Introduction: Harnessing the Power of Translation APIs
In today's globalized world, the ability to communicate across language barriers is more critical than ever. Translation APIs offer a powerful and efficient solution, enabling developers to seamlessly integrate language translation capabilities into their applications. Whether you're building a multilingual website, a global e-commerce platform, or a real-time communication tool, a translation API can be a game-changer. This comprehensive guide will delve into the world of translation APIs, exploring top providers, key considerations, integration techniques, and future trends.
Realtime Translation APIs Example
Real-time transcription turns spoken words into instant text, transforming how meetings, webinars, and classes are experienced. With VideoSDK, integrating this feature into your React app is intuitive and customizable.
<iframe
src="
https://www.youtube.com/embed/bhPgjMXL6H8?rel=0
" style=" position: absolute; top: 0; left: 0; width: 100%; height: 100%; border: 0; " allowfullscreen frameborder="0"</iframe> </div>
What is a Translation API?
A translation API (Application Programming Interface) is a cloud-based service that allows software applications to automatically translate text or speech from one language to another. These APIs leverage machine learning and neural networks to provide accurate and efficient translations, enabling developers to bypass the complexities of building their own translation engines. The API provides an interface to send the text to be translated and receives the translated text in the desired language.
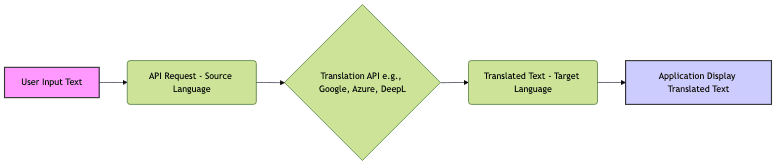
The Growing Importance of Machine Translation
Machine translation has evolved dramatically in recent years, thanks to advancements in neural networks and deep learning. Neural Machine Translation APIs now deliver significantly improved accuracy and fluency compared to traditional rule-based systems. This progress has made API translation a viable solution for a wide range of applications, from customer support chatbots to content localization workflows. The increasing demand for global communication fuels the continued growth and refinement of machine translation APIs.
Benefits of Using a Translation API
Using a translation API offers numerous advantages for developers:
- Cost-effectiveness: Avoid the expense of building and maintaining your own translation infrastructure.
- Scalability: Handle large volumes of translation requests with ease.
- Speed: Obtain near real-time translations for dynamic content.
- Accuracy: Leverage state-of-the-art neural machine translation APIs for high-quality results.
- Ease of Integration: Simple APIs allow for quick integration into existing applications.
Top Translation API Providers: A Comparative Analysis
Choosing the right translation API provider is crucial for the success of your project. Here's a comparative analysis of some of the leading players in the market:
Google Cloud Translation API
Google Cloud Translation API is a powerful cloud translation API that leverages Google's extensive machine learning expertise. It supports a vast number of languages and offers features like language detection and custom models. It also allows both REST API Translation and JSON Translation API calls. The Google Cloud Translation API is a robust choice for applications requiring high accuracy and scalability.
Python
1from google.cloud import translate_v2 as translate
2
3client = translate.Client()
4
5text = 'Hello, world!'
6target = 'es'
7
8translation = client.translate(text, target_language=target)
9
10print(f"Text: {text}")
11print(f"Translation: {translation['translatedText']}")
12
Azure Cognitive Services Translator
Azure Cognitive Services Translator provides language translation API capabilities with a focus on enterprise-grade security and compliance. It supports a wide range of languages and offers features like custom terminology and real-time conversation translation. The Azure Translator API is a strong option for organizations seeking a secure and reliable translation solution.
C#
1using Microsoft.Azure.CognitiveServices.Translator;
2using System.Threading.Tasks;
3
4class Program
5{
6 static async Task Main(string[] args)
7 {
8 string subscriptionKey = "YOUR_SUBSCRIPTION_KEY";
9 string endpoint = "YOUR_ENDPOINT";
10 string text = "Hello, world!";
11 string toLanguage = "es";
12
13 var client = new TranslatorClient(new ApiKeyServiceClientCredentials(subscriptionKey)) { Endpoint = endpoint };
14 var result = await client.TranslateAsync(new string[] { text }, toLanguage, "en");
15
16 System.Console.WriteLine($"Translation: {result[0].Translations[0].Text}");
17 }
18}
19
Amazon Translate
Amazon Translate is a machine translation API service that offers fast and cost-effective translation. It supports a growing number of languages and is tightly integrated with other AWS services. The Amazon Translate API is a good choice for applications that require high throughput and seamless integration with the AWS ecosystem.
Java
1import com.amazonaws.auth.AWSCredentialsProvider;
2import com.amazonaws.auth.profile.ProfileCredentialsProvider;
3import com.amazonaws.services.translate.AmazonTranslate;
4import com.amazonaws.services.translate.AmazonTranslateClientBuilder;
5import com.amazonaws.services.translate.model.TranslateTextRequest;
6import com.amazonaws.services.translate.model.TranslateTextResult;
7
8public class TranslateExample {
9
10 public static void main(String[] args) {
11 AWSCredentialsProvider credentialsProvider = new ProfileCredentialsProvider("default");
12
13 AmazonTranslate translate = AmazonTranslateClientBuilder.standard()
14 .withCredentials(credentialsProvider)
15 .withRegion("us-west-2") // Replace with your region
16 .build();
17
18 TranslateTextRequest request = new TranslateTextRequest()
19 .withText("Hello, world!")
20 .withSourceLanguageCode("en")
21 .withTargetLanguageCode("es");
22
23 TranslateTextResult result = translate.translateText(request);
24
25 System.out.println("Translation: " + result.getTranslatedText());
26 }
27}
28
DeepL API
DeepL API is known for its exceptional translation quality, often surpassing other providers in terms of fluency and naturalness. While it supports fewer languages than some competitors, its focus on accuracy makes it a preferred choice for applications where quality is paramount. DeepL offers both free translation API tiers with limited usage, as well as a paid translation API with more capacity.
Key Features and Considerations when Choosing a Translation API
Selecting the best translation API for your needs requires careful consideration of several factors:
Accuracy and Quality
The accuracy of the API translation is arguably the most important factor. Look for APIs that utilize neural machine translation and have a proven track record of delivering high-quality results. Evaluate the API's performance with your specific content and language pairs.
Supported Languages and Language Pairs
Ensure that the API supports all the languages you need to translate. Check for the availability of specific language pairs, as some APIs may offer better support for certain combinations than others. Consider whether the API supports dialects or regional variations.
API Features and Functionality
Evaluate the API's features and functionality to ensure they meet your requirements. Some APIs offer features like language detection, custom terminology, and real-time translation. Consider whether you need features like document translation, speech-to-text translation, or subtitle translation.
Pricing and Scalability
Understand the translation API pricing model and ensure it aligns with your budget and usage patterns. Most APIs offer tiered pricing based on the volume of translation requests. Consider the API's scalability to handle potential spikes in demand. Evaluate if there are differences in API translation cost optimization based on different providers.
Security and Data Privacy
Protect the privacy of your data by choosing an API provider with robust security measures. Ensure that the API complies with relevant data privacy regulations. Understand how the API provider handles and stores your data.
Integrating Translation APIs into Your Applications
Integrating a translation API into your application involves several steps:
Choosing the Right Programming Language
Most translation APIs offer translation API SDK for popular programming languages like Python, Java, Node.js, PHP, and C#. Choose the language that best suits your project and development expertise. You can use Python Translation API, Java Translation API, Node.js Translation API, PHP Translation API, or C# Translation API depending on your language choice.
Authentication and API Keys
Obtain an API key or other authentication credentials from your chosen provider. Securely store and manage your API keys to prevent unauthorized access.
Making API Calls and Handling Responses
Use the API's documentation to understand how to make translation requests. Send the text to be translated and specify the source and target languages. Parse the API's response to extract the translated text. Here's an example of calling the API using curl:
curl
1curl -X POST \
2 'https://api.example.com/translate' \
3 -H 'Content-Type: application/json' \
4 -H 'Authorization: Bearer YOUR_API_KEY' \
5 -d '{
6 "text": "Hello, world!",
7 "source_language": "en",
8 "target_language": "es"
9 }'
10
Error Handling and Best Practices
Implement robust error handling to gracefully manage potential issues like network errors, invalid API keys, or rate limits. Follow best practices for API usage, such as caching translated text and optimizing request payloads.
Real-world Examples and Use Cases
Translation APIs are used in a wide variety of applications, including:
- Multilingual Websites: Automatically translate website content for international audiences.
- E-commerce Platforms: Translate product descriptions and customer reviews for global shoppers.
- Customer Support Chatbots: Provide real-time translation for customer support interactions.
- Document Translation: Translate large documents for business or legal purposes.
- Video Translation API: Translate audio from video content to generate subtitles and dubbing in multiple languages.
- Subtitle translation API: Automatic subtitle translation using translation API services.
- Speech-to-text translation API: Translate speech to text to translate automatically
Advanced Techniques and Future Trends
As machine translation technology continues to evolve, several advanced techniques and future trends are emerging:
Custom Models and Training Data
Some translation API services allow you to train custom models with your own data. This can significantly improve accuracy for specialized terminology and domains.
Handling Specialized Terminology and Domains
Develop strategies for handling specialized terminology and domains, such as medical or legal jargon. This may involve using custom dictionaries or training custom models.
Neural Machine Translation Advancements
Neural Machine Translation is constantly evolving, with new architectures and training techniques emerging regularly. Stay up-to-date on the latest advancements to leverage the most accurate and fluent translation models.
The Rise of Multilingual Models
Multilingual models are capable of translating between multiple languages with a single model. This can simplify development and improve efficiency for applications that support a large number of languages. The Best Translation API will embrace these technologies to stay competitive.
Conclusion: The Future of Translation APIs
Translation APIs are transforming the way we communicate across language barriers. With advancements in machine learning and neural networks, these APIs are becoming increasingly accurate, efficient, and accessible. By leveraging the power of automated translation APIs, developers can create truly global applications that connect people from all over the world. Understanding the various providers, the nuances of API translation accuracy, and the ability to perform API translation integration effectively will be crucial for developers.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ