What is OpenVidu?
OpenVidu is an open-source platform that simplifies the integration of real-time video communications into web and mobile applications. Leveraging the power of WebRTC (Web Real-Time Communication),
OpenVidu
allows developers to easily add video conferencing, live streaming, and video recording features to their applications. With its user-friendly API and robust feature set, OpenVidu has become a popular choice for businesses and developers looking to implement high-quality video communication solutions.Importance of WebRTC in Modern Web Applications
WebRTC is a cutting-edge technology that enables peer-to-peer communication directly between browsers without the need for plugins or external software. This makes it possible to transmit audio, video, and data seamlessly, providing a foundation for a wide range of applications such as video conferencing, file sharing, and real-time chat. By using WebRTC, developers can create interactive and engaging user experiences that work across different devices and platforms.
Overview of Kurento as the Underlying Technology
Kurento is a powerful media server that acts as the backbone of OpenVidu. It provides advanced media processing capabilities, including real-time filtering, recording, and broadcasting. Kurento supports a variety of media codecs and protocols, ensuring compatibility with a wide range of devices and applications. By integrating Kurento, OpenVidu enhances the capabilities of WebRTC, offering features like media transcoding, composite streams, and more.
With OpenVidu and Kurento, developers can build sophisticated video communication applications that are scalable, reliable, and easy to manage. This combination of technologies provides a flexible and powerful solution for implementing real-time video features in any application.
Let`s Start to Build OpenVidu WebRTC Application
Create a New OpenVidu App
To get started with OpenVidu, the first step is to create a new project. Whether you're building a web or mobile application, setting up your project correctly is crucial for a smooth development process. Here’s a simple guide to create a new OpenVidu application:
Set Up Your Development Environment
Ensure you have Node.js installed on your system. You can download it from
Node.js official website
.Install Git for version control from
Git official website
.Clone the OpenVidu Project:
Open your terminal and run the following command to clone the OpenVidu tutorials repository:
bash
1 git clone https://github.com/OpenVidu/openvidu-tutorials.git
2
- Navigate to the cloned directory:
bash cd openvidu-tutorials
Install OpenVidu
With the project set up, the next step is to install the necessary dependencies. This involves setting up the OpenVidu server and the client-side libraries.
Install Dependencies:
Navigate to the specific tutorial or example you want to run, for instance, the
openvidu-getaroom
example:bash
1 cd openvidu-getaroom
2
- Install the required Node.js packages using npm:
bash npm install
Set Up OpenVidu Server
Download the OpenVidu server from the
OpenVidu Downloads page
.Start the server with the following command:
bash
1 ./openvidu start
2
Structure of the Project
Understanding the structure of your OpenVidu project is essential for efficient development. Here’s a breakdown of the typical project structure:
- public/: Contains static files like HTML, CSS, and client-side JavaScript.
- src/: Contains server-side code, usually written in JavaScript or TypeScript.
- package.json: Lists project dependencies and scripts.
- openvidu-config.js: Configuration file for OpenVidu settings.
App Architecture
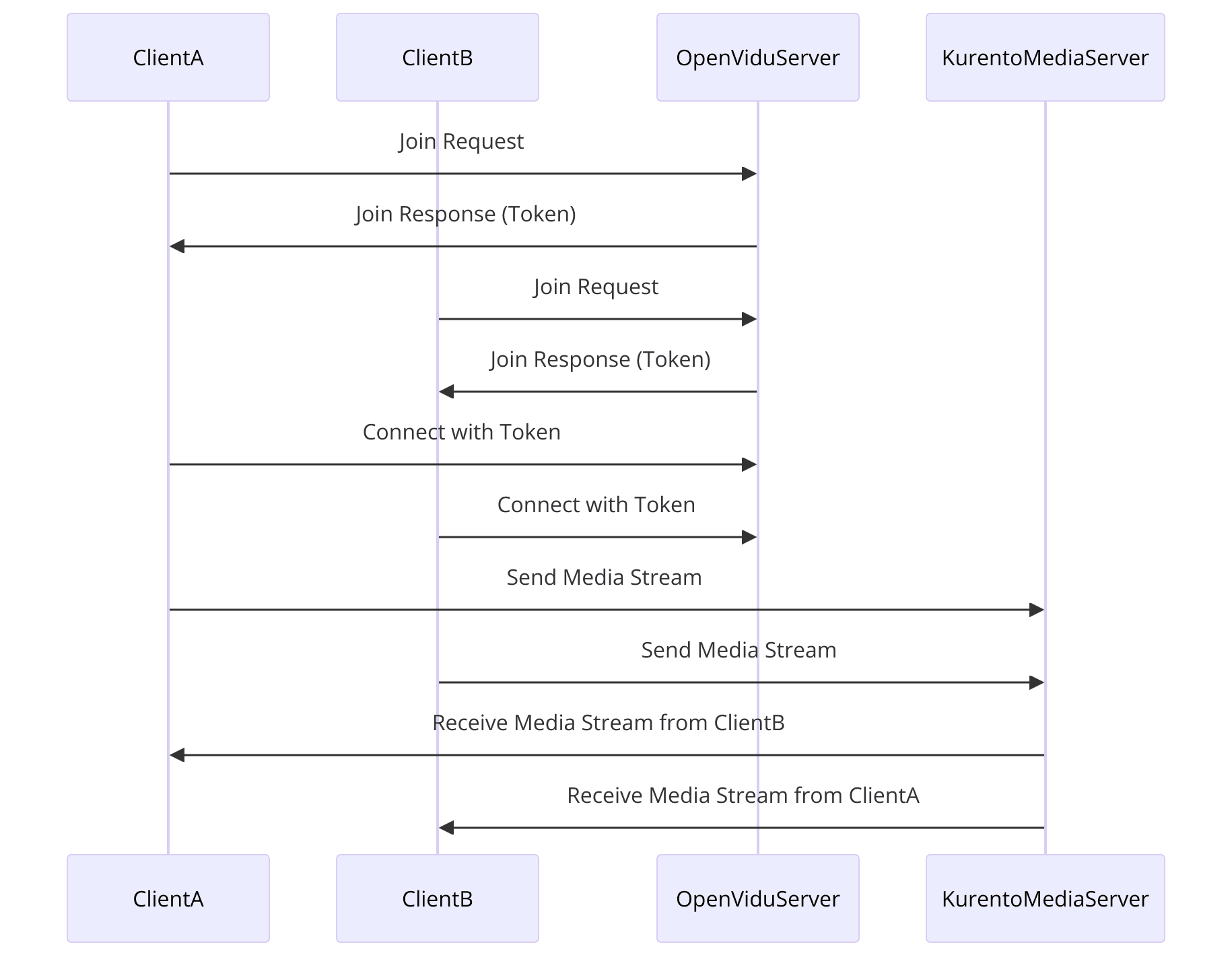
Client-Side Components
- HTML and CSS: Define the user interface of your application.
- JavaScript: Handles the integration with OpenVidu, including setting up video streams and managing user interactions.
Server-Side Components
- Express.js: A web application framework for handling HTTP requests and serving the client-side files.
- OpenVidu Node.js SDK: Provides methods to interact with the OpenVidu server, manage sessions, and handle tokens.
OpenVidu Server
- Acts as the signaling server for WebRTC connections.
- Manages media streams and coordinates communication between clients.
By following this structure, you can ensure that your OpenVidu application is well-organized and maintainable, making it easier to implement advanced features and scale your application as needed.
Step 1: Setting Up the Environment
Get Started with index.html
Setting up the environment for your OpenVidu application begins with creating and configuring your main HTML file,
index.html
. This file serves as the entry point for your application, defining the structure and elements of your web interface. Follow these steps to set up your index.html
and include the necessary components for integrating OpenVidu.Create the index.html
File
In the
public
directory of your project, create a new file named index.html
.Basic HTML Structure
Start by adding the basic HTML structure to your file:
HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>OpenVidu Video Call</title>
7 <link rel="stylesheet" href="styles.css">
8 </head>
9 <body>
10 <div id="video-container"></div>
11 <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
12 <script src="https://unpkg.com/@openvidu/openvidu-browser"></script>
13 <script src="app.js"></script>
14 </body>
15 </html>
16
Basic HTML Structure for Integrating OpenVidu
The basic structure includes a
<div>
element to hold the video streams, links to necessary CSS and JavaScript files, and references to OpenVidu libraries.Add Video Container
Within the
<body>
tag, add a container to hold the video streams:HTML
1 <div id="video-container">
2 <!-- Local and remote video streams will be appended here -->
3 </div>
4
Link to CSS for Styling
Reference a CSS file (e.g.,
styles.css
) to style your application. This file will define the layout and appearance of your video container and other UI elements.Include jQuery and OpenVidu Browser Library
Add script tags to include jQuery and the OpenVidu Browser library. These libraries provide essential functionalities for handling WebRTC connections and user interactions.
Reference Your Main JavaScript File
Link to your main JavaScript file (e.g.,
app.js
) where you will write the logic for connecting to the OpenVidu server and managing video streams.Setting Up the Project Directory
Ensure your project directory is well-organized, with separate folders for HTML, CSS, and JavaScript files. A typical structure might look like this:
1/public
2 /css
3 styles.css
4 /js
5 app.js
6 index.html
7package.json
8server.js
9
Configuring app.js
The
app.js
file is where you will implement the logic for your OpenVidu application. Start by initializing the OpenVidu session and setting up the connection.Initialize OpenVidu
Add the following code to
app.js
to initialize the OpenVidu object and set up a session:JavaScript
1 const OV = new OpenVidu();
2 let session = OV.initSession();
3
Connect to the Session
Implement the logic to connect to the session and handle events like stream creation and participant joining:
JavaScript
1 session.on('streamCreated', (event) => {
2 const subscriber = session.subscribe(event.stream, 'video-container');
3 subscriber.on('videoElementCreated', (event) => {
4 event.element.play();
5 });
6 });
7
8 // Function to get token from the server
9 getToken().then(token => {
10 session.connect(token)
11 .then(() => {
12 // Connection successful
13 })
14 .catch(error => {
15 console.error('Error connecting to the session:', error);
16 });
17 });
18
19 function getToken() {
20 return fetch('/api/get-token')
21 .then(response => response.json())
22 .then(data => data.token);
23 }
24
By following these steps, you will have a basic setup for your OpenVidu application, with a structured HTML file and the initial configuration in place. This setup will serve as the foundation for adding more advanced features and functionalities in the subsequent steps.
In the next part, we will move on to wireframing all components and designing the user interface for your video call application.
Step 2: Wireframe All Components
Designing the User Interface
Creating a well-designed user interface (UI) is crucial for ensuring a smooth and intuitive experience for users of your OpenVidu application. In this step, we will design the layout for the video call interface, add placeholders for video elements, and style the interface with CSS.
Creating the Layout for the Video Call Interface
To start, we need to set up a basic structure for the video call interface within the
index.html
file. This involves defining elements for displaying video streams, controls, and other UI components.Define the Video Container
Add the following HTML structure within the
<body>
tag of index.html
:HTML
1 <div id="video-container">
2 <div id="local-video" class="video-box"></div>
3 <div id="remote-videos" class="video-box"></div>
4 </div>
5 <div id="controls">
6 <button id="mute-audio">Mute Audio</button>
7 <button id="disable-video">Disable Video</button>
8 <button id="share-screen">Share Screen</button>
9 </div>
10
Add CSS for Layout and Styling
Create a CSS file (
styles.css
) in the public/css
directory and link it in index.html
:HTML
1 <link rel="stylesheet" href="css/styles.css">
2
Define the styles in
styles.css
to position and style the video containers and controls:CSS
1 body {
2 font-family: Arial, sans-serif;
3 display: flex;
4 flex-direction: column;
5 align-items: center;
6 justify-content: center;
7 height: 100vh;
8 margin: 0;
9 background-color: #f0f0f0;
10 }
11
12 #video-container {
13 display: flex;
14 flex-wrap: wrap;
15 justify-content: center;
16 width: 80%;
17 margin-bottom: 20px;
18 }
19
20 .video-box {
21 width: 45%;
22 margin: 10px;
23 background-color: #000;
24 position: relative;
25 }
26
27 #controls {
28 display: flex;
29 justify-content: center;
30 gap: 10px;
31 }
32
33 button {
34 padding: 10px 20px;
35 font-size: 16px;
36 cursor: pointer;
37 background-color: #007bff;
38 color: #fff;
39 border: none;
40 border-radius: 5px;
41 }
42
43 button:hover {
44 background-color: #0056b3;
45 }
46
Adding Placeholders for Video Elements
The placeholders for the video elements will help us visualize where the local and remote video streams will be displayed.
Local Video Placeholder
The
#local-video
div will be used to display the local video stream:HTML
1 <div id="local-video" class="video-box"></div>
2
Remote Videos Placeholder
The
#remote-videos
div will hold the video streams of remote participants:HTML
1 <div id="remote-videos" class="video-box"></div>
2
Styling the Interface with CSS
Good styling improves the usability and aesthetics of your application. The CSS defined earlier styles the video containers and control buttons. You can further customize the styles to match your application’s theme and branding.
Video Box Styling
Each video box is given a fixed width, margin, and background color to distinguish them:
CSS
1 .video-box {
2 width: 45%;
3 margin: 10px;
4 background-color: #000;
5 position: relative;
6 }
7
Control Buttons Styling
Control buttons are styled to be visually appealing and user-friendly:
CSS
1 button {
2 padding: 10px 20px;
3 font-size: 16px;
4 cursor: pointer;
5 background-color: #007bff;
6 color: #fff;
7 border: none;
8 border-radius: 5px;
9 }
10
11 button:hover {
12 background-color: #0056b3;
13 }
14
Bringing It All Together
With the HTML structure in place and the CSS styles defined, your application's interface is now ready to display video streams and provide user controls. This setup ensures a clean and responsive layout, enhancing the overall user experience.
HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>OpenVidu Video Call</title>
7 <link rel="stylesheet" href="css/styles.css">
8</head>
9<body>
10 <div id="video-container">
11 <div id="local-video" class="video-box"></div>
12 <div id="remote-videos" class="video-box"></div>
13 </div>
14 <div id="controls">
15 <button id="mute-audio">Mute Audio</button>
16 <button id="disable-video">Disable Video</button>
17 <button id="share-screen">Share Screen</button>
18 </div>
19 <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
20 <script src="https://unpkg.com/@openvidu/openvidu-browser"></script>
21 <script src="js/app.js"></script>
22</body>
23</html>
24
In the next part, we will implement the functionality for the join screen, allowing users to connect to the OpenVidu session and start their video calls. This will involve adding JavaScript to handle user input and OpenVidu session management.
Step 3: Implementing the Join Screen
Creating the Join Screen
The join screen is the first interaction point for users when they enter your video call application. It allows users to input their name and join the session. This step involves creating the HTML, CSS, and JavaScript necessary to implement the join screen functionality.
HTML and CSS for the Join Screen
First, we'll create the HTML structure and CSS styles for the join screen.
Add Join Screen HTML
Modify the
index.html
file to include the join screen elements:HTML
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>OpenVidu Video Call</title>
7 <link rel="stylesheet" href="css/styles.css">
8 </head>
9 <body>
10 <div id="join-screen">
11 <h2>Join the Video Call</h2>
12 <input type="text" id="username" placeholder="Enter your name">
13 <button id="join-button">Join</button>
14 </div>
15 <div id="video-screen" style="display: none;">
16 <div id="video-container">
17 <div id="local-video" class="video-box"></div>
18 <div id="remote-videos" class="video-box"></div>
19 </div>
20 <div id="controls">
21 <button id="mute-audio">Mute Audio</button>
22 <button id="disable-video">Disable Video</button>
23 <button id="share-screen">Share Screen</button>
24 </div>
25 </div>
26 <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
27 <script src="https://unpkg.com/@openvidu/openvidu-browser"></script>
28 <script src="js/app.js"></script>
29 </body>
30 </html>
31
Add Join Screen CSS
Define styles for the join screen in
styles.css
:CSS
1 #join-screen {
2 display: flex;
3 flex-direction: column;
4 align-items: center;
5 justify-content: center;
6 height: 100vh;
7 background-color: #f0f0f0;
8 }
9
10 #join-screen h2 {
11 margin-bottom: 20px;
12 font-size: 24px;
13 color: #333;
14 }
15
16 #join-screen input {
17 padding: 10px;
18 font-size: 16px;
19 margin-bottom: 20px;
20 width: 80%;
21 max-width: 300px;
22 border: 1px solid #ccc;
23 border-radius: 5px;
24 }
25
26 #join-screen button {
27 padding: 10px 20px;
28 font-size: 16px;
29 cursor: pointer;
30 background-color: #007bff;
31 color: #fff;
32 border: none;
33 border-radius: 5px;
34 }
35
36 #join-screen button:hover {
37 background-color: #0056b3;
38 }
39
Adding Functionality with JavaScript
Now, let's add the JavaScript to handle user input and manage the transition from the join screen to the video call screen.
Handle User Input and Join Button
Update the
app.js
file to add event listeners and functions for handling the join process:JavaScript
1 document.addEventListener('DOMContentLoaded', () => {
2 const joinButton = document.getElementById('join-button');
3 const usernameInput = document.getElementById('username');
4 const joinScreen = document.getElementById('join-screen');
5 const videoScreen = document.getElementById('video-screen');
6
7 joinButton.addEventListener('click', () => {
8 const username = usernameInput.value.trim();
9 if (username) {
10 joinScreen.style.display = 'none';
11 videoScreen.style.display = 'block';
12 initializeSession(username);
13 } else {
14 alert('Please enter your name.');
15 }
16 });
17 });
18
19 function initializeSession(username) {
20 const OV = new OpenVidu();
21 const session = OV.initSession();
22
23 session.on('streamCreated', (event) => {
24 const subscriber = session.subscribe(event.stream, 'remote-videos');
25 subscriber.on('videoElementCreated', (event) => {
26 event.element.play();
27 });
28 });
29
30 getToken().then(token => {
31 session.connect(token, { clientData: username })
32 .then(() => {
33 // Create a publisher for the local video
34 const publisher = OV.initPublisher('local-video');
35 session.publish(publisher);
36 })
37 .catch(error => {
38 console.error('Error connecting to the session:', error);
39 });
40 });
41
42 function getToken() {
43 return fetch('/api/get-token')
44 .then(response => response.json())
45 .then(data => data.token);
46 }
47 }
48
Connecting to the OpenVidu Server
In the
initializeSession
function, we connect to the OpenVidu server using a token. This token is obtained from your server backend, which handles the authentication and session creation.Backend Token Generation
Ensure your backend API
/api/get-token
generates and returns a valid OpenVidu token. This typically involves creating a session and generating a token for that session.By following these steps, you have implemented a functional join screen for your OpenVidu application. Users can enter their name and join the video call, transitioning from the join screen to the main video interface. The JavaScript code handles user input, initializes the OpenVidu session, and manages video streams.
In the next part, we will focus on implementing the controls for the video call, allowing users to mute/unmute audio, enable/disable video, and share their screen. This will enhance the functionality and user experience of your OpenVidu application.
Step 4: Implementing Controls
Adding Video Controls
In this step, we will implement the controls for the video call, allowing users to mute/unmute audio, enable/disable video, and share their screen. These controls are essential for providing a complete and user-friendly video conferencing experience.
HTML for Controls
The HTML structure for the controls has already been defined in the
index.html
file. We have buttons for muting audio, disabling video, and sharing the screen:HTML
1<div id="controls">
2 <button id="mute-audio">Mute Audio</button>
3 <button id="disable-video">Disable Video</button>
4 <button id="share-screen">Share Screen</button>
5</div>
6
JavaScript for Control Functionality
Next, we will add the JavaScript code to handle the functionality of these buttons. This involves attaching event listeners to the buttons and implementing the corresponding actions.
Initialize Publisher and Controls
Update the
initializeSession
function in app.js
to include event listeners for the control buttons:JavaScript
1 function initializeSession(username) {
2 const OV = new OpenVidu();
3 const session = OV.initSession();
4
5 session.on('streamCreated', (event) => {
6 const subscriber = session.subscribe(event.stream, 'remote-videos');
7 subscriber.on('videoElementCreated', (event) => {
8 event.element.play();
9 });
10 });
11
12 getToken().then(token => {
13 session.connect(token, { clientData: username })
14 .then(() => {
15 // Create a publisher for the local video
16 const publisher = OV.initPublisher('local-video', {
17 audioSource: undefined, // The source of audio. If undefined default microphone
18 videoSource: undefined, // The source of video. If undefined default webcam
19 publishAudio: true, // Whether you want to start publishing with your audio unmuted or not
20 publishVideo: true, // Whether you want to start publishing with your video enabled or not
21 resolution: '640x480', // The resolution of your video
22 frameRate: 30, // The frame rate of your video
23 insertMode: 'APPEND', // How the video is inserted in the target element 'video-container'
24 mirror: false // Whether to mirror your local video or not
25 });
26 session.publish(publisher);
27
28 // Add event listeners for control buttons
29 document.getElementById('mute-audio').addEventListener('click', () => {
30 publisher.publishAudio(!publisher.stream.audioActive);
31 document.getElementById('mute-audio').textContent = publisher.stream.audioActive ? 'Mute Audio' : 'Unmute Audio';
32 });
33
34 document.getElementById('disable-video').addEventListener('click', () => {
35 publisher.publishVideo(!publisher.stream.videoActive);
36 document.getElementById('disable-video').textContent = publisher.stream.videoActive ? 'Disable Video' : 'Enable Video';
37 });
38
39 document.getElementById('share-screen').addEventListener('click', () => {
40 shareScreen(session, publisher);
41 });
42 })
43 .catch(error => {
44 console.error('Error connecting to the session:', error);
45 });
46 });
47
48 function getToken() {
49 return fetch('/api/get-token')
50 .then(response => response.json())
51 .then(data => data.token);
52 }
53
54 function shareScreen(session, publisher) {
55 OV.initPublisherAsync('local-video', {
56 videoSource: 'screen' // Pass 'screen' as the video source
57 }).then(screenPublisher => {
58 session.unpublish(publisher);
59 session.publish(screenPublisher);
60
61 // Stop screen sharing when the user clicks the button again
62 screenPublisher.on('streamDestroyed', () => {
63 session.unpublish(screenPublisher);
64 session.publish(publisher);
65 document.getElementById('share-screen').textContent = 'Share Screen';
66 });
67
68 document.getElementById('share-screen').textContent = 'Stop Sharing';
69 }).catch(error => {
70 console.error('Error sharing screen: ', error);
71 });
72 }
73 }
74
Functionality Explanation
Mute/Unmute Audio
The
mute-audio
button toggles the audio state of the publisher. When clicked, it mutes or unmutes the audio and updates the button text accordingly.Enable/Disable Video
The
disable-video
button toggles the video state of the publisher. When clicked, it disables or enables the video stream and updates the button text accordingly.Share Screen
The
share-screen
button starts or stops screen sharing. When clicked, it initializes a new publisher with the video source set to screen
. It also handles stopping the screen share and reverting to the webcam feed when clicked again.By implementing these controls, users can manage their audio and video streams, enhancing their experience in the video call application. The ability to mute/unmute audio, enable/disable video, and share the screen are essential features for any video conferencing application.
In the next part, we will focus on implementing the participant view, which involves displaying the video streams of all participants in the session. This will further enrich the functionality and user experience of your OpenVidu application.
Step 5: Implementing Participant View
Managing Participants
The participant view is crucial for displaying the video streams of all users in the session. This step involves managing the addition and removal of participant video streams dynamically as users join and leave the session.
Displaying Video Streams of Multiple Participants
Handling Stream Events
We will update the
initializeSession
function to handle participant events such as stream creation and destruction.JavaScript
1 function initializeSession(username) {
2 const OV = new OpenVidu();
3 const session = OV.initSession();
4
5 // Handle stream creation (when a new participant joins)
6 session.on('streamCreated', (event) => {
7 const subscriber = session.subscribe(event.stream, 'remote-videos');
8 subscriber.on('videoElementCreated', (event) => {
9 event.element.play();
10 const videoBox = document.createElement('div');
11 videoBox.className = 'video-box';
12 videoBox.id = `participant-${event.stream.streamId}`;
13 videoBox.appendChild(event.element);
14 document.getElementById('remote-videos').appendChild(videoBox);
15 });
16 });
17
18 // Handle stream destruction (when a participant leaves)
19 session.on('streamDestroyed', (event) => {
20 const videoBox = document.getElementById(`participant-${event.stream.streamId}`);
21 if (videoBox) {
22 videoBox.parentNode.removeChild(videoBox);
23 }
24 });
25
26 getToken().then(token => {
27 session.connect(token, { clientData: username })
28 .then(() => {
29 // Create a publisher for the local video
30 const publisher = OV.initPublisher('local-video', {
31 audioSource: undefined,
32 videoSource: undefined,
33 publishAudio: true,
34 publishVideo: true,
35 resolution: '640x480',
36 frameRate: 30,
37 insertMode: 'APPEND',
38 mirror: false
39 });
40 session.publish(publisher);
41
42 // Add event listeners for control buttons
43 document.getElementById('mute-audio').addEventListener('click', () => {
44 publisher.publishAudio(!publisher.stream.audioActive);
45 document.getElementById('mute-audio').textContent = publisher.stream.audioActive ? 'Mute Audio' : 'Unmute Audio';
46 });
47
48 document.getElementById('disable-video').addEventListener('click', () => {
49 publisher.publishVideo(!publisher.stream.videoActive);
50 document.getElementById('disable-video').textContent = publisher.stream.videoActive ? 'Disable Video' : 'Enable Video';
51 });
52
53 document.getElementById('share-screen').addEventListener('click', () => {
54 shareScreen(session, publisher);
55 });
56 })
57 .catch(error => {
58 console.error('Error connecting to the session:', error);
59 });
60 });
61
62 function getToken() {
63 return fetch('/api/get-token')
64 .then(response => response.json())
65 .then(data => data.token);
66 }
67
68 function shareScreen(session, publisher) {
69 OV.initPublisherAsync('local-video', {
70 videoSource: 'screen'
71 }).then(screenPublisher => {
72 session.unpublish(publisher);
73 session.publish(screenPublisher);
74
75 screenPublisher.on('streamDestroyed', () => {
76 session.unpublish(screenPublisher);
77 session.publish(publisher);
78 document.getElementById('share-screen').textContent = 'Share Screen';
79 });
80
81 document.getElementById('share-screen').textContent = 'Stop Sharing';
82 }).catch(error => {
83 console.error('Error sharing screen: ', error);
84 });
85 }
86 }
87
Handling Participant Events
Stream Created
When a new participant joins the session, the
streamCreated
event is triggered. This event allows us to subscribe to the new participant's stream and add their video element to the remote-videos
container.Stream Destroyed
When a participant leaves the session, the
streamDestroyed
event is triggered. This event removes the participant's video element from the remote-videos
container.Code Examples for Managing Participant Views
The following JavaScript code ensures that participant video streams are dynamically added and removed as users join and leave the session:
Stream Creation Handling
Adds a new video element for each participant who joins the session.
JavaScript
1 session.on('streamCreated', (event) => {
2 const subscriber = session.subscribe(event.stream, 'remote-videos');
3 subscriber.on('videoElementCreated', (event) => {
4 event.element.play();
5 const videoBox = document.createElement('div');
6 videoBox.className = 'video-box';
7 videoBox.id = `participant-${event.stream.streamId}`;
8 videoBox.appendChild(event.element);
9 document.getElementById('remote-videos').appendChild(videoBox);
10 });
11 });
12
Stream Destruction Handling
Removes the video element of the participant who leaves the session.
JavaScript
1 session.on('streamDestroyed', (event) => {
2 const videoBox = document.getElementById(`participant-${event.stream.streamId}`);
3 if (videoBox) {
4 videoBox.parentNode.removeChild(videoBox);
5 }
6 });
7
By implementing the participant view, your OpenVidu application can now dynamically manage the video streams of all participants in the session. This functionality is essential for creating a seamless and interactive video conferencing experience.
In the next part, we will focus on running your code and testing the application to ensure everything works as expected. This will involve starting the OpenVidu server, launching the application in a browser, and performing some basic tests.
Step 6: Running Your Code and Conclusion
Now that we have implemented the core functionalities of our OpenVidu application, it's time to run the code and test the application to ensure everything works as expected. Follow these steps to start the OpenVidu server, launch the application, and perform some basic tests.
Starting the OpenVidu Server
Download and Launch OpenVidu Server
If you haven’t already, download the OpenVidu server from the
OpenVidu Downloads page
.Start the server by running the following command in your terminal:
bash
1 ./openvidu start
2
Configure OpenVidu
Ensure you have set the necessary configuration options, such as
OPENVIDU_SECRET
and OPENVIDU_PUBLICURL
, in your docker-compose.yml
or environment variables. This configuration ensures that your OpenVidu server is secure and accessible.Launching the Application in a Browser
Start Your Application
Navigate to your project directory and start your application server. If you’re using a Node.js server, the command will typically be:
bash
1 node server.js
2
Open Your Browser
Open your preferred web browser and navigate to
http://localhost:YOUR_PORT
. Replace YOUR_PORT
with the port number your application server is running on (commonly 3000
or 5000
).Testing and Debugging Tips
Join the Session
Enter your name in the join screen and click the “Join” button. Ensure that your video stream appears in the local video container and any other participants' video streams appear in the remote video container.
Test Controls
Test the mute/unmute audio, enable/disable video, and share screen functionalities by clicking the corresponding buttons. Verify that the video and audio states toggle correctly and that screen sharing works as expected.
Check Console for Errors
Open the browser’s developer console (usually accessible with
F12
or Ctrl+Shift+I
) and check for any errors or warnings. Debugging information and error messages can help you identify and fix issues in your code.Conclusion
In this comprehensive guide, we build a video chat app with OpenVidu WebRTC using Javascript
from scratch. By following these instructions, and step by step guide you have learned how to set up your environment, wireframe components, implement a join screen, add video controls, and manage participant views. With your application running, you can now explore advanced features and customize your application further.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ