Introduction to Mastodon WebRTC
Mastodon is a decentralized social network that operates on open-source software, providing users with an alternative to centralized social media platforms. Built on a federated model, Mastodon allows users to create their own servers, or "instances," which can communicate with each other seamlessly. This decentralized approach ensures greater control over data, enhanced privacy, and the ability to customize the user experience to meet the needs of diverse communities.
WebRTC (Web Real-Time Communication) is a technology that enables real-time communication directly between web browsers without the need for plugins. Utilizing a set of standards, WebRTC facilitates peer-to-peer video, audio, and data sharing, making it a powerful tool for creating interactive applications such as video conferencing, file transfer, and live streaming.
Integrating WebRTC into Mastodon can significantly enhance the platform's functionality by enabling real-time communication features. This integration can bring about a more dynamic and interactive user experience, allowing Mastodon to offer features such as video calls, voice chats, and real-time collaborative tools directly within the platform. This makes Mastodon not just a place for social interaction through posts and messages but also a hub for real-time, multimedia-rich communication.
The following sections of this article will guide you through the process of integrating WebRTC into a Mastodon instance, from setting up the project environment to implementing various WebRTC features. By the end of this guide, you will have a Mastodon application that leverages the power of WebRTC to offer enhanced communication capabilities.
Getting Started with the Code!
In this section, we'll walk you through the process of creating a new Mastodon app with WebRTC integration. We'll cover the initial setup, installation of necessary packages, and the overall project structure.
Create a New Mastodon WebRTC App
Before we begin, ensure you have the necessary prerequisites installed on your system:
- Ruby (version 2.5 or higher)
- Node.js (version 12 or higher)
- Yarn package manager
- PostgreSQL database
- Redis server
To create a new Mastodon project, follow these steps:
Clone the Mastodon repository
sh
1 git clone https://github.com/mastodon/mastodon.git
2 cd mastodon
Set up the environment
Copy the example environment file and configure it according to your setup:
sh
1 cp .env.production.sample .env.production
Install dependencies
Install Ruby and Node.js dependencies using Bundler and Yarn:
sh
1 bundle install
2 yarn install
Database setup
Create and migrate the database:
sh
1 RAILS_ENV=production bundle exec rails db:setup
Install Necessary Packages
To integrate WebRTC, you'll need to install additional Node.js packages that facilitate real-time communication. These packages include
simple-peer
for WebRTC peer connections and socket.io
for signaling.[a] Install WebRTC packages
sh
1 yarn add simple-peer socket.io
[b] Set up Socket.IO server
In the project directory, create a new file
server/socket.js
to handle signaling:JavaScript
1 const io = require('socket.io')(3000);
2
3 io.on('connection', socket => {
4 socket.on('signal', data => {
5 io.to(data.room).emit('signal', data);
6 });
7
8 socket.on('join', room => {
9 socket.join(room);
10 });
11 });
Structure of the Project
Understanding the project structure is crucial for efficient development. Here is an overview of the key directories and files in a Mastodon project:
- app/: Contains the Rails application code, including models, views, and controllers.
- config/: Configuration files for the Rails application.
- db/: Database schema and migration files.
- public/: Static files served by the web server.
- server/: Custom server-side scripts (e.g.,
socket.js
for WebRTC signaling). - frontend/: JavaScript and CSS assets for the client-side interface.
App Architecture
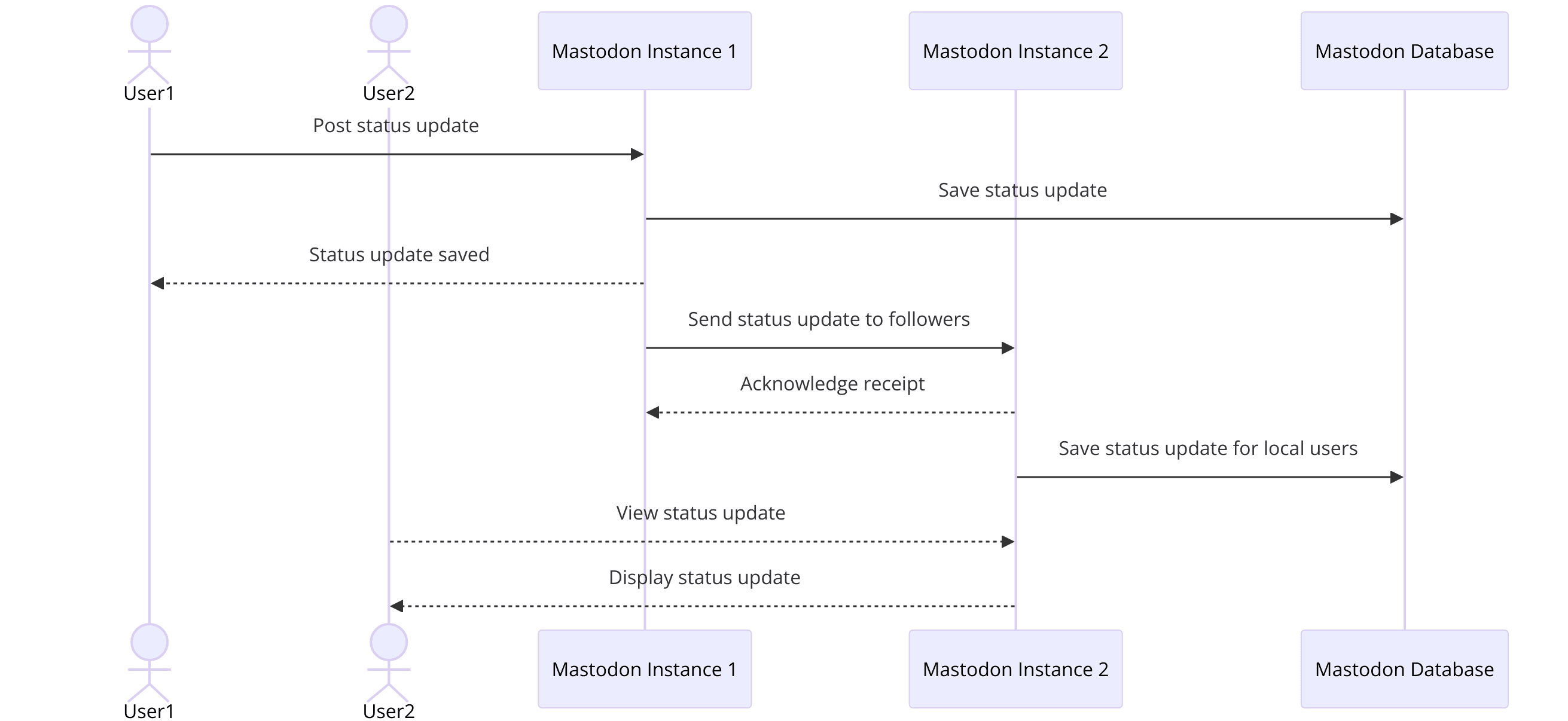
- Backend: A Socket.IO server to handle signaling between peers.
- Frontend: WebRTC APIs to manage peer-to-peer connections and user interface components for video and audio streams.
In the next sections, we will delve into the detailed implementation of each component, starting with setting up the WebRTC integration.
Step 1: Get Started with WebRTC Integration
Integrating WebRTC into your Mastodon project begins with setting up the necessary files and configuring the basic WebRTC functionality. This step will guide you through creating the essential WebRTC integration file and configuring it for your Mastodon application.
File: webrtc_integration.js
[a] Create the File
In your project’s frontend directory, create a new file named
webrtc_integration.js
. This file will handle the core WebRTC functionality.sh
1 touch frontend/webrtc_integration.js
[b] Basic WebRTC Setup
Open
webrtc_integration.js
and add the following code to establish a basic WebRTC connection using the simple-peer
library:JavaScript
1 const SimplePeer = require('simple-peer');
2 const io = require('socket.io-client');
3
4 const socket = io.connect('http://localhost:3000');
5 let localPeer;
6 let remotePeerId;
7
8 // Function to initialize a WebRTC connection
9 function initWebRTC(isInitiator, stream) {
10 localPeer = new SimplePeer({
11 initiator: isInitiator,
12 stream: stream,
13 trickle: false
14 });
15
16 localPeer.on('signal', data => {
17 socket.emit('signal', { room: 'webrtc_room', signal: data });
18 });
19
20 localPeer.on('stream', remoteStream => {
21 // Display the remote stream in a video element
22 const remoteVideo = document.getElementById('remoteVideo');
23 remoteVideo.srcObject = remoteStream;
24 remoteVideo.play();
25 });
26
27 socket.on('signal', data => {
28 if (data.signal) {
29 localPeer.signal(data.signal);
30 }
31 });
32 }
33
34 // Function to start a WebRTC session
35 function startSession() {
36 navigator.mediaDevices.getUserMedia({ video: true, audio: true })
37 .then(stream => {
38 const localVideo = document.getElementById('localVideo');
39 localVideo.srcObject = stream;
40 localVideo.play();
41 initWebRTC(true, stream);
42 })
43 .catch(err => console.error('Error accessing media devices.', err));
44 }
45
46 // Event listener for joining a room
47 socket.on('connect', () => {
48 socket.emit('join', 'webrtc_room');
49 });
50
51 // Export the startSession function
52 module.exports = { startSession };
[c] Add HTML Elements
Ensure your HTML file includes video elements to display the local and remote streams:
HTML
1 <video id="localVideo" autoplay muted></video>
2 <video id="remoteVideo" autoplay></video>
With this basic setup, your application can now initialize a WebRTC connection and handle video streams. The next steps will involve wireframing the components and implementing user interface elements to manage these connections efficiently.
Step 2: Wireframe all the Components
In this step, we will outline the process of wireframing the WebRTC components within the Mastodon interface. Wireframing helps in visualizing the layout and user interaction flow before diving into the detailed implementation. This section focuses on creating a user-friendly interface for initiating and managing WebRTC sessions.
Wireframing Components
[a] Join Screen
The join screen allows users to start or join a WebRTC session. It includes a simple form for entering the session details and a button to initiate the connection.
HTML
1 <div id="joinScreen">
2 <h2>Join WebRTC Session</h2>
3 <button id="startSessionBtn">Start Session</button>
4 </div>
[b] Video Chat Interface
Once the session is started, users will see the local and remote video streams. The interface includes controls for managing the session, such as mute/unmute, and end call buttons.
HTML
1 <div id="videoChat">
2 <video id="localVideo" autoplay muted></video>
3 <video id="remoteVideo" autoplay></video>
4 <div id="controls">
5 <button id="muteBtn">Mute</button>
6 <button id="endCallBtn">End Call</button>
7 </div>
8 </div>
[c] CSS for Layout
Apply basic CSS to ensure the components are displayed correctly and responsively.
CSS
1 #joinScreen, #videoChat {
2 display: flex;
3 flex-direction: column;
4 align-items: center;
5 justify-content: center;
6 }
7
8 #videoChat {
9 display: none;
10 }
11
12 video {
13 width: 45%;
14 margin: 10px;
15 }
16
17 #controls {
18 margin-top: 10px;
19 }
20
21 button {
22 margin: 5px;
23 padding: 10px;
24 }
[d] JavaScript to Toggle Views
Add JavaScript to toggle between the join screen and the video chat interface.
JavaScript
1 document.getElementById('startSessionBtn').addEventListener('click', () => {
2 document.getElementById('joinScreen').style.display = 'none';
3 document.getElementById('videoChat').style.display = 'flex';
4 startSession(); // Call the function to start the WebRTC session
5 });
6
7 document.getElementById('endCallBtn').addEventListener('click', () => {
8 document.getElementById('joinScreen').style.display = 'flex';
9 document.getElementById('videoChat').style.display = 'none';
10 // Add logic to end the WebRTC session
11 });
By wireframing these components, you can ensure a smooth and intuitive user experience for initiating and managing WebRTC sessions within the Mastodon platform. In the next step, we will implement the join screen functionality.
Step 3: Implement Join Screen
The join screen is the entry point for users to start or join a WebRTC session within your Mastodon application. In this step, we will implement the join screen functionality, which includes setting up the interface and handling user interactions to initiate WebRTC connections.
Join Screen Implementation
[a] HTML Structure
Ensure your HTML file includes the necessary elements for the join screen.
HTML
1 <div id="joinScreen">
2 <h2>Join WebRTC Session</h2>
3 <button id="startSessionBtn">Start Session</button>
4 </div>
5 <div id="videoChat" style="display: none;">
6 <video id="localVideo" autoplay muted></video>
7 <video id="remoteVideo" autoplay></video>
8 <div id="controls">
9 <button id="muteBtn">Mute</button>
10 <button id="endCallBtn">End Call</button>
11 </div>
12 </div>
[b] CSS Styling
Add CSS to style the join screen and video chat interface.
CSS
1 #joinScreen, #videoChat {
2 display: flex;
3 flex-direction: column;
4 align-items: center;
5 justify-content: center;
6 margin-top: 50px;
7 }
8
9 video {
10 width: 45%;
11 margin: 10px;
12 border: 1px solid #ccc;
13 }
14
15 #controls {
16 margin-top: 10px;
17 }
18
19 button {
20 margin: 5px;
21 padding: 10px;
22 cursor: pointer;
23 background-color: #007BFF;
24 color: white;
25 border: none;
26 border-radius: 5px;
27 }
28
29 button:hover {
30 background-color: #0056b3;
31 }
[c] JavaScript Functionality
Add JavaScript to handle the button click event for starting the session.
JavaScript
1 document.getElementById('startSessionBtn').addEventListener('click', () => {
2 document.getElementById('joinScreen').style.display = 'none';
3 document.getElementById('videoChat').style.display = 'flex';
4 startSession(); // Function to initialize WebRTC session
5 });
6
7 document.getElementById('endCallBtn').addEventListener('click', () => {
8 document.getElementById('joinScreen').style.display = 'flex';
9 document.getElementById('videoChat').style.display = 'none';
10 endSession(); // Function to terminate WebRTC session
11 });
[d] WebRTC Session Initialization
Modify the
startSession
function in webrtc_integration.js
to handle the user media stream and initiate the WebRTC connection.JavaScript
1 function startSession() {
2 navigator.mediaDevices.getUserMedia({ video: true, audio: true })
3 .then(stream => {
4 const localVideo = document.getElementById('localVideo');
5 localVideo.srcObject = stream;
6 localVideo.play();
7 initWebRTC(true, stream); // Initialize WebRTC as the initiator
8 })
9 .catch(err => console.error('Error accessing media devices.', err));
10 }
11
12 function endSession() {
13 if (localPeer) {
14 localPeer.destroy(); // Terminate the peer connection
15 }
16 }
By implementing the join screen, users can now initiate WebRTC sessions seamlessly. The next step will focus on implementing the control features, such as muting the microphone and ending the call.
Step 4: Implement Controls
In this step, we will implement the control features for the WebRTC session within your Mastodon application. These controls will include buttons for muting/unmuting the microphone and ending the call. These functionalities enhance user experience by providing essential tools to manage their WebRTC sessions effectively.
Controls Implementation
[a] HTML Structure
Ensure the controls are included in your HTML file within the video chat interface.
HTML
1 <div id="videoChat" style="display: none;">
2 <video id="localVideo" autoplay muted></video>
3 <video id="remoteVideo" autoplay></video>
4 <div id="controls">
5 <button id="muteBtn">Mute</button>
6 <button id="endCallBtn">End Call</button>
7 </div>
8 </div>
[b] JavaScript for Controls
Add JavaScript to handle the mute/unmute and end call functionalities.
JavaScript
1 let isMuted = false;
2
3 document.getElementById('muteBtn').addEventListener('click', () => {
4 const localStream = document.getElementById('localVideo').srcObject;
5 localStream.getAudioTracks().forEach(track => track.enabled = !track.enabled);
6 isMuted = !isMuted;
7 document.getElementById('muteBtn').textContent = isMuted ? 'Unmute' : 'Mute';
8 });
9
10 document.getElementById('endCallBtn').addEventListener('click', () => {
11 document.getElementById('joinScreen').style.display = 'flex';
12 document.getElementById('videoChat').style.display = 'none';
13 endSession(); // Function to terminate WebRTC session
14 });
15
16 function endSession() {
17 if (localPeer) {
18 localPeer.destroy(); // Terminate the peer connection
19 }
20 // Reset the local video stream
21 const localVideo = document.getElementById('localVideo');
22 localVideo.srcObject.getTracks().forEach(track => track.stop());
23 localVideo.srcObject = null;
24 }
[c] Function to Toggle Audio Tracks
The function to mute and unmute the audio tracks of the local stream ensures that users can control their microphone during a session.
JavaScript
1 function toggleMute() {
2 const localStream = document.getElementById('localVideo').srcObject;
3 localStream.getAudioTracks().forEach(track => {
4 track.enabled = !track.enabled;
5 });
6 isMuted = !isMuted;
7 document.getElementById('muteBtn').textContent = isMuted ? 'Unmute' : 'Mute';
8 }
9
10 document.getElementById('muteBtn').addEventListener('click', toggleMute);
[d] Ending the Call
The function to end the call will clean up the WebRTC session and reset the interface.
JavaScript
1 function endSession() {
2 if (localPeer) {
3 localPeer.destroy(); // Terminate the peer connection
4 }
5 const localVideo = document.getElementById('localVideo');
6 localVideo.srcObject.getTracks().forEach(track => track.stop());
7 localVideo.srcObject = null;
8 document.getElementById('joinScreen').style.display = 'flex';
9 document.getElementById('videoChat').style.display = 'none';
10 }
11
12 document.getElementById('endCallBtn').addEventListener('click', endSession);
By implementing these controls, users have the necessary tools to manage their WebRTC sessions effectively. In the next step, we will focus on creating a participant view to display all participants in the session.
Step 5: Implement Participant View
In this step, we will implement the participant view, which displays all participants in the WebRTC session. This feature is crucial for providing a comprehensive and interactive experience by showing the video streams of all connected users.
Participant View Implementation
[a] HTML Structure
Ensure your HTML file includes a container to display the participant video streams.
HTML
1 <div id="videoChat" style="display: none;">
2 <video id="localVideo" autoplay muted></video>
3 <div id="remoteVideos"></div>
4 <div id="controls">
5 <button id="muteBtn">Mute</button>
6 <button id="endCallBtn">End Call</button>
7 </div>
8 </div>
[b] JavaScript to Handle Remote Streams
Update your WebRTC integration code to handle multiple participants and display their video streams.
JavaScript
1 const SimplePeer = require('simple-peer');
2 const io = require('socket.io-client');
3
4 const socket = io.connect('http://localhost:3000');
5 let localPeer;
6 let peers = {}; // Store peer connections
7
8 // Function to initialize a WebRTC connection
9 function initWebRTC(isInitiator, stream) {
10 localPeer = new SimplePeer({
11 initiator: isInitiator,
12 stream: stream,
13 trickle: false
14 });
15
16 localPeer.on('signal', data => {
17 socket.emit('signal', { room: 'webrtc_room', signal: data });
18 });
19
20 localPeer.on('stream', remoteStream => {
21 addRemoteStream(remoteStream);
22 });
23
24 socket.on('signal', data => {
25 if (data.signal) {
26 localPeer.signal(data.signal);
27 }
28 });
29 }
30
31 // Function to add remote stream to the participant view
32 function addRemoteStream(stream) {
33 const remoteVideo = document.createElement('video');
34 remoteVideo.srcObject = stream;
35 remoteVideo.autoplay = true;
36 remoteVideo.playsInline = true;
37 document.getElementById('remoteVideos').appendChild(remoteVideo);
38 }
39
40 // Function to start a WebRTC session
41 function startSession() {
42 navigator.mediaDevices.getUserMedia({ video: true, audio: true })
43 .then(stream => {
44 const localVideo = document.getElementById('localVideo');
45 localVideo.srcObject = stream;
46 localVideo.play();
47 initWebRTC(true, stream); // Initialize WebRTC as the initiator
48 })
49 .catch(err => console.error('Error accessing media devices.', err));
50 }
51
52 // Event listener for joining a room
53 socket.on('connect', () => {
54 socket.emit('join', 'webrtc_room');
55 });
56
57 // Export the startSession function
58 module.exports = { startSession };
[c] CSS for Participant View
Add CSS to style the remote video elements and ensure a responsive layout.
CSS
1 #remoteVideos {
2 display: flex;
3 flex-wrap: wrap;
4 justify-content: center;
5 margin-top: 20px;
6 }
7
8 #remoteVideos video {
9 width: 45%;
10 margin: 10px;
11 border: 1px solid #ccc;
12 background-color: #000;
13 }
[d] JavaScript to Handle New Participants
Update the socket event to handle new participants joining the session.
JavaScript
1 socket.on('newParticipant', data => {
2 if (!peers[data.id]) {
3 const peer = new SimplePeer({ initiator: false });
4 peers[data.id] = peer;
5
6 peer.on('signal', signal => {
7 socket.emit('signal', { id: data.id, signal: signal });
8 });
9
10 peer.on('stream', remoteStream => {
11 addRemoteStream(remoteStream);
12 });
13
14 peer.signal(data.signal);
15 }
16 });
By implementing the participant view, users can see all participants in the WebRTC session, enhancing the interactive experience. The next step will focus on running and testing your complete Mastodon WebRTC application.
Step 6: Run Your Code Now
With all the components in place, it's time to run and test your Mastodon WebRTC application. Follow these steps to ensure everything works seamlessly.
[a] Start the Mastodon Server
Make sure your Mastodon server is running:
sh
1 RAILS_ENV=production bundle exec rails s
[b] Start the WebRTC Signaling Server
Run your Socket.IO server to handle signaling:
sh
1 node server/socket.js
[c] Access Your Application
Open your Mastodon instance in a web browser and navigate to the page with your WebRTC integration.
[d] Test the Functionality
- Click the "Start Session" button.
- Allow access to your camera and microphone.
- Verify that your local video stream is displayed.
- Test the mute/unmute and end call functionalities.
- Ensure that remote participants' video streams are displayed correctly.
Congratulations! You've successfully integrated WebRTC into your Mastodon instance, enabling real-time communication features. This integration enhances user interaction by providing video and audio capabilities directly within the social network platform and
social wall
.Conclusion
This guide walked you through setting up a Mastodon WebRTC application, from project initialization and environment setup to implementing key features like the join screen, controls, and participant view. By following these steps, you've created a dynamic and interactive Mastodon instance that leverages the power of WebRTC.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ