Introduction to "RTSPtoWeb WebRTC" Technology
In the world of real-time video streaming, the ability to convert RTSP streams to a format consumable by web browsers is a game-changer. This is where RTSPtoWeb WebRTC comes into play. Developed with Go, RTSPtoWeb is a powerful tool that bridges the gap between traditional RTSP streams and modern WebRTC technology, making live streaming more accessible and seamless.
What is RTSPtoWeb WebRTC?
RTSPtoWeb is an open-source project that allows developers to convert RTSP (Real-Time Streaming Protocol) streams into WebRTC (Web Real-Time Communication) streams. RTSP is a well-established protocol used for establishing and controlling media sessions between endpoints. However, it is not natively supported by web browsers. On the other hand, WebRTC is a cutting-edge technology designed for peer-to-peer communication within web browsers, providing low-latency and high-quality media streaming capabilities.
By using RTSPtoWeb, you can take advantage of the strengths of both protocols. RTSPtoWeb captures RTSP streams from IP cameras or other sources, processes them, and delivers them as WebRTC streams. This conversion ensures that your live video streams can be directly accessed and viewed in web browsers without the need for additional plugins or software.
Key Benefits of RTSPtoWeb WebRTC
Browser Compatibility
One of the primary advantages of using RTSPtoWeb WebRTC is its compatibility with all major web browsers. Since WebRTC is natively supported by browsers like Chrome, Firefox, and Safari, your audience can easily view your streams without any compatibility issues.
Low Latency
WebRTC is known for its low-latency performance, which is crucial for real-time applications such as surveillance, live events, and interactive broadcasts. By converting RTSP streams to WebRTC, RTSPtoWeb ensures that your viewers experience minimal delay.
Scalability
RTSPtoWeb is built with scalability in mind. Whether you are streaming to a handful of viewers or a large audience, the application can handle the load efficiently, making it suitable for various use cases, from small-scale deployments to enterprise-level solutions.
Open Source and Extensible
Being an open-source project, RTSPtoWeb allows developers to modify and extend its capabilities to fit their specific needs. The active community and comprehensive documentation make it easy to get started and contribute to the project.
In the following sections, we will dive deeper into how to set up and use RTSPtoWeb WebRTC, providing you with step-by-step instructions and practical code examples. Whether you're a seasoned developer or new to web streaming technologies, this guide will help you leverage the power of RTSPtoWeb to deliver high-quality, real-time video streams directly to your audience's browsers.
Getting Started with the Code!
In this section, we will guide you through the initial setup and basic configuration of the RTSPtoWeb WebRTC application. By the end of this part, you'll have a running project that you can build upon in the following steps.
Create a New RTSPtoWeb WebRTC App
Prerequisites
Before you begin, ensure you have the following installed on your system:
- Go (Golang) development environment
- Git
Cloning the Repository
First, you need to clone the RTSPtoWeb repository from GitHub. Open your terminal and execute the following command:
bash
1git clone https://github.com/deepch/RTSPtoWeb.git
2cd RTSPtoWeb
3
This command will clone the repository and navigate into the project directory.
Install
Installing Dependencies
Next, you'll need to install the necessary dependencies. The RTSPtoWeb project uses Go modules for dependency management. Run the following command to install the dependencies:
bash
1go mod tidy
2
This command will download and install all the required Go packages specified in the
go.mod
file.Structure of the Project
Understanding the structure of the project is crucial for effective development. Here's a brief overview of the key directories and files:
main.go
: The entry point of the application where the main function resides.pkg/
: Contains various packages used by the application.web/
: Holds the web assets such as HTML, CSS, and JavaScript files.config.json
: Configuration file for setting up RTSP streams and other parameters.
App Architecture
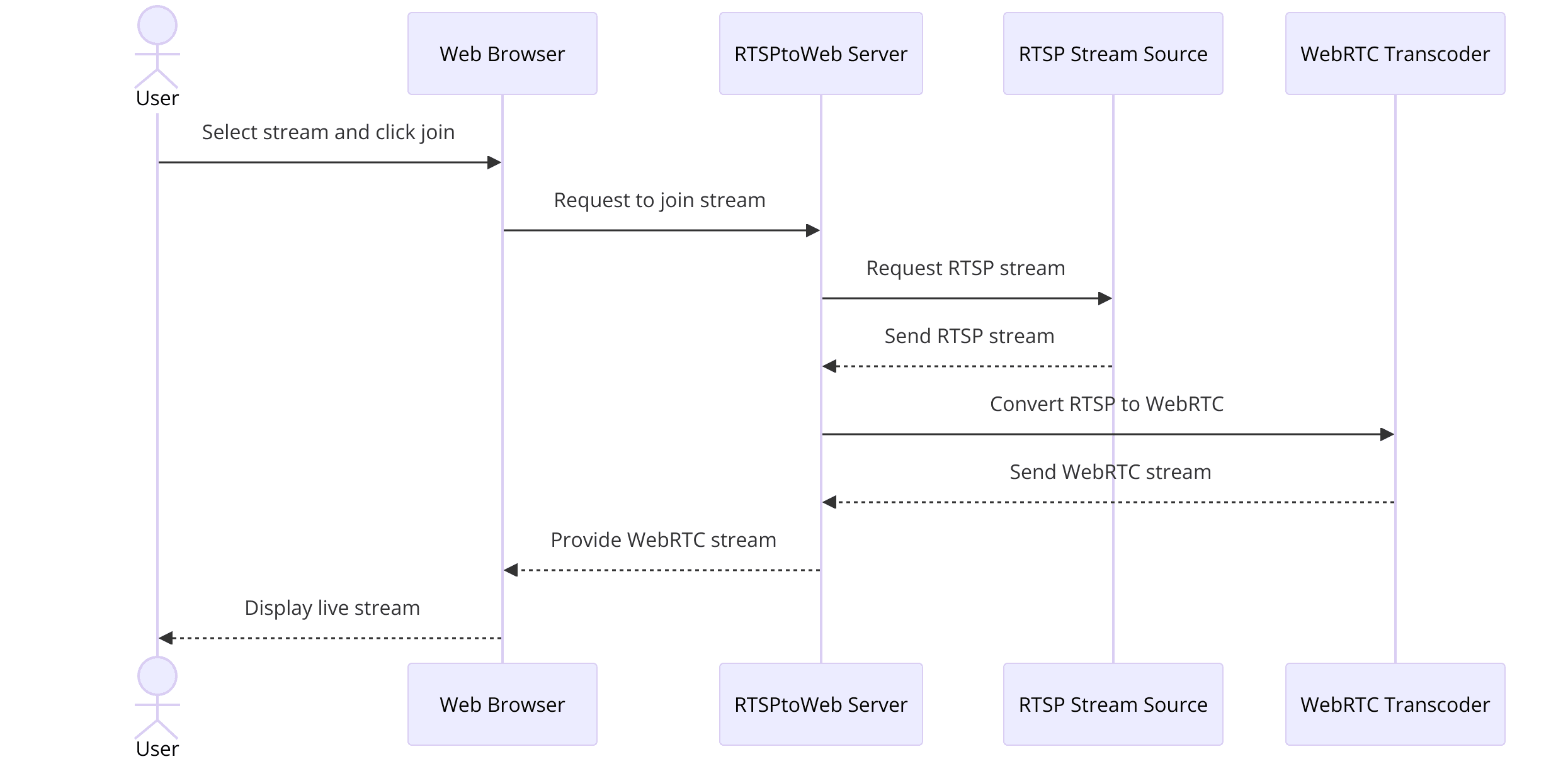
- RTSP Stream Capture: The application captures RTSP streams from configured sources (e.g., IP cameras).
- Stream Processing: The captured streams are processed and transcoded to WebRTC format.
- Web Server: A built-in web server serves the WebRTC streams to clients via a web interface.
Detailed Setup
[a] Configuration
Open the
config.json
file and configure your RTSP stream sources. Here is an example configuration:1 {
2 "streams": {
3 "example_stream": {
4 "url": "rtsp://username:password@camera_ip:554/stream",
5 "on_demand": false,
6 "disable_audio": false
7 }
8 }
9 }
10
[b] Running the Application
Once you've configured your streams, you can start the application by running:
bash
1 go run main.go
2
This command will start the RTSPtoWeb application, and you should see logs indicating that the server is running and the streams are being processed.
[c] Accessing the Web Interface
Open a web browser and navigate to
http://localhost:8083
. You should see the web interface where you can view the live WebRTC streams.By following these steps, you’ve set up the basic RTSPtoWeb WebRTC application. In the next sections, we will dive deeper into each component, adding features and refining the application to suit your needs.
Step 1: Get Started with "main.go"
In this section, we will delve into the
main.go
file, which serves as the entry point for the RTSPtoWeb WebRTC application. Setting up this file correctly is crucial for initializing and running the application smoothly.Setting up main.go
The
main.go
file is where the application's main function resides. This function is responsible for starting the application, initializing the necessary components, and setting up the server to handle RTSP to WebRTC conversion.Initial Setup of main.go
First, open the
main.go
file in your preferred code editor. The file should look something like this at the beginning:GO
1package main
2
3import (
4 "log"
5 "os"
6 "github.com/deepch/RTSPtoWeb/pkg/rtsp"
7 "github.com/deepch/RTSPtoWeb/pkg/webserver"
8)
9
10func main() {
11 // Load configuration
12 config, err := rtsp.LoadConfig("config.json")
13 if err != nil {
14 log.Fatalf("Error loading configuration: %v", err)
15 }
16
17 // Initialize RTSP server
18 rtspServer, err := rtsp.NewServer(config)
19 if err != nil {
20 log.Fatalf("Error initializing RTSP server: %v", err)
21 }
22
23 // Start the web server
24 err = webserver.Start(rtspServer, config)
25 if err != nil {
26 log.Fatalf("Error starting web server: %v", err)
27 }
28
29 log.Println("RTSPtoWeb WebRTC application started successfully.")
30}
31
Explanation of the Main Function
Package Declaration
The file starts by declaring the package
main
, which tells Go that this file is the entry point of the application.Importing Packages
The necessary packages are imported. This includes:
log
andos
for logging and interacting with the operating system.rtsp
andwebserver
from the RTSPtoWeb project for handling RTSP streams and the web server.
Loading Configuration
The
rtsp.LoadConfig
function loads the configuration from config.json
. If there is an error during this process, the application logs the error and terminates.Initializing RTSP Server
The
rtsp.NewServer
function initializes the RTSP server using the loaded configuration. This server will handle the incoming RTSP streams.Starting the Web Server
The
webserver.Start
function starts the web server, passing the RTSP server instance and configuration. This web server will convert the RTSP streams to WebRTC and serve them to clients.Logging Success
Finally, a log message is printed to indicate that the application has started successfully.
Key Components and Their Roles
Configuration Loading
The configuration file (
config.json
) holds essential settings for the application, including RTSP stream URLs and server parameters.RTSP Server Initialization
The RTSP server is responsible for capturing and processing RTSP streams from the configured sources.
Web Server
The web server component serves the WebRTC streams to clients via a web interface, enabling browser compatibility.
By setting up the
main.go
file correctly, you ensure that the RTSPtoWeb WebRTC application initializes and runs smoothly. In the next steps, we will focus on wireframing the components and implementing the user interface elements necessary for a functional streaming application.Step 2: Wireframe All the Components
In this section, we will outline the essential components needed for the RTSPtoWeb WebRTC application. Understanding the interaction between these components will help in structuring the application effectively and ensure a smooth development process.
Component Layout
To build a robust RTSPtoWeb WebRTC application, it's crucial to have a clear understanding of the main components and their interactions. Here, we will wireframe the primary components involved:
RTSP Stream Source
This is the origin of the video stream, typically an IP camera or any other RTSP-enabled device.
RTSP Server
Captures the RTSP stream from the source and prepares it for processing.
WebRTC Transcoder
Converts the RTSP stream into WebRTC format, ensuring it can be consumed by web browsers.
Web Server
Serves the WebRTC streams to clients through a web interface.
Web Interface (Client-Side)
The front-end interface where users can view and interact with the streams.
High-Level Overview of Interactions
RTSP Stream Source to RTSP Server
The RTSP server captures the video feed from the RTSP stream source. This involves establishing a connection and maintaining the stream.
RTSP Server to WebRTC Transcoder
The captured RTSP stream is passed to the WebRTC transcoder. This component handles the conversion process, ensuring the stream is compatible with WebRTC.
WebRTC Transcoder to Web Server
The transcoded stream is then forwarded to the web server. The web server is responsible for delivering the stream to the clients.
Web Server to Web Interface
Finally, the web server sends the WebRTC stream to the web interface, where it can be accessed and viewed by users in their browsers.
Implementing the Components
RTSP Stream Source
Typically configured in the
config.json
file. An example configuration might look like this:JSON
1 {
2 "streams": {
3 "example_stream": {
4 "url": "rtsp://username:password@camera_ip:554/stream",
5 "on_demand": false,
6 "disable_audio": false
7 }
8 }
9 }
10
RTSP Server Initialization in main.go
The RTSP server is initialized as shown in Part 3. This involves loading the configuration and setting up the server to capture streams.
WebRTC Transcoder Setup
The transcoder is typically integrated within the RTSP server setup. It handles the conversion internally, so no separate setup is needed in most cases.
Web Server Configuration in main.go
The web server is started using the configuration and the RTSP server instance. This is done in the
main.go
file:GO
1 // Start the web server
2 err = webserver.Start(rtspServer, config)
3 if err != nil {
4 log.Fatalf("Error starting web server: %v", err)
5 }
6
Creating the Web Interface
The web interface is usually built using HTML, CSS, and JavaScript. Here’s a basic example of what the HTML structure might look like:
HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTSPtoWeb WebRTC</title>
7 </head>
8 <body>
9 <h1>RTSP to WebRTC Stream</h1>
10 <div id="video-container">
11 <video id="video" autoplay playsinline></video>
12 </div>
13 <script src="webrtc.js"></script>
14 </body>
15 </html>
16
The
webrtc.js
file would contain the JavaScript code necessary to handle the WebRTC connection and stream the video.By wireframing all the components and understanding their interactions, you lay a solid foundation for building the RTSPtoWeb WebRTC application. In the next steps, we will focus on implementing specific features, starting with the join screen.
Step 3: Implement Join Screen
In this section, we will create the join screen for our RTSPtoWeb WebRTC application. The join screen is the initial interface where users can select and join a live stream. This involves setting up the HTML structure and adding the necessary JavaScript to handle user interactions.
Creating the Join Screen
The join screen will allow users to select from available RTSP streams and initiate the WebRTC connection. Here’s how to implement it:
[a] HTML Structure
First, let's create the HTML structure for the join screen. Add the following code to your
index.html
file:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTSPtoWeb WebRTC</title>
7 <style>
8 body {
9 font-family: Arial, sans-serif;
10 text-align: center;
11 margin-top: 50px;
12 }
13 #join-screen {
14 margin: 20px;
15 }
16 #video-container {
17 display: none;
18 }
19 </style>
20</head>
21<body>
22 <h1>Join a Live Stream</h1>
23 <div id="join-screen">
24 <label for="stream-select">Select Stream:</label>
25 <select id="stream-select">
26 <option value="example_stream">Example Stream</option>
27 <!-- Add more options here based on available streams -->
28 </select>
29 <button id="join-button">Join</button>
30 </div>
31 <div id="video-container">
32 <video id="video" autoplay playsinline></video>
33 </div>
34 <script src="webrtc.js"></script>
35</body>
36</html>
37
This HTML structure includes a simple form for selecting a stream and a button to join the selected stream. When the user clicks the "Join" button, the selected stream will be displayed in the video container.
[b] JavaScript Code
Next, we need to add the JavaScript code to handle the join button click event and establish the WebRTC connection. Create a new file named
webrtc.js
and add the following code:JavaScript
1document.getElementById('join-button').addEventListener('click', function() {
2 const streamName = document.getElementById('stream-select').value;
3 joinStream(streamName);
4});
5
6function joinStream(streamName) {
7 const videoContainer = document.getElementById('video-container');
8 const videoElement = document.getElementById('video');
9
10 // Show the video container
11 videoContainer.style.display = 'block';
12
13 // Replace with actual WebRTC setup code
14 const wsUrl = `wss://yourserver.com/ws/${streamName}`; // WebSocket URL for the WebRTC stream
15 const pc = new RTCPeerConnection();
16 const ws = new WebSocket(wsUrl);
17
18 ws.onmessage = (message) => {
19 const signal = JSON.parse(message.data);
20 if (signal.sdp) {
21 pc.setRemoteDescription(new RTCSessionDescription(signal.sdp)).then(() => {
22 if (signal.sdp.type === 'offer') {
23 pc.createAnswer().then(answer => {
24 pc.setLocalDescription(answer).then(() => {
25 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
26 });
27 });
28 }
29 });
30 } else if (signal.candidate) {
31 pc.addIceCandidate(new RTCIceCandidate(signal.candidate));
32 }
33 };
34
35 pc.onicecandidate = (event) => {
36 if (event.candidate) {
37 ws.send(JSON.stringify({ 'candidate': event.candidate }));
38 }
39 };
40
41 pc.ontrack = (event) => {
42 videoElement.srcObject = event.streams[0];
43 };
44
45 navigator.mediaDevices.getUserMedia({ video: true, audio: true }).then(stream => {
46 stream.getTracks().forEach(track => pc.addTrack(track, stream));
47 }).catch(error => {
48 console.error('Error accessing media devices.', error);
49 });
50
51 // Start WebRTC negotiation
52 pc.createOffer().then(offer => {
53 pc.setLocalDescription(offer).then(() => {
54 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
55 });
56 });
57}
58
Explanation of the JavaScript Code
Event Listener for Join Button
- When the user clicks the "Join" button, the
joinStream
function is called with the selected stream name.
Join Stream Function
- The
joinStream
function displays the video container and sets up the WebRTC connection. - The WebSocket connection to the server is established using the URL for the selected stream.
- The WebRTC peer connection (
RTCPeerConnection
) is created, and signaling messages are exchanged via WebSocket to establish the connection. - The video stream is set as the source for the video element in the HTML.
WebRTC Negotiation
- The
onmessage
handler processes incoming signaling messages to set up the WebRTC connection. - The
onicecandidate
handler sends ICE candidates to the server. - The
ontrack
handler sets the received video stream to the video element. - User media (camera and microphone) is accessed and added to the peer connection.
By implementing this join screen, you provide users with an interface to select and join live streams. In the next step, we will add controls to the interface to enhance user interaction with the streams.
Step 4: Implement Controls
Adding user controls to your RTSPtoWeb WebRTC application enhances user interaction and provides a better viewing experience. In this section, we will implement basic controls such as play, pause, and stop functionalities.
[a] HTML Structure for Controls
First, we need to update the HTML to include the control buttons. Modify your
index.html
file to add the controls within the video container:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTSPtoWeb WebRTC</title>
7 <style>
8 body {
9 font-family: Arial, sans-serif;
10 text-align: center;
11 margin-top: 50px;
12 }
13 #join-screen {
14 margin: 20px;
15 }
16 #video-container {
17 display: none;
18 margin-top: 20px;
19 }
20 #controls {
21 margin-top: 10px;
22 }
23 button {
24 margin: 0 10px;
25 }
26 </style>
27</head>
28<body>
29 <h1>Join a Live Stream</h1>
30 <div id="join-screen">
31 <label for="stream-select">Select Stream:</label>
32 <select id="stream-select">
33 <option value="example_stream">Example Stream</option>
34 <!-- Add more options here based on available streams -->
35 </select>
36 <button id="join-button">Join</button>
37 </div>
38 <div id="video-container">
39 <video id="video" autoplay playsinline controls></video>
40 <div id="controls">
41 <button id="play-button">Play</button>
42 <button id="pause-button">Pause</button>
43 <button id="stop-button">Stop</button>
44 </div>
45 </div>
46 <script src="webrtc.js"></script>
47</body>
48</html>
49
This HTML structure includes play, pause, and stop buttons inside a
div
with the id controls
.[b] JavaScript Code for Controls
Next, we need to add JavaScript code to handle these control buttons. Update your
webrtc.js
file with the following code:JavaScript
1document.getElementById('join-button').addEventListener('click', function() {
2 const streamName = document.getElementById('stream-select').value;
3 joinStream(streamName);
4});
5
6document.getElementById('play-button').addEventListener('click', function() {
7 const videoElement = document.getElementById('video');
8 videoElement.play();
9});
10
11document.getElementById('pause-button').addEventListener('click', function() {
12 const videoElement = document.getElementById('video');
13 videoElement.pause();
14});
15
16document.getElementById('stop-button').addEventListener('click', function() {
17 const videoElement = document.getElementById('video');
18 videoElement.pause();
19 videoElement.currentTime = 0; // Reset the video to the beginning
20});
21
22function joinStream(streamName) {
23 const videoContainer = document.getElementById('video-container');
24 const videoElement = document.getElementById('video');
25
26 // Show the video container
27 videoContainer.style.display = 'block';
28
29 // Replace with actual WebRTC setup code
30 const wsUrl = `wss://yourserver.com/ws/${streamName}`; // WebSocket URL for the WebRTC stream
31 const pc = new RTCPeerConnection();
32 const ws = new WebSocket(wsUrl);
33
34 ws.onmessage = (message) => {
35 const signal = JSON.parse(message.data);
36 if (signal.sdp) {
37 pc.setRemoteDescription(new RTCSessionDescription(signal.sdp)).then(() => {
38 if (signal.sdp.type === 'offer') {
39 pc.createAnswer().then(answer => {
40 pc.setLocalDescription(answer).then(() => {
41 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
42 });
43 });
44 }
45 });
46 } else if (signal.candidate) {
47 pc.addIceCandidate(new RTCIceCandidate(signal.candidate));
48 }
49 };
50
51 pc.onicecandidate = (event) => {
52 if (event.candidate) {
53 ws.send(JSON.stringify({ 'candidate': event.candidate }));
54 }
55 };
56
57 pc.ontrack = (event) => {
58 videoElement.srcObject = event.streams[0];
59 };
60
61 navigator.mediaDevices.getUserMedia({ video: true, audio: true }).then(stream => {
62 stream.getTracks().forEach(track => pc.addTrack(track, stream));
63 }).catch(error => {
64 console.error('Error accessing media devices.', error);
65 });
66
67 // Start WebRTC negotiation
68 pc.createOffer().then(offer => {
69 pc.setLocalDescription(offer).then(() => {
70 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
71 });
72 });
73}
74
Explanation of the JavaScript Code
Event Listeners for Control Buttons
- Play Button: Resumes the video playback by calling the
play
method on the video element. - Pause Button: Pauses the video playback by calling the
pause
method on the video element. - Stop Button: Pauses the video and resets the current time to the beginning, effectively stopping the video.
Join Stream Function
- The
joinStream
function remains the same as previously described. It handles the WebRTC setup and streams the video to the video element.
By implementing these controls, you provide users with the ability to control the playback of the live stream, enhancing their viewing experience. In the next step, we will focus on building the participant view to handle multiple viewers and improve the application's overall functionality.
Step 5: Implement Participant View
In this section, we will enhance the RTSPtoWeb WebRTC application by implementing a participant view. This will allow multiple viewers to join and watch the same stream concurrently, offering a more interactive and scalable streaming experience.
HTML Structure for Participant View
We will extend the existing HTML to support multiple participants. Modify your
index.html
file to include a list of participants and a container for their streams:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>RTSPtoWeb WebRTC</title>
7 <style>
8 body {
9 font-family: Arial, sans-serif;
10 text-align: center;
11 margin-top: 50px;
12 }
13 #join-screen {
14 margin: 20px;
15 }
16 #video-container {
17 display: none;
18 margin-top: 20px;
19 }
20 #controls {
21 margin-top: 10px;
22 }
23 button {
24 margin: 0 10px;
25 }
26 #participants {
27 display: flex;
28 flex-wrap: wrap;
29 justify-content: center;
30 margin-top: 20px;
31 }
32 .participant {
33 margin: 10px;
34 }
35 video {
36 max-width: 100%;
37 }
38 </style>
39</head>
40<body>
41 <h1>Join a Live Stream</h1>
42 <div id="join-screen">
43 <label for="stream-select">Select Stream:</label>
44 <select id="stream-select">
45 <option value="example_stream">Example Stream</option>
46 <!-- Add more options here based on available streams -->
47 </select>
48 <button id="join-button">Join</button>
49 </div>
50 <div id="video-container">
51 <video id="video" autoplay playsinline controls></video>
52 <div id="controls">
53 <button id="play-button">Play</button>
54 <button id="pause-button">Pause</button>
55 <button id="stop-button">Stop</button>
56 </div>
57 </div>
58 <div id="participants"></div>
59 <script src="webrtc.js"></script>
60</body>
61</html>
62
In this structure, the
#participants
div will hold individual video elements for each participant.JavaScript Code for Participant View
Now, we need to update our JavaScript to handle multiple participants. Modify your
webrtc.js
file with the following code:JavaScript
1document.getElementById('join-button').addEventListener('click', function() {
2 const streamName = document.getElementById('stream-select').value;
3 joinStream(streamName);
4});
5
6document.getElementById('play-button').addEventListener('click', function() {
7 const videoElement = document.getElementById('video');
8 videoElement.play();
9});
10
11document.getElementById('pause-button').addEventListener('click', function() {
12 const videoElement = document.getElementById('video');
13 videoElement.pause();
14});
15
16document.getElementById('stop-button').addEventListener('click', function() {
17 const videoElement = document.getElementById('video');
18 videoElement.pause();
19 videoElement.currentTime = 0; // Reset the video to the beginning
20});
21
22function joinStream(streamName) {
23 const videoContainer = document.getElementById('video-container');
24 const videoElement = document.getElementById('video');
25 const participantsContainer = document.getElementById('participants');
26
27 // Show the video container
28 videoContainer.style.display = 'block';
29
30 // Replace with actual WebRTC setup code
31 const wsUrl = `wss://yourserver.com/ws/${streamName}`; // WebSocket URL for the WebRTC stream
32 const pc = new RTCPeerConnection();
33 const ws = new WebSocket(wsUrl);
34
35 ws.onmessage = (message) => {
36 const signal = JSON.parse(message.data);
37 if (signal.sdp) {
38 pc.setRemoteDescription(new RTCSessionDescription(signal.sdp)).then(() => {
39 if (signal.sdp.type === 'offer') {
40 pc.createAnswer().then(answer => {
41 pc.setLocalDescription(answer).then(() => {
42 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
43 });
44 });
45 }
46 });
47 } else if (signal.candidate) {
48 pc.addIceCandidate(new RTCIceCandidate(signal.candidate));
49 }
50 };
51
52 pc.onicecandidate = (event) => {
53 if (event.candidate) {
54 ws.send(JSON.stringify({ 'candidate': event.candidate }));
55 }
56 };
57
58 pc.ontrack = (event) => {
59 videoElement.srcObject = event.streams[0];
60 addParticipantView(event.streams[0]);
61 };
62
63 navigator.mediaDevices.getUserMedia({ video: true, audio: true }).then(stream => {
64 stream.getTracks().forEach(track => pc.addTrack(track, stream));
65 }).catch(error => {
66 console.error('Error accessing media devices.', error);
67 });
68
69 // Start WebRTC negotiation
70 pc.createOffer().then(offer => {
71 pc.setLocalDescription(offer).then(() => {
72 ws.send(JSON.stringify({ 'sdp': pc.localDescription }));
73 });
74 });
75}
76
77function addParticipantView(stream) {
78 const participantsContainer = document.getElementById('participants');
79 const participantDiv = document.createElement('div');
80 participantDiv.className = 'participant';
81 const participantVideo = document.createElement('video');
82 participantVideo.autoplay = true;
83 participantVideo.playsinline = true;
84 participantVideo.srcObject = stream;
85 participantDiv.appendChild(participantVideo);
86 participantsContainer.appendChild(participantDiv);
87}
88
Explanation of the JavaScript Code
Event Listeners for Control Buttons
- Play Button: Resumes the video playback by calling the
play
method on the video element. - Pause Button: Pauses the video playback by calling the
pause
method on the video element. - Stop Button: Pauses the video and resets the current time to the beginning, effectively stopping the video.
Join Stream Function
- The
joinStream
function displays the video container and sets up the WebRTC connection. - A WebSocket connection is established to the server using the URL for the selected stream.
- The WebRTC peer connection (
RTCPeerConnection
) is created, and signaling messages are exchanged via WebSocket to establish the connection. - The video stream is set as the source for the video element, and the
addParticipantView
function is called to add the stream to the participant view.
Add Participant View Function
- This function creates a new
div
for each participant, including avideo
element to display their stream. - The participant view is appended to the
#participants
container, allowing multiple viewers to be displayed concurrently.
By implementing the participant view, you enable multiple viewers to join and watch the same stream, enhancing the interactivity and scalability of your RTSPtoWeb WebRTC application. In the next step, we will focus on running your code and troubleshooting any common issues.
Step 6: Run Your Code Now
Now that you have set up the RTSPtoWeb WebRTC application with a join screen, user controls, and participant view, it’s time to run your code and see everything in action. This section will guide you through the process of running the application and troubleshooting common issues.
Running the Application
Start the RTSPtoWeb Application
Ensure you have your Go environment set up and dependencies installed as mentioned in Part 2. Then, run the application with the following command:
bash
1 go run main.go
2
You should see output indicating that the RTSPtoWeb server is running and ready to accept connections.
Access the Web Interface
Open a web browser and navigate to
http://localhost:8083
. You should see the join screen where you can select a stream and click the "Join" button to start watching the live stream.Interacting with the Stream
- Play: Click the "Play" button to resume playback if the video is paused.
- Pause: Click the "Pause" button to pause the video stream.
- Stop: Click the "Stop" button to stop the video and reset it to the beginning.
Troubleshooting Common Issues
Server Not Starting
If the server fails to start, check the following:
- Ensure Go is installed correctly and your environment variables are set up.
- Verify that you have installed all dependencies using
go mod tidy
. - Check the
config.json
file for any syntax errors or incorrect configurations.
WebSocket Connection Issues
If you encounter WebSocket connection issues:
- Ensure that the WebSocket URL in the
webrtc.js
file is correct and matches your server’s address. - Verify that your server is running and accessible from the browser.
No Video Stream
If the video stream is not appearing:
- Ensure your RTSP stream source is working correctly and accessible.
- Check the browser console for any errors related to WebRTC or media devices.
- Make sure the
addParticipantView
function is correctly adding the video elements to the DOM.
Cross-Origin Resource Sharing (CORS) Issues
If you encounter CORS issues:
- Make sure your server is configured to allow CORS requests from your web application’s domain.
Conclusion
Congratulations! You have successfully set up the RTSPtoWeb WebRTC application. By following the steps outlined in this guide, you now have a functional application that allows multiple users to join and watch live RTSP streams through their web browsers. This setup provides a robust and scalable solution for real-time streaming applications.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ