Introduction
What is BigBlueButton WebRTC Technology?
BigBlueButton is an open-source web conferencing system designed specifically for online learning. It provides a robust platform for virtual classrooms, allowing educators to deliver engaging and interactive lessons to students anywhere in the world. With features like real-time sharing of audio, video, slides, chat, and screen, BigBlueButton has become a preferred choice for educational institutions seeking a reliable and scalable virtual classroom solution.
At the heart of BigBlueButton's seamless real-time communication capabilities is WebRTC (Web Real-Time Communication) technology. WebRTC is a powerful open-source project that enables peer-to-peer audio, video, and data sharing directly within web browsers, without the need for plugins. This technology ensures low latency and high-quality communication, which are critical for maintaining an effective online learning environment.
To enhance its functionality, BigBlueButton integrates with Freeswitch and Kurento. Freeswitch is a scalable open-source telephony platform that supports various communication protocols, enabling advanced audio routing and processing. Kurento, on the other hand, is a media server capable of handling complex multimedia streams, providing features such as media transcoding, recording, and broadcasting. Together, these technologies create a comprehensive framework that powers BigBlueButton's advanced virtual classroom capabilities, ensuring a smooth and engaging experience for both educators and students.
Getting Started with the Code
Getting started with BigBlueButton involves setting up a development environment, installing necessary dependencies, and understanding the project's structure and architecture. This section will guide you through these steps to help you build a robust virtual classroom application using BigBlueButton, WebRTC, Freeswitch, and Kurento.
Create a New BigBlueButton App
To begin, you need to set up your development environment by cloning the BigBlueButton repository from GitHub. Open your terminal and run the following commands:
bash
1git clone https://github.com/bigbluebutton/bigbluebutton.git
2cd bigbluebutton
3
Install Dependencies
Next, you need to install the necessary libraries and dependencies required for BigBlueButton. Make sure you have Node.js and npm (Node Package Manager) installed on your system. Run the following command to install all required dependencies:
bash
1npm install
2
This will download and install all the necessary packages for BigBlueButton to function correctly.
Structure of the Project
Understanding the project directory structure is crucial for navigating and modifying the BigBlueButton codebase. Here’s a brief overview of the key files and folders:
- /bigbluebutton-html5: Contains the front-end code for the HTML5 client.
- /bigbluebutton-web: Contains the web application code.
- /bigbluebutton-apps: Contains server-side applications.
- /lib: Contains libraries and helper functions.
- /resources: Contains configuration files and resource bundles.
Each directory serves a specific purpose, and understanding their roles will help you navigate the project more efficiently.
App Architecture
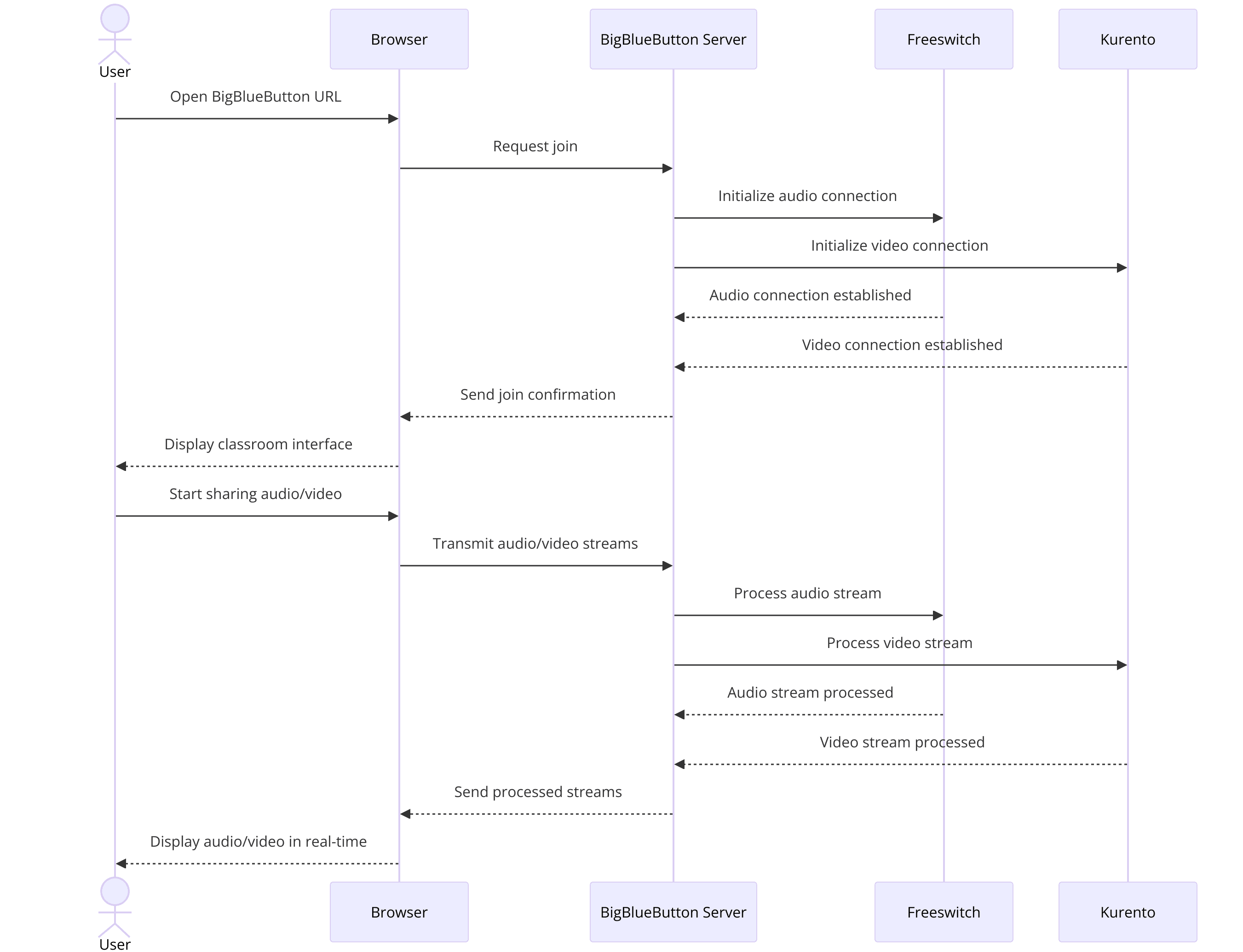
- WebRTC: Enables real-time audio and video communication within the browser.
- Freeswitch: Manages audio processing and routing.
- Kurento: Handles media streaming, transcoding, and recording.
- Node.js: Powers the backend logic and serves the front-end application.
- Redis: Manages real-time data storage and messaging.
This modular architecture ensures that each component performs a specific role, contributing to the overall functionality and reliability of the BigBlueButton platform.
By setting up your development environment and familiarizing yourself with the project structure and architecture, you're now ready to dive deeper into building and customizing your BigBlueButton application. The next sections will guide you through the specific steps to implement various features and functionalities.
Step 1: Get Started with Initial Setup
Before diving into the implementation of BigBlueButton's features, it's crucial to set up the foundational components that enable the platform to function smoothly. This involves setting up Freeswitch, configuring Kurento Media Server, and integrating these components with BigBlueButton.
Setting Up Freeswitch
Freeswitch is an essential part of BigBlueButton, handling the audio routing and processing. Follow these steps to install and configure Freeswitch:
[a] Install Freeswitch
Begin by updating your system packages and installing Freeswitch from the official repository. Open your terminal and run the following commands:
bash
1 sudo apt-get update
2 sudo apt-get install -y freeswitch
3
[b] Configure Freeswitch
Once installed, you need to configure Freeswitch for use with BigBlueButton. This involves editing configuration files located in the
/etc/freeswitch
directory. Open the freeswitch.xml
configuration file:bash
1 sudo nano /etc/freeswitch/freeswitch.xml
2
Make necessary changes to integrate with BigBlueButton, such as setting up SIP profiles and dial plans.
[c] Start Freeswitch
After configuring, start the Freeswitch service:
bash
1 sudo systemctl start freeswitch
2 sudo systemctl enable freeswitch
3
Configuring Kurento Media Server
Kurento Media Server handles the media streaming, transcoding, and recording functionalities of BigBlueButton. Follow these steps to set up Kurento:
[a] Install Kurento
Add the Kurento repository and install the server using the following commands:
bash
1 sudo apt-key adv --keyserver keyserver.ubuntu.com --recv-keys 5AFA7A83
2 sudo apt-get update
3 sudo apt-get install -y kurento-media-server
4
[b] Configure Kurento
Modify the Kurento configuration to optimize it for BigBlueButton. The configuration file is located at
/etc/kurento/kurento.conf.json
. Open this file:bash
1 sudo nano /etc/kurento/kurento.conf.json
2
Adjust settings such as the network interfaces and media pipeline options to suit your environment.
[c] Start Kurento
Start the Kurento Media Server service:
bash
1 sudo systemctl start kurento-media-server
2 sudo systemctl enable kurento-media-server
3
Integrating Freeswitch and Kurento with BigBlueButton
With both Freeswitch and Kurento set up, the next step is to integrate these components with BigBlueButton. This involves configuring BigBlueButton to use Freeswitch for audio processing and Kurento for media handling.
[a] Configure BigBlueButton
Edit the BigBlueButton configuration files to point to the Freeswitch and Kurento servers. The primary configuration file is located at
/etc/bigbluebutton/bbb-web.properties
. Open this file:bash
1 sudo nano /etc/bigbluebutton/bbb-web.properties
2
Add or modify the following entries:
1 freeswitch.ip=127.0.0.1
2 freeswitch.port=8021
3 kurento.ip=127.0.0.1
4 kurento.port=8888
5
[b] Restart BigBlueButton
After making these changes, restart the BigBlueButton service to apply the new configurations:
bash
1 sudo bbb-conf --restart
2
By completing these setup steps, you've established a solid foundation for BigBlueButton. Freeswitch and Kurento are now configured and integrated, enabling advanced audio and media capabilities. In the next section, we will move on to creating a wireframe for the components, setting the stage for developing the user interface and functionality.
Step 2: Wireframe All the Components
Creating a wireframe is an essential step in designing the user interface (UI) for your BigBlueButton application. This step helps you visualize the layout and interaction of various components, ensuring a seamless user experience. In this section, we will discuss the component architecture, tools for wireframing, and an example wireframe for a virtual classroom.
Understanding the Component Architecture
BigBlueButton's UI comprises several key components that facilitate interaction and communication in a virtual classroom. Understanding these components is crucial for effective wireframing. Here are the primary components:
- Join Screen: The entry point for users, where they can enter their names and join the classroom.
- Main Classroom Interface: The primary interface where the video, audio, chat, and presentation components are displayed.
- Controls: Buttons and options for managing audio, video, screen sharing, and other interactive features.
- Participant List: A list of all participants in the classroom, showing their status and roles.
- Chat: A text-based communication tool for participants to interact during the session.
- Presentation Area: The section where the instructor can share slides or other educational materials.
Creating a Basic Wireframe
Wireframing involves creating a simple, visual layout of your application’s interface. There are various tools available for wireframing, such as Balsamiq, Figma, and Sketch. These tools provide drag-and-drop features that make it easy to create and modify wireframes.
Step-by-Step Wireframe Creation:
Choose a Wireframing Tool
Select a wireframing tool that suits your needs. For this example, we’ll use Figma due to its ease of use and collaborative features.
Set Up the Workspace
Open Figma and create a new project. Set up the workspace by defining the dimensions of your application interface.
Design the Join Screen
- Header: Include the BigBlueButton logo and a welcoming message.
- Input Field: Add an input field for users to enter their names.
- Join Button: Include a button labeled "Join" for users to enter the classroom.
Design the Main Classroom Interface
- Video Area: Reserve a large section for the video streams of the participants.
- Controls: Place buttons for mute/unmute, start/stop video, share screen, and end session at the bottom.
- Participant List: Add a sidebar on the right showing the list of participants.
- Chat: Include a chat window at the bottom right.
- Presentation Area: Allocate space in the center for sharing slides or other materials.
By wireframing your BigBlueButton application, you ensure that all UI components are thoughtfully planned and arranged, providing a user-friendly experience. In the next section, we will delve into implementing the join screen, bringing your wireframe to life with HTML and CSS.
Step 3: Implement Join Screen
The join screen is the first interaction users have with your BigBlueButton application. It is crucial to make this screen intuitive and user-friendly to ensure a smooth entry into the virtual classroom. In this section, we will implement the join screen using HTML, CSS, and JavaScript to integrate WebRTC functionalities for real-time user entry.
Join Screen Basics
The join screen typically includes the following elements:
- A header with the application logo or name.
- An input field for users to enter their names.
- A button to join the classroom.
[a] Coding the Join Screen
Let's start by creating the HTML structure for the join screen. Open your preferred code editor and create a new file named
join.html
. Add the following HTML code:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Join Classroom - BigBlueButton</title>
7 <link rel="stylesheet" href="styles.css">
8</head>
9<body>
10 <div class="join-container">
11 <header>
12 <h1>BigBlueButton</h1>
13 <p>Welcome to your virtual classroom</p>
14 </header>
15 <main>
16 <form id="join-form">
17 <input type="text" id="username" placeholder="Enter your name" required>
18 <button type="submit">Join</button>
19 </form>
20 </main>
21 </div>
22 <script src="app.js"></script>
23</body>
24</html>
25
[b] Styling the Join Screen
Next, create a CSS file named
styles.css
to style the join screen. Add the following CSS code:CSS
1body {
2 font-family: Arial, sans-serif;
3 display: flex;
4 justify-content: center;
5 align-items: center;
6 height: 100vh;
7 margin: 0;
8 background-color: #f4f4f4;
9}
10
11.join-container {
12 text-align: center;
13 background: #fff;
14 padding: 2rem;
15 border-radius: 8px;
16 box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
17}
18
19header h1 {
20 margin: 0;
21 font-size: 2rem;
22 color: #333;
23}
24
25header p {
26 color: #777;
27}
28
29form {
30 margin-top: 2rem;
31}
32
33input[type="text"] {
34 padding: 0.5rem;
35 font-size: 1rem;
36 border: 1px solid #ccc;
37 border-radius: 4px;
38 width: 80%;
39}
40
41button {
42 padding: 0.5rem 1rem;
43 font-size: 1rem;
44 color: #fff;
45 background-color: #007bff;
46 border: none;
47 border-radius: 4px;
48 cursor: pointer;
49 margin-top: 1rem;
50}
51
52button:hover {
53 background-color: #0056b3;
54}
55
[c] Integrating WebRTC for Real-Time User Entry
Now, we need to handle the form submission and integrate WebRTC functionalities to manage user entry in real-time. Create a JavaScript file named
app.js
and add the following code:JavaScript
1document.getElementById('join-form').addEventListener('submit', function(event) {
2 event.preventDefault();
3
4 const username = document.getElementById('username').value.trim();
5
6 if (username) {
7 // Here you can add WebRTC functionalities for user entry
8 // For now, we will simply redirect the user to the classroom interface
9 localStorage.setItem('username', username);
10 window.location.href = 'classroom.html';
11 } else {
12 alert('Please enter your name');
13 }
14});
15
In this script, when the form is submitted, we capture the username and store it locally. This is a placeholder for integrating WebRTC functionalities where you would typically set up the connection and manage user entry in real-time.
By completing these steps, you have implemented a functional join screen for your BigBlueButton application. This join screen captures the user's name and prepares them to enter the virtual classroom. In the next section, we will move on to implementing the controls, providing users with the ability to manage their audio, video, and screen sharing functionalities.
Step 4: Implement Controls
The controls in a BigBlueButton application are vital for managing various functionalities such as audio, video, and screen sharing. Implementing these controls effectively ensures that users have a seamless experience during their virtual classroom sessions. In this section, we will add the necessary controls and integrate them with WebRTC for real-time interaction.
Controls Overview
The primary controls we will implement include:
- Mute/Unmute Audio
- Start/Stop Video
- Share/Stop Screen
- End Session
Each control will be represented by a button and will use WebRTC functionalities to manage the corresponding actions.
[a] Coding the Controls
First, update the HTML structure to include buttons for the controls. Open your
classroom.html
file and add the following code:HTML
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>Classroom - BigBlueButton</title>
7 <link rel="stylesheet" href="styles.css">
8</head>
9<body>
10 <div class="classroom-container">
11 <header>
12 <h1>Classroom</h1>
13 <p>Welcome, <span id="username-display"></span></p>
14 </header>
15 <main>
16 <div id="video-container"></div>
17 <div id="controls">
18 <button id="mute-btn">Mute</button>
19 <button id="video-btn">Start Video</button>
20 <button id="screen-share-btn">Share Screen</button>
21 <button id="end-session-btn">End Session</button>
22 </div>
23 </main>
24 </div>
25 <script src="app.js"></script>
26</body>
27</html>
28
[b] Styling the Controls
Next, update your
styles.css
file to style the controls:CSS
1.classroom-container {
2 text-align: center;
3 padding: 2rem;
4}
5
6header h1 {
7 font-size: 2rem;
8 margin: 0;
9}
10
11header p {
12 font-size: 1rem;
13 color: #555;
14}
15
16#video-container {
17 margin-top: 2rem;
18 border: 1px solid #ccc;
19 padding: 1rem;
20 border-radius: 8px;
21 background-color: #f9f9f9;
22}
23
24#controls {
25 margin-top: 1rem;
26}
27
28#controls button {
29 padding: 0.5rem 1rem;
30 font-size: 1rem;
31 margin: 0.5rem;
32 border: none;
33 border-radius: 4px;
34 cursor: pointer;
35 background-color: #007bff;
36 color: #fff;
37}
38
39#controls button:hover {
40 background-color: #0056b3;
41}
42
[c] Integrating WebRTC for Control Actions
Now, let’s add the JavaScript code to handle the control actions using WebRTC. Update your
app.js
file with the following code:JavaScript
1document.addEventListener('DOMContentLoaded', function() {
2 const username = localStorage.getItem('username');
3 document.getElementById('username-display').innerText = username;
4
5 const muteBtn = document.getElementById('mute-btn');
6 const videoBtn = document.getElementById('video-btn');
7 const screenShareBtn = document.getElementById('screen-share-btn');
8 const endSessionBtn = document.getElementById('end-session-btn');
9
10 let localStream;
11 let isAudioMuted = false;
12 let isVideoStarted = false;
13 let isScreenSharing = false;
14
15 // Get user media (audio and video)
16 navigator.mediaDevices.getUserMedia({ audio: true, video: true })
17 .then(stream => {
18 localStream = stream;
19 const videoContainer = document.getElementById('video-container');
20 const videoElement = document.createElement('video');
21 videoElement.srcObject = localStream;
22 videoElement.autoplay = true;
23 videoElement.muted = true; // Mute local video playback
24 videoContainer.appendChild(videoElement);
25 })
26 .catch(error => console.error('Error accessing media devices.', error));
27
28 // Mute/Unmute Audio
29 muteBtn.addEventListener('click', function() {
30 isAudioMuted = !isAudioMuted;
31 localStream.getAudioTracks()[0].enabled = !isAudioMuted;
32 muteBtn.innerText = isAudioMuted ? 'Unmute' : 'Mute';
33 });
34
35 // Start/Stop Video
36 videoBtn.addEventListener('click', function() {
37 isVideoStarted = !isVideoStarted;
38 localStream.getVideoTracks()[0].enabled = isVideoStarted;
39 videoBtn.innerText = isVideoStarted ? 'Stop Video' : 'Start Video';
40 });
41
42 // Share/Stop Screen
43 screenShareBtn.addEventListener('click', function() {
44 if (!isScreenSharing) {
45 navigator.mediaDevices.getDisplayMedia({ video: true })
46 .then(screenStream => {
47 const screenTrack = screenStream.getVideoTracks()[0];
48 localStream.removeTrack(localStream.getVideoTracks()[0]);
49 localStream.addTrack(screenTrack);
50 screenTrack.onended = function() {
51 localStream.removeTrack(screenTrack);
52 localStream.addTrack(screenStream.getVideoTracks()[0]);
53 isScreenSharing = false;
54 screenShareBtn.innerText = 'Share Screen';
55 };
56 isScreenSharing = true;
57 screenShareBtn.innerText = 'Stop Sharing';
58 })
59 .catch(error => console.error('Error sharing screen.', error));
60 } else {
61 const screenTrack = localStream.getVideoTracks()[0];
62 screenTrack.stop();
63 isScreenSharing = false;
64 screenShareBtn.innerText = 'Share Screen';
65 }
66 });
67
68 // End Session
69 endSessionBtn.addEventListener('click', function() {
70 localStream.getTracks().forEach(track => track.stop());
71 window.location.href = 'join.html';
72 });
73});
74
In this script, we handle user interactions for the controls:
- Mute/Unmute Audio: Toggles the audio track’s enabled state and updates the button text.
- Start/Stop Video: Toggles the video track’s enabled state and updates the button text.
- Share/Stop Screen: Allows users to share their screen and replace the video track with the screen track.
- End Session: Stops all media tracks and redirects the user back to the join screen.
By implementing these controls, users can manage their audio, video, and screen sharing functionalities during a virtual classroom session, enhancing the overall experience. In the next section, we will focus on implementing the participant view to manage and display all classroom participants.
Step 5: Implement Participant View
The participant view is a crucial component of the BigBlueButton application, allowing users to see who else is in the session and interact with them. This view provides essential functionality for managing participants, such as displaying participant names, roles, and statuses. In this section, we will implement the participant view using HTML, CSS, and JavaScript to dynamically manage participants.
Participant View Design
The participant view should include:
- A list of all participants in the session.
- Indicators for participant roles (e.g., presenter, viewer).
- Status indicators (e.g., muted, unmuted).
- Options to manage participants (e.g., mute, remove).
[a] Coding the Participant View
First, update the
classroom.html
file to include a section for the participant list. Add the following code inside the main
tag:HTML
1<main>
2 <div id="video-container"></div>
3 <div id="participant-list">
4 <h2>Participants</h2>
5 <ul id="participants"></ul>
6 </div>
7 <div id="controls">
8 <button id="mute-btn">Mute</button>
9 <button id="video-btn">Start Video</button>
10 <button id="screen-share-btn">Share Screen</button>
11 <button id="end-session-btn">End Session</button>
12 </div>
13</main>
14
[b] Styling the Participant View
Next, update your
styles.css
file to style the participant list:CSS
1#participant-list {
2 margin-top: 1rem;
3 border: 1px solid #ccc;
4 padding: 1rem;
5 border-radius: 8px;
6 background-color: #f9f9f9;
7}
8
9#participant-list h2 {
10 margin: 0;
11 font-size: 1.5rem;
12 color: #333;
13}
14
15#participants {
16 list-style: none;
17 padding: 0;
18}
19
20#participants li {
21 padding: 0.5rem;
22 border-bottom: 1px solid #ddd;
23 display: flex;
24 justify-content: space-between;
25 align-items: center;
26}
27
28.participant-name {
29 font-weight: bold;
30}
31
32.participant-status {
33 font-size: 0.9rem;
34 color: #777;
35}
36
[c] Integrating WebRTC for Participant Management
Now, let's add JavaScript code to manage the participant list dynamically. Update your
app.js
file with the following code:JavaScript
1document.addEventListener('DOMContentLoaded', function() {
2 const username = localStorage.getItem('username');
3 document.getElementById('username-display').innerText = username;
4
5 const muteBtn = document.getElementById('mute-btn');
6 const videoBtn = document.getElementById('video-btn');
7 const screenShareBtn = document.getElementById('screen-share-btn');
8 const endSessionBtn = document.getElementById('end-session-btn');
9 const participantsList = document.getElementById('participants');
10
11 let localStream;
12 let isAudioMuted = false;
13 let isVideoStarted = false;
14 let isScreenSharing = false;
15 let participants = [];
16
17 // Get user media (audio and video)
18 navigator.mediaDevices.getUserMedia({ audio: true, video: true })
19 .then(stream => {
20 localStream = stream;
21 const videoContainer = document.getElementById('video-container');
22 const videoElement = document.createElement('video');
23 videoElement.srcObject = localStream;
24 videoElement.autoplay = true;
25 videoElement.muted = true; // Mute local video playback
26 videoContainer.appendChild(videoElement);
27
28 // Add local participant to the list
29 addParticipant({ name: username, id: 'local', role: 'presenter', status: 'unmuted' });
30 })
31 .catch(error => console.error('Error accessing media devices.', error));
32
33 // Add participant to the list
34 function addParticipant(participant) {
35 participants.push(participant);
36 updateParticipantsView();
37 }
38
39 // Update participant list view
40 function updateParticipantsView() {
41 participantsList.innerHTML = '';
42 participants.forEach(participant => {
43 const participantItem = document.createElement('li');
44 participantItem.innerHTML = `
45 <span class="participant-name">${participant.name}</span>
46 <span class="participant-role">${participant.role}</span>
47 <span class="participant-status">${participant.status}</span>
48 `;
49 participantsList.appendChild(participantItem);
50 });
51 }
52
53 // Mute/Unmute Audio
54 muteBtn.addEventListener('click', function() {
55 isAudioMuted = !isAudioMuted;
56 localStream.getAudioTracks()[0].enabled = !isAudioMuted;
57 muteBtn.innerText = isAudioMuted ? 'Unmute' : 'Mute';
58 updateLocalParticipantStatus(isAudioMuted ? 'muted' : 'unmuted');
59 });
60
61 // Start/Stop Video
62 videoBtn.addEventListener('click', function() {
63 isVideoStarted = !isVideoStarted;
64 localStream.getVideoTracks()[0].enabled = isVideoStarted;
65 videoBtn.innerText = isVideoStarted ? 'Stop Video' : 'Start Video';
66 });
67
68 // Share/Stop Screen
69 screenShareBtn.addEventListener('click', function() {
70 if (!isScreenSharing) {
71 navigator.mediaDevices.getDisplayMedia({ video: true })
72 .then(screenStream => {
73 const screenTrack = screenStream.getVideoTracks()[0];
74 localStream.removeTrack(localStream.getVideoTracks()[0]);
75 localStream.addTrack(screenTrack);
76 screenTrack.onended = function() {
77 localStream.removeTrack(screenTrack);
78 localStream.addTrack(screenStream.getVideoTracks()[0]);
79 isScreenSharing = false;
80 screenShareBtn.innerText = 'Share Screen';
81 };
82 isScreenSharing = true;
83 screenShareBtn.innerText = 'Stop Sharing';
84 })
85 .catch(error => console.error('Error sharing screen.', error));
86 } else {
87 const screenTrack = localStream.getVideoTracks()[0];
88 screenTrack.stop();
89 isScreenSharing = false;
90 screenShareBtn.innerText = 'Share Screen';
91 }
92 });
93
94 // End Session
95 endSessionBtn.addEventListener('click', function() {
96 localStream.getTracks().forEach(track => track.stop());
97 window.location.href = 'join.html';
98 });
99
100 // Update local participant status
101 function updateLocalParticipantStatus(status) {
102 const localParticipant = participants.find(p => p.id === 'local');
103 if (localParticipant) {
104 localParticipant.status = status;
105 updateParticipantsView();
106 }
107 }
108});
109
In this script:
- Managing Participants: We maintain an array of participants and dynamically update the participant list whenever there are changes.
- Adding Local Participant: The local user is added to the participant list upon joining.
- Updating Participant Status: We update the local participant’s status when the mute/unmute button is toggled.
By implementing the participant view, users can see and manage the list of participants, enhancing the interaction and management capabilities within the virtual classroom. In the next section, we will focus on running and testing your BigBlueButton application to ensure all features work seamlessly.
Step 6: Run Your Code Now
After implementing the various features of your BigBlueButton application, it's time to compile and run your code. This section will guide you through the final steps to ensure everything is set up correctly and your application is functioning as expected.
Running the Application
To run your BigBlueButton application, follow these steps:
[a] Start the BigBlueButton Server
Ensure that your BigBlueButton server is up and running. If you haven't started it yet, use the following command:
bash
1 sudo bbb-conf --start
2
[b] Open the Application in a Browser
Open your preferred web browser and navigate to the URL where your BigBlueButton application is hosted. If you are running it locally, this might be
http://localhost:3000
.[c] Join the Classroom
You should see the join screen where you can enter your name and join the classroom. Enter your name and click the "Join" button to proceed to the main classroom interface.
Testing the Setup
To ensure that your BigBlueButton application is functioning correctly, perform the following tests:
Test Audio and Video
- Join the classroom and verify that your video is displayed in the video container.
- Test the mute/unmute button to ensure your audio can be toggled on and off.
- Verify that the video start/stop button works as expected.
Test Screen Sharing
- Click the "Share Screen" button to initiate screen sharing.
- Verify that your screen is being shared and displayed correctly.
- Test stopping the screen share and ensure the video feed returns to your camera.
Test Participant View
- Ensure that your name appears in the participant list.
- Verify that the status (e.g., muted/unmuted) is updated correctly in the participant list when you toggle the mute button.
Test Session End
- Click the "End Session" button and verify that all media tracks are stopped and you are redirected to the join screen.
By performing these tests, you can ensure that all the features of your BigBlueButton application are working as intended.
Conclusion
In this article, we walked through the process of building a BigBlueButton application with WebRTC integration. We started by setting up the initial components, created a join screen, implemented various controls, and added a participant view. By following these steps, you have created a functional virtual classroom application that leverages the power of WebRTC for real-time communication.
BigBlueButton, with its robust features and open-source nature, provides an excellent platform for online learning and virtual classrooms. By customizing and extending its capabilities, you can create a tailored solution that meets the specific needs of your educational environment.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ