React Native Chatbot: A Comprehensive Guide
Introduction
This article provides a comprehensive guide to developing chatbots using React Native. We will explore various approaches, libraries, and APIs to help you build engaging and functional chatbots for your mobile applications. We will cover everything from choosing the right architecture to deploying your finished product.
Choosing the Right Chatbot Approach
There are several approaches to building a React Native chatbot, each with its own strengths and weaknesses. Choosing the right one depends on the complexity of your desired chatbot and your available resources.
Rule-Based Chatbots
Rule-based chatbots are the simplest type. They follow a predefined set of rules to respond to user input. These rules are typically based on keywords or phrases. They are easy to implement but can be inflexible and limited in their ability to handle complex conversations.
AI-Powered Chatbots (using Dialogflow, Rasa, etc.)
AI-powered chatbots use machine learning and natural language processing (NLP) to understand user intent and provide more natural and dynamic responses. These chatbots can handle a wider range of user inputs and can learn from past conversations to improve their performance. Services like Dialogflow, Rasa, and Amazon Lex provide powerful tools for building AI-powered chatbots. Building AI-powered chatbots often involves training NLP models with data.
Hybrid Approach
A hybrid approach combines the benefits of both rule-based and AI-powered chatbots. This approach can use rule-based logic for simple tasks and AI-powered NLP for more complex interactions. This approach offers a balance between simplicity and flexibility.
This section will discuss the advantages and disadvantages of each approach, guiding the reader towards selecting the best fit for their project needs and technical expertise. Consider the trade-offs between development time, complexity, and the desired level of sophistication when choosing your approach to
react native chatbot development
.Selecting a React Native Chatbot Library
Several React Native libraries can simplify the process of building a chatbot UI. These libraries provide pre-built components for displaying chat messages, handling user input, and managing the overall chat interface. Choosing the right library is essential for efficient
react native chatbot
development.React Native Gifted Chat
React Native Gifted Chat is a popular and well-maintained library that provides a complete chat UI solution. It includes features like typing indicators, image support, and location sharing. It's highly customizable and offers a great starting point for most
react native chat widget
implementations.1import { GiftedChat } from 'react-native-gifted-chat'
2
3const MyChatComponent = () => {
4 const [messages, setMessages] = React.useState([]);
5
6 React.useEffect(() => {
7 setMessages([
8 {
9 _id: 1,
10 text: 'Hello developer',
11 createdAt: new Date(),
12 user: {
13 _id: 2,
14 name: 'React Native Bot',
15 avatar: 'https://placeimg.com/140/140/any',
16 },
17 },
18 ])
19 }, [])
20
21 const onSend = React.useCallback((messages = []) => {
22 setMessages(previousMessages => GiftedChat.append(previousMessages, messages))
23 }, [])
24
25 return (
26 <GiftedChat
27 messages={messages}
28 onSend={messages => onSend(messages)}
29 user={{
30 _id: 1,
31 }}
32 />
33 )
34}
35
36export default MyChatComponent;
37
react-native-chatbot-messenger
A lightweight and flexible alternative, react-native-chatbot-messenger, allows more control over the UI but requires more manual setup. Great if you want a simple chat and want to build every component your self.
1import Chat from 'react-native-chatbot-messenger'
2
3const MyChatComponent = () => {
4 const messages = [
5 {
6 _id: 1,
7 text: 'Hello! How can I help you?',
8 createdAt: new Date(),
9 user: {
10 _id: 2,
11 name: 'Bot',
12 },
13 },
14 ];
15
16 return (
17 <Chat
18 messages={messages}
19 onSend={(newMessage) => console.log('New Message:', newMessage)}
20 user={{ _id: 1, name: 'User' }}
21 />
22 );
23};
24
25export default MyChatComponent;
26
Library 3: react-native-dialogflow
react-native-dialogflow helps you easily integrate Dialogflow into your React Native app. This library is specifically useful if you're building an
AI chatbot react native
solution powered by Dialogflow.Integrating with a Backend Service
While the React Native app handles the UI, a backend service is often necessary for managing complex logic, data persistence, and scaling. This is especially true when building chatbots that require accessing databases, performing calculations, or
integrating chatbot in react native
with other services.Here's a mermaid diagram showing the data flow:
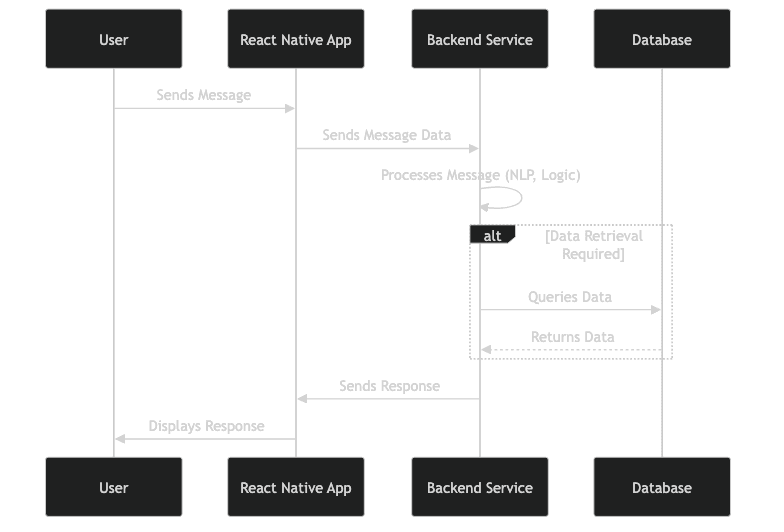
Connecting to Firebase
Firebase is a popular backend-as-a-service (BaaS) that provides a real-time database, authentication, and cloud functions. It's a great option for building scalable and serverless chatbots. You can create a
React Native Firebase Chatbot
to store your conversational data in Firebase Realtime Database or Firestore and use Cloud Functions to implement custom logic.1import firebase from '@react-native-firebase/app';
2import '@react-native-firebase/database';
3
4const sendMessageToFirebase = (message) => {
5 firebase.database().ref('messages').push({
6 text: message.text,
7 createdAt: firebase.database.ServerValue.TIMESTAMP,
8 user: {
9 _id: message.user._id,
10 },
11 });
12};
13
14// Example usage:
15sendMessageToFirebase({ text: 'Hello from React Native!', user: { _id: 1 } });
16
Connecting to a Custom Backend
For more control and customization, you can connect your React Native chatbot to a custom backend built with Node.js, Python, or another language. This approach requires more setup and maintenance but provides greater flexibility. You can use REST APIs or WebSockets to communicate between your React Native app and your backend. You can also handle the
react native chatbot with backend
entirely by yourself.Designing the User Interface
The user interface (UI) is a crucial aspect of any chatbot. A well-designed UI can make the chatbot more engaging, user-friendly, and effective.
Creating an Engaging Chat UI
Use clear and concise language. Design visually appealing chat bubbles. Implement smooth animations and transitions. Consider using different colors and fonts to differentiate between user messages and bot responses. Thoughtful
react native chatbot design
significantly impacts user experience.1import React from 'react';
2import { View, Text, StyleSheet } from 'react-native';
3
4const ChatBubble = ({ text, isUser }) => {
5 return (
6 <View style={[styles.bubble, isUser ? styles.userBubble : styles.botBubble]}>
7 <Text style={isUser ? styles.userText : styles.botText}>{text}</Text>
8 </View>
9 );
10};
11
12const styles = StyleSheet.create({
13 bubble: {
14 borderRadius: 20,
15 padding: 10,
16 marginBottom: 5,
17 },
18 userBubble: {
19 backgroundColor: '#DCF8C6',
20 alignSelf: 'flex-end',
21 },
22 botBubble: {
23 backgroundColor: '#E5E5EA',
24 alignSelf: 'flex-start',
25 },
26 userText: {
27 color: 'black',
28 },
29 botText: {
30 color: 'black',
31 },
32});
33
34export default ChatBubble;
35
Handling User Input and Bot Responses
Use a text input component to allow users to enter their messages. Display the user's message and the bot's response in a clear and organized manner. Provide visual feedback to the user, such as a loading indicator while the bot is processing their request.
1import React, { useState } from 'react';
2import { View, TextInput, Button, FlatList, Text } from 'react-native';
3
4const ChatScreen = () => {
5 const [messages, setMessages] = useState([]);
6 const [inputText, setInputText] = useState('');
7
8 const handleSend = () => {
9 if (inputText.trim() !== '') {
10 setMessages([...messages, { text: inputText, sender: 'user' }]);
11 // Simulate bot response (replace with actual bot logic)
12 setTimeout(() => {
13 setMessages([...messages, { text: `You said: ${inputText}`, sender: 'bot' }]);
14 }, 500);
15 setInputText('');
16 }
17 };
18
19 return (
20 <View>
21 <FlatList
22 data={messages}
23 renderItem={({ item }) => <Text>{item.sender}: {item.text}</Text>}
24 keyExtractor={(item, index) => index.toString()}
25 />
26 <TextInput
27 value={inputText}
28 onChangeText={setInputText}
29 placeholder="Type your message..."
30 />
31 <Button title="Send" onPress={handleSend} />
32 </View>
33 );
34};
35
36export default ChatScreen;
37
Implementing Advanced UI Features
Consider adding features like typing indicators, read receipts, and image/video support to enhance the user experience.
Integrating Natural Language Processing (NLP)
Natural language processing (NLP) is a crucial component of intelligent chatbots. NLP enables the chatbot to understand user intent, extract relevant information, and generate appropriate responses. By utilizing
NLP React Native
applications can provide more human-like conversations.Utilizing NLP APIs
Several NLP APIs are available, such as Dialogflow, Rasa, and Amazon Lex. These APIs provide pre-trained models for intent recognition, entity extraction, and sentiment analysis.
Dialogflow React Native Chatbot
, Rasa React Native Chatbot
, and Amazon Lex React Native Chatbot
integrations are common approaches.1// Example using Dialogflow (requires Dialogflow setup and API key)
2import dialogflow from '@react-native-firebase/dialogflow';
3
4const sendToDialogflow = async (text) => {
5 try {
6 const response = await dialogflow().requestQuery(text);
7 return response.queryResult.fulfillmentText;
8 } catch (error) {
9 console.error('Dialogflow error:', error);
10 return 'Sorry, I encountered an error.';
11 }
12};
13
14// Example usage:
15sendToDialogflow('What is the weather like today?').then(response => {
16 console.log('Dialogflow response:', response);
17});
18
Building Custom NLP Models
For advanced use cases, you can build custom NLP models using libraries like TensorFlow or PyTorch. This approach requires more expertise and resources but allows for greater control and customization. This path is often chosen for specialized tasks or when pre-trained models don't meet specific needs. The advanced aspect of developing a chatbot can involve custom
NLP React Native
solutions to tailor the chatbot behavior exactly to the need.Testing and Debugging
Thorough testing and debugging are essential for ensuring the chatbot's functionality and reliability. Test different scenarios, handle errors gracefully, and debug common issues such as incorrect intent recognition or unexpected responses.
Deployment
To
deploy react native chatbot
, follow these steps:- Build your React Native application for both iOS and Android platforms.
- Configure your backend service and ensure it is accessible from the internet.
- Submit your application to the App Store and Google Play Store.
Conclusion
This guide covered the essential aspects of building React Native chatbots. By leveraging the various libraries and APIs available, developers can create engaging and functional conversational interfaces for their mobile applications. Understanding concepts like
conversational AI react native
, and integrating libraries for handling LSI React Native Chatbot
functionalities can further enhance the chatbot's capabilities.Learn more about
React Native
ExploreDialogflow's capabilities
DiscoverRasa's open-source framework
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ