What is AIORTC WebRTC?
AIORTC WebRTC is an open-source library that brings the power of WebRTC to Python developers. WebRTC itself is a set of protocols and APIs that enable real-time communication directly between browsers and devices. It supports video, voice, and generic data to be sent between peers, allowing developers to create high-performance, real-time communication applications without the need for proprietary plugins or additional software.
AIORTC WebRTC
is a powerful tool in the realm of real-time communication technologies, facilitating seamless interaction over the internet. Leveraging WebRTC (Web Real-Time Communication
) protocols, AIORTC provides a Python-based framework for implementing real-time audio, video, and data communication in applications. This technology is increasingly essential in modern applications where immediate interaction and data exchange are critical, such as video conferencing, live streaming, and IoT (Internet of Things) integrations.In today's interconnected world, real-time communication is a cornerstone for various applications, from social media to telemedicine. AIORTC stands out by offering a robust, Python-centric approach, making it accessible to a wide range of developers and simplifying the integration of real-time capabilities into diverse projects. Its relevance extends beyond just desktop and mobile applications, significantly impacting server environments and IoT systems, where real-time data exchange is paramount.
Key features of AIORTC include
- Peer-to-Peer Communication: Establishes direct communication between peers, reducing latency and enhancing performance.
- Media Stream Handling: Manages audio and video streams efficiently, making it ideal for video conferencing and streaming applications.
- Data Channels: Facilitates the exchange of arbitrary data between peers, enabling applications like file sharing and collaborative editing.
- Python Integration: Provides a Pythonic interface to WebRTC, making it easier for Python developers to leverage this powerful technology.
By integrating AIORTC into Python applications, developers can harness the full potential of WebRTC in a familiar programming environment. This integration is particularly beneficial for server-side applications and IoT devices, where Python is often the language of choice due to its simplicity and versatility. AIORTC thus bridges the gap between real-time communication needs and Python's extensive ecosystem, paving the way for innovative applications and solutions in various domains.
Getting Started with the Code
Create a New AIORTC WebRTC App
Creating a new AIORTC WebRTC application involves setting up the development environment and ensuring all necessary dependencies are installed. Here's a step-by-step guide to get you started:
Setting Up the Development Environment
Before diving into the code, you need to set up your development environment. Ensure you have Python installed on your system. It's recommended to use a virtual environment to manage your project dependencies effectively.
[a] Install Python
Download and install the latest version of Python from
python.org
.[b] Set Up a Virtual Environment
bash
1 python -m venv aiortc_env
2 source aiortc_env/bin/activate # On Windows use `aiortc_env\Scripts\activate`
3
Install AIORTC
With your environment set up, the next step is to install the AIORTC library. You can do this using pip, Python's package installer.
[a] Install AIORTC
bash
1 pip install aiortc
2
[b] Verify Installation
Ensure the installation is successful by importing AIORTC in a Python script.
Python
1 import aiortc
2 print("AIORTC installed successfully!")
3
Structure of the Project
A well-structured project layout is crucial for maintainability and scalability. Here’s a recommended structure for your AIORTC WebRTC application:
1aiortc_webrtc_app/
2├── main.py
3├── signaling.py
4├── peer.py
5├── static/
6│ ├── css/
7│ └── js/
8├── templates/
9│ └── index.html
10├── README.md
11└── requirements.txt
12
- main.py: The entry point of your application.
- signaling.py: Handles signaling mechanisms between peers.
- peer.py: Manages peer connections and media streams.
- static/: Contains static files like CSS and JavaScript.
- templates/: Holds HTML templates for your application’s UI.
- README.md: Documentation for your project.
- requirements.txt: Lists all the dependencies for your project.
App Architecture
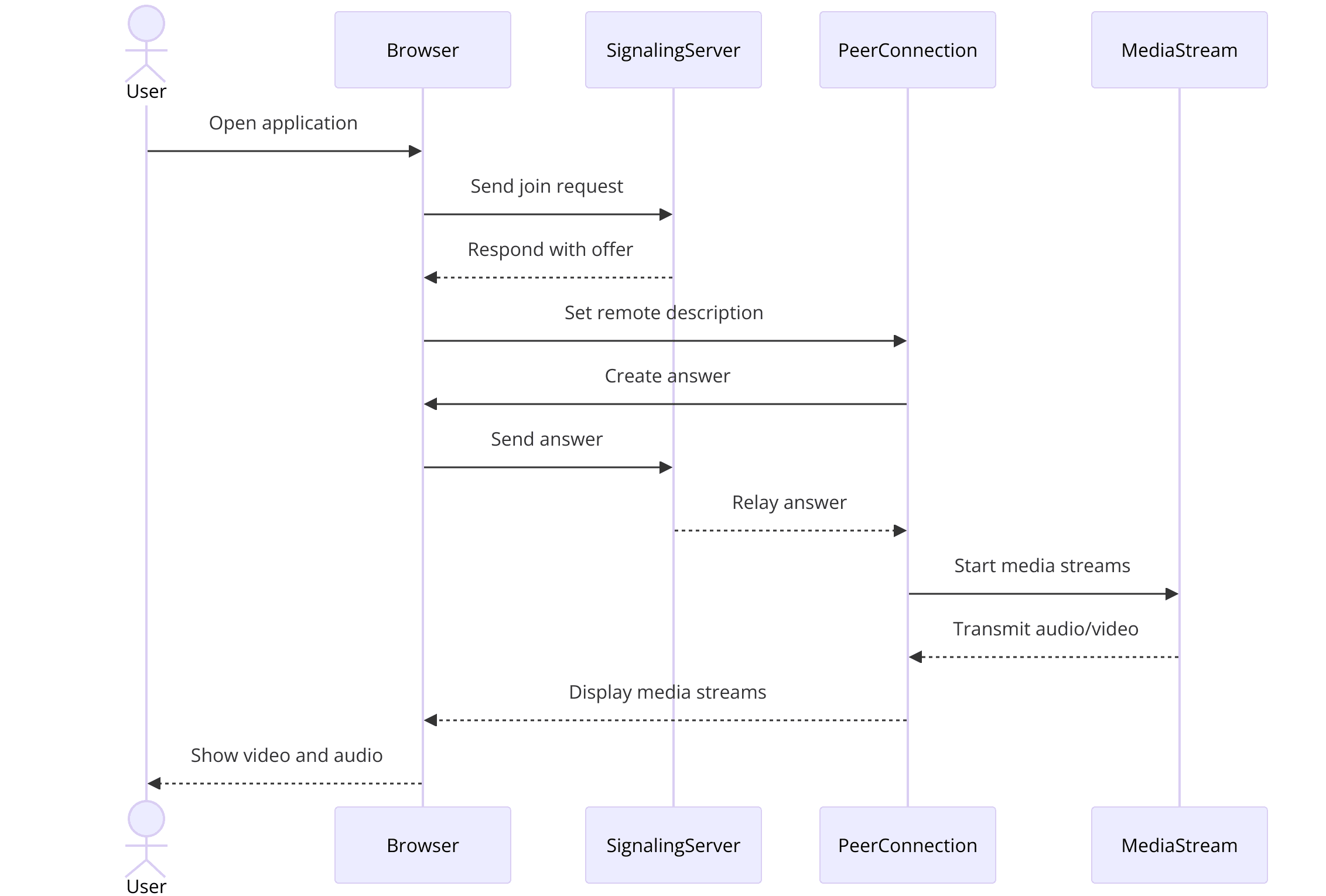
Client-Side
- HTML/CSS/JavaScript: For the user interface and handling user interactions.
- WebRTC API: Used to capture and transmit media streams.
Server-Side
- Python with AIORTC: Manages signaling, peer connections, and media processing.
- WebSocket Server: Facilitates real-time communication between clients and the server for signaling purposes.
Overview of the Application Architecture
- User Interface: The front-end interface allows users to join the communication session, manage controls, and view participants.
- Signaling Server: The server handles signaling data (session initiation, ICE candidates) to establish peer connections.
- Media Processing: AIORTC processes media streams, handles encoding/decoding, and manages peer connections.
By setting up your development environment, installing AIORTC, and structuring your project effectively, you lay a solid foundation for building a robust AIORTC WebRTC application. The following sections will dive deeper into the implementation details, guiding you through creating a fully functional real-time communication app using AIORTC.
Step 1: Get Started with Main.py
Creating the Entry Point
The
main.py
file serves as the entry point for your AIORTC WebRTC application. This is where you'll set up the basic configuration, import necessary libraries, and write the main function to initialize and run your application.[a] Setting Up main.py
Start by creating a new file named
main.py
in the root directory of your project. This file will contain the core logic to start your application.[b] Importing Necessary Libraries
You'll need to import several libraries to manage WebRTC functionality, handle signaling, and run the server. Here are the essential imports:
Python
1import asyncio
2import logging
3from aiortc import RTCPeerConnection, RTCSessionDescription
4from aiohttp import web
5import json
6
[c] Basic Configuration and Setup
Next, configure the logging and set up the basic routes for your web server.
Python
1logging.basicConfig(level=logging.INFO)
2
3async def index(request):
4 content = open('templates/index.html', 'r').read()
5 return web.Response(content_type='text/html', text=content)
6
7app = web.Application()
8app.router.add_get('/', index)
9
Writing the Main Function
The main function initializes the web server and sets up the WebRTC connections. This involves creating signaling mechanisms and handling peer connections.
[a] Creating the Main Function
Define the
main
function that will start the web server and manage WebRTC signaling:Python
1async def main():
2 # Create a WebRTC peer connection
3 pc = RTCPeerConnection()
4
5 # Define the signaling mechanism
6 async def offer(request):
7 params = await request.json()
8 offer = RTCSessionDescription(sdp=params['sdp'], type=params['type'])
9
10 await pc.setRemoteDescription(offer)
11 answer = await pc.createAnswer()
12 await pc.setLocalDescription(answer)
13
14 return web.Response(
15 content_type='application/json',
16 text=json.dumps({'sdp': pc.localDescription.sdp, 'type': pc.localDescription.type})
17 )
18
19 app.router.add_post('/offer', offer)
20
21 # Start the web server
22 runner = web.AppRunner(app)
23 await runner.setup()
24 site = web.TCPSite(runner, 'localhost', 8080)
25 await site.start()
26
27 logging.info("Server started at http://localhost:8080")
28
29# Run the main function
30if __name__ == '__main__':
31 asyncio.run(main())
32
[b] Handling WebRTC Signaling
The
offer
function handles the WebRTC signaling process. It receives an offer from a client, sets it as the remote description for the peer connection, creates an answer, and sends the answer back to the client.[c] Running the Server
Finally, the
main
function starts the web server on localhost:8080
. When you run this script, your server will be up and running, ready to handle WebRTC connections.Testing Your Setup
Run the Server
Ensure your virtual environment is activated and run the
main.py
script:bash
1python main.py
2
Verify the Server is Running
Open a web browser and navigate to
http://localhost:8080
. You should see the content of index.html
displayed.By completing these steps, you've set up the core structure for your AIORTC WebRTC application. The
main.py
file now serves as the backbone of your application, handling WebRTC peer connections and signaling. In the next parts, you'll build on this foundation by adding more components and functionality to create a fully-fledged real-time communication app.Step 2: Wireframe All the Components
Designing the Application Structure
Before diving into the implementation details, it's essential to plan and wireframe the main components of your AIORTC WebRTC application. This step ensures that you have a clear roadmap for your development process, helping you visualize the user interface and understand how different components interact with each other.
Planning the UI and UX Components
Start by sketching a basic layout of your application. The main components to consider include:
- Join Screen: Where users enter their information and join the session.
- Control Panel: Provides controls for muting/unmuting, starting/stopping the video, etc.
- Participant View: Displays the video and audio streams of all participants.
Wireframing the Main Components
Use a tool like Figma, Sketch, or even pen and paper to create a wireframe of your application's UI. This wireframe will serve as a blueprint for the subsequent development stages.
Defining Key Components
With the wireframe in place, you can now define the key components of your application. Each component will have specific roles and responsibilities, ensuring a modular and maintainable codebase.
[a] Join Screen
The join screen is the first point of interaction for users. It should be simple and intuitive, allowing users to quickly enter their information and join the session.
- HTML: The join screen will have input fields for the user's name and a button to join the session.
- CSS: Styles to ensure a clean and responsive design.
- JavaScript: Handles the form submission and triggers the connection setup.
Example
index.html
structure for the join screen:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>AIORTC WebRTC App</title>
6 <link rel="stylesheet" href="static/css/style.css">
7</head>
8<body>
9 <div id="join-screen">
10 <h2>Join the Session</h2>
11 <input type="text" id="username" placeholder="Enter your name">
12 <button id="join-button">Join</button>
13 </div>
14 <script src="static/js/main.js"></script>
15</body>
16</html>
17
[b] Control Panel
The control panel provides users with functionalities to control their audio and video streams. This includes buttons to mute/unmute the microphone, start/stop the video, and possibly share the screen.
- HTML: Buttons for each control feature.
- CSS: Styles for a user-friendly control panel.
- JavaScript: Event handlers for each control button.
Example control panel structure in
index.html
:HTML
1<div id="control-panel">
2 <button id="mute-button">Mute</button>
3 <button id="video-button">Stop Video</button>
4</div>
5
[c] Participant View
The participant view displays the video and audio streams of all participants in the session. It should dynamically adjust as participants join or leave.
- HTML: Container elements to hold the video streams.
- CSS: Styles to ensure a responsive grid layout.
- JavaScript: Logic to handle the addition and removal of video streams dynamically.
Example participant view structure in
index.html
:HTML
1<div id="participant-view">
2 <!-- Video elements will be dynamically added here -->
3</div>
4
Explanation of Their Roles and Interactions
Join Screen Interaction
When the user enters their name and clicks the "Join" button, a JavaScript function captures this input and initiates the WebRTC connection process. The server (handled in
main.py
) receives the join request and sets up the peer connection.Control Panel Interaction
The control buttons allow users to control their audio and video streams. For example, clicking the "Mute" button will mute the user's microphone. JavaScript functions handle these actions and update the peer connection accordingly.
Participant View Interaction
As users join the session, their video streams are added to the participant view. JavaScript functions dynamically create video elements and append them to the participant view container. When a user leaves, their video element is removed, ensuring the layout remains clean and organized.
By defining and wireframing these key components, you've set the stage for implementing a user-friendly and functional AIORTC WebRTC application. In the next steps, you'll focus on bringing these components to life with actual code, ensuring a seamless real-time communication experience.
Step 3: Implement Join Screen
Creating the Join Screen UI
The join screen is a crucial part of your AIORTC WebRTC application, as it serves as the entry point for users to connect to the session. Implementing this screen involves designing a simple yet intuitive interface and handling user inputs effectively.
[a] Designing the User Interface for the Join Screen
The join screen should be straightforward, allowing users to enter their name and join the session with a single click. Here's an example of how to set up the HTML and CSS for the join screen.
HTML Structure (
templates/index.html
):HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>AIORTC WebRTC App</title>
6 <link rel="stylesheet" href="static/css/style.css">
7</head>
8<body>
9 <div id="join-screen">
10 <h2>Join the Session</h2>
11 <input type="text" id="username" placeholder="Enter your name">
12 <button id="join-button">Join</button>
13 </div>
14 <script src="static/js/main.js"></script>
15</body>
16</html>
17
CSS for Styling (
static/css/style.css
):CSS
1body {
2 font-family: Arial, sans-serif;
3 background-color: #f0f0f0;
4 display: flex;
5 justify-content: center;
6 align-items: center;
7 height: 100vh;
8 margin: 0;
9}
10
11#join-screen {
12 background: #ffffff;
13 padding: 20px;
14 border-radius: 8px;
15 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
16 text-align: center;
17}
18
19#join-screen h2 {
20 margin-bottom: 20px;
21}
22
23#join-screen input {
24 width: 80%;
25 padding: 10px;
26 margin-bottom: 20px;
27 border: 1px solid #ccc;
28 border-radius: 4px;
29}
30
31#join-screen button {
32 padding: 10px 20px;
33 border: none;
34 border-radius: 4px;
35 background-color: #007bff;
36 color: white;
37 cursor: pointer;
38}
39
40#join-screen button:hover {
41 background-color: #0056b3;
42}
43
[b] Handling User Input
Once the user enters their name and clicks the "Join" button, you need to capture this input and initiate the connection process. This involves writing JavaScript to handle the form submission and trigger the WebRTC setup.
JavaScript for Handling Input (
static/js/main.js
):JavaScript
1document.addEventListener('DOMContentLoaded', () => {
2 const joinButton = document.getElementById('join-button');
3 joinButton.addEventListener('click', async () => {
4 const username = document.getElementById('username').value.trim();
5 if (username) {
6 try {
7 await joinSession(username);
8 } catch (error) {
9 console.error('Error joining session:', error);
10 }
11 } else {
12 alert('Please enter your name');
13 }
14 });
15});
16
17async function joinSession(username) {
18 const response = await fetch('/offer', {
19 method: 'POST',
20 headers: {
21 'Content-Type': 'application/json'
22 },
23 body: JSON.stringify({ username })
24 });
25
26 if (!response.ok) {
27 throw new Error('Failed to join session');
28 }
29
30 const data = await response.json();
31 // Handle the WebRTC offer and set up the connection
32 console.log('Joined session:', data);
33}
34
[c] Capturing and Processing User Input
In the
main.js
script, the joinSession
function sends the user's name to the server when the "Join" button is clicked. The server (handled in main.py
) receives this data and sets up the peer connection.Server-Side Handling (
main.py
):Modify the
offer
function to handle the username and establish the WebRTC connection:Python
1async def offer(request):
2 params = await request.json()
3 username = params.get('username')
4 offer = RTCSessionDescription(sdp=params['sdp'], type=params['type'])
5
6 await pc.setRemoteDescription(offer)
7 answer = await pc.createAnswer()
8 await pc.setLocalDescription(answer)
9
10 response_data = {
11 'sdp': pc.localDescription.sdp,
12 'type': pc.localDescription.type,
13 'username': username
14 }
15 return web.Response(
16 content_type='application/json',
17 text=json.dumps(response_data)
18 )
19
By following these steps, you've implemented a functional join screen for your AIORTC WebRTC application. Users can now enter their name and join the session, triggering the WebRTC setup process and preparing for real-time communication. In the next step, you'll focus on implementing the control panel, adding more functionality to your application.
Step 4: Implement Controls
Adding Control Features
The control panel is a vital part of your AIORTC WebRTC application, providing users with the ability to manage their audio and video streams. Implementing basic controls such as mute/unmute and start/stop video enhances the user experience significantly.
[a] Implementing Basic Controls
To begin, you will create buttons for muting/unmuting the microphone and starting/stopping the video. These controls will interact with the WebRTC API to manage the media streams.
HTML for Control Panel (
templates/index.html
):Add the control panel within the body of your existing HTML structure:
HTML
1<body>
2 <div id="join-screen">
3 <h2>Join the Session</h2>
4 <input type="text" id="username" placeholder="Enter your name">
5 <button id="join-button">Join</button>
6 </div>
7 <div id="control-panel" style="display: none;">
8 <button id="mute-button">Mute</button>
9 <button id="video-button">Stop Video</button>
10 </div>
11 <div id="participant-view" style="display: none;">
12 <!-- Video elements will be dynamically added here -->
13 </div>
14 <script src="static/js/main.js"></script>
15</body>
16
CSS for Control Panel (
static/css/style.css
):CSS
1#control-panel {
2 margin-top: 20px;
3 text-align: center;
4}
5
6#control-panel button {
7 padding: 10px 20px;
8 margin: 0 10px;
9 border: none;
10 border-radius: 4px;
11 background-color: #007bff;
12 color: white;
13 cursor: pointer;
14}
15
16#control-panel button:hover {
17 background-color: #0056b3;
18}
19
[b] JavaScript for Handling Control Actions (static/js/main.js
)
Enhance the existing JavaScript to handle the mute/unmute and start/stop video functionalities.
JavaScript
1document.addEventListener('DOMContentLoaded', () => {
2 const joinButton = document.getElementById('join-button');
3 const muteButton = document.getElementById('mute-button');
4 const videoButton = document.getElementById('video-button');
5
6 let localStream = null;
7 let pc = null;
8
9 joinButton.addEventListener('click', async () => {
10 const username = document.getElementById('username').value.trim();
11 if (username) {
12 try {
13 await joinSession(username);
14 document.getElementById('join-screen').style.display = 'none';
15 document.getElementById('control-panel').style.display = 'block';
16 document.getElementById('participant-view').style.display = 'block';
17 } catch (error) {
18 console.error('Error joining session:', error);
19 }
20 } else {
21 alert('Please enter your name');
22 }
23 });
24
25 muteButton.addEventListener('click', () => {
26 if (localStream) {
27 const audioTrack = localStream.getAudioTracks()[0];
28 audioTrack.enabled = !audioTrack.enabled;
29 muteButton.textContent = audioTrack.enabled ? 'Mute' : 'Unmute';
30 }
31 });
32
33 videoButton.addEventListener('click', () => {
34 if (localStream) {
35 const videoTrack = localStream.getVideoTracks()[0];
36 videoTrack.enabled = !videoTrack.enabled;
37 videoButton.textContent = videoTrack.enabled ? 'Stop Video' : 'Start Video';
38 }
39 });
40
41 async function joinSession(username) {
42 const response = await fetch('/offer', {
43 method: 'POST',
44 headers: {
45 'Content-Type': 'application/json'
46 },
47 body: JSON.stringify({ username })
48 });
49
50 if (!response.ok) {
51 throw new Error('Failed to join session');
52 }
53
54 const data = await response.json();
55 pc = new RTCPeerConnection();
56 pc.ontrack = (event) => {
57 const video = document.createElement('video');
58 video.srcObject = event.streams[0];
59 video.autoplay = true;
60 document.getElementById('participant-view').appendChild(video);
61 };
62
63 localStream = await navigator.mediaDevices.getUserMedia({ audio: true, video: true });
64 localStream.getTracks().forEach(track => pc.addTrack(track, localStream));
65
66 const offer = new RTCSessionDescription(data);
67 await pc.setRemoteDescription(offer);
68 const answer = await pc.createAnswer();
69 await pc.setLocalDescription(answer);
70
71 await fetch('/answer', {
72 method: 'POST',
73 headers: {
74 'Content-Type': 'application/json'
75 },
76 body: JSON.stringify({
77 sdp: pc.localDescription.sdp,
78 type: pc.localDescription.type
79 })
80 });
81 }
82});
83
[c] Backend Integration
Ensure that your server-side code can handle the new signaling messages for the WebRTC connection.
Server-Side Handling (
main.py
):Add a new route to handle the answer from the client.
Python
1async def answer(request):
2 params = await request.json()
3 answer = RTCSessionDescription(sdp=params['sdp'], type=params['type'])
4
5 await pc.setRemoteDescription(answer)
6 return web.Response(status=200)
7
8app.router.add_post('/answer', answer)
9
[d] Ensuring Real-Time Updates and Feedback
With these changes, your application now includes a fully functional control panel that allows users to mute/unmute their microphone and start/stop their video. The JavaScript handles the user actions and updates the WebRTC connection accordingly. The server processes the necessary signaling messages to maintain the peer connections.
By implementing these controls, you have enhanced the interactivity and usability of your AIORTC WebRTC application, providing users with essential tools to manage their communication effectively. In the next step, you will focus on implementing the participant view to display video streams dynamically.
Step 5: Implement Participant View
Designing the Participant View
The participant view is a crucial component of your AIORTC WebRTC application, displaying the video and audio streams of all participants in the session. This step involves creating a responsive and adaptive layout that can dynamically adjust as participants join or leave the session.
[a] Layout and Design Considerations
The participant view should be flexible and able to handle multiple video streams. A grid layout is ideal for this purpose, ensuring that all video streams are visible and appropriately sized.
HTML Structure for Participant View (
templates/index.html
):Ensure the participant view container is included in your existing HTML structure:
HTML
1<body>
2 <div id="join-screen">
3 <h2>Join the Session</h2>
4 <input type="text" id="username" placeholder="Enter your name">
5 <button id="join-button">Join</button>
6 </div>
7 <div id="control-panel" style="display: none;">
8 <button id="mute-button">Mute</button>
9 <button id="video-button">Stop Video</button>
10 </div>
11 <div id="participant-view" style="display: none;">
12 <!-- Video elements will be dynamically added here -->
13 </div>
14 <script src="static/js/main.js"></script>
15</body>
16
CSS for Participant View (
static/css/style.css
):CSS
1#participant-view {
2 display: flex;
3 flex-wrap: wrap;
4 justify-content: center;
5 margin-top: 20px;
6}
7
8#participant-view video {
9 margin: 10px;
10 border: 1px solid #ccc;
11 border-radius: 8px;
12 width: 30%;
13 max-width: 400px;
14}
15
[b] Real-time Data Handling
The JavaScript logic for handling the participant view involves dynamically adding and removing video elements as participants join or leave the session.
JavaScript for Handling Participant View (
static/js/main.js
):Enhance the existing JavaScript to manage the participant view effectively.
JavaScript
1document.addEventListener('DOMContentLoaded', () => {
2 const joinButton = document.getElementById('join-button');
3 const muteButton = document.getElementById('mute-button');
4 const videoButton = document.getElementById('video-button');
5
6 let localStream = null;
7 let pc = null;
8 const participantView = document.getElementById('participant-view');
9
10 joinButton.addEventListener('click', async () => {
11 const username = document.getElementById('username').value.trim();
12 if (username) {
13 try {
14 await joinSession(username);
15 document.getElementById('join-screen').style.display = 'none';
16 document.getElementById('control-panel').style.display = 'block';
17 participantView.style.display = 'flex';
18 } catch (error) {
19 console.error('Error joining session:', error);
20 }
21 } else {
22 alert('Please enter your name');
23 }
24 });
25
26 muteButton.addEventListener('click', () => {
27 if (localStream) {
28 const audioTrack = localStream.getAudioTracks()[0];
29 audioTrack.enabled = !audioTrack.enabled;
30 muteButton.textContent = audioTrack.enabled ? 'Mute' : 'Unmute';
31 }
32 });
33
34 videoButton.addEventListener('click', () => {
35 if (localStream) {
36 const videoTrack = localStream.getVideoTracks()[0];
37 videoTrack.enabled = !videoTrack.enabled;
38 videoButton.textContent = videoTrack.enabled ? 'Stop Video' : 'Start Video';
39 }
40 });
41
42 async function joinSession(username) {
43 const response = await fetch('/offer', {
44 method: 'POST',
45 headers: {
46 'Content-Type': 'application/json'
47 },
48 body: JSON.stringify({ username })
49 });
50
51 if (!response.ok) {
52 throw new Error('Failed to join session');
53 }
54
55 const data = await response.json();
56 pc = new RTCPeerConnection();
57 pc.ontrack = (event) => {
58 const video = document.createElement('video');
59 video.srcObject = event.streams[0];
60 video.autoplay = true;
61 participantView.appendChild(video);
62 };
63
64 localStream = await navigator.mediaDevices.getUserMedia({ audio: true, video: true });
65 localStream.getTracks().forEach(track => pc.addTrack(track, localStream));
66
67 const offer = new RTCSessionDescription(data);
68 await pc.setRemoteDescription(offer);
69 const answer = await pc.createAnswer();
70 await pc.setLocalDescription(answer);
71
72 await fetch('/answer', {
73 method: 'POST',
74 headers: {
75 'Content-Type': 'application/json'
76 },
77 body: JSON.stringify({
78 sdp: pc.localDescription.sdp,
79 type: pc.localDescription.type
80 })
81 });
82 }
83});
84
[c] Managing Participant Data
The
ontrack
event handler in the JavaScript code dynamically creates and appends video elements to the participant-view
container when new media streams are received. This ensures that all participants' video streams are displayed in real-time.[d] Handling Participant Disconnects
To ensure the participant view remains clean and organized, you should also handle the removal of video elements when participants leave the session. This can be done by listening for the
onremovetrack
event or implementing a custom mechanism to detect when participants disconnect.JavaScript for Handling Participant Disconnects (
static/js/main.js
):JavaScript
1pc.onremovetrack = (event) => {
2 const stream = event.streams[0];
3 const videoElements = document.querySelectorAll('video');
4 videoElements.forEach(video => {
5 if (video.srcObject === stream) {
6 video.remove();
7 }
8 });
9};
10
By implementing the participant view, you ensure that your AIORTC WebRTC application can dynamically display video streams, providing a seamless and interactive real-time communication experience. In the final step, you will focus on running and testing your application to ensure everything works as expected.
Step 6: Run Your Code Now
Final Steps
Now that you've implemented the key components of your AIORTC WebRTC application, it's time to run and test the application to ensure everything works as expected.
Running and Testing the Application
Ensure your virtual environment is activated, and run the
main.py
script to start the server:bash
1python main.py
2
Open a web browser and navigate to
http://localhost:8080
. You should see the join screen where you can enter your name and join the session. Test the following functionalities:- Enter your name and click the "Join" button to initiate the WebRTC connection.
- Verify that the control panel and participant view are displayed after joining the session.
- Test the mute/unmute button to ensure the microphone can be toggled on and off.
- Test the start/stop video button to ensure the video stream can be toggled on and off.
- Join the session from multiple devices/browsers to ensure that all participants' video streams are displayed correctly.
Debugging Common Issues
During testing, you might encounter some common issues. Here are a few troubleshooting tips:
- No Video or Audio: Ensure that your browser has permissions to access the camera and microphone. Check the browser's security settings and allow access if necessary.
- Connection Errors: Verify that the signaling server is running correctly and that the WebRTC connection setup messages are being exchanged properly. Check the browser console for any error messages and debug accordingly.
- UI Not Updating: Ensure that the JavaScript functions handling the UI updates are correctly implemented and that the DOM elements are being manipulated as expected.
Deployment Considerations
Once you have thoroughly tested your application locally, you may want to deploy it to a production environment. Here are some best practices for deploying AIORTC WebRTC applications:
Use a Reverse Proxy
Utilize a reverse proxy server like Nginx to handle incoming requests and forward them to your AIORTC application. This can improve performance, security, and scalability.
Secure Your Application
Implement HTTPS to secure the communication between clients and your server. Obtain an SSL certificate and configure your web server to use HTTPS.
Scalability
Consider using a cloud-based service for deploying your application, such as AWS, Google Cloud, or Azure. These platforms provide scalable infrastructure and additional services that can help manage your application effectively.
Monitoring and Logging
Implement monitoring and logging to keep track of your application's performance and identify any issues. Use tools like Prometheus, Grafana, and ELK Stack for monitoring and logging.
Conclusion
In this article, we have explored how to build a real-time communication application using AIORTC WebRTC. We started with setting up the development environment and installing the necessary dependencies. We then structured our project and implemented key components, including the join screen, control panel, and participant view. Finally, we tested our application to ensure everything works as expected and discussed deployment considerations for a production environment.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ