Introduction to SIP.js WebRTC
What is SIP.js?
SIP.js is a powerful JavaScript library that enables developers to incorporate real-time communications into web applications using the SIP (Session Initiation Protocol) over WebRTC (Web Real-Time Communication). It simplifies the process of setting up and managing WebRTC connections, making it easier for developers to build robust, scalable, and high-quality communication solutions.
The Importance of WebRTC in Modern Web Applications
WebRTC is a groundbreaking technology that allows for real-time audio, video, and data sharing directly between browsers without the need for plugins or external applications. This has revolutionized web communication, enabling features such as video conferencing, voice calls, and file sharing to be integrated seamlessly into web applications.
Benefits of Using SIP.js for WebRTC Signaling
Using SIP.js for WebRTC signaling offers several key advantages:
- Ease of Use: SIP.js abstracts much of the complexity involved in WebRTC signaling, providing a straightforward API for developers.
- Interoperability: It supports a wide range of SIP servers and clients, ensuring that applications built with SIP.js can communicate with other SIP-based systems.
- Flexibility: SIP.js is highly customizable, allowing developers to tailor the signaling process to meet specific application requirements.
- Scalability: The library is designed to handle high volumes of traffic, making it suitable for both small-scale applications and large, enterprise-level deployments.
By leveraging SIP.js, developers can quickly and efficiently integrate WebRTC capabilities into their applications, providing users with a seamless and high-quality communication experience. This introduction serves as the foundation for understanding how to harness the power of SIP.js and WebRTC, setting the stage for the detailed, step-by-step implementation guide that follows.
Getting Started with SIP.js WebRTC
Create a New SIP.js WebRTC App
To kickstart your journey with SIP.js and WebRTC, we'll guide you through creating a new SIP.js WebRTC application from scratch. This section covers setting up the development environment, installing SIP.js, structuring the project, and understanding the app architecture.
Setting Up the Development Environment
Before diving into the code, ensure your development environment is ready. You'll need Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from the official Node.js website.
Once Node.js and npm are installed, create a new directory for your project and navigate into it:
bash
1mkdir sipjs-webrtc-app
2cd sipjs-webrtc-app
3
Installing SIP.js
To use SIP.js in your project, you need to install it via npm. Run the following command to add SIP.js to your project dependencies:
bash
1npm install sip.js
2
This command installs SIP.js and adds it to your project's
package.json
file.Structure of the Project
A well-structured project is crucial for maintainability and scalability. Here's a basic structure for your SIP.js WebRTC app:
1sipjs-webrtc-app/
2├── node_modules/
3├── src/
4│ ├── components/
5│ ├── styles/
6│ ├── App.js
7│ ├── index.js
8├── .gitignore
9├── package.json
10├── README.md
11
src/components/
: This directory will contain your React components.src/styles/
: This directory will store your CSS files.src/App.js
: The main React component that will serve as the entry point for your app.src/index.js
: The JavaScript file that renders your React app to the DOM.
App Architecture
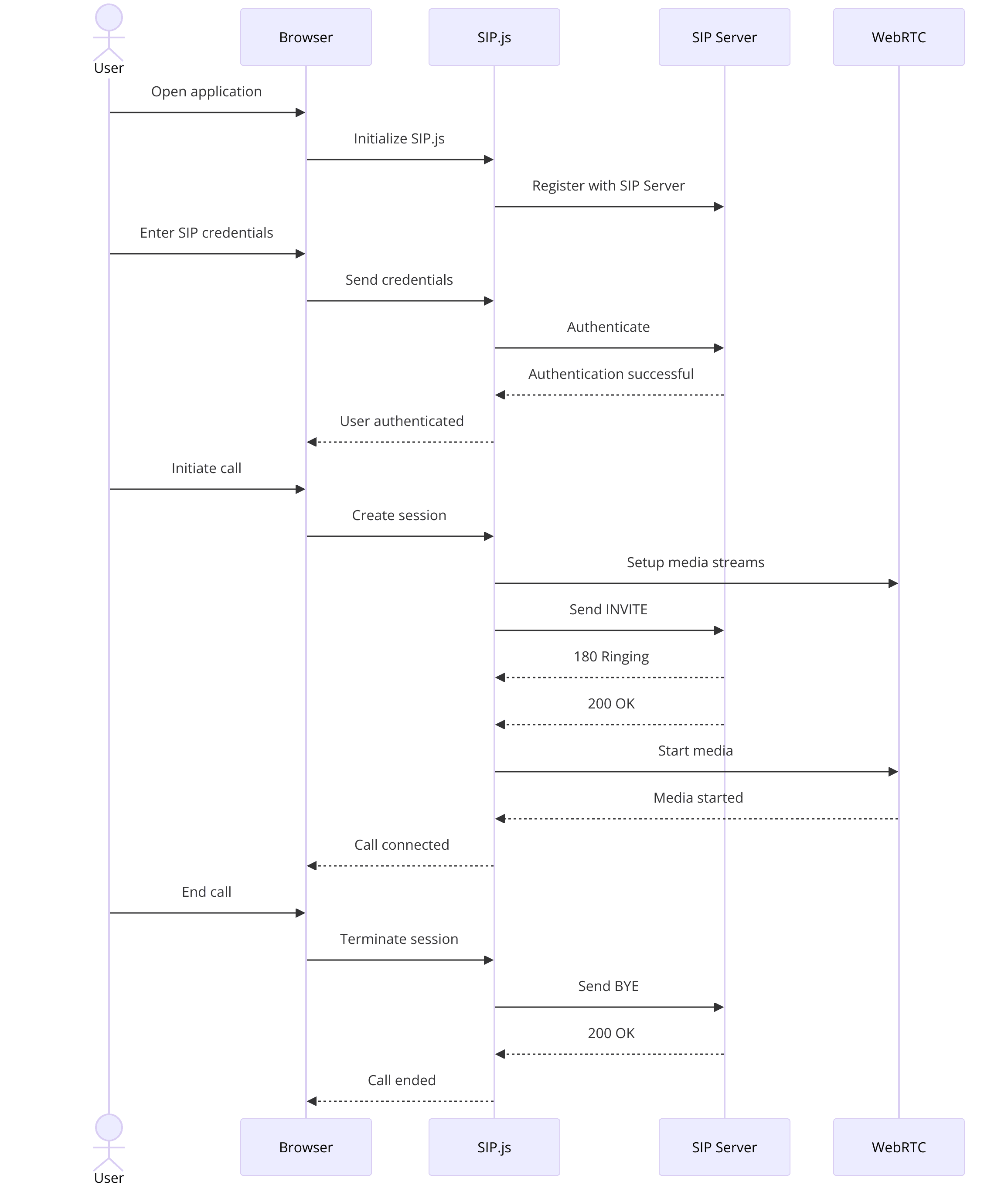
User Interface (UI)
This includes all the visual elements of your app, such as buttons, forms, and displays for audio/video streams. You'll build the UI using React components.
SIP.js Integration
SIP.js handles the signaling part of the WebRTC connection. This involves establishing and managing communication sessions between clients.
WebRTC Media Streams
WebRTC takes care of capturing and transmitting audio and video streams. You'll use the WebRTC API to manage these media streams.
Server-Side Components
While this guide focuses on the client-side, a SIP server (like Asterisk or FreeSWITCH) is required to facilitate the signaling between clients. Ensure you have a SIP server set up and configured properly.
By the end of this section, you should have a new project directory set up with SIP.js installed and a basic project structure in place. This foundation will allow you to build out the rest of your SIP.js WebRTC application with a clear and organized approach. In the next sections, we'll dive into the detailed implementation steps, starting with setting up the basic configuration and getting connected to a SIP server.
Step 1: Get Started with Basic Setup
In this section, we'll walk you through the initial setup for your SIP.js WebRTC application. We'll cover creating the initial project files, configuring basic settings, and connecting to a SIP server. By the end of this step, you'll have a solid foundation to build upon.
Setting Up the Project
First, ensure you are in your project directory:
bash
1cd sipjs-webrtc-app
2
Now, let's create the initial project files. We'll start by setting up the
index.html
, index.js
, and App.js
files.[a] Create index.html
In the
src
directory, create an index.html
file:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>SIP.js WebRTC App</title>
7</head>
8<body>
9 <div id="root"></div>
10 <script src="index.js"></script>
11</body>
12</html>
13
This basic HTML file sets up a
div
with an id
of root
where our React app will be rendered.[b] Create index.js
Next, create an
index.js
file in the src
directory. This file will render our main React component into the root
div:JavaScript
1import React from 'react';
2import ReactDOM from 'react-dom';
3import App from './App';
4
5ReactDOM.render(<App />, document.getElementById('root'));
6
[c] Create App.js
Now, create an
App.js
file in the src
directory. This file will be the main component of our React app:JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3
4function App() {
5 const [userAgent, setUserAgent] = useState(null);
6
7 const handleConnect = () => {
8 const ua = new UA({
9 uri: 'sip:your_username@sip_server.com',
10 transportOptions: {
11 wsServers: ['wss://sip_server.com/ws'],
12 },
13 authorizationUser: 'your_username',
14 password: 'your_password',
15 });
16
17 ua.start();
18 setUserAgent(ua);
19 };
20
21 return (
22 <div>
23 <h1>SIP.js WebRTC App</h1>
24 <button onClick={handleConnect}>Connect</button>
25 </div>
26 );
27}
28
29export default App;
30
Configuring Basic Settings
In the
App.js
file, we have a basic configuration for the SIP.js user agent (UA). Here’s a breakdown of the configuration options:- uri: This is the SIP address of the user. Replace
your_username
with your actual SIP username andsip_server.com
with your SIP server’s domain. - transportOptions: This includes the WebSocket server address used for signaling. Replace
sip_server.com
with your actual SIP server’s domain. - authorizationUser and password: These are your SIP credentials. Replace
your_username
andyour_password
with your actual SIP username and password.
Connecting to a SIP Server
The
handleConnect
function creates a new instance of the SIP.js UA
and starts it. When the user clicks the "Connect" button, this function is called, initiating the connection to the SIP server.Before running the app, make sure you have a SIP server (like Asterisk or FreeSWITCH) set up and configured properly. This server will handle the signaling for WebRTC connections.
Running the Application
To run the application, you need a development server. You can use a tool like
create-react-app
to quickly set up a development server if you haven't already.If you used create-react-app, start the development server with:
bash
1npm start
2
Open your browser and navigate to
http://localhost:3000
. You should see a simple interface with a "Connect" button. Clicking this button will initiate the connection to your SIP server.By following these steps, you have set up the initial configuration for your SIP.js WebRTC application. You created the necessary project files, configured basic settings, and connected to a SIP server. This groundwork prepares you for the subsequent steps, where you'll add more functionality and refine your app. Next, we'll dive into designing the user interface and wiring up all the components.
Step 2: Wireframe All the Components
In this step, we'll focus on designing the user interface (UI) of your SIP.js WebRTC application. A well-structured UI is essential for providing a smooth user experience. We'll create a basic wireframe, identify key components, and structure the HTML layout.
Designing the User Interface
The UI of a SIP.js WebRTC application typically includes elements such as login screens, call controls, and participant views. We'll start by outlining these components and their functions.
Key Components
- Join Screen: Allows users to enter their credentials and connect to the SIP server.
- Call Controls: Provides buttons for initiating, muting, holding, and ending calls.
- Participant View: Displays a list of active participants in the call.
Structuring the HTML Layout
Now, let's translate this wireframe into a structured HTML layout using React components. We'll update the
App.js
file to include placeholders for each component.Update App.js
JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3
4function App() {
5 const [userAgent, setUserAgent] = useState(null);
6
7 const handleConnect = () => {
8 const ua = new UA({
9 uri: `sip:${username}@${server}`,
10 transportOptions: {
11 wsServers: [`wss://${server}/ws`],
12 },
13 authorizationUser: username,
14 password: password,
15 });
16
17 ua.start();
18 setUserAgent(ua);
19 };
20
21 return (
22 <div>
23 <h1>SIP.js WebRTC App</h1>
24 <JoinScreen onConnect={handleConnect} />
25 {userAgent && <CallControls userAgent={userAgent} />}
26 {userAgent && <ParticipantView userAgent={userAgent} />}
27 </div>
28 );
29}
30
31function JoinScreen({ onConnect }) {
32 const [username, setUsername] = useState('');
33 const [password, setPassword] = useState('');
34 const [server, setServer] = useState('');
35
36 return (
37 <div>
38 <h2>Join Screen</h2>
39 <input
40 type="text"
41 placeholder="Username"
42 value={username}
43 onChange={(e) => setUsername(e.target.value)}
44 />
45 <input
46 type="password"
47 placeholder="Password"
48 value={password}
49 onChange={(e) => setPassword(e.target.value)}
50 />
51 <input
52 type="text"
53 placeholder="Server"
54 value={server}
55 onChange={(e) => setServer(e.target.value)}
56 />
57 <button onClick={() => onConnect(username, password, server)}>Connect</button>
58 </div>
59 );
60}
61
62function CallControls({ userAgent }) {
63 return (
64 <div>
65 <h2>Call Controls</h2>
66 <button onClick={() => userAgent.invite('sip:callee@sip_server.com')}>Start Call</button>
67 <button onClick={() => userAgent.mute()}>Mute</button>
68 <button onClick={() => userAgent.hold()}>Hold</button>
69 <button onClick={() => userAgent.terminateSessions()}>End Call</button>
70 </div>
71 );
72}
73
74function ParticipantView({ userAgent }) {
75 return (
76 <div>
77 <h2>Participant View</h2>
78 <ul>
79 {userAgent.sessions.map((session, index) => (
80 <li key={index}>{session.remoteIdentity.displayName || session.remoteIdentity.uri.toString()}</li>
81 ))}
82 </ul>
83 </div>
84 );
85}
86
87export default App;
88
Explanation of the Code
JoinScreen
Component: This component includes input fields for username, password, and server, and a "Connect" button to initiate the connection to the SIP server.CallControls
Component: This component provides buttons to start a call, mute, hold, and end the call. TheuserAgent
object is used to control the call actions.ParticipantView
Component: This component displays the list of active participants in the call. It maps over theuserAgent.sessions
array to display each participant.
By structuring your application in this way, you separate concerns and make your code more modular and maintainable.
In this section, you designed the UI of your SIP.js WebRTC application by creating a basic wireframe and structuring the HTML layout using React components. This sets the stage for implementing the join screen, call controls, and participant view in subsequent steps. Next, we'll dive deeper into implementing the join screen functionality.
Step 3: Implement Join Screen
In this step, we will focus on implementing the join screen, which allows users to enter their credentials and connect to the SIP server. This involves designing the interface, handling user inputs, and validating user credentials.
Creating the Join Screen
The join screen is the first interaction point for users. It collects necessary information such as username, password, and server address, and initiates the connection to the SIP server.
Update the JoinScreen
Component
We will enhance the
JoinScreen
component to handle user input and connect to the SIP server upon clicking the "Connect" button.Updated
JoinScreen
Component:JavaScript
1import React, { useState } from 'react';
2
3function JoinScreen({ onConnect }) {
4 const [username, setUsername] = useState('');
5 const [password, setPassword] = useState('');
6 const [server, setServer] = useState('');
7 const [error, setError] = useState('');
8
9 const handleConnect = () => {
10 if (!username || !password || !server) {
11 setError('All fields are required');
12 return;
13 }
14 setError('');
15 onConnect(username, password, server);
16 };
17
18 return (
19 <div>
20 <h2>Join Screen</h2>
21 {error && <p style={{ color: 'red' }}>{error}</p>}
22 <input
23 type="text"
24 placeholder="Username"
25 value={username}
26 onChange={(e) => setUsername(e.target.value)}
27 />
28 <input
29 type="password"
30 placeholder="Password"
31 value={password}
32 onChange={(e) => setPassword(e.target.value)}
33 />
34 <input
35 type="text"
36 placeholder="Server"
37 value={server}
38 onChange={(e) => setServer(e.target.value)}
39 />
40 <button onClick={handleConnect}>Connect</button>
41 </div>
42 );
43}
44
45export default JoinScreen;
46
Explanation
- State Management: We use React's
useState
hook to manage the input values (username
,password
,server
) and any error messages. - Input Fields: The component includes three input fields for the username, password, and server. Each input updates its corresponding state.
- Error Handling: If any input field is empty when the user tries to connect, an error message is displayed.
- Handle Connect: The
handleConnect
function validates the inputs and invokes theonConnect
callback with the input values if validation passes.
Update the App.js
to Handle Connection Logic
We need to modify the
App.js
file to handle the connection logic when the user submits their credentials.Updated
App.js
:JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3import JoinScreen from './components/JoinScreen';
4import CallControls from './components/CallControls';
5import ParticipantView from './components/ParticipantView';
6
7function App() {
8 const [userAgent, setUserAgent] = useState(null);
9
10 const handleConnect = (username, password, server) => {
11 const ua = new UA({
12 uri: `sip:${username}@${server}`,
13 transportOptions: {
14 wsServers: [`wss://${server}/ws`],
15 },
16 authorizationUser: username,
17 password: password,
18 });
19
20 ua.start();
21 setUserAgent(ua);
22 };
23
24 return (
25 <div>
26 <h1>SIP.js WebRTC App</h1>
27 {!userAgent && <JoinScreen onConnect={handleConnect} />}
28 {userAgent && <CallControls userAgent={userAgent} />}
29 {userAgent && <ParticipantView userAgent={userAgent} />}
30 </div>
31 );
32}
33
34export default App;
35
Explanation
- State Management: The
userAgent
state holds the instance of the SIP.js user agent. - Handle Connect: The
handleConnect
function creates and starts a newUA
instance with the provided credentials and server address. It then updates theuserAgent
state with the new instance. - Conditional Rendering: The
JoinScreen
component is displayed only ifuserAgent
isnull
. Once connected, theCallControls
andParticipantView
components are rendered.
Styling the Join Screen
To improve the user experience, we'll add some basic styles to the join screen. Create a
styles.css
file in the src/styles
directory and add the following CSS:CSS
1/* src/styles/styles.css */
2body {
3 font-family: Arial, sans-serif;
4 background-color: #f0f0f0;
5 display: flex;
6 justify-content: center;
7 align-items: center;
8 height: 100vh;
9 margin: 0;
10}
11
12div {
13 background-color: #fff;
14 padding: 20px;
15 border-radius: 8px;
16 box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
17 width: 300px;
18}
19
20input {
21 display: block;
22 width: 100%;
23 margin-bottom: 10px;
24 padding: 8px;
25 border: 1px solid #ccc;
26 border-radius: 4px;
27}
28
29button {
30 width: 100%;
31 padding: 10px;
32 background-color: #007bff;
33 color: #fff;
34 border: none;
35 border-radius: 4px;
36 cursor: pointer;
37}
38
39button:hover {
40 background-color: #0056b3;
41}
42
Import the CSS file in your
index.js
:JavaScript
1import './styles/styles.css';
2
In this step, we implemented the join screen for our SIP.js WebRTC application. We designed the user interface, handled user inputs, validated credentials, and connected to the SIP server. This join screen serves as the entry point for users to access the application's real-time communication features. In the next steps, we will implement the call controls and participant view to enhance the application's functionality.
Step 4: Implement Controls
In this step, we'll implement the call controls for your SIP.js WebRTC application. These controls will allow users to start calls, mute, hold, and end calls. This section involves designing the interface for the controls, writing the logic for call actions, and integrating these functionalities into the application.
Adding Call Controls
The call controls are essential for managing the state of the call and providing users with necessary actions to handle their communication effectively.
[a] Create CallControls
Component
First, let's create a new
CallControls
component. This component will include buttons for starting a call, muting, holding, and ending a call.Create
CallControls.js
:JavaScript
1import React from 'react';
2
3function CallControls({ userAgent }) {
4 const handleStartCall = () => {
5 const target = prompt('Enter the SIP address of the callee:');
6 if (target) {
7 userAgent.invite(target);
8 }
9 };
10
11 const handleMute = () => {
12 userAgent.getSession().mute();
13 };
14
15 const handleHold = () => {
16 userAgent.getSession().hold();
17 };
18
19 const handleEndCall = () => {
20 userAgent.getSession().terminate();
21 };
22
23 return (
24 <div>
25 <h2>Call Controls</h2>
26 <button onClick={handleStartCall}>Start Call</button>
27 <button onClick={handleMute}>Mute</button>
28 <button onClick={handleHold}>Hold</button>
29 <button onClick={handleEndCall}>End Call</button>
30 </div>
31 );
32}
33
34export default CallControls;
35
Explanation
- handleStartCall: Prompts the user to enter the SIP address of the callee and initiates a call using
userAgent.invite()
. - handleMute: Mutes the current session using
userAgent.getSession().mute()
. - handleHold: Puts the current session on hold using
userAgent.getSession().hold()
. - handleEndCall: Terminates the current session using
userAgent.getSession().terminate()
.
[b] Integrate CallControls
Component
Now, we need to integrate the
CallControls
component into the main application. Update the App.js
file to include this component.Updated
App.js
:JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3import JoinScreen from './components/JoinScreen';
4import CallControls from './components/CallControls';
5import ParticipantView from './components/ParticipantView';
6
7function App() {
8 const [userAgent, setUserAgent] = useState(null);
9
10 const handleConnect = (username, password, server) => {
11 const ua = new UA({
12 uri: `sip:${username}@${server}`,
13 transportOptions: {
14 wsServers: [`wss://${server}/ws`],
15 },
16 authorizationUser: username,
17 password: password,
18 });
19
20 ua.start();
21 setUserAgent(ua);
22 };
23
24 return (
25 <div>
26 <h1>SIP.js WebRTC App</h1>
27 {!userAgent && <JoinScreen onConnect={handleConnect} />}
28 {userAgent && <CallControls userAgent={userAgent} />}
29 {userAgent && <ParticipantView userAgent={userAgent} />}
30 </div>
31 );
32}
33
34export default App;
35
Explanation
Conditional Rendering: The
CallControls
component is rendered only if the userAgent
is not null
.[c] Enhance Call Control Functionalities
To handle the call actions correctly, we need to ensure the
userAgent
has an active session. We will add session management in the App.js
.Update
App.js
for Session Management:JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3import JoinScreen from './components/JoinScreen';
4import CallControls from './components/CallControls';
5import ParticipantView from './components/ParticipantView';
6
7function App() {
8 const [userAgent, setUserAgent] = useState(null);
9 const [session, setSession] = useState(null);
10
11 const handleConnect = (username, password, server) => {
12 const ua = new UA({
13 uri: `sip:${username}@${server}`,
14 transportOptions: {
15 wsServers: [`wss://${server}/ws`],
16 },
17 authorizationUser: username,
18 password: password,
19 });
20
21 ua.start();
22 ua.on('invite', (incomingSession) => {
23 incomingSession.accept();
24 setSession(incomingSession);
25 });
26 setUserAgent(ua);
27 };
28
29 const handleStartCall = (target) => {
30 const outgoingSession = userAgent.invite(target);
31 setSession(outgoingSession);
32 };
33
34 const handleMute = () => {
35 if (session) {
36 session.mute();
37 }
38 };
39
40 const handleHold = () => {
41 if (session) {
42 session.hold();
43 }
44 };
45
46 const handleEndCall = () => {
47 if (session) {
48 session.terminate();
49 setSession(null);
50 }
51 };
52
53 return (
54 <div>
55 <h1>SIP.js WebRTC App</h1>
56 {!userAgent && <JoinScreen onConnect={handleConnect} />}
57 {userAgent && (
58 <CallControls
59 onStartCall={handleStartCall}
60 onMute={handleMute}
61 onHold={handleHold}
62 onEndCall={handleEndCall}
63 />
64 )}
65 {userAgent && <ParticipantView userAgent={userAgent} />}
66 </div>
67 );
68}
69
70export default App;
71
Explanation:
- Session Management: The
session
state is used to manage the current SIP session. - Event Handling: The
userAgent
listens for incoming calls and accepts them automatically, setting the session state. - Updated Call Control Functions: The call control functions now check for the existence of a session before performing actions.
[d] Update CallControls
Component for New Props
Update the
CallControls
component to use the new props for handling call actions.Updated
CallControls.js
:JavaScript
1import React from 'react';
2
3function CallControls({ onStartCall, onMute, onHold, onEndCall }) {
4 const handleStartCall = () => {
5 const target = prompt('Enter the SIP address of the callee:');
6 if (target) {
7 onStartCall(target);
8 }
9 };
10
11 return (
12 <div>
13 <h2>Call Controls</h2>
14 <button onClick={handleStartCall}>Start Call</button>
15 <button onClick={onMute}>Mute</button>
16 <button onClick={onHold}>Hold</button>
17 <button onClick={onEndCall}>End Call</button>
18 </div>
19 );
20}
21
22export default CallControls;
23
Explanation:
Prop Handling: The component now uses props (
onStartCall
, onMute
, onHold
, onEndCall
) to handle call actions, ensuring the main logic resides in App.js
.In this step, we implemented the call controls for the SIP.js WebRTC application. We created a
CallControls
component, integrated it into the main application, and ensured proper session management for handling call actions. These controls allow users to start, mute, hold, and end calls, enhancing the application's functionality and user experience. In the next step, we will focus on implementing the participant view to display active call participants.Step 5: Implement Participant View
In this step, we'll implement the participant view for your SIP.js WebRTC application. This feature will display a list of active participants in the call, providing a clear overview of who is currently connected. We will update the
ParticipantView
component to reflect this functionality.Displaying Call Participants
The participant view will dynamically update to show the current call participants. We need to ensure that our application listens for changes in the session state and updates the participant list accordingly.
[a] Update ParticipantView
Component
First, we'll create a
ParticipantView
component that lists the active participants in the call.Create
ParticipantView.js
:JavaScript
1import React, { useEffect, useState } from 'react';
2
3function ParticipantView({ session }) {
4 const [participants, setParticipants] = useState([]);
5
6 useEffect(() => {
7 if (session) {
8 const updateParticipants = () => {
9 const newParticipants = [];
10 if (session.remoteIdentity.displayName) {
11 newParticipants.push(session.remoteIdentity.displayName);
12 } else {
13 newParticipants.push(session.remoteIdentity.uri.toString());
14 }
15 setParticipants(newParticipants);
16 };
17
18 updateParticipants();
19
20 session.on('accepted', updateParticipants);
21 session.on('bye', () => setParticipants([]));
22 session.on('terminated', () => setParticipants([]));
23
24 return () => {
25 session.off('accepted', updateParticipants);
26 session.off('bye', () => setParticipants([]));
27 session.off('terminated', () => setParticipants([]));
28 };
29 }
30 }, [session]);
31
32 return (
33 <div>
34 <h2>Participant View</h2>
35 <ul>
36 {participants.map((participant, index) => (
37 <li key={index}>{participant}</li>
38 ))}
39 </ul>
40 </div>
41 );
42}
43
44export default ParticipantView;
45
Explanation:
- State Management: We use the
useState
hook to manage the list of participants. - Effect Hook: The
useEffect
hook listens for changes in the session and updates the participant list accordingly. - Event Handling: The component listens for
accepted
,bye
, andterminated
events to update the participant list.
[b] Integrate ParticipantView
Component
Next, we'll integrate the
ParticipantView
component into the main application. We need to pass the current session to the ParticipantView
component.Updated
App.js
:JavaScript
1import React, { useState } from 'react';
2import { UA } from 'sip.js';
3import JoinScreen from './components/JoinScreen';
4import CallControls from './components/CallControls';
5import ParticipantView from './components/ParticipantView';
6
7function App() {
8 const [userAgent, setUserAgent] = useState(null);
9 const [session, setSession] = useState(null);
10
11 const handleConnect = (username, password, server) => {
12 const ua = new UA({
13 uri: `sip:${username}@${server}`,
14 transportOptions: {
15 wsServers: [`wss://${server}/ws`],
16 },
17 authorizationUser: username,
18 password: password,
19 });
20
21 ua.start();
22 ua.on('invite', (incomingSession) => {
23 incomingSession.accept();
24 setSession(incomingSession);
25 });
26 setUserAgent(ua);
27 };
28
29 const handleStartCall = (target) => {
30 const outgoingSession = userAgent.invite(target);
31 setSession(outgoingSession);
32 };
33
34 const handleMute = () => {
35 if (session) {
36 session.mute();
37 }
38 };
39
40 const handleHold = () => {
41 if (session) {
42 session.hold();
43 }
44 };
45
46 const handleEndCall = () => {
47 if (session) {
48 session.terminate();
49 setSession(null);
50 }
51 };
52
53 return (
54 <div>
55 <h1>SIP.js WebRTC App</h1>
56 {!userAgent && <JoinScreen onConnect={handleConnect} />}
57 {userAgent && (
58 <CallControls
59 onStartCall={handleStartCall}
60 onMute={handleMute}
61 onHold={handleHold}
62 onEndCall={handleEndCall}
63 />
64 )}
65 {session && <ParticipantView session={session} />}
66 </div>
67 );
68}
69
70export default App;
71
Explanation:
- Session Prop: The
session
state is passed to theParticipantView
component, allowing it to display the active participants. - Conditional Rendering: The
ParticipantView
component is rendered only if there is an active session.
[c] Enhancing Participant Management
For a more comprehensive participant view, you might want to display more details or manage multiple participants if your application supports group calls. The basic implementation can be extended as needed to suit your application's requirements.
Extended
ParticipantView
for Group Calls:If your application supports group calls, you might need to handle multiple participants. Here's an example extension for the
ParticipantView
component:JavaScript
1import React, { useEffect, useState } from 'react';
2
3function ParticipantView({ session }) {
4 const [participants, setParticipants] = useState([]);
5
6 useEffect(() => {
7 if (session) {
8 const updateParticipants = () => {
9 const newParticipants = session.dialogs.map(dialog => dialog.remoteIdentity.displayName || dialog.remoteIdentity.uri.toString());
10 setParticipants(newParticipants);
11 };
12
13 updateParticipants();
14
15 session.on('progress', updateParticipants);
16 session.on('accepted', updateParticipants);
17 session.on('bye', updateParticipants);
18 session.on('terminated', updateParticipants);
19
20 return () => {
21 session.off('progress', updateParticipants);
22 session.off('accepted', updateParticipants);
23 session.off('bye', updateParticipants);
24 session.off('terminated', updateParticipants);
25 };
26 }
27 }, [session]);
28
29 return (
30 <div>
31 <h2>Participant View</h2>
32 <ul>
33 {participants.map((participant, index) => (
34 <li key={index}>{participant}</li>
35 ))}
36 </ul>
37 </div>
38 );
39}
40
41export default ParticipantView;
42
Explanation:
- Group Calls: The updated
ParticipantView
handles multiple participants by mapping over the session dialogs. - Event Handling: The component listens for additional events (
progress
,accepted
,bye
,terminated
) to keep the participant list updated.
In this step, we implemented the participant view for the SIP.js WebRTC application. This component dynamically displays the list of active participants in a call, providing users with clear visibility of who is connected. We ensured that the participant view updates in real-time based on session events. This enhancement improves the overall user experience by keeping them informed about the current call participants. In the next step, we will focus on running and testing the application to ensure everything works as expected.
Step 6: Run Your Code Now
In this final step, we will focus on running and testing your SIP.js WebRTC application to ensure everything works as expected. We'll cover how to start the application, test its functionality, and troubleshoot common issues. This section will also include some tips for preparing your app for production deployment.
Running the Application
To run your application, you'll need to start your development server. If you used a tool like
create-react-app
to set up your project, you can use the following command:bash
1npm start
2
This command will start the development server and open your application in the default web browser. The application should be accessible at
http://localhost:3000
.Testing the Application
Once your application is running, you can test its functionality by following these steps:
Open the Application
Navigate to
http://localhost:3000
in your web browser.Connect to SIP Server
- Enter your SIP username, password, and server address in the Join Screen.
- Click the "Connect" button.
- If the connection is successful, the call controls and participant view should appear.
Test Call Controls
- Start a Call: Click the "Start Call" button and enter the SIP address of the callee. Verify that the call is initiated.
- Mute: Click the "Mute" button to mute the call. Verify that the call is muted.
- Hold: Click the "Hold" button to put the call on hold. Verify that the call is on hold.
- End Call: Click the "End Call" button to terminate the call. Verify that the call is ended.
Check Participant View
- Ensure that the participant view displays the active call participants.
- Verify that the participant list updates dynamically based on the call status.
Troubleshooting Common Issues
If you encounter any issues while running your application, here are some common troubleshooting tips:
Connection Issues
- Ensure that your SIP server is running and accessible.
- Verify the SIP username, password, and server address are correct.
- Check for any network issues or firewall settings that might be blocking the connection.
Call Controls Not Working
- Ensure that the
session
object is properly initialized and available. - Check the console for any errors related to SIP.js or WebRTC.
Participant View Not Updating
- Verify that the event listeners are correctly set up in the
ParticipantView
component. - Ensure that the session events (
accepted
,bye
,terminated
) are being triggered correctly.
Preparing for Production Deployment
Once you have tested your application and ensured it works as expected, you can prepare it for production deployment. Here are some steps to consider:
Build the Application
Use the following command to create a production build of your application:
bash
1 npm run build
2
This will generate optimized static files for deployment.
Configure SIP Server
- Ensure that your SIP server is properly configured and secured for production use.
- Consider using a reliable SIP provider to handle production traffic.
Deploy the Application
- Deploy the built files to a web server or a cloud service provider like AWS, Google Cloud, or Azure.
- Ensure that your deployment environment supports WebSocket connections required for SIP.js.
Monitor and Maintain
- Set up monitoring and logging to track the performance and usage of your application.
- Regularly update dependencies and security patches to keep your application secure.
Conclusion
By following this guide, you have successfully built and tested a SIP.js WebRTC application. You have learned how to create a join screen, implement call controls, and display call participants. With these features in place, your application is ready for production deployment.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ