Introduction to Socket.IO Usage
Socket.IO is a powerful JavaScript library for real-time, bidirectional, and event-based communication. It enables you to build dynamic and interactive web applications that require instant data updates.
What is Socket.IO?
Socket.IO is a library that enables real-time, bidirectional communication between web clients and servers. It consists of a Node.js server and a JavaScript client library. Socket.IO uses WebSocket as the primary transport, but falls back to other techniques such as HTTP long polling when WebSocket is not supported.
Why Use Socket.IO?
Socket.IO simplifies the process of building real-time applications. It provides a simple and easy-to-use API for handling connections, sending messages, and managing events. Its fallback mechanisms ensure broad compatibility across different browsers and network environments. Furthermore, Socket.IO supports features like broadcasting, namespaces, and rooms, making it easy to create complex real-time applications such as chat applications and online games.
Key Features and Benefits
- Real-time Communication: Enables instant data updates between the server and clients.
- Bidirectional Communication: Allows both the server and clients to send and receive messages.
- Event-Based Communication: Uses events to trigger actions and exchange data.
- Automatic Reconnection: Handles disconnections and automatically reconnects clients.
- Broad Compatibility: Supports a wide range of browsers and devices with fallback mechanisms.
- Namespaces and Rooms: Organizes connections into logical groups for targeted communication.
- Acknowledgements: Ensures message delivery and provides request-response patterns.
Setting up a Socket.IO Server
Setting up a Socket.IO server involves configuring a Node.js server and integrating the Socket.IO library. This section will guide you through the basic steps.
Node.js Server Setup
First, you need to have Node.js installed on your machine. Then, create a new Node.js project and install the
socket.io
package using npm:1npm install socket.io
2
Here's a basic Node.js Socket.IO server setup:
Javascript
1const { createServer } = require("http");
2const { Server } = require("socket.io");
3
4const httpServer = createServer();
5const io = new Server(httpServer, {
6 cors: {
7 origin: "http://localhost:3000", // Replace with your client's origin
8 methods: ["GET", "POST"]
9 }
10});
11
12io.on("connection", (socket) => {
13 console.log("A user connected");
14
15 socket.on("disconnect", () => {
16 console.log("User disconnected");
17 });
18
19 socket.on("chat message", (msg) => {
20 console.log("message: " + msg);
21 io.emit("chat message", msg); // Broadcast to all connected clients
22 });
23});
24
25httpServer.listen(3001, () => {
26 console.log('listening on *:3001');
27});
28
This code creates an HTTP server and attaches Socket.IO to it. The
io.on('connection', ...)
block handles new client connections. Inside this block, you can define event listeners for incoming messages (like "chat message") and emit events to send data to clients.Choosing the Right Socket.IO Version
It's important to choose the correct version of Socket.IO for your project. Different versions may have different features, bug fixes, and compatibility requirements. Always refer to the official Socket.IO documentation for version-specific information and migration guides.
Handling CORS
Cross-Origin Resource Sharing (CORS) is a security mechanism that restricts web pages from making requests to a different domain than the one that served the web page. When using Socket.IO, you need to configure CORS to allow connections from your client-side application. This is typically done by setting the
cors
option when creating the Socket.IO server instance.For example:
Javascript
1const io = new Server(httpServer, {
2 cors: {
3 origin: "http://localhost:3000", // Replace with your client's origin
4 methods: ["GET", "POST"]
5 }
6});
7
Replace
http://localhost:3000
with the actual origin of your client-side application.Connecting to a Socket.IO Server
To establish a real-time connection, you need to connect the client-side application to the Socket.IO server. This involves using the Socket.IO client library in your web application.
Client-Side Connection
Include the Socket.IO client library in your HTML file. You can either download it from the Socket.IO website or use a CDN:
HTML
1<script src="https://cdn.socket.io/4.7.2/socket.io.min.js" integrity="sha384-Lc9LJmPTgK/w5T/mI48lEzQ6bI5hQ5QcQ5i7C7F5y/d7WvY6e1A5iJm/w5W9bWJ" crossorigin="anonymous"></script>
2
Then, use the following JavaScript code to connect to the Socket.IO server:
Javascript
1const socket = io("http://localhost:3001"); // Replace with your server URL
2
3socket.on("connect", () => {
4 console.log("Connected to server");
5});
6
7socket.on("disconnect", () => {
8 console.log("Disconnected from server");
9});
10
Connecting with Different Frameworks
Socket.IO can be easily integrated with popular JavaScript frameworks like React, Vue, and Angular. Here's a brief overview of how to use Socket.IO with each framework:
- React: Use the
useEffect
hook to establish the connection when the component mounts and clean up the connection when the component unmounts. Consider using a context to provide the socket instance to different components. - Vue: Use the
mounted
lifecycle hook to establish the connection and thebeforeDestroy
hook to clean up the connection. You can also use Vuex to manage the socket state. - Angular: Use the
ngOnInit
lifecycle hook to establish the connection and thengOnDestroy
hook to clean up the connection. Consider creating a Socket.IO service that can be injected into components.
In all cases, remember to properly manage the socket connection's lifecycle to avoid memory leaks and unexpected behavior. For example with React, you could have something like this:
JSX
1import React, { useEffect } from 'react';
2import io from 'socket.io-client';
3
4function MyComponent() {
5 useEffect(() => {
6 const socket = io('http://localhost:3001');
7
8 socket.on('connect', () => {
9 console.log('Connected!');
10 });
11
12 socket.on('message', (data) => {
13 console.log('Received message:', data);
14 });
15
16 return () => {
17 socket.disconnect();
18 };
19 }, []);
20
21 return (
22 <div>
23 {/* Your component content */}
24 </div>
25 );
26}
27
28export default MyComponent;
29
Handling Disconnections and Reconnections
Socket.IO provides automatic reconnection capabilities. When a client disconnects from the server, Socket.IO will automatically attempt to reconnect. You can listen for the
disconnect
and reconnect
events to handle these scenarios in your application.Javascript
1socket.on("disconnect", () => {
2 console.log("Disconnected from server");
3});
4
5socket.on("reconnect", () => {
6 console.log("Reconnected to server");
7});
8
You can also configure the reconnection attempts and delay using the
reconnectionAttempts
and reconnectionDelay
options.Emitting and Receiving Events
Socket.IO uses events to communicate between the server and clients. You can emit events to send data and listen for events to receive data.
Basic Event Handling
To emit an event, use the
socket.emit()
method. To listen for an event, use the socket.on()
method.Javascript
1// Server-side
2socket.on("new message", (data) => {
3 console.log("Received new message: " + data);
4 socket.emit("new message", data);
5});
6
7// Client-side
8socket.emit("new message", "Hello from client!");
9
10socket.on("new message", (data) => {
11 console.log("Received new message: " + data);
12});
13
Using Acknowledgements
Acknowledgements allow you to implement request-response patterns with Socket.IO. When emitting an event, you can provide a callback function that will be executed when the server acknowledges the event.
Javascript
1// Server-side
2socket.on("add user", (username, callback) => {
3 console.log("User added: " + username);
4 callback({
5 numUsers: 1
6 });
7});
8
9// Client-side
10socket.emit("add user", "John", (data) => {
11 console.log(data.numUsers);
12});
13
Broadcasting Events
Broadcasting allows you to send an event to all connected clients except the sender. Use
socket.broadcast.emit()
on the server side for this.Javascript
1// Server-side
2socket.on("new message", (data) => {
3 socket.broadcast.emit("new message", data);
4});
5
To broadcast to all clients including the sender, use
io.emit()
as shown in the initial Node.js server setup example.Namespaces and Rooms
Namespaces and rooms allow you to organize connections into logical groups. Namespaces are like separate communication channels, while rooms are subsets of connections within a namespace. This makes it easier to target specific groups of clients without having to manually manage lists of socket IDs.
- Namespaces: Create separate Socket.IO instances on different paths.
- Rooms: Clients can join and leave rooms. Events can be emitted to specific rooms.
Here's an example of using rooms:
Javascript
1// Server-side
2io.on("connection", (socket) => {
3 socket.join("room1");
4
5 socket.on("new message", (data) => {
6 io.to("room1").emit("new message", data);
7 });
8});
9
10// Client-side
11socket.emit("new message", "Hello from room1!");
12
Advanced Socket.IO Usage
Beyond the basics, Socket.IO offers advanced features for building robust and scalable real-time applications.
Authentication and Authorization
Authentication and authorization are crucial for securing your Socket.IO applications. You can use various methods to authenticate clients, such as JWT (JSON Web Tokens) or session-based authentication. Once a client is authenticated, you can implement authorization rules to control access to specific resources or functionalities.
- Authentication: Verify the identity of the user connecting.
- Authorization: Determine what the authenticated user is allowed to do.
A common approach is to use middleware on the server to verify a token sent by the client upon connection. If the token is valid, the connection proceeds; otherwise, it's rejected.
Error Handling and Logging
Proper error handling and logging are essential for debugging and maintaining your Socket.IO applications. Implement error handling mechanisms to catch and handle exceptions. Use logging to track events, errors, and other relevant information. Implement try-catch blocks and
socket.on('error', ...)
to catch exceptions. For logging, consider using libraries like winston
or morgan
.Performance Optimization Techniques
To ensure optimal performance, consider the following optimization techniques:
- Minimize Data Transfer: Reduce the amount of data sent over the network.
- Use Compression: Compress data before sending it.
- Optimize Event Handling: Avoid unnecessary event listeners and handlers.
- Scale Horizontally: Distribute the load across multiple servers using a load balancer.
- Use Redis or other message broker: To scale socket.io across multiple instances you need to keep track of the connections with a message broker like redis.
Real-World Applications of Socket.IO
Socket.IO is suitable for various real-world applications that require real-time communication.
Chat Applications
Socket.IO is commonly used to build chat applications. It enables users to send and receive messages in real-time, creating a dynamic and interactive chat experience. The basic example provided above showcases the core functionality for real-time chat.
Real-time Collaboration Tools
Socket.IO can power real-time collaboration tools, such as collaborative document editors, project management tools, and whiteboarding applications. It allows multiple users to work together simultaneously and see each other's changes in real-time.
Gaming and Multiplayer Applications
Socket.IO is also used in gaming and multiplayer applications. It provides the real-time communication infrastructure needed for players to interact with each other and the game world.
Here is a basic architecture of a multiplayer game using socket.io
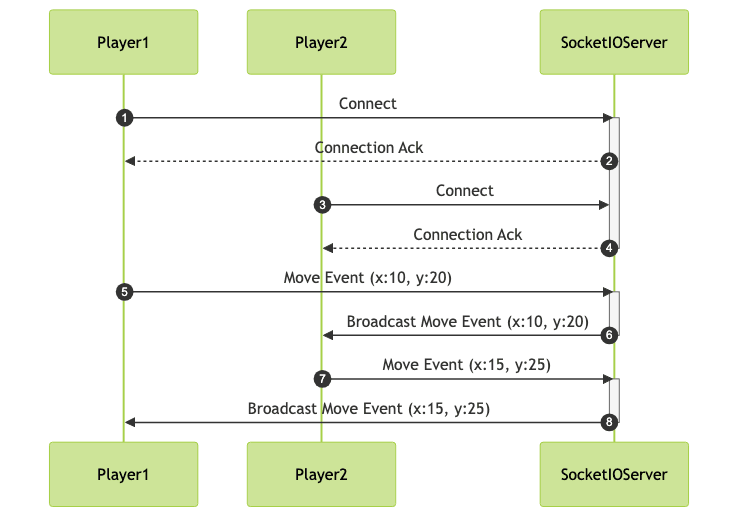
Security Considerations
Security is a critical aspect of Socket.IO application development. Here are some important considerations.
Preventing Cross-Site Scripting (XSS) Attacks
XSS attacks occur when malicious scripts are injected into web pages. To prevent XSS attacks, sanitize user input and escape output before rendering it in the browser. Do not trust data coming from the client. Libraries like
DOMPurify
can help sanitize HTML content.Protecting Against Injection Attacks
Injection attacks occur when malicious code is injected into database queries or other system commands. To protect against injection attacks, validate and sanitize user input before using it in queries or commands. Use parameterized queries or prepared statements to prevent SQL injection. Never trust data coming from the client.
Authentication and Authorization Best Practices
Implement robust authentication and authorization mechanisms to protect your Socket.IO applications. Use strong passwords, multi-factor authentication, and proper authorization rules. Regularly review and update your security practices.
Conclusion and Further Resources
Socket.IO empowers developers to create real-time applications with ease. By understanding its core concepts and applying best practices, you can build dynamic and interactive web experiences.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ