WebRTC (Web Real-Time Communication) is a powerful technology that enables real-time audio, video, and data communication between browsers and devices. This blog post will guide you through the intricacies of building a WebRTC group chat application, covering essential concepts, implementation details, and advanced considerations.
What is WebRTC Group Chat?
Understanding WebRTC
WebRTC is an open-source project providing web browsers and mobile applications with real-time communication (RTC) capabilities via simple APIs. It eliminates the need for intermediaries, plugins or downloads. WebRTC's core components include APIs for capturing and streaming audio and video, establishing peer-to-peer connections, and exchanging data.
Defining WebRTC Group Chat
WebRTC group chat extends the basic peer-to-peer communication to multiple participants. Instead of a one-on-one conversation, a group chat allows several users to communicate simultaneously through text, audio, and video. This functionality opens doors for collaborative applications, real-time gaming, and interactive educational platforms.
Advantages of WebRTC Group Chat
- Low Latency: WebRTC minimizes latency by enabling direct peer-to-peer communication where possible.
- No Plugins Required: WebRTC is natively supported by modern browsers, eliminating the need for browser plugins.
- Security: WebRTC incorporates robust security features, including encryption, to protect user data.
- Cost-Effective: Reduces server load, especially for media streaming, by leveraging peer-to-peer connections.
- Flexibility: WebRTC supports a wide range of use cases, from simple text chats to complex video conferencing solutions.
How WebRTC Group Chat Works
Signaling and Peer-to-Peer Connection
WebRTC establishes peer-to-peer connections using a process called signaling. Signaling involves exchanging metadata between peers to negotiate the connection. This metadata includes session descriptions (SDP) specifying media capabilities and network information (ICE candidates).
Signaling itself is not part of the WebRTC API and requires an external signaling server. Common signaling mechanisms include WebSocket, Socket.IO, and HTTP-based solutions.
ICE Candidates and STUN/TURN Servers
Interactive Connectivity Establishment (ICE) is a framework that enables peers to discover the best path for communication, considering factors like firewalls and network address translation (NAT). ICE gathers potential connection paths, called ICE candidates, which include:
- Host Candidates: Direct connections using the peer's IP address.
- SRVREFLEXIVE Candidates: IP address that is received by a STUN server.
- RELAY Candidates: IP address and port given by a TURN server.
STUN (Session Traversal Utilities for NAT) servers help peers discover their public IP address and port. TURN (Traversal Using Relays around NAT) servers act as relays when direct peer-to-peer connections are not possible, such as when peers are behind restrictive firewalls.
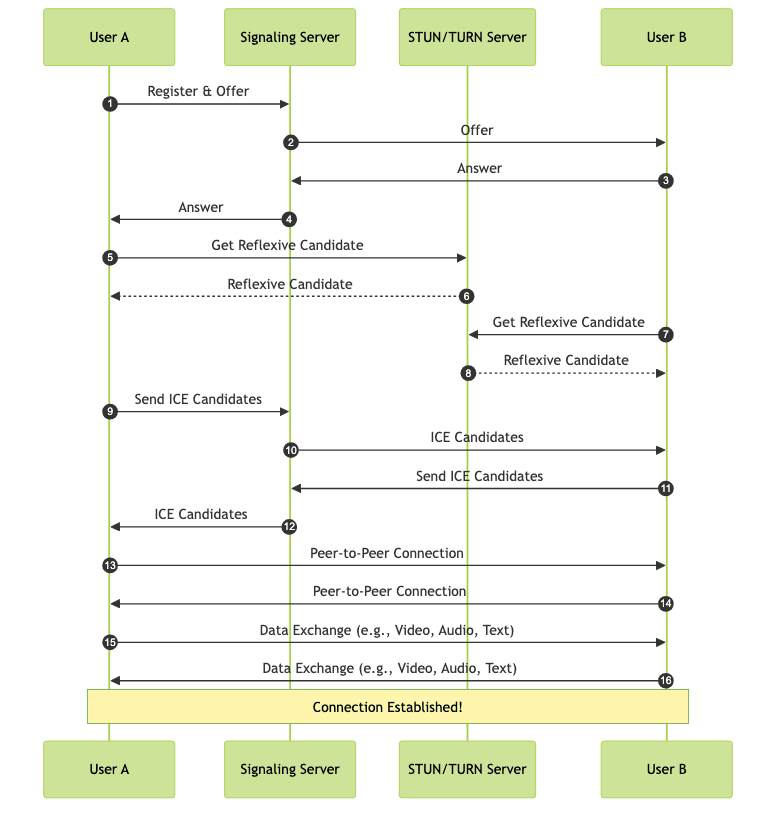
javascript
1// Basic WebRTC connection establishment
2const configuration = { iceServers: [{ urls: 'stun:stun.l.google.com:19302' }] };
3
4const peerConnection = new RTCPeerConnection(configuration);
5
6peerConnection.onicecandidate = event => {
7 if (event.candidate) {
8 // Send the candidate to the remote peer via the signaling server
9 console.log('ICE candidate:', event.candidate);
10 }
11};
12
13peerConnection.ondatachannel = event => {
14 const dataChannel = event.channel;
15 dataChannel.onmessage = event => {
16 console.log('Received message:', event.data);
17 };
18};
19
20peerConnection.createDataChannel('chat');
21
Data Channels for Text and other Data
WebRTC data channels provide a bidirectional, peer-to-peer communication channel for arbitrary data. Data channels can be used for text messaging, file transfer, or any other type of data exchange. Data channels operate over either reliable or unreliable transport protocols (SCTP).
javascript
1// Sending a message via WebRTC data channel
2const dataChannel = peerConnection.createDataChannel('chat');
3
4dataChannel.onopen = () => {
5 dataChannel.send('Hello from peer!');
6};
7
8dataChannel.onmessage = event => {
9 console.log('Received message:', event.data);
10};
11
Handling Multiple Peers (Mesh vs. Server-mediated)
In a group chat scenario, you need to consider how to handle multiple peers. There are two main architectures:
- Mesh Architecture: Each peer connects directly to every other peer in the group. This approach offers low latency but has scalability limitations. The number of connections grows quadratically with the number of participants (O(n^2)), making it unsuitable for large groups.
- Server-Mediated Architecture: Peers connect to a central server (e.g., Selective Forwarding Unit or SFU), which relays media streams between participants. This approach improves scalability, as each peer only needs to maintain a single connection to the server. However, it introduces additional latency and server costs.
Building a WebRTC Group Chat Application
Choosing a Signaling Server
The signaling server is responsible for facilitating the initial connection establishment between peers. Several options are available:
- Node.js with Socket.IO: A popular choice for real-time applications, offering flexibility and scalability.
- Firebase Realtime Database: A cloud-based solution for real-time data synchronization.
- Ably: A globally distributed real-time messaging platform with built-in WebRTC support.
- Custom Implementation: Using WebSockets or other protocols with any backend language like Go, Python, etc.
Frontend Development (JavaScript, HTML, CSS)
The frontend involves creating the user interface for the chat application using HTML, CSS, and JavaScript. The interface should include elements for displaying messages, entering text, and managing users.
html
1<!DOCTYPE html>
2<html>
3<head>
4 <title>WebRTC Group Chat</title>
5</head>
6<body>
7 <div id="chat-container">
8 <div id="messages"></div>
9 <input type="text" id="message-input">
10 <button id="send-button">Send</button>
11 </div>
12 <script src="script.js"></script>
13</body>
14</html>
15
javascript
1// JavaScript function to handle incoming messages
2const messagesDiv = document.getElementById('messages');
3
4function handleIncomingMessage(message) {
5 const messageElement = document.createElement('div');
6 messageElement.textContent = message;
7 messagesDiv.appendChild(messageElement);
8}
9
Backend Development (if using a custom server)
If you are using a custom signaling server, you will need to develop the backend logic to handle signaling messages, manage user connections, and potentially implement other features such as user authentication and presence.
Testing and Debugging
Testing and debugging are crucial steps in the development process. Use browser developer tools to inspect WebRTC connections, analyze network traffic, and identify potential issues. Tools like Wireshark can also be helpful for capturing and analyzing network packets. Using online WebRTC tools is great for checking if you have any leaks.
Advanced Concepts and Considerations
Scalability Challenges and Solutions
Scalability is a major challenge for WebRTC group chat applications, especially with mesh architectures. Server-mediated architectures (SFUs) provide a more scalable solution but introduce additional complexity and cost. Consider using load balancing and auto-scaling to handle increasing traffic.
Security Implications and Best Practices
Security is paramount when building WebRTC applications. Always use secure signaling protocols (e.g., WSS) and ensure that all communication is encrypted. Implement robust authentication and authorization mechanisms to prevent unauthorized access.
Error Handling and Robustness
Implement comprehensive error handling to gracefully handle unexpected events such as network disconnections, ICE failures, and signaling server errors. Provide informative error messages to users and implement retry mechanisms where appropriate.
Optimizing Performance for Low-Bandwidth Environments
Optimize video and audio codecs to reduce bandwidth consumption. Implement adaptive bitrate streaming to dynamically adjust the quality of media streams based on network conditions. Prioritize audio over video in low-bandwidth environments.
WebRTC Group Chat Libraries and Frameworks
Overview of popular libraries
- Simple Peer: A lightweight library that simplifies WebRTC peer-to-peer connections.
https://github.com/feross/simple-peer
- PeerJS: Provides a high-level API for WebRTC, simplifying signaling and peer management.
https://peerjs.com/
- Jitsi Meet: An open-source video conferencing platform with a robust WebRTC implementation.
https://jitsi.org/
- Daily.co: Provides prebuilt video and audio APIs.
https://www.daily.co/
Comparison of features and ease of use
Each library offers different features and levels of abstraction. Simple Peer is ideal for simple peer-to-peer connections, while PeerJS provides a more comprehensive API. Jitsi Meet is a full-featured platform that can be customized and integrated into other applications. Daily.co's prebuilt API's abstract the entire WebRTC implementation process allowing developers to quickly build and deploy video and audio. The choice depends on the specific requirements of your application.
Real-World Applications of WebRTC Group Chat
Examples in various industries
- Gaming: Real-time voice and video chat for multiplayer games.
- Education: Interactive online classrooms and virtual tutoring sessions.
- Healthcare: Telemedicine consultations and remote patient monitoring.
- Collaboration: Video conferencing and screen sharing for remote teams.
Future Trends in WebRTC Group Chat
- Integration with AI for features like noise cancellation and automatic translation.
- Enhanced security features to protect against evolving threats.
- Improved scalability and performance for large-scale group chat applications.
Learn More
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ