Introduction to WebRTC App Development
WebRTC (Web Real-Time Communication) has revolutionized the way we build real-time communication applications. It enables seamless peer-to-peer communication directly within web browsers and mobile apps, eliminating the need for plugins or native downloads. This article provides a comprehensive guide to WebRTC app development, covering everything from its architecture and components to implementation, optimization, and deployment.
What is WebRTC?
WebRTC is an open-source project providing real-time communication capabilities to web browsers and native mobile apps. It supports video, voice, and generic data transmission between peers, empowering developers to create interactive and engaging applications.
Why Choose WebRTC for App Development?
WebRTC offers several compelling advantages for building real-time communication apps. It's natively supported by major web browsers, reducing development complexity. Its peer-to-peer architecture minimizes latency and server load, leading to improved performance. Moreover, WebRTC's open-source nature allows for customization and integration with other technologies. Choosing WebRTC for your WebRTC application development offers a robust and efficient solution.
Benefits of WebRTC
WebRTC provides several key benefits:
- Real-time Communication: Enables low-latency video, voice, and data transmission.
- Browser Compatibility: Supported by most modern web browsers.
- Peer-to-Peer: Reduces server load and latency.
- Open Source: Allows for customization and extension.
- Cost-Effective: Reduces infrastructure costs due to P2P architecture.
WebRTC Architecture and Components
Understanding the WebRTC architecture is crucial for successful WebRTC app development. WebRTC relies on several key components to establish and maintain peer-to-peer connections. These connections allows you to build WebRTC mobile app or WebRTC web app.
Understanding the WebRTC Architecture
WebRTC architecture involves the browser acting as a client, communicating directly with other browsers without needing an intermediary server for media. This direct peer-to-peer connection is facilitated by signaling, STUN, and TURN servers.
The signaling server facilitates the exchange of metadata and session information. STUN (Session Traversal Utilities for NAT) servers help discover the public IP address of the client, while TURN (Traversal Using Relays around NAT) servers act as relays when a direct connection cannot be established.
Key Components of a WebRTC Application
A typical WebRTC application comprises the following components:
- Signaling Server: Facilitates the exchange of signaling messages between peers.
- STUN Server: Helps peers discover their public IP addresses.
- TURN Server: Relays traffic when direct peer-to-peer connections fail.
- WebRTC API: Provides JavaScript APIs for accessing media devices and establishing peer connections.
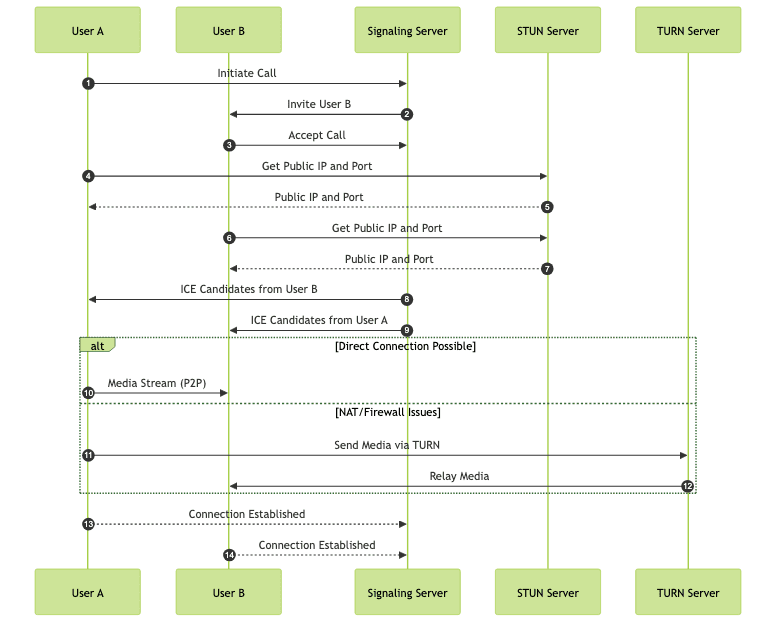
Signaling Server
The signaling server is responsible for exchanging metadata between peers before a direct connection is established. This includes session descriptions (SDP) and ICE candidates. Consider using websockets as transport layer to implement the signaling process.
Node.js Signaling Server
1const WebSocket = require('ws');
2
3const wss = new WebSocket.Server({ port: 8080 });
4
5wss.on('connection', ws => {
6 console.log('Client connected');
7
8 ws.on('message', message => {
9 console.log(`Received message: ${message}`);
10
11 wss.clients.forEach(client => {
12 if (client !== ws && client.readyState === WebSocket.OPEN) {
13 client.send(message);
14 }
15 });
16 });
17
18 ws.on('close', () => {
19 console.log('Client disconnected');
20 });
21});
22
23console.log('Signaling server started on port 8080');
24
STUN and TURN Servers
STUN (Session Traversal Utilities for NAT) servers are used to discover the public IP address of clients behind Network Address Translation (NAT). TURN (Traversal Using Relays around NAT) servers relay traffic when direct peer-to-peer connections cannot be established due to firewall restrictions.
Choosing the Right WebRTC Framework and SDK
Selecting the appropriate WebRTC framework and SDK is crucial for efficient and successful development. Several options are available, each with its own strengths and weaknesses. WebRTC SDK and WebRTC libraries are the building blocks for your application.
Popular WebRTC Frameworks and SDKs
Several frameworks and SDKs simplify WebRTC app development. Some popular choices include:
- JavaScript WebRTC API: The native browser API provides the foundation for WebRTC communication.
- React Native WebRTC: Allows building cross-platform mobile apps using React Native.
- Flutter WebRTC: Enables cross-platform development with Flutter.
- Native WebRTC SDKs: For native iOS and Android development.
JavaScript WebRTC
The JavaScript WebRTC API provides direct access to WebRTC functionalities within web browsers. It offers fine-grained control over the communication process, making it suitable for custom applications. Using Javascript WebRTC you can create WebRTC web app.
React Native WebRTC
React Native WebRTC allows you to build cross-platform mobile applications using React Native. It provides a bridge between JavaScript and native WebRTC implementations, enabling access to device cameras and microphones. It is important for WebRTC mobile app development.
Other Frameworks (Flutter, NativeScript, etc.)
Flutter WebRTC and NativeScript WebRTC offer alternative options for cross-platform development. Flutter provides a rich set of UI components and a performant rendering engine, while NativeScript allows leveraging native platform APIs. These options are helpful for cross-platform WebRTC app development.
Factors to Consider When Choosing a Framework
When selecting a WebRTC framework, consider the following factors:
- Platform Support: Ensure the framework supports your target platforms (web, iOS, Android).
- Ease of Use: Evaluate the learning curve and development experience.
- Community Support: Check for active community forums and documentation.
- Performance: Assess the framework's impact on application performance.
- Customization: Determine the level of customization offered.
Developing a WebRTC App: A Step-by-Step Guide
This section provides a step-by-step guide to developing a WebRTC application. This will give you a good understanding on how to build WebRTC app.
Setting up the Development Environment
- Install Node.js and npm (Node Package Manager).
- Set up a code editor (e.g., Visual Studio Code).
- Create a new project directory.
- Initialize a new npm project:
npm init -y
Designing the User Interface
Create a simple user interface with HTML and CSS. Include video elements for local and remote streams, along with buttons for starting and stopping calls. When designing the UI, keep in mind WebRTC best practices.
Implementing Core WebRTC Functionality
Use the JavaScript WebRTC API to access media devices, establish peer connections, and exchange media streams. Implement the following steps:
- Get User Media: Access the user's camera and microphone.
- Create Peer Connection: Create
RTCPeerConnection
objects for each peer. - Add Tracks: Add media tracks to the peer connection.
- Create and Send Offer: Generate an SDP offer and send it to the remote peer.
- Receive and Process Answer: Receive the SDP answer and set it as the remote description.
- Exchange ICE Candidates: Exchange ICE candidates with the remote peer.
JavaScript: Basic WebRTC Connection
1const localVideo = document.getElementById('localVideo');
2const remoteVideo = document.getElementById('remoteVideo');
3
4let localStream;
5let remoteStream;
6let peerConnection;
7
8async function startWebRTC() {
9 try {
10 localStream = await navigator.mediaDevices.getUserMedia({ video: true, audio: true });
11 localVideo.srcObject = localStream;
12
13 peerConnection = new RTCPeerConnection();
14
15 localStream.getTracks().forEach(track => {
16 peerConnection.addTrack(track, localStream);
17 });
18
19 peerConnection.ontrack = event => {
20 remoteVideo.srcObject = event.streams[0];
21 };
22
23 // Signaling logic here (createOffer, sendOffer, etc.)
24
25 } catch (error) {
26 console.error('Error starting WebRTC:', error);
27 }
28}
29
30startWebRTC();
31
Handling Signaling and Peer Connections
The signaling process involves exchanging session descriptions (SDP) and ICE candidates between peers. Use WebSockets to establish a persistent connection between the peers and the signaling server.
- Establish WebSocket Connection: Connect to the signaling server.
- Exchange SDP Offers and Answers: Send and receive SDP messages.
- Exchange ICE Candidates: Send and receive ICE candidates.
JavaScript: Signaling with WebSockets
1const socket = new WebSocket('ws://localhost:8080');
2
3socket.onopen = () => {
4 console.log('Connected to signaling server');
5};
6
7socket.onmessage = event => {
8 const message = JSON.parse(event.data);
9
10 switch (message.type) {
11 case 'offer':
12 // Handle offer
13 break;
14 case 'answer':
15 // Handle answer
16 break;
17 case 'candidate':
18 // Handle ICE candidate
19 break;
20 }
21};
22
23function sendMessage(message) {
24 socket.send(JSON.stringify(message));
25}
26
Advanced WebRTC Techniques
Advanced WebRTC techniques can improve performance, scalability, and security.
Optimizing WebRTC Performance
- Codec Selection: Choose efficient video and audio codecs.
- Bandwidth Estimation: Adapt video quality based on available bandwidth.
- Congestion Control: Implement congestion control algorithms.
- Optimize resolution: Choose the lowest possible resolution that still meet the need.
Implementing Scalability
- SFU (Selective Forwarding Unit): Use an SFU to forward media streams to multiple participants.
- MCU (Multipoint Control Unit): Use an MCU to mix media streams into a single stream.
- Load Balancing: Distribute traffic across multiple servers.
Addressing Security Concerns
- Encryption: Use DTLS-SRTP for encrypting media streams.
- Authentication: Implement user authentication and authorization.
- Signaling Security: Secure the signaling channel with HTTPS or WSS.
- Firewall Configuration: Configure firewalls to allow WebRTC traffic.
Deploying Your WebRTC Application
Deploying your WebRTC application involves choosing a suitable platform, testing, optimizing, and monitoring.
Choosing a Deployment Platform
- Web Hosting: Deploy your web application to a web hosting provider.
- Cloud Platforms: Use cloud platforms like AWS, Google Cloud, or Azure.
- Mobile App Stores: Deploy your mobile app to the Apple App Store or Google Play Store.
Testing and Optimization
- Cross-Browser Testing: Test your application on different browsers.
- Performance Testing: Measure and optimize application performance.
- User Acceptance Testing (UAT): Conduct UAT to gather user feedback.
Monitoring and Maintenance
- Monitor Application Performance: Track key performance metrics.
- Address Bugs and Issues: Fix bugs and address user issues promptly.
- Update Dependencies: Keep dependencies up to date with the latest versions.
WebRTC App Development Use Cases and Examples
WebRTC has a wide range of use cases, including:
Video Conferencing
WebRTC enables real-time video conferencing solutions, allowing users to connect and collaborate remotely. This is a typical WebRTC use cases that empowers collaboration.
Live Streaming
WebRTC can be used for live streaming applications, enabling real-time broadcasting of video and audio content. WebRTC live streaming could be used for educational, commercial and many other proposes.
VoIP Calling
WebRTC facilitates VoIP calling applications, enabling voice communication over the internet. It is a cost effective solution with decent sound quality for WebRTC application development.
Other Use Cases (e.g., remote collaboration, telehealth)
WebRTC can also be used for remote collaboration tools, telehealth applications, and other real-time communication scenarios. It opens a new era for collaboration and healthcare.
Conclusion and Future of WebRTC
WebRTC is a powerful technology that has transformed real-time communication. Its versatility, ease of use, and open-source nature make it an excellent choice for building a wide range of applications. As technology evolves, WebRTC is expected to play an increasingly important role in shaping the future of real-time communication.
Resources:
- WebRTC Interoperability:
Browser compatibility information
- Example WebRTC project:
https://github.com/webrtc/samples
A helpful open-source project
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ