It offers a wide range of features, including:
- Support for multiple video and audio codecs
- Advanced signaling capabilities
- Built-in support for STUN/TURN servers
- Customizable UI components
Objective-C
1// Handling audio tracks with SDK 2
2#import <SDKName2/SDKName2.h>
3
4SDKAudioTrack *audioTrack = [SDKAudioTrack trackWithID:@"audio1" source:audioSource];
5[peerConnection addTrack:audioTrack toStream:stream];
6
7[audioTrack setEnabled:YES];
8
Option 3: [SDK Name 3]
[SDK Name 3] focuses on providing a simple and easy-to-use API for WebRTC iOS implementation. It is a good choice for developers who are new to WebRTC or who need to quickly integrate real-time communication functionality into their applications. Key features include:
- Simplified API for common WebRTC tasks
- Built-in support for signaling
- Automatic bandwidth management
- Excellent documentation and support
JavaScript
1// Implementing screen sharing with SDK 3
2const configuration = { iceServers: [{ urls: 'stun:stun.l.google.com:19302' }] };
3const pc = new RTCPeerConnection(configuration);
4
5navigator.mediaDevices.getDisplayMedia({ video: true, audio: false })
6 .then(stream => {
7 stream.getTracks().forEach(track => pc.addTrack(track, stream));
8 })
9 .catch(err => console.error('Error accessing screen share:', err));
10
Option 4: Open Source Options
For developers who prefer to work with open-source libraries, the official Google WebRTC iOS library is a viable option. While it requires more manual configuration and setup, it offers maximum flexibility and control over the underlying WebRTC iOS architecture. Be aware that ongoing maintenance and staying up-to-date with the latest WebRTC specifications will fall on your team if you choose this route.
Integrating a WebRTC SDK into Your iOS Project: A Step-by-Step Guide
This section provides a step-by-step guide on integrating a WebRTC SDK into your iOS project.
Setting Up Your Development Environment
Before you begin, ensure that you have the following:
- Xcode installed on your macOS system
- A valid Apple Developer account
- Basic knowledge of Swift or Objective-C
Choosing the Right SDK and Installation Method
Select the WebRTC SDK for iOS that best aligns with your project's needs. Most SDKs offer installation via CocoaPods, Swift Package Manager, or manual integration. Choose the method that is most convenient for you.
Project Configuration and Dependencies
Add the necessary dependencies to your project using the chosen installation method. For example, if using CocoaPods, add the SDK to your
Podfile
and run pod install
.Implementing Core WebRTC Functionality
Initialize the SDK and implement the core WebRTC functionalities, such as establishing peer connections, managing audio and video streams, and handling signaling.
Swift
1// Setting up a peer connection
2import WebRTC
3
4let factory = RTCPeerConnectionFactory()
5let config = RTCConfiguration()
6config.iceServers = [RTCIceServer(urlStrings: ["stun:stun.l.google.com:19302"])]
7
8let peerConnection = factory.peerConnection(with: config, constraints: RTCMediaConstraints(mandatoryConstraints: nil, optionalConstraints: nil), delegate: self)
9
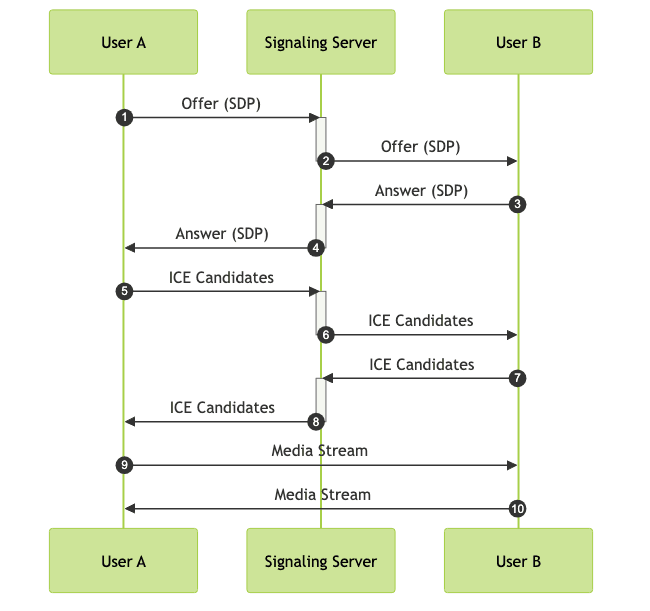
Advanced WebRTC Features and Optimizations for iOS
Once you have the basic WebRTC functionality in place, you can explore advanced features and optimizations to enhance the user experience and improve performance.
Signaling and Session Management
Signaling is the process of exchanging metadata between peers to establish a connection. Choose a signaling protocol that is compatible with your SDK and application requirements. Common signaling protocols include SIP, XMPP, and custom WebSocket-based protocols. Proper signaling is critical for successful WebRTC iOS streaming.
STUN/TURN Server Configuration
STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers are used to help peers discover their public IP addresses and relay traffic when direct peer-to-peer connections are not possible. Configure your SDK to use STUN/TURN servers to ensure reliable connectivity in various network environments.
Bandwidth Management and Optimization
Implement bandwidth management techniques to optimize the quality of the audio and video streams based on the available network bandwidth. This may involve adjusting the video resolution, frame rate, and audio bitrate. Consider using adaptive bitrate streaming (ABR) techniques.
Handling Errors and Debugging
Implement robust error handling mechanisms to gracefully handle unexpected errors and provide informative feedback to the user. Use debugging tools to identify and resolve issues during development.
Best Practices for Secure and Reliable WebRTC iOS Applications
Security and reliability are paramount when building WebRTC iOS applications.
Data Encryption and Security
Ensure that all data transmitted over WebRTC connections is encrypted using DTLS (Datagram Transport Layer Security) and SRTP (Secure Real-time Transport Protocol). Implement appropriate authentication and authorization mechanisms to protect against unauthorized access.
Error Handling and Robustness
Implement comprehensive error handling to prevent crashes and ensure a stable user experience. Handle network connectivity issues, signaling failures, and media stream errors gracefully.
Performance Monitoring and Optimization
Monitor the performance of your WebRTC iOS app to identify and address potential bottlenecks. Track metrics such as CPU usage, memory consumption, and network latency. Optimize your code and configurations to improve performance and reduce resource consumption. Monitoring WebRTC iOS performance is crucial for delivering a quality experience.
Conclusion: Choosing the Right Path for Your WebRTC iOS Project
Choosing the right WebRTC SDK for iOS is a critical decision that can significantly impact the success of your real-time communication application. By carefully considering the factors outlined in this guide, you can make an informed choice and build a robust, secure, and scalable application that meets your specific needs. Remember to prioritize security, performance, and user experience throughout the development process. Understanding the intricacies of WebRTC iOS architecture is key to a successful WebRTC iOS implementation.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ