What is WebRTC Video Conferencing?
Introduction to WebRTC
WebRTC (Web Real-Time Communication) is a free, open-source project providing web browsers and mobile applications with real-time communication (RTC) via simple APIs. WebRTC video conferencing leverages these APIs to enable browser-based video calls without the need for plugins or downloads, offering a powerful and flexible platform for real-time communication.
Key Features and Benefits of WebRTC
WebRTC boasts several key features that make it an attractive choice for developers:
- Real-Time Communication: Enables low-latency, high-quality video and audio streaming.
- Browser-Based: Operates directly within web browsers, eliminating the need for plugins.
- Open Source: Free to use and modify, fostering innovation and community development.
- Cross-Platform: Supports various operating systems and devices.
- Secure: Incorporates built-in encryption for secure communication.
How WebRTC Enables Real-Time Communication
WebRTC empowers real-time communication by providing the necessary components for audio and video capture, encoding, and transmission directly within the browser. It handles the complexities of network traversal and media encoding, allowing developers to focus on building their applications rather than dealing with low-level networking details. By leveraging protocols like UDP, TCP, and DTLS, WebRTC establishes secure and efficient peer-to-peer connections for seamless video conferencing experiences.
Architecture and Components of WebRTC Video Conferencing
WebRTC video conferencing relies on a specific architecture and a few key components to function effectively. These components work together to establish and maintain real-time connections between peers.
Signaling Servers
Signaling servers are crucial for WebRTC. They handle the initial negotiation between peers before a direct connection can be established. This negotiation includes exchanging information about media capabilities (codecs, resolutions), network information (IP addresses, ports), and other parameters necessary for setting up the peer-to-peer connection. Signaling is not standardized within WebRTC; developers are free to use any protocol they prefer (e.g., WebSocket, SIP, custom HTTP APIs).
STUN and TURN Servers
STUN (Session Traversal Utilities for NAT) and TURN (Traversal Using Relays around NAT) servers play a vital role in overcoming Network Address Translation (NAT) and firewall restrictions. STUN servers help peers discover their public IP addresses, while TURN servers act as relays when direct peer-to-peer connections are impossible due to network configurations.
ICE Candidate Negotiation
ICE (Interactive Connectivity Establishment) is a framework that WebRTC uses to find the best possible path for communication between peers. During ICE candidate negotiation, each peer gathers potential connection candidates (combinations of IP addresses and ports) and exchanges them with the other peer. These candidates are then tested to determine the optimal route.
JavaScript
1// Example of gathering ICE candidates
2peerConnection.onicecandidate = (event) => {
3 if (event.candidate) {
4 console.log('ICE candidate:', event.candidate);
5 // Send the candidate to the other peer via the signaling server
6 }
7};
8
Peer-to-Peer Connections
Once the ICE negotiation is complete, a peer-to-peer connection is established directly between the participants. This connection allows for low-latency, high-bandwidth communication without the need for intermediaries (unless a TURN server is used as a relay).
Media Streams and Codecs
WebRTC utilizes
MediaStream
objects to represent audio and video data. These streams can be obtained from the user's camera and microphone. WebRTC supports various codecs for encoding and decoding media, including VP8, VP9, and H.264 for video, and Opus and G.711 for audio. The choice of codec depends on factors such as bandwidth availability and desired quality.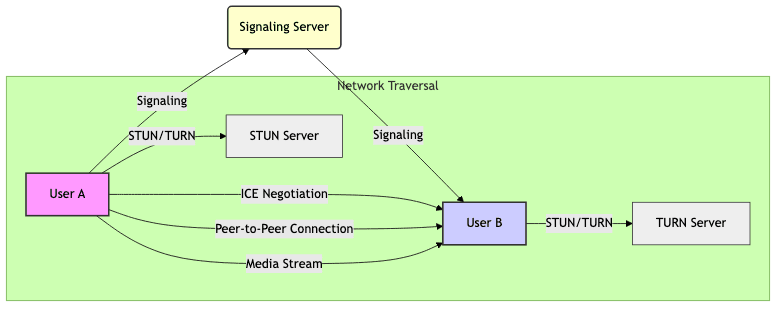
Implementing WebRTC Video Conferencing: A Practical Guide
Implementing WebRTC video conferencing requires careful planning and execution. Here's a practical guide to help you get started.
Choosing the Right WebRTC Framework or Library
Several WebRTC frameworks and libraries simplify the development process. Some popular options include:
- SimpleWebRTC: A high-level library that handles much of the complexity of WebRTC.
- PeerJS: Another popular library for simplifying peer-to-peer communication.
- Kurento: A media server that extends WebRTC capabilities with advanced features.
- Jitsi Videobridge: A selective forwarding unit (SFU) designed for large-scale video conferences.
The choice of framework depends on your specific requirements and the level of control you need over the underlying WebRTC APIs.
Setting up a Signaling Server
The signaling server is responsible for exchanging information between peers before establishing a direct connection. You can implement a signaling server using various technologies, such as:
- WebSocket: A popular choice for real-time bidirectional communication.
- Socket.IO: A library that simplifies WebSocket communication.
- REST APIs: A more traditional approach using HTTP requests.
The signaling server should handle tasks such as user registration, room management, and message routing.
Handling Peer Connections
Once the signaling server is set up, you can start handling peer connections. This involves creating
RTCPeerConnection
objects, gathering ICE candidates, and exchanging SDP (Session Description Protocol) offers and answers.JavaScript
1// Establishing a peer connection
2const peerConnection = new RTCPeerConnection(configuration);
3
4peerConnection.onicecandidate = (event) => {
5 if (event.candidate) {
6 // Send the ICE candidate to the other peer
7 signalingServer.send(JSON.stringify({'ice': event.candidate}));
8 }
9};
10
11peerConnection.onnegotiationneeded = async () => {
12 try {
13 const offer = await peerConnection.createOffer();
14 await peerConnection.setLocalDescription(offer);
15 // Send the offer to the other peer
16 signalingServer.send(JSON.stringify({'sdp': peerConnection.localDescription}));
17 } catch (err) {
18 console.error(err);
19 }
20};
21
Managing Media Streams
Managing media streams involves obtaining audio and video from the user's device, adding them to the
RTCPeerConnection
, and displaying the remote stream. You can use the getUserMedia
API to access the user's media.JavaScript
1// Handling media streams
2navigator.mediaDevices.getUserMedia({ video: true, audio: true })
3 .then((stream) => {
4 localVideo.srcObject = stream;
5 stream.getTracks().forEach(track => peerConnection.addTrack(track, stream));
6 })
7 .catch((error) => {
8 console.error('Error accessing media devices.', error);
9 });
10
11peerConnection.ontrack = (event) => {
12 remoteVideo.srcObject = event.streams[0];
13};
14
Implementing Error Handling and Robustness
Error handling is crucial for building robust WebRTC applications. You should handle potential errors such as network connectivity issues, media device access failures, and signaling server errors. Implement retry mechanisms and provide informative error messages to the user.
Security Considerations in WebRTC Video Conferencing
Security is paramount when implementing WebRTC video conferencing. WebRTC incorporates several built-in security mechanisms, but developers must also take additional precautions.
Encryption and Confidentiality
WebRTC uses DTLS (Datagram Transport Layer Security) for encrypting media streams and SRTP (Secure Real-time Transport Protocol) for securing the audio and video data during transmission. These protocols ensure confidentiality and prevent eavesdropping.
Authentication and Authorization
While WebRTC encrypts the streams, it doesn't inherently handle user authentication or authorization. You need to implement your own mechanisms to verify the identity of users and control access to video conferences. This might involve integrating with an existing authentication system or creating a custom solution.
Preventing Denial-of-Service Attacks
WebRTC applications can be vulnerable to denial-of-service (DoS) attacks. To mitigate this risk, you should implement rate limiting, input validation, and other security measures to prevent malicious actors from overwhelming your servers or disrupting video conferences.
Data Integrity and Tamper-Proofing
To ensure data integrity and prevent tampering, you can use techniques such as digital signatures and checksums. These techniques can verify that the data has not been modified during transmission.
Scalability and Performance Optimization
Scalability and performance are critical for WebRTC video conferencing applications, especially when supporting a large number of concurrent users.
Optimizing Media Streams
Optimizing media streams involves adjusting the video and audio quality based on network conditions. You can use techniques such as adaptive bitrate streaming (ABS) to dynamically adjust the bitrate to maintain a smooth video experience. Also, consider simulcast, which involves sending multiple video streams with different resolutions and frame rates, allowing the receiver to choose the most appropriate stream based on its network conditions.
Efficient Signaling
Efficient signaling is crucial for minimizing latency and reducing server load. Use lightweight signaling protocols such as WebSocket and optimize your signaling server code to handle a large number of concurrent connections.
Load Balancing and Server Clustering
Load balancing and server clustering can distribute the load across multiple servers, improving scalability and reliability. Use a load balancer to distribute incoming requests to different servers and implement server clustering to provide redundancy in case of server failures.
Choosing the Right Infrastructure
The choice of infrastructure can significantly impact the performance and scalability of your WebRTC video conferencing application. Consider using a cloud-based infrastructure with auto-scaling capabilities to handle fluctuating demand.
WebRTC Video Conferencing Use Cases and Applications
WebRTC video conferencing has a wide range of use cases and applications across various industries.
Telehealth and Remote Healthcare
WebRTC enables telehealth and remote healthcare by providing a secure and reliable platform for virtual consultations, remote patient monitoring, and telemedicine applications. Doctors can conduct virtual appointments with patients, monitor their vital signs remotely, and provide timely medical advice.
Online Education and Training
WebRTC powers online education and training by enabling virtual classrooms, interactive lectures, and remote learning experiences. Students can participate in live classes, collaborate with peers, and access educational resources from anywhere in the world.
Business Collaboration and Communication
WebRTC facilitates business collaboration and communication by enabling video conferencing, screen sharing, and instant messaging. Teams can collaborate on projects, conduct virtual meetings, and communicate effectively regardless of their location.
Social Networking and Entertainment
WebRTC enhances social networking and entertainment by enabling live streaming, video chat, and interactive gaming experiences. Users can connect with friends and family, share live videos, and participate in online games with real-time communication.
Comparing WebRTC with Traditional Video Conferencing Solutions
WebRTC offers several advantages over traditional video conferencing solutions.
Cost Comparison
WebRTC is often more cost-effective than traditional solutions, as it eliminates the need for proprietary hardware and software. WebRTC is open-source, reducing licensing fees.
Scalability Comparison
WebRTC can be more scalable than traditional solutions, as it leverages peer-to-peer connections and cloud-based infrastructure. Traditional systems often require expensive hardware upgrades to scale.
Feature Comparison
WebRTC offers a wide range of features, including video conferencing, screen sharing, and instant messaging. While traditional solutions may offer some of these features, WebRTC is often more flexible and customizable.
Security Comparison
WebRTC incorporates built-in security mechanisms, such as encryption and authentication. Traditional solutions may have security vulnerabilities or require additional security measures.
The Future of WebRTC Video Conferencing
The future of WebRTC video conferencing looks promising, with emerging technologies and trends shaping its evolution.
Emerging Technologies and Trends
- AI-powered video conferencing: AI can enhance video quality, reduce background noise, and provide real-time translation.
- WebAssembly (Wasm): Wasm can improve the performance of WebRTC applications by enabling near-native code execution in the browser.
- 5G and edge computing: 5G and edge computing can reduce latency and improve the quality of WebRTC video conferences.
Potential Developments and Improvements
- Improved scalability and performance: Ongoing efforts to optimize WebRTC for large-scale deployments.
- Enhanced security: Continued development of security protocols to address emerging threats.
- Seamless integration with other technologies: Integration with IoT devices, virtual reality, and augmented reality.
Conclusion
WebRTC video conferencing is a powerful technology that enables real-time communication in web browsers and mobile applications. By understanding the architecture, implementation, security, and scalability aspects of WebRTC, developers can build robust and innovative video conferencing solutions.
Learn more about WebRTC:
Dive deeper into the technical details of WebRTC.
Explore WebRTC APIs:
Discover the powerful APIs that power WebRTC.
Check out this great tutorial:
Follow a comprehensive WebRTC tutorial to build your own applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ