WebRTC JavaScript Example: Build Real-Time Apps
WebRTC (Web Real-Time Communication) empowers web browsers and mobile applications with real-time communication capabilities via simple APIs. This tutorial will guide you through building a basic WebRTC application using JavaScript, covering essential concepts and practical examples.
What is WebRTC?
WebRTC is a free, open-source project providing browsers and mobile applications with real-time communication (RTC) capabilities via simple APIs. It allows audio and video communication to work inside web pages by allowing direct peer-to-peer communication, eliminating the need for plugins or native apps.
Why use WebRTC with JavaScript?
JavaScript's ubiquity in web development makes it the ideal language for leveraging WebRTC. Combining WebRTC with JavaScript allows you to build real-time communication features directly into your web applications without needing additional software or complex server-side configurations. This makes development faster and more accessible.
Benefits of WebRTC
- No Plugins Required: WebRTC is built directly into modern browsers.
- Peer-to-Peer Communication: Reduces latency and server load.
- Open Source and Free: Accessible to everyone.
- Cross-Platform Compatibility: Works on various browsers and devices.
- Security: Built-in encryption for secure communication.
Prerequisites
Before we begin, ensure you have the following:
- Basic knowledge of HTML, CSS, and JavaScript.
- Node.js and npm installed on your system.
- A modern web browser (Chrome, Firefox, Safari).
Setting up Your Development Environment
Let's set up your environment to start building your WebRTC application.
Installing Node.js and npm
Node.js and npm (Node Package Manager) are essential for running a local development server and managing project dependencies. Download and install Node.js from the official website:
https://nodejs.org/
. npm comes bundled with Node.js.To verify your installation, open your terminal and run:
1node -v
2npm -v
3
You should see the version numbers for both Node.js and npm.
Creating a Project Directory
Create a new directory for your WebRTC project:
1mkdir webrtc-javascript-example
2cd webrtc-javascript-example
3
Initialize a new npm project:
1npm init -y
2
This creates a
package.json
file in your project directory.Setting up an HTML file
Create an
index.html
file in your project directory. This file will contain the basic HTML structure for your application:1<!DOCTYPE html>
2<html>
3<head>
4 <title>WebRTC JavaScript Example</title>
5</head>
6<body>
7 <h1>WebRTC Video Chat</h1>
8 <video id="localVideo" autoplay muted></video>
9 <video id="remoteVideo" autoplay></video>
10 <button id="callButton">Call</button>
11
12 <script src="script.js"></script>
13</body>
14</html>
15
A Simple WebRTC Video Chat Example
Now, let's build a simple WebRTC video chat application.
Basic HTML Structure
As shown above, our
index.html
file includes two video elements (localVideo
and remoteVideo
) to display the local and remote video streams, and a button to initiate the call. We also link to a script.js
file, which will contain the JavaScript code for our WebRTC logic.JavaScript Code for Establishing Connection
Create a
script.js
file in your project directory. This file will contain the core WebRTC JavaScript code.First, let's get references to the HTML elements and define some global variables:
1const localVideo = document.getElementById('localVideo');
2const remoteVideo = document.getElementById('remoteVideo');
3const callButton = document.getElementById('callButton');
4
5let localStream;
6let remoteStream;
7let peerConnection;
8
9const configuration = {
10 iceServers: [{ urls: 'stun:stun.l.google.com:19302' }],
11};
12
13
The
configuration
object specifies the STUN server to use for NAT traversal. STUN servers help determine the public IP address of the client.Next, use
getUserMedia
to access the user's camera and microphone:1// Code Snippet: getUserMedia
2async function startVideo() {
3 try {
4 localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
5 localVideo.srcObject = localStream;
6 } catch (error) {
7 console.error('Error accessing media devices:', error);
8 }
9}
10
11startVideo();
12
The
startVideo
function uses navigator.mediaDevices.getUserMedia
to request access to the user's camera and microphone. The returned stream is then assigned to the srcObject
property of the localVideo
element.Now, let's set up the
callButton
click event to initiate the WebRTC connection:1callButton.addEventListener('click', async () => {
2 console.log('Call button clicked');
3 startCall();
4});
5
6async function startCall() {
7 console.log('Starting the call');
8 peerConnection = new RTCPeerConnection(configuration);
9
10 peerConnection.onicecandidate = (event) => {
11 if (event.candidate) {
12 console.log('ICE candidate:', event.candidate);
13 // Send the ICE candidate to the remote peer
14 // (This part requires a signaling server)
15 }
16 };
17
18 peerConnection.ontrack = (event) => {
19 console.log('Remote stream received');
20 remoteVideo.srcObject = event.streams[0];
21 };
22
23 localStream.getTracks().forEach((track) => {
24 peerConnection.addTrack(track, localStream);
25 });
26
27 // Code Snippet: createOffer
28 try {
29 const offer = await peerConnection.createOffer();
30 // Code Snippet: setLocalDescription
31 await peerConnection.setLocalDescription(offer);
32 console.log('Offer created:', offer);
33
34 // Send the offer to the remote peer
35 // (This part requires a signaling server)
36
37 //Simulate answer for local testing (remove in real application)
38 setTimeout(async () => {
39 console.log('Simulating Answer');
40 // Code Snippet: setRemoteDescription
41 await peerConnection.setRemoteDescription(offer);
42
43 // Code Snippet: createAnswer
44 const answer = await peerConnection.createAnswer();
45
46 // Code Snippet: setLocalDescription
47 await peerConnection.setLocalDescription(answer);
48 console.log('Answer created:', answer);
49
50 await peerConnection.setRemoteDescription(answer);
51 }, 3000);
52
53 } catch (error) {
54 console.error('Error creating offer:', error);
55 }
56}
57
This code does the following:
- Creates a new
RTCPeerConnection
instance. - Sets up event listeners for
icecandidate
andtrack
events. - Adds the local media tracks to the peer connection.
- Creates an offer using
peerConnection.createOffer
. - Sets the local description using
peerConnection.setLocalDescription
. - Sends the offer to the remote peer through a signaling server.
Handling ICE Candidates
ICE (Interactive Connectivity Establishment) is a framework used to discover the best way for two peers to communicate. ICE candidates are potential network addresses that can be used to establish a connection. The
onicecandidate
event is fired whenever a new ICE candidate is gathered.1// Code Snippet: onicecandidate
2peerConnection.onicecandidate = (event) => {
3 if (event.candidate) {
4 console.log('ICE candidate:', event.candidate);
5 // Send the ICE candidate to the remote peer via signaling server
6 }
7};
8
When an ICE candidate is available, it needs to be sent to the remote peer through a signaling server. The remote peer will then add the candidate to its peer connection.
Note: A signaling server is required to exchange SDP (Session Description Protocol) offers/answers and ICE candidates between peers. The implementation of a signaling server is beyond the scope of this example. For development purposes, you can simulate the answer. However, for production, you will need to use a signaling server.
Advanced WebRTC Concepts
Data Channels
WebRTC data channels enable peer-to-peer exchange of arbitrary data. This is useful for applications requiring data transfer alongside audio and video, such as file sharing or game data.
1// Code Snippet: Data Channel creation and data sending/receiving
2let dataChannel = peerConnection.createDataChannel('myDataChannel');
3
4dataChannel.onopen = () => {
5 console.log('Data channel opened');
6 dataChannel.send('Hello, world!');
7};
8
9dataChannel.onmessage = (event) => {
10 console.log('Received message:', event.data);
11};
12
13peerConnection.ondatachannel = (event) => {
14 dataChannel = event.channel;
15 dataChannel.onopen = () => {
16 console.log('Data channel opened by remote peer');
17 };
18
19 dataChannel.onmessage = (event) => {
20 console.log('Received message from remote peer:', event.data);
21 };
22};
23
24
The
createDataChannel
method creates a new data channel. The onopen
event is fired when the data channel is ready, and the send
method is used to send data. The onmessage
event is fired when data is received. The ondatachannel
event is fired when the remote peer opens the data channel.Screen Sharing
WebRTC also supports screen sharing, allowing users to share their screen with other participants in a call.
1// Code Snippet: Enabling screen sharing with getUserMedia
2async function startScreenShare() {
3 try {
4 const stream = await navigator.mediaDevices.getDisplayMedia({
5 video: true,
6 audio: false
7 });
8
9 localVideo.srcObject = stream;
10 stream.getVideoTracks()[0].onended = () => {
11 stopScreenShare();
12 };
13 } catch (err) {
14 console.error("Error accessing screen: " + err);
15 }
16}
17
18async function stopScreenShare() {
19 try {
20 await startVideo();
21 } catch (err) {
22 console.error("Error stop sharing screen: " + err);
23 }
24}
25
26callButton.addEventListener('click', async () => {
27 startScreenShare();
28});
29
The
getDisplayMedia
method requests access to the user's screen. The onended
function is called when the stream is closed.Browser compatibility considerations:
getDisplayMedia
requires HTTPS and user permission to be enabled.Audio Only Communication
WebRTC can be configured for audio-only communication by adjusting the constraints passed to
getUserMedia
:1// Code Snippet: Configuring constraints for audio only
2async function startAudio() {
3 try {
4 const stream = await navigator.mediaDevices.getUserMedia({
5 audio: true,
6 video: false
7 });
8 localVideo.srcObject = stream;
9 } catch (err) {
10 console.error("Error accessing audio: " + err);
11 }
12}
13
Setting
video: false
in the constraints object will request only the audio stream.Handling Errors and Debugging
When working with WebRTC, it's essential to handle errors gracefully. Common errors include:
NotAllowedError
: The user denied access to the camera or microphone.NotFoundError
: The requested media device is not available.NotReadableError
: The media device is already in use.OverconstrainedError
: The specified constraints cannot be satisfied.
Use
try...catch
blocks to handle these errors and provide informative messages to the user. Use console.log
statements to debug and trace the flow of your application. Additionally, browser developer tools offer insights into WebRTC connection stats and potential issues.Optimizing WebRTC Performance
Bandwidth Management
WebRTC automatically adjusts the video bitrate based on network conditions. However, you can further optimize bandwidth usage by setting constraints on the video resolution and frame rate. For example:
1const constraints = {
2 video: {
3 width: { max: 640 },
4 frameRate: { max: 30 }
5 },
6 audio: true
7};
8
Code Optimization
- Minimize unnecessary re-renders by caching frequently accessed DOM elements.
- Use efficient data structures and algorithms for data processing.
- Avoid blocking the main thread with long-running tasks.
- Use Promises and async/await for asynchronous operations to keep the code clean and readable.
Security Considerations for WebRTC
- Use HTTPS: WebRTC requires HTTPS for security reasons.
- Secure Signaling: Protect your signaling server from unauthorized access.
- Validate Inputs: Sanitize and validate all data received from remote peers.
- Encryption: WebRTC uses DTLS for encryption, ensure it is enabled.
- Permissions: Prompt the user for camera and microphone access with clear explanations.
Conclusion
This tutorial provided a basic introduction to WebRTC with JavaScript. You have learned how to set up a development environment, establish a peer-to-peer connection, and implement data channels and screen sharing. By understanding these fundamental concepts, you can build powerful real-time communication applications. Explore the resources below to deepen your knowledge and explore advanced features of WebRTC.
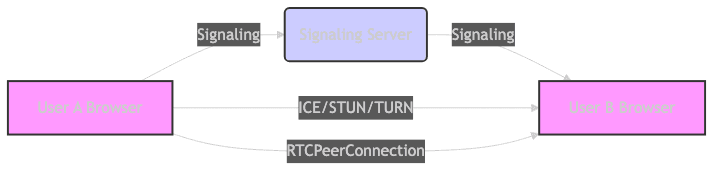
Resources:
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ