Introduction to SipSorcery WebRTC
Web Real-Time Communication (WebRTC) is a groundbreaking technology that enables real-time audio, video, and data sharing between browsers and mobile applications without the need for internal or external plugins. It's designed to provide peer-to-peer communication, making it ideal for applications such as video conferencing, file transfer, and live streaming. However, integrating WebRTC into a project can be complex, requiring a solid understanding of both the technology and its implementation.
What is SipSorcery WebRTC?
SipSorcery is an open-source project that simplifies the process of implementing WebRTC in C# environments. Originally developed for VoIP (Voice over IP) applications, SipSorcery has evolved to support WebRTC, providing a powerful framework for developers working with C#, desktop applications, servers, and even Unity/Xbox platforms.
The primary goal of SipSorcery is to offer a comprehensive toolkit that bridges the gap between the complexities of WebRTC and the robust capabilities of C#. It provides pre-built components, essential functions, and detailed documentation that can significantly reduce the development time and effort required to build WebRTC-enabled applications.
By leveraging SipSorcery, developers can focus more on the functionality and user experience of their applications rather than the intricacies of WebRTC. This makes it an invaluable tool for anyone looking to implement real-time communication features in their projects, whether for personal use, enterprise solutions, or gaming applications on platforms like Unity and Xbox.
In this article, we will guide you through the process of setting up and using SipSorcery for WebRTC applications. From installation and project structure to implementing key features and running your application, we will cover everything you need to get started with SipSorcery WebRTC in your C# projects.
Getting Started with the Code
Getting started with SipSorcery WebRTC involves setting up your development environment, installing the necessary tools, and understanding the project structure and architecture. Follow these steps to create a new SipSorcery WebRTC application and get up and running quickly.
Creating a New SipSorcery WebRTC App
[a] Installation
Before diving into the code, you need to set up your development environment. Here’s what you need:
- Visual Studio: Download and install Visual Studio, a powerful IDE for C# development. Ensure you have the latest version.
- .NET SDK: Download and install the .NET SDK from the official Microsoft website. This is essential for building and running C# applications.
- Git: Install Git to clone the SipSorcery repository. You can download it from the official Git website.
[b] Cloning the Repository
Once you have the necessary tools installed, clone the SipSorcery repository from GitHub:
bash
1git clone https://github.com/sipsorcery-org/sipsorcery.git
2
Navigate to the cloned directory:
bash
1cd sipsorcery
2
[c] Setting Up the Development Environment
Open the cloned repository in Visual Studio. Follow these steps:
- Open Visual Studio.
- Select “Open a project or solution”.
- Navigate to the
sipsorcery
directory and open thesipsorcery.sln
solution file.
Project Structure Overview
Understanding the structure of your project is crucial for efficient development. The SipSorcery repository contains several key directories and files:
- src: Contains the source code for the SipSorcery library.
- examples: Includes example projects demonstrating how to use SipSorcery.
- tests: Contains unit tests for the SipSorcery library.
- docs: Documentation and guides for using SipSorcery.
Familiarize yourself with these directories as they will be essential for development and troubleshooting.
App Architecture
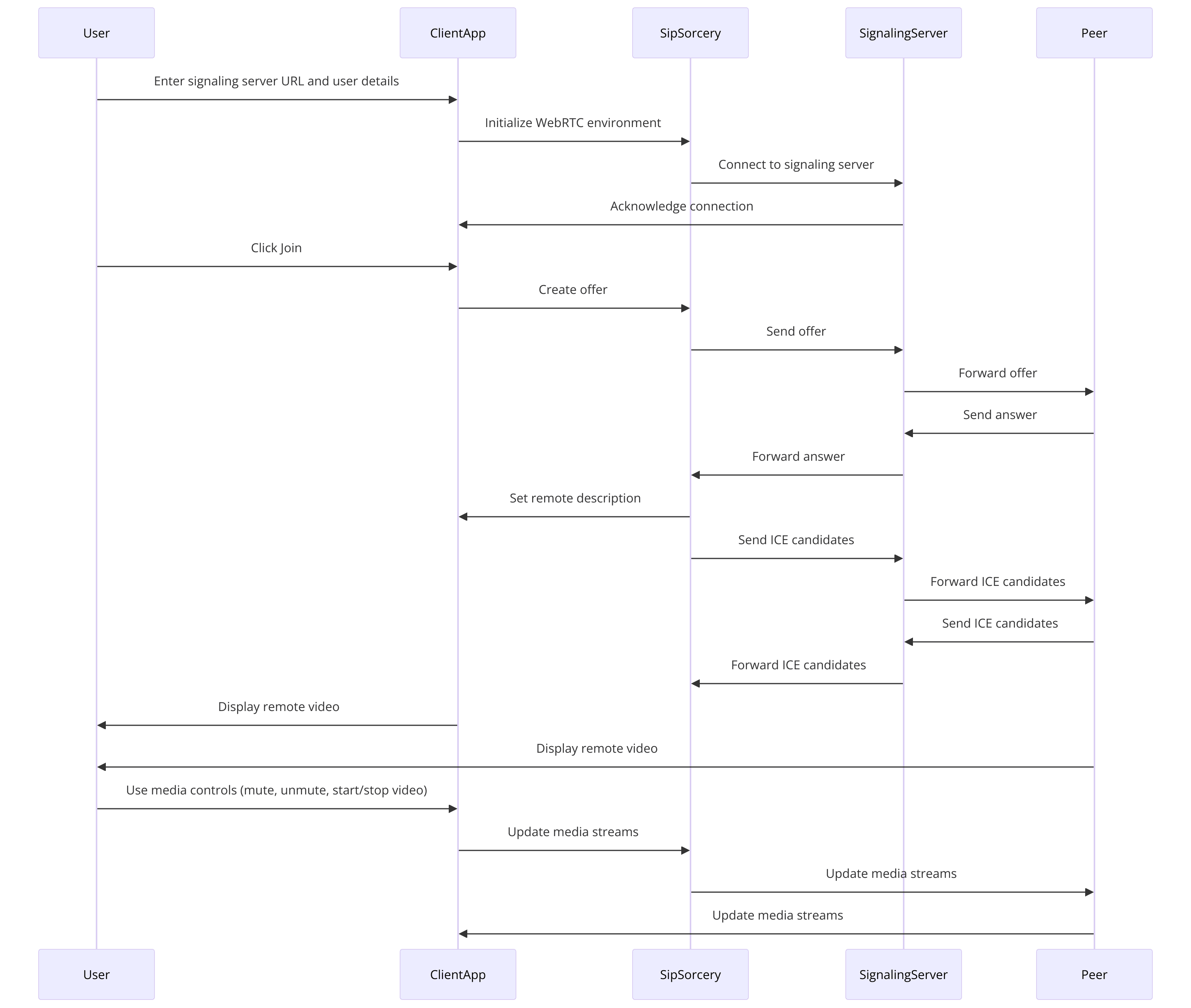
- Signaling: Establishes and manages the connection between peers. SipSorcery handles the signaling process, making it easier to connect WebRTC peers.
- Media Streams: Manages the audio and video streams. SipSorcery provides robust methods to handle media streams, ensuring smooth and efficient communication.
- ICE (Interactive Connectivity Establishment): Handles the process of finding the best path to connect peers. SipSorcery simplifies ICE management, making it more accessible for developers.
By understanding these components and how they interact, you can effectively build and manage your WebRTC applications using SipSorcery.
Example Project
To help you get started, SipSorcery provides several example projects. Open the
examples
directory and explore the various sample applications. These examples cover different aspects of WebRTC implementation, from basic signaling to complex media stream handling.Building the Project
Once you have set up your environment and explored the project structure, it’s time to build the project:
- In Visual Studio, right-click on the solution in the Solution Explorer.
- Select “Build Solution” to compile the project.
Ensure there are no errors and that the build completes successfully. You are now ready to start developing your SipSorcery WebRTC application.
By following these steps, you have set up your development environment, installed the necessary tools, and understood the project structure and architecture. You are now ready to dive into the code and start building your SipSorcery WebRTC application. In the next sections, we will guide you through implementing key features, from initializing the WebRTC environment to managing media streams and controls.
Step 1: Get Started with Main File
Now that you have set up your development environment and understood the project structure, it's time to start coding. In this section, we will walk you through the process of initializing the WebRTC environment in the main file of your SipSorcery WebRTC application.
Detailed Explanation of the Main File
The main file of your SipSorcery WebRTC application is where the core initialization and setup take place. Typically, this file is named
Program.cs
in C# projects. It serves as the entry point for your application and contains the necessary code to set up and run the WebRTC functionality.Step-by-Step Guide
[a] Open Program.cs
Open the
Program.cs
file in Visual Studio. This file should be located in the root directory of your project.[b] Add Necessary Namespaces
At the beginning of the
Program.cs
file, add the required namespaces. These namespaces include the SipSorcery libraries and other necessary C# libraries for WebRTC functionality.csharp
1 using System;
2 using SIPSorcery.Net;
3 using SIPSorceryMedia;
4 using WebSocketSharp;
5
[c] Initialize WebRTC Configuration
In the
Main
method, initialize the WebRTC configuration. This involves setting up the signaling server, creating an RTC peer connection, and configuring media streams.csharp
1 static void Main(string[] args)
2 {
3 Console.WriteLine("Starting SipSorcery WebRTC Application...");
4
5 // Initialize WebRTC Configuration
6 RTCConfiguration config = new RTCConfiguration
7 {
8 iceServers = new List<RTCIceServer>
9 {
10 new RTCIceServer { urls = "stun:stun.l.google.com:19302" }
11 }
12 };
13
14 // Create a new peer connection
15 RTCPeerConnection peerConnection = new RTCPeerConnection(config);
16
17 // Set up event handlers
18 peerConnection.OnIceCandidate += (candidate) =>
19 {
20 Console.WriteLine($"ICE Candidate: {candidate}");
21 };
22
23 peerConnection.OnTrack += (track) =>
24 {
25 Console.WriteLine($"New track received: {track.Kind}");
26 };
27
28 // Add media tracks
29 MediaStream mediaStream = new MediaStream();
30 var audioTrack = new MediaStreamTrack(MediaStreamTrackKind.Audio);
31 mediaStream.AddTrack(audioTrack);
32 peerConnection.AddStream(mediaStream);
33
34 Console.WriteLine("WebRTC Configuration Initialized.");
35 }
36
[d] Set Up Signaling
WebRTC requires a signaling mechanism to exchange connection information between peers. SipSorcery can use WebSocket for signaling. Here’s how to set it up:
csharp
1 // Establish WebSocket connection for signaling
2 using (WebSocket ws = new WebSocket("wss://your_signaling_server"))
3 {
4 ws.OnMessage += (sender, e) =>
5 {
6 // Handle signaling messages
7 var message = e.Data;
8 Console.WriteLine($"Signaling Message: {message}");
9
10 if (message.Contains("offer"))
11 {
12 var offer = new RTCSessionDescriptionInit
13 {
14 type = RTCSdpType.offer,
15 sdp = message
16 };
17 peerConnection.SetRemoteDescription(offer);
18 var answer = peerConnection.CreateAnswer(null);
19 peerConnection.SetLocalDescription(answer);
20 ws.Send(answer.sdp);
21 }
22 else if (message.Contains("answer"))
23 {
24 var answer = new RTCSessionDescriptionInit
25 {
26 type = RTCSdpType.answer,
27 sdp = message
28 };
29 peerConnection.SetRemoteDescription(answer);
30 }
31 else if (message.Contains("candidate"))
32 {
33 var candidate = new RTCIceCandidateInit
34 {
35 candidate = message
36 };
37 peerConnection.AddIceCandidate(candidate);
38 }
39 };
40
41 ws.Connect();
42 Console.WriteLine("WebSocket Signaling Connected.");
43 }
44
[e] Running the Application
To run the application, press
F5
in Visual Studio or use the dotnet run
command in the terminal:bash
1 dotnet run
2
This will compile and execute your application, initializing the WebRTC environment and setting up the signaling mechanism.
By following these steps, you have successfully initialized the WebRTC environment in your SipSorcery WebRTC application. You now have a basic setup that can handle signaling, establish peer connections, and manage media streams. In the next sections, we will dive deeper into implementing additional features, such as creating the UI, managing controls, and handling participant views.
Step 2: Wireframe All the Components
After setting up the main file and initializing the WebRTC environment, the next step is to design the user interface (UI) for your SipSorcery WebRTC application. This involves creating a wireframe for all the components and connecting them to the backend logic. In this section, we'll guide you through designing a basic UI and integrating it with the WebRTC functionality.
Creating the UI Wireframe
To design the UI, you can use tools and libraries that support C# and desktop applications. For simplicity, we'll use Windows Forms, a UI framework for building Windows desktop applications in C#.
[a] Create a New Windows Forms Project
If you haven't already created a Windows Forms project, you can do so by following these steps:
- Open Visual Studio.
- Select “Create a new project”.
- Choose “Windows Forms App (.NET)” and click “Next”.
- Name your project and click “Create”.
[b] Design the Main Form
Open the
Form1.cs
file. This is the main form of your application where you will design the UI. Use the Designer view to drag and drop UI components onto the form.UI Components:
- TextBox: For inputting signaling server URL.
- Button: For connecting to the signaling server.
- ListBox: To display logs and connection status.
- Panel: For rendering video streams (local and remote).
Example Layout
csharp
1 // Form1.Designer.cs
2 private System.Windows.Forms.TextBox txtSignalingServer;
3 private System.Windows.Forms.Button btnConnect;
4 private System.Windows.Forms.ListBox lstLog;
5 private System.Windows.Forms.Panel pnlLocalVideo;
6 private System.Windows.Forms.Panel pnlRemoteVideo;
7
8 private void InitializeComponent()
9 {
10 this.txtSignalingServer = new System.Windows.Forms.TextBox();
11 this.btnConnect = new System.Windows.Forms.Button();
12 this.lstLog = new System.Windows.Forms.ListBox();
13 this.pnlLocalVideo = new System.Windows.Forms.Panel();
14 this.pnlRemoteVideo = new System.Windows.Forms.Panel();
15
16 // txtSignalingServer
17 this.txtSignalingServer.Location = new System.Drawing.Point(12, 12);
18 this.txtSignalingServer.Name = "txtSignalingServer";
19 this.txtSignalingServer.Size = new System.Drawing.Size(400, 20);
20 this.txtSignalingServer.TabIndex = 0;
21
22 // btnConnect
23 this.btnConnect.Location = new System.Drawing.Point(420, 10);
24 this.btnConnect.Name = "btnConnect";
25 this.btnConnect.Size = new System.Drawing.Size(75, 23);
26 this.btnConnect.TabIndex = 1;
27 this.btnConnect.Text = "Connect";
28 this.btnConnect.UseVisualStyleBackColor = true;
29 this.btnConnect.Click += new System.EventHandler(this.btnConnect_Click);
30
31 // lstLog
32 this.lstLog.FormattingEnabled = true;
33 this.lstLog.Location = new System.Drawing.Point(12, 50);
34 this.lstLog.Name = "lstLog";
35 this.lstLog.Size = new System.Drawing.Size(483, 95);
36 this.lstLog.TabIndex = 2;
37
38 // pnlLocalVideo
39 this.pnlLocalVideo.Location = new System.Drawing.Point(12, 160);
40 this.pnlLocalVideo.Name = "pnlLocalVideo";
41 this.pnlLocalVideo.Size = new System.Drawing.Size(240, 180);
42 this.pnlLocalVideo.TabIndex = 3;
43
44 // pnlRemoteVideo
45 this.pnlRemoteVideo.Location = new System.Drawing.Point(255, 160);
46 this.pnlRemoteVideo.Name = "pnlRemoteVideo";
47 this.pnlRemoteVideo.Size = new System.Drawing.Size(240, 180);
48 this.pnlRemoteVideo.TabIndex = 4;
49
50 // Form1
51 this.ClientSize = new System.Drawing.Size(507, 352);
52 this.Controls.Add(this.txtSignalingServer);
53 this.Controls.Add(this.btnConnect);
54 this.Controls.Add(this.lstLog);
55 this.Controls.Add(this.pnlLocalVideo);
56 this.Controls.Add(this.pnlRemoteVideo);
57 this.Name = "Form1";
58 this.Text = "SipSorcery WebRTC";
59 }
60
[c] Connecting UI Components with Backend Logic
Next, you need to connect the UI components to the backend logic that you set up in
Program.cs
. Specifically, you will handle the connect button click event to initiate the WebRTC setup.Code Snippets:
csharp
1 // Form1.cs
2 using System;
3 using System.Windows.Forms;
4 using SIPSorcery.Net;
5 using SIPSorceryMedia;
6 using WebSocketSharp;
7
8 public partial class Form1 : Form
9 {
10 private RTCPeerConnection _peerConnection;
11 private WebSocket _webSocket;
12
13 public Form1()
14 {
15 InitializeComponent();
16 }
17
18 private void btnConnect_Click(object sender, EventArgs e)
19 {
20 string signalingServerUrl = txtSignalingServer.Text;
21 if (string.IsNullOrEmpty(signalingServerUrl))
22 {
23 MessageBox.Show("Please enter a signaling server URL.");
24 return;
25 }
26
27 InitializeWebRTC(signalingServerUrl);
28 }
29
30 private void InitializeWebRTC(string signalingServerUrl)
31 {
32 // Initialize WebRTC configuration
33 RTCConfiguration config = new RTCConfiguration
34 {
35 iceServers = new List<RTCIceServer>
36 {
37 new RTCIceServer { urls = "stun:stun.l.google.com:19302" }
38 }
39 };
40
41 _peerConnection = new RTCPeerConnection(config);
42
43 // Set up event handlers
44 _peerConnection.OnIceCandidate += (candidate) =>
45 {
46 lstLog.Items.Add($"ICE Candidate: {candidate}");
47 };
48
49 _peerConnection.OnTrack += (track) =>
50 {
51 lstLog.Items.Add($"New track received: {track.Kind}");
52 // Handle video rendering on pnlLocalVideo or pnlRemoteVideo
53 };
54
55 // Add media tracks
56 MediaStream mediaStream = new MediaStream();
57 var audioTrack = new MediaStreamTrack(MediaStreamTrackKind.Audio);
58 mediaStream.AddTrack(audioTrack);
59 _peerConnection.AddStream(mediaStream);
60
61 // Establish WebSocket connection for signaling
62 _webSocket = new WebSocket(signalingServerUrl);
63 _webSocket.OnMessage += (sender, e) =>
64 {
65 var message = e.Data;
66 lstLog.Items.Add($"Signaling Message: {message}");
67
68 if (message.Contains("offer"))
69 {
70 var offer = new RTCSessionDescriptionInit
71 {
72 type = RTCSdpType.offer,
73 sdp = message
74 };
75 _peerConnection.SetRemoteDescription(offer);
76 var answer = _peerConnection.CreateAnswer(null);
77 _peerConnection.SetLocalDescription(answer);
78 _webSocket.Send(answer.sdp);
79 }
80 else if (message.Contains("answer"))
81 {
82 var answer = new RTCSessionDescriptionInit
83 {
84 type = RTCSdpType.answer,
85 sdp = message
86 };
87 _peerConnection.SetRemoteDescription(answer);
88 }
89 else if (message.Contains("candidate"))
90 {
91 var candidate = new RTCIceCandidateInit
92 {
93 candidate = message
94 };
95 _peerConnection.AddIceCandidate(candidate);
96 }
97 };
98
99 _webSocket.Connect();
100 lstLog.Items.Add("WebSocket Signaling Connected.");
101 }
102 }
103
[d] Running the Application
To run the application, press
F5
in Visual Studio. This will compile and execute your application, opening the Windows Forms UI that you designed.By following these steps, you have created a basic UI for your SipSorcery WebRTC application and connected it to the backend logic. You are now ready to proceed to the next steps, where we will implement additional features such as the join screen, media controls, and participant views.
Step 3: Implement Join Screen
The join screen is a crucial component of any WebRTC application, as it serves as the entry point for users to connect to the session. This screen typically includes fields for entering user details and buttons to join or create a session. In this section, we will design and implement a join screen for your SipSorcery WebRTC application.
Designing the Join Screen Interface
To design the join screen, we will extend our existing Windows Forms application by adding new UI elements. These elements will collect the necessary information from the user and trigger the connection process when the user clicks the join button.
[a] Adding UI Components
Open the
Form1.cs
file in the Designer view and add the following components:- Label: To prompt the user for their name.
- TextBox: For the user to enter their name.
- Button: For the user to join the session.
Example Layout:
csharp
1 // Form1.Designer.cs
2 private System.Windows.Forms.Label lblName;
3 private System.Windows.Forms.TextBox txtName;
4 private System.Windows.Forms.Button btnJoin;
5
6 private void InitializeComponent()
7 {
8 this.lblName = new System.Windows.Forms.Label();
9 this.txtName = new System.Windows.Forms.TextBox();
10 this.btnJoin = new System.Windows.Forms.Button();
11
12 // lblName
13 this.lblName.AutoSize = true;
14 this.lblName.Location = new System.Drawing.Point(12, 40);
15 this.lblName.Name = "lblName";
16 this.lblName.Size = new System.Drawing.Size(38, 13);
17 this.lblName.TabIndex = 0;
18 this.lblName.Text = "Name:";
19
20 // txtName
21 this.txtName.Location = new System.Drawing.Point(56, 37);
22 this.txtName.Name = "txtName";
23 this.txtName.Size = new System.Drawing.Size(200, 20);
24 this.txtName.TabIndex = 1;
25
26 // btnJoin
27 this.btnJoin.Location = new System.Drawing.Point(270, 35);
28 this.btnJoin.Name = "btnJoin";
29 this.btnJoin.Size = new System.Drawing.Size(75, 23);
30 this.btnJoin.TabIndex = 2;
31 this.btnJoin.Text = "Join";
32 this.btnJoin.UseVisualStyleBackColor = true;
33 this.btnJoin.Click += new System.EventHandler(this.btnJoin_Click);
34
35 // Adding components to the form
36 this.Controls.Add(this.lblName);
37 this.Controls.Add(this.txtName);
38 this.Controls.Add(this.btnJoin);
39
40 // Other existing components
41 // ...
42 }
43
[b] Handling User Input and Validations
Next, we need to handle the user's input and validate it before proceeding with the connection. We'll do this by implementing the
btnJoin_Click
event handler.Code Snippets:
csharp
1 // Form1.cs
2 using System;
3 using System.Windows.Forms;
4
5 public partial class Form1 : Form
6 {
7 private string _userName;
8
9 public Form1()
10 {
11 InitializeComponent();
12 }
13
14 private void btnJoin_Click(object sender, EventArgs e)
15 {
16 _userName = txtName.Text.Trim();
17 if (string.IsNullOrEmpty(_userName))
18 {
19 MessageBox.Show("Please enter your name.");
20 return;
21 }
22
23 ConnectToSession();
24 }
25
26 private void ConnectToSession()
27 {
28 // Use the _userName variable as needed
29 lstLog.Items.Add($"User {_userName} is joining the session...");
30
31 // Proceed with WebRTC connection setup
32 InitializeWebRTC();
33 }
34
35 private void InitializeWebRTC()
36 {
37 // WebRTC initialization logic
38 // ...
39 }
40 }
41
[c] Integrating with SipSorcery Backend
In the
ConnectToSession
method, we use the user's name to personalize the connection process. The InitializeWebRTC
method, as defined earlier, sets up the WebRTC environment and signaling. Ensure this method includes necessary adjustments to handle user-specific details if required.Example Integration:
csharp
1 private void InitializeWebRTC()
2 {
3 // Initialize WebRTC configuration
4 RTCConfiguration config = new RTCConfiguration
5 {
6 iceServers = new List<RTCIceServer>
7 {
8 new RTCIceServer { urls = "stun:stun.l.google.com:19302" }
9 }
10 };
11
12 _peerConnection = new RTCPeerConnection(config);
13
14 // Set up event handlers
15 _peerConnection.OnIceCandidate += (candidate) =>
16 {
17 lstLog.Items.Add($"ICE Candidate: {candidate}");
18 };
19
20 _peerConnection.OnTrack += (track) =>
21 {
22 lstLog.Items.Add($"New track received: {track.Kind}");
23 // Handle video rendering on pnlLocalVideo or pnlRemoteVideo
24 };
25
26 // Add media tracks
27 MediaStream mediaStream = new MediaStream();
28 var audioTrack = new MediaStreamTrack(MediaStreamTrackKind.Audio);
29 mediaStream.AddTrack(audioTrack);
30 _peerConnection.AddStream(mediaStream);
31
32 // Establish WebSocket connection for signaling
33 _webSocket = new WebSocket("wss://your_signaling_server");
34 _webSocket.OnMessage += (sender, e) =>
35 {
36 var message = e.Data;
37 lstLog.Items.Add($"Signaling Message: {message}");
38
39 if (message.Contains("offer"))
40 {
41 var offer = new RTCSessionDescriptionInit
42 {
43 type = RTCSdpType.offer,
44 sdp = message
45 };
46 _peerConnection.SetRemoteDescription(offer);
47 var answer = _peerConnection.CreateAnswer(null);
48 _peerConnection.SetLocalDescription(answer);
49 _webSocket.Send(answer.sdp);
50 }
51 else if (message.Contains("answer"))
52 {
53 var answer = new RTCSessionDescriptionInit
54 {
55 type = RTCSdpType.answer,
56 sdp = message
57 };
58 _peerConnection.SetRemoteDescription(answer);
59 }
60 else if (message.Contains("candidate"))
61 {
62 var candidate = new RTCIceCandidateInit
63 {
64 candidate = message
65 };
66 _peerConnection.AddIceCandidate(candidate);
67 }
68 };
69
70 _webSocket.Connect();
71 lstLog.Items.Add("WebSocket Signaling Connected.");
72 }
73
[d] Running the Application
To run the application, press
F5
in Visual Studio. This will compile and execute your application, displaying the join screen where users can enter their name and join the WebRTC session.By following these steps, you have successfully designed and implemented a join screen for your SipSorcery WebRTC application. This screen allows users to enter their details and connect to the session, providing a seamless entry point for your WebRTC application. In the next steps, we will implement additional features such as media controls and participant views.
Step 4: Implement Controls
Implementing controls for your SipSorcery WebRTC application is essential for managing the media streams effectively. Controls allow users to mute/unmute audio, start/stop video, and perform other necessary actions during a WebRTC session. In this section, we will add media control buttons to the UI and connect them to the backend logic to handle these functionalities.
Adding Media Control Buttons to the UI
To start, we'll extend the existing UI by adding buttons for media controls. These controls will allow users to toggle audio and video streams during the session.
[a] Adding UI Components
Open the
Form1.cs
file in the Designer view and add the following components:- Button: To mute/unmute audio.
- Button: To start/stop video.
Example Layout:
csharp
1 // Form1.Designer.cs
2 private System.Windows.Forms.Button btnMute;
3 private System.Windows.Forms.Button btnVideo;
4
5 private void InitializeComponent()
6 {
7 this.btnMute = new System.Windows.Forms.Button();
8 this.btnVideo = new System.Windows.Forms.Button();
9
10 // btnMute
11 this.btnMute.Location = new System.Drawing.Point(12, 350);
12 this.btnMute.Name = "btnMute";
13 this.btnMute.Size = new System.Drawing.Size(75, 23);
14 this.btnMute.TabIndex = 5;
15 this.btnMute.Text = "Mute";
16 this.btnMute.UseVisualStyleBackColor = true;
17 this.btnMute.Click += new System.EventHandler(this.btnMute_Click);
18
19 // btnVideo
20 this.btnVideo.Location = new System.Drawing.Point(100, 350);
21 this.btnVideo.Name = "btnVideo";
22 this.btnVideo.Size = new System.Drawing.Size(75, 23);
23 this.btnVideo.TabIndex = 6;
24 this.btnVideo.Text = "Stop Video";
25 this.btnVideo.UseVisualStyleBackColor = true;
26 this.btnVideo.Click += new System.EventHandler(this.btnVideo_Click);
27
28 // Adding components to the form
29 this.Controls.Add(this.btnMute);
30 this.Controls.Add(this.btnVideo);
31
32 // Other existing components
33 // ...
34 }
35
[b] Implementing Event Handlers for the Controls
Next, we need to implement the event handlers for the buttons to toggle the audio and video streams. We'll do this by creating methods that handle these actions in the
Form1.cs
file.Code Snippets:
csharp
1 // Form1.cs
2 using System;
3 using System.Windows.Forms;
4 using SIPSorcery.Net;
5
6 public partial class Form1 : Form
7 {
8 private RTCPeerConnection _peerConnection;
9 private WebSocket _webSocket;
10 private bool _isMuted = false;
11 private bool _isVideoStopped = false;
12 private MediaStreamTrack _audioTrack;
13 private MediaStreamTrack _videoTrack;
14
15 public Form1()
16 {
17 InitializeComponent();
18 }
19
20 private void btnMute_Click(object sender, EventArgs e)
21 {
22 _isMuted = !_isMuted;
23 if (_audioTrack != null)
24 {
25 _audioTrack.Enabled = !_isMuted;
26 btnMute.Text = _isMuted ? "Unmute" : "Mute";
27 }
28 }
29
30 private void btnVideo_Click(object sender, EventArgs e)
31 {
32 _isVideoStopped = !_isVideoStopped;
33 if (_videoTrack != null)
34 {
35 _videoTrack.Enabled = !_isVideoStopped;
36 btnVideo.Text = _isVideoStopped ? "Start Video" : "Stop Video";
37 }
38 }
39
40 private void InitializeWebRTC()
41 {
42 // Initialize WebRTC configuration
43 RTCConfiguration config = new RTCConfiguration
44 {
45 iceServers = new List<RTCIceServer>
46 {
47 new RTCIceServer { urls = "stun:stun.l.google.com:19302" }
48 }
49 };
50
51 _peerConnection = new RTCPeerConnection(config);
52
53 // Set up event handlers
54 _peerConnection.OnIceCandidate += (candidate) =>
55 {
56 lstLog.Items.Add($"ICE Candidate: {candidate}");
57 };
58
59 _peerConnection.OnTrack += (track) =>
60 {
61 lstLog.Items.Add($"New track received: {track.Kind}");
62 // Handle video rendering on pnlLocalVideo or pnlRemoteVideo
63 };
64
65 // Add media tracks
66 MediaStream mediaStream = new MediaStream();
67 _audioTrack = new MediaStreamTrack(MediaStreamTrackKind.Audio);
68 _videoTrack = new MediaStreamTrack(MediaStreamTrackKind.Video);
69 mediaStream.AddTrack(_audioTrack);
70 mediaStream.AddTrack(_videoTrack);
71 _peerConnection.AddStream(mediaStream);
72
73 // Establish WebSocket connection for signaling
74 _webSocket = new WebSocket("wss://your_signaling_server");
75 _webSocket.OnMessage += (sender, e) =>
76 {
77 var message = e.Data;
78 lstLog.Items.Add($"Signaling Message: {message}");
79
80 if (message.Contains("offer"))
81 {
82 var offer = new RTCSessionDescriptionInit
83 {
84 type = RTCSdpType.offer,
85 sdp = message
86 };
87 _peerConnection.SetRemoteDescription(offer);
88 var answer = _peerConnection.CreateAnswer(null);
89 _peerConnection.SetLocalDescription(answer);
90 _webSocket.Send(answer.sdp);
91 }
92 else if (message.Contains("answer"))
93 {
94 var answer = new RTCSessionDescriptionInit
95 {
96 type = RTCSdpType.answer,
97 sdp = message
98 };
99 _peerConnection.SetRemoteDescription(answer);
100 }
101 else if (message.Contains("candidate"))
102 {
103 var candidate = new RTCIceCandidateInit
104 {
105 candidate = message
106 };
107 _peerConnection.AddIceCandidate(candidate);
108 }
109 };
110
111 _webSocket.Connect();
112 lstLog.Items.Add("WebSocket Signaling Connected.");
113 }
114 }
115
[c] Running the Application
To run the application, press
F5
in Visual Studio. This will compile and execute your application, opening the Windows Forms UI with the new media control buttons.- Mute/Unmute: Click the "Mute" button to mute your audio, and click it again to unmute.
- Start/Stop Video: Click the "Stop Video" button to stop your video stream, and click it again to start the video.
By following these steps, you have successfully implemented media controls for your SipSorcery WebRTC application. These controls allow users to manage their audio and video streams effectively, enhancing the overall user experience. In the next steps, we will implement the participant view and handle dynamic layouts for multiple video streams.
Step 5: Implement Participant View
Implementing a participant view is crucial for displaying multiple participants in a WebRTC session. This involves creating a dynamic layout that can handle video streams from various users as they join or leave the session. In this section, we will design and implement the participant view for your SipSorcery WebRTC application.
Designing the Participant View Layout
To design the participant view, we will extend the existing UI by adding panels to display the video streams of participants. These panels will dynamically adjust as participants join or leave the session.
[a] Adding UI Components
Open the
Form1.cs
file in the Designer view and add a container (e.g., FlowLayoutPanel
) to hold the video panels for participants.Example Layout:
csharp
1 // Form1.Designer.cs
2 private System.Windows.Forms.FlowLayoutPanel flpParticipants;
3
4 private void InitializeComponent()
5 {
6 this.flpParticipants = new System.Windows.Forms.FlowLayoutPanel();
7
8 // flpParticipants
9 this.flpParticipants.Location = new System.Drawing.Point(12, 160);
10 this.flpParticipants.Name = "flpParticipants";
11 this.flpParticipants.Size = new System.Drawing.Size(480, 180);
12 this.flpParticipants.TabIndex = 5;
13
14 // Adding components to the form
15 this.Controls.Add(this.flpParticipants);
16
17 // Other existing components
18 // ...
19 }
20
[b] Rendering Participant Streams
To display the video streams, we need to handle the
OnTrack
event and dynamically create video panels for each participant.Code Snippets:
csharp
1 // Form1.cs
2 using System;
3 using System.Drawing;
4 using System.Windows.Forms;
5 using SIPSorcery.Net;
6 using SIPSorceryMedia;
7
8 public partial class Form1 : Form
9 {
10 private RTCPeerConnection _peerConnection;
11 private WebSocket _webSocket;
12
13 public Form1()
14 {
15 InitializeComponent();
16 }
17
18 private void InitializeWebRTC()
19 {
20 // Initialize WebRTC configuration
21 RTCConfiguration config = new RTCConfiguration
22 {
23 iceServers = new List<RTCIceServer>
24 {
25 new RTCIceServer { urls = "stun:stun.l.google.com:19302" }
26 }
27 };
28
29 _peerConnection = new RTCPeerConnection(config);
30
31 // Set up event handlers
32 _peerConnection.OnIceCandidate += (candidate) =>
33 {
34 lstLog.Items.Add($"ICE Candidate: {candidate}");
35 };
36
37 _peerConnection.OnTrack += (track) =>
38 {
39 lstLog.Items.Add($"New track received: {track.Kind}");
40 Invoke((Action)(() => AddParticipantVideo(track)));
41 };
42
43 // Add media tracks
44 MediaStream mediaStream = new MediaStream();
45 var audioTrack = new MediaStreamTrack(MediaStreamTrackKind.Audio);
46 var videoTrack = new MediaStreamTrack(MediaStreamTrackKind.Video);
47 mediaStream.AddTrack(audioTrack);
48 mediaStream.AddTrack(videoTrack);
49 _peerConnection.AddStream(mediaStream);
50
51 // Establish WebSocket connection for signaling
52 _webSocket = new WebSocket("wss://your_signaling_server");
53 _webSocket.OnMessage += (sender, e) =>
54 {
55 var message = e.Data;
56 lstLog.Items.Add($"Signaling Message: {message}");
57
58 if (message.Contains("offer"))
59 {
60 var offer = new RTCSessionDescriptionInit
61 {
62 type = RTCSdpType.offer,
63 sdp = message
64 };
65 _peerConnection.SetRemoteDescription(offer);
66 var answer = _peerConnection.CreateAnswer(null);
67 _peerConnection.SetLocalDescription(answer);
68 _webSocket.Send(answer.sdp);
69 }
70 else if (message.Contains("answer"))
71 {
72 var answer = new RTCSessionDescriptionInit
73 {
74 type = RTCSdpType.answer,
75 sdp = message
76 };
77 _peerConnection.SetRemoteDescription(answer);
78 }
79 else if (message.Contains("candidate"))
80 {
81 var candidate = new RTCIceCandidateInit
82 {
83 candidate = message
84 };
85 _peerConnection.AddIceCandidate(candidate);
86 }
87 };
88
89 _webSocket.Connect();
90 lstLog.Items.Add("WebSocket Signaling Connected.");
91 }
92
93 private void AddParticipantVideo(MediaStreamTrack track)
94 {
95 var videoPanel = new Panel
96 {
97 Width = 160,
98 Height = 120,
99 BorderStyle = BorderStyle.FixedSingle
100 };
101
102 var videoLabel = new Label
103 {
104 Text = track.Kind,
105 Dock = DockStyle.Top,
106 TextAlign = ContentAlignment.MiddleCenter
107 };
108
109 videoPanel.Controls.Add(videoLabel);
110
111 // For actual video rendering, integrate with a video library like DirectShow, FFmpeg, etc.
112 // Example:
113 // var videoPlayer = new VideoPlayer(track);
114 // videoPlayer.Dock = DockStyle.Fill;
115 // videoPanel.Controls.Add(videoPlayer);
116
117 flpParticipants.Controls.Add(videoPanel);
118 }
119
[c] Handling Dynamic Layouts
As participants join or leave the session, the layout of the participant view should adjust dynamically. The
FlowLayoutPanel
automatically handles the flow and alignment of child controls. When a new participant joins, a new panel is added to the FlowLayoutPanel
, and when a participant leaves, the corresponding panel can be removed.Code Snippets:
csharp
1 private void RemoveParticipantVideo(MediaStreamTrack track)
2 {
3 foreach (Control control in flpParticipants.Controls)
4 {
5 if (control is Panel panel && panel.Controls[0] is Label label && label.Text == track.Kind)
6 {
7 flpParticipants.Controls.Remove(panel);
8 break;
9 }
10 }
11 }
12
13 private void InitializeWebRTC()
14 {
15 // Existing initialization code
16
17 // Additional event handler for removing tracks
18 _peerConnection.OnRemoveTrack += (track) =>
19 {
20 lstLog.Items.Add($"Track removed: {track.Kind}");
21 Invoke((Action)(() => RemoveParticipantVideo(track)));
22 };
23 }
24
[d] Running the Application
To run the application, press
F5
in Visual Studio. This will compile and execute your application, displaying the participant view where multiple video streams can be shown dynamically as participants join or leave the WebRTC session.By following these steps, you have successfully implemented a participant view for your SipSorcery WebRTC application. This view dynamically adjusts to display video streams from multiple participants, enhancing the overall user experience. In the next step, we will focus on running your complete WebRTC application and ensuring everything works as expected.
Step 6: Run Your Code Now
After implementing the participant view and all the necessary functionalities, it's time to compile and run your SipSorcery WebRTC application. This final step involves testing the application to ensure everything works as expected, from establishing connections to managing media streams and handling dynamic layouts for multiple participants.
Final Setup Steps Before Running the Application
Verify Your Code
Ensure all the code is correctly implemented and there are no syntax errors. Review each part to confirm that all necessary components are included.
Dependencies and Libraries
Make sure all required dependencies and libraries are installed. You can use NuGet Package Manager in Visual Studio to manage and install any missing packages.
Configuration
Double-check your WebSocket signaling server URL and ensure it's correctly configured in the
InitializeWebRTC
method.Running the Application
Compile and Run
Press
F5
in Visual Studio or use the following command in the terminal to compile and run the application:bash
1 dotnet run
2
Testing Functionality
- Join Screen: Enter your name and the signaling server URL, then click "Join" to connect to the session.
- Media Controls: Use the "Mute" and "Stop Video" buttons to toggle audio and video streams.
- Participant View: Ensure that video panels for participants are dynamically added and removed as users join and leave the session.
Troubleshooting Common Issues
- Connection Problems: If you encounter issues connecting to the signaling server, verify the server URL and ensure the server is running.
- Media Stream Issues: If audio or video streams are not working, check the initialization of media tracks and ensure they are correctly added to the peer connection.
- UI Layout Problems: If the participant view layout is not displaying correctly, review the layout configuration in the
FlowLayoutPanel
and ensure panels are being added and removed as expected.
Verifying Functionality
Test with Multiple Participants
- Open multiple instances of the application and connect to the same signaling server.
- Verify that video streams from different participants are displayed correctly.
- Test the media controls for each participant to ensure they work independently.
Stress Testing
- Simulate a scenario with a large number of participants to test the application's performance.
- Monitor the application's behavior and ensure it handles the load without crashes or significant performance degradation.
Edge Cases
- Test edge cases such as participants joining and leaving rapidly.
- Check the application's stability and ensure it recovers gracefully from unexpected scenarios.
Conclusion
Congratulations! You have successfully built and run your SipSorcery WebRTC application. By following the steps outlined in this guide, you have implemented key functionalities including initializing the WebRTC environment, creating a join screen, implementing media controls, and designing a dynamic participant view.
This application provides a robust foundation for building advanced WebRTC applications using SipSorcery and C#. You can further enhance it by adding features such as screen sharing, recording, or integrating with other services.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ