Introduction: Harnessing the Power of Google's Real-Time Speech-to-Text
What is Google Real-Time Speech-to-Text?
Google Real-Time Speech-to-Text is a powerful service that leverages Google's advanced machine learning models to convert spoken audio into written text with minimal latency. It's part of the Google Cloud Speech-to-Text API, offering developers the ability to integrate accurate and efficient speech recognition capabilities into their applications. This technology transcends simple dictation; it allows for true real-time transcription of conversations, lectures, and other audio streams.
The Importance of Real-Time Transcription
Real-time transcription is revolutionizing various industries. It enables immediate accessibility for individuals with hearing impairments through live captioning, enhances productivity in meetings and conferences with instant transcripts, and facilitates more efficient communication in call centers with automated transcript analysis. The ability to quickly and accurately convert speech to text unlocks a wealth of possibilities, from improved user experiences to data-driven insights.
Applications of Google's Real-Time Speech-to-Text
The applications of Google's Real-Time Speech-to-Text are vast and varied. Some key use cases include:
- Live Captioning: Providing real-time captions for video conferencing, webinars, and live events.
- Meeting Transcription: Automatically transcribing meeting discussions for record-keeping and follow-up actions.
- Call Center Analytics: Analyzing call transcripts to identify trends, improve customer service, and ensure compliance.
- Voice Search and Control: Enabling voice-activated search and control in applications and devices.
- Dictation and Note-Taking: Assisting users with dictation tasks and real-time note-taking.
- Accessibility: Providing real-time transcription for individuals with hearing impairments.
Understanding the Google Cloud Speech-to-Text API
The Google Cloud Speech-to-Text API is the backbone of Google's real-time speech recognition capabilities. It offers a robust and scalable platform for converting audio data into text.
Key Features and Capabilities
The API boasts a rich set of features, including:
- Real-Time and Asynchronous Recognition: Supports both real-time (streaming) and asynchronous (batch) transcription.
- Language Support: Extensive language support, covering numerous dialects and accents.
- Custom Vocabulary: Ability to customize the vocabulary to improve accuracy for specific domains or industries.
- Noise Reduction: Advanced noise reduction algorithms to handle challenging audio environments.
- Word-Level Timestamps: Provides timestamps for each word in the transcript, enabling synchronization with audio and video.
- Speaker Diarization: Identifies different speakers in an audio stream.
- Automatic Punctuation: Automatically adds punctuation to the transcribed text.
API Architecture and Workflow
The API operates through a client-server architecture. Your application sends audio data to the Google Cloud Speech-to-Text service, which processes the audio and returns a transcript. For real-time transcription, a streaming connection is established, allowing for continuous audio input and immediate transcript output.
Here is a Mermaid diagram describing the workflow:
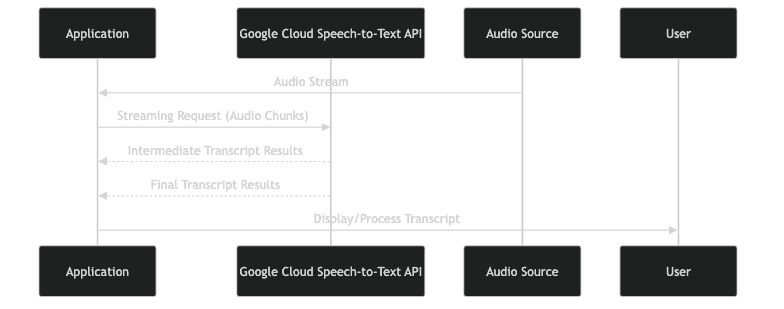
Choosing the Right Model for Your Needs
The Google Cloud Speech-to-Text API offers various models optimized for different use cases. For example, there are models specifically trained for phone calls, video content, and command-and-control applications. Selecting the appropriate model can significantly impact accuracy and performance. Consider the characteristics of your audio data (e.g., background noise, language, accent) when choosing a model. Also consider the asynchronous vs synchronous use case. Asynchronous is best if you don't need immediate transcription.
Setting Up and Using the Google Cloud Speech-to-Text API
Before you can start using the Google Cloud Speech-to-Text API, you need to set up a Google Cloud project and configure your environment.
Prerequisites and Account Setup
- Google Cloud Account: You'll need a Google Cloud account. If you don't have one, you can sign up for a free trial.
- Billing Account: Enable billing for your Google Cloud project. The Speech-to-Text API is a paid service, but Google offers a free tier for limited usage.
Creating a Project and Enabling the API
- Create a Google Cloud Project: In the Google Cloud Console, create a new project.
- Enable the Speech-to-Text API: Navigate to the API Library and enable the Cloud Speech-to-Text API for your project.
Authentication and Authorization
To access the API, you need to authenticate your application using a service account. A service account is a special type of Google account that represents your application rather than a user.
- Create a Service Account: In the Google Cloud Console, create a service account with the "Cloud Speech-to-Text API" role.
- Download a Service Account Key: Download the service account key as a JSON file. This file contains the credentials that your application will use to authenticate with the API.
1import os
2from google.oauth2 import service_account
3
4# Path to your service account key file
5key_path = 'path/to/your/service_account_key.json'
6
7# Set the GOOGLE_APPLICATION_CREDENTIALS environment variable
8os.environ['GOOGLE_APPLICATION_CREDENTIALS'] = key_path
9
10# Create credentials from the service account key
11credentials = service_account.Credentials.from_service_account_file(key_path)
12
13print("Authentication successful!")
14
Installing and Utilizing Client Libraries
Google provides client libraries for various programming languages, making it easier to interact with the Speech-to-Text API. These libraries handle the complexities of API requests and responses, allowing you to focus on your application logic.
1from google.cloud import speech
2
3# Instantiates a client
4client = speech.SpeechClient()
5
6# The name of the audio file to transcribe
7audio_file = 'path/to/your/audio.raw'
8
9with open(audio_file, 'rb') as f:
10 content = f.read()
11
12# In practice this should be chunked for long audio
13audio = speech.RecognitionAudio(content=content)
14
15config = speech.RecognitionConfig(
16 encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
17 sample_rate_hertz=16000,
18 language_code='en-US',
19)
20
21# Detects speech in the audio file
22response = client.recognize(config=config, audio=audio)
23
24for result in response.results:
25 print('Transcript: {}'.format(result.alternatives[0].transcript))
26
Real-Time Transcription with the Google Cloud Speech-to-Text API
Real-time transcription, also known as streaming recognition, allows you to transcribe audio as it's being recorded. This is ideal for applications like live captioning and real-time meeting transcription.
Streaming Recognition Explained
In streaming recognition, the audio is sent to the API in chunks, rather than as a single file. The API returns intermediate results as it processes the audio, and a final result when it has finished processing a chunk. This allows you to display the transcript in near real-time.
Implementing Streaming Recognition with the API
Implementing streaming recognition involves establishing a bidirectional stream with the Speech-to-Text API. Your application sends audio data to the stream, and the API returns transcription results through the same stream.
1import io
2import os
3
4from google.cloud import speech
5
6def transcribe_streaming(stream_file):
7 """Streams transcription of the given audio file."""
8 client = speech.SpeechClient()
9
10 with io.open(stream_file, "rb") as audio_file:
11 content = audio_file.read()
12
13 # In practice, stream should be a generator yielding chunks of audio data
14 audio = speech.RecognitionAudio(content=content)
15 config = speech.RecognitionConfig(
16 encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
17 sample_rate_hertz=16000,
18 language_code="en-US",
19 )
20
21 streaming_config = speech.StreamingRecognitionConfig(config=config, interim_results=True)
22
23 requests = (speech.StreamingRecognizeRequest(audio_content=chunk) for chunk in [content])
24
25 responses = client.streaming_recognize(config=streaming_config, requests=requests)
26
27 # Now, put the transcription responses to use.
28 for response in responses:
29 # Once the transcription is complete, the result contains the
30 # is_final result.
31 for result in response.results:
32 # The first alternative is the most likely one.
33 alternative = result.alternatives[0]
34 print(f"Transcript: {alternative.transcript}")
35
36
37
38
39
Handling Intermediary and Final Results
When using streaming recognition, the API returns both intermediary and final results. Intermediary results are preliminary transcripts that may change as the API processes more audio. Final results are the confirmed transcripts for a given segment of audio. Your application should display intermediary results to provide users with immediate feedback, and update the display with final results as they become available.
interim_results=True
is important for streaming recognition to work.Advanced Features and Customization
The Google Cloud Speech-to-Text API offers several advanced features and customization options to enhance accuracy and tailor the API to your specific needs.
Language Support and Customization
The API supports a wide range of languages and dialects. You can specify the language code when making a request to ensure accurate transcription. Additionally, you can create custom vocabulary to improve recognition of specific words or phrases that are common in your domain.
Model Selection and Optimization
As mentioned earlier, the API offers different models optimized for various use cases. Choosing the appropriate model can significantly improve accuracy. You can also optimize the API's performance by adjusting parameters such as the audio encoding and sample rate.
Handling Noise and Background Sounds
Noisy audio environments can significantly impact transcription accuracy. The Speech-to-Text API includes noise reduction algorithms to mitigate the effects of background noise. You can also use external noise reduction tools to pre-process the audio before sending it to the API.
Improving Accuracy and Performance
To improve accuracy and performance, consider the following tips:
- Use High-Quality Audio: Ensure that the audio input is clear and free from distortion.
- Select the Appropriate Model: Choose the model that best matches the characteristics of your audio data.
- Customize the Vocabulary: Create a custom vocabulary to improve recognition of domain-specific terms.
- Optimize Audio Encoding: Experiment with different audio encodings and sample rates to find the optimal settings for your audio data.
- Implement Error Handling: Implement robust error handling to gracefully handle API errors and network issues.
Comparing Google Cloud Speech-to-Text with Other Solutions
While Google Cloud Speech-to-Text is a leading solution, several other speech recognition APIs and services are available.
Key Competitors and Their Strengths
- Amazon Transcribe: Another cloud-based speech recognition service with similar features and capabilities.
- Microsoft Azure Speech to Text: Part of the Azure Cognitive Services suite, offering speech recognition and other AI capabilities.
- IBM Watson Speech to Text: A powerful speech recognition service with advanced customization options.
Feature Comparison Table
Feature | Google Cloud Speech-to-Text | Amazon Transcribe | Microsoft Azure Speech to Text | IBM Watson Speech to Text |
---|---|---|---|---|
Real-Time | Yes | Yes | Yes | Yes |
Asynchronous | Yes | Yes | Yes | Yes |
Language Support | Extensive | Extensive | Extensive | Extensive |
Custom Vocabulary | Yes | Yes | Yes | Yes |
Speaker Diarization | Yes | Yes | Yes | Yes |
Pricing | Competitive | Competitive | Competitive | Competitive |
Real-World Applications and Case Studies
The Google Cloud Speech-to-Text API is being used in various industries to solve real-world problems.
Examples in Different Industries
- Healthcare: Transcribing doctor-patient conversations for medical records.
- Media and Entertainment: Providing live captions for broadcast TV and streaming services.
- Education: Transcribing lectures for students with hearing impairments.
- Finance: Analyzing call center conversations to identify fraud and improve customer service.
Success Stories and Testimonials
Many organizations have reported significant benefits from using the Google Cloud Speech-to-Text API, including improved accuracy, reduced costs, and enhanced efficiency.
Troubleshooting and Best Practices
Like any technology, the Google Cloud Speech-to-Text API can encounter issues. Here's how to solve them and some best practices to follow.
Common Issues and Solutions
- Authentication Errors: Verify that your service account key is valid and that the
GOOGLE_APPLICATION_CREDENTIALS
environment variable is set correctly. - API Rate Limits: Be mindful of the API rate limits and implement appropriate retry mechanisms.
- Transcription Errors: Improve accuracy by using high-quality audio, selecting the appropriate model, and customizing the vocabulary.
Tips for Optimizing Performance
- Use a CDN: Use a content delivery network (CDN) to cache API responses and reduce latency.
- Optimize Audio Compression: Compress audio data to reduce bandwidth consumption.
- Implement Caching: Cache transcription results to avoid unnecessary API calls.
Conclusion: The Future of Google Real-Time Speech-to-Text
Google Real-Time Speech-to-Text is a transformative technology with the potential to revolutionize various industries. As speech recognition technology continues to evolve, we can expect even more accurate, efficient, and versatile applications of this powerful API. The future looks bright for speech-to-text, offering endless possibilities for innovation and accessibility.
Google Cloud Speech-to-Text Documentation
: "Learn more about the Google Cloud Speech-to-Text API"Google Cloud Pricing
: "Check out the pricing details for Google Cloud Speech-to-Text"A Relevant Blog Post or Article on Real-Time Transcription
: "Discover additional insights into real-time transcription from this expert article"
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ