OpenAI Voice Generator API: A Comprehensive Guide
Introduction: Harnessing the Power of OpenAI's Voice Generation
OpenAI's
openai voice generator api
offers a powerful way to convert text into realistic and engaging speech. This technology opens doors to a wide range of applications, from accessibility solutions to innovative content creation tools. This guide will walk you through everything you need to know to get started with the OpenAI text to speech
API, also known as the openai tts api
. We'll explore its features, capabilities, and best practices.What is the OpenAI Voice Generator API?
The
openai voice generator api
is a cloud-based service that allows developers to programmatically generate speech from text. It leverages advanced ai voice generator api
models to produce high-quality, natural-sounding audio. It's a powerful tool for anyone looking to integrate speech synthesis
into their applications.OpenAI Voice Generator example
This project integrates VideoSDK, OpenAI Realtime APIs to create an AI Translator Agent. Below are the setup instructions.
<iframe
src="
https://www.youtube.com/embed/aKIkGsKc1fc?rel=0
" style=" position: absolute; top: 0; left: 0; width: 100%; height: 100%; border: 0; " allowfullscreen frameborder="0"</iframe> </div>
Key Features and Benefits
- High-Quality Speech: Produces natural and human-like speech.
- Customization: Offers options to adjust voice parameters like speed, pitch, and tone.
- Multiple Languages: Supports a variety of languages and voices.
- Easy Integration: Simple API calls make it easy to integrate into existing applications.
- Scalability: Designed to handle a large number of requests.
Target Audience
This guide is intended for developers, programmers, and anyone interested in using the OpenAI
speech api
to build voice-enabled applications. Whether you're a seasoned developer or just starting out, this guide will provide you with the knowledge and resources you need to succeed.Understanding the OpenAI Voice Generator API
The OpenAI
convert text to speech api
provides a streamlined way to generate audio from text. Understanding its core components is crucial for effective utilization.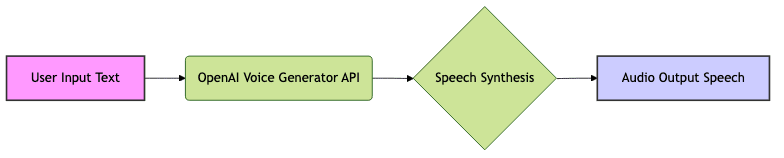
API Endpoints and Functionality
The primary endpoint for the
openai voice generator api
is used to send text data and receive audio data. The API accepts text as input and returns an audio file (e.g., MP3) as output. You can control various aspects of the generated speech through parameters in the API request.Authentication and API Keys
To access the OpenAI API, you need an API key. You can obtain an API key by signing up for an OpenAI account on their website. Keep your API key secure, as it is used to authenticate your requests and track your usage.
python
1import openai
2
3# Set your OpenAI API key
4openai.api_key = "YOUR_OPENAI_API_KEY" # Replace with your actual API key
5
6# Example of setting the API key in an environment variable:
7# import os
8# openai.api_key = os.environ.get("OPENAI_API_KEY")
9
Supported Languages and Voices
The OpenAI API supports a range of languages and voices. Refer to the official
openai api documentation
for the most up-to-date list of supported languages and available tts models
. Experiment with different voices to find the best fit for your application.Rate Limits and Pricing
The OpenAI API has rate limits to prevent abuse and ensure fair usage. The
openai api pricing
is based on usage, typically calculated per character or request. It's important to understand the rate limits and pricing structure to optimize your API usage and avoid unexpected costs. Check the OpenAI website for the most current pricing details.Getting Started with the OpenAI Voice Generator API
This section guides you through the initial steps of setting up your development environment and making your first API call to the OpenAI
generate speech api
.Setting up your Development Environment
Before you can start using the OpenAI API, you need to set up your development environment. This typically involves installing the OpenAI Python library.
python
1# Install the OpenAI Python library
2pip install openai
3
Making your First API Call
Here's a simple Python example of generating speech using the OpenAI API. This
openai api examples
demonstrates a basic request.python
1import openai
2
3openai.api_key = "YOUR_OPENAI_API_KEY" # Replace with your actual API key
4
5def generate_speech(text, voice="nova"):
6 try:
7 response = openai.audio.speech.create(
8 model="tts-1",
9 voice=voice,
10 input=text
11 )
12 response.stream_to_file("output.mp3")
13 print("Speech generated successfully!")
14
15 except Exception as e:
16 print(f"Error generating speech: {e}")
17
18
19# Example usage
20text_to_speak = "Hello, this is a test of the OpenAI voice generator API."
21generate_speech(text_to_speak)
22
23
Handling API Responses and Error Codes
It's essential to handle API responses and error codes gracefully. The OpenAI API returns standard HTTP status codes to indicate success or failure. Understanding these codes and implementing proper error handling is crucial for building robust applications.
python
1import openai
2
3openai.api_key = "YOUR_OPENAI_API_KEY" # Replace with your actual API key
4
5
6def generate_speech(text, voice="nova"):
7 try:
8 response = openai.audio.speech.create(
9 model="tts-1",
10 voice=voice,
11 input=text
12 )
13 response.stream_to_file("output.mp3")
14 print("Speech generated successfully!")
15
16 except openai.APIConnectionError as e:
17 print(f"Failed to connect to OpenAI API: {e}")
18 except openai.RateLimitError as e:
19 print(f"OpenAI API request exceeded rate limit: {e}")
20 except Exception as e:
21 print(f"An unexpected error occurred: {e}")
22
23
24# Example usage
25text_to_speak = "This is a test."
26generate_speech(text_to_speak)
27
Advanced Usage and Customization
Beyond basic text-to-speech conversion, the OpenAI API offers advanced features for customizing voice parameters and integrating with other services. This section delves into these advanced capabilities.
Customizing Voice Parameters (Speed, Pitch, Tone)
The OpenAI API allows you to adjust voice parameters such as speed and pitch to fine-tune the generated speech. These parameters can be set in the API request to achieve the desired voice characteristics.
python
1import openai
2
3openai.api_key = "YOUR_OPENAI_API_KEY" # Replace with your actual API key
4
5
6def generate_speech(text, voice="nova", speed=1.2):
7 try:
8 response = openai.audio.speech.create(
9 model="tts-1",
10 voice=voice,
11 input=text, # Add speed parameter
12 speed=speed
13 )
14 response.stream_to_file("output.mp3")
15 print("Speech generated successfully!")
16
17 except Exception as e:
18 print(f"Error generating speech: {e}")
19
20
21# Example usage
22text_to_speak = "This is a test with a faster speed."
23generate_speech(text_to_speak, speed=1.5)
24
25
Streaming Audio for Real-time Applications
For real-time applications like chatbots or live narration, streaming audio is essential. The OpenAI API supports streaming audio, allowing you to receive the audio data in chunks as it is generated.
python
1import openai
2
3openai.api_key = "YOUR_OPENAI_API_KEY"
4
5
6def stream_speech(text, voice="nova"):
7 try:
8 response = openai.audio.speech.create(
9 model="tts-1",
10 voice=voice,
11 input=text
12 )
13
14 # Stream the audio data
15 with open("output.mp3", "wb") as f:
16 f.write(response.content)
17
18 print("Streaming audio saved to output.mp3")
19
20 except Exception as e:
21 print(f"Error streaming audio: {e}")
22
23
24# Example usage
25text_to_speak = "This is a test of streaming audio."
26stream_speech(text_to_speak)
27
Integrating with Other APIs and Services
The
openai voice generator api
can be integrated with other APIs and services to create more complex and powerful applications. For example, you can combine it with a speech recognition
API to build a voice-controlled application, or with natural language processing (NLP)
to generate dynamic and personalized content. You can even perform voice modulation
to produce different kinds of voices using the speech synthesis capability.Building a Voice-Enabled Application (Example)
Consider building a simple voice-enabled news reader application. This application would use an API to fetch news articles, then use the OpenAI
ai text to speech
API to read the articles aloud to the user.Best Practices and Optimization
To maximize the efficiency and cost-effectiveness of using the OpenAI API, follow these best practices.
Efficient API Usage
Minimize the number of API calls by batching requests when possible. Cache frequently used audio to avoid generating the same audio repeatedly. Be mindful of the API's rate limits and pricing structure.
Handling Large Audio Files
For large audio files, consider streaming the audio data instead of generating the entire file at once. This reduces memory usage and improves performance. The
openai api python
or openai api javascript
examples provide a good starting point.Optimizing for Cost
Monitor your API usage and adjust your application accordingly. Experiment with different voice parameters and
tts models
to find the optimal balance between quality and cost. Consider using lower-cost models for non-critical applications.Real-World Applications and Use Cases
The OpenAI
speech synthesis
API has a wide range of real-world applications across various industries.Accessibility and Inclusivity
Generate audio descriptions for visual content, providing access to information for visually impaired users. Create audio versions of written materials, making them accessible to individuals with reading disabilities.
Content Creation and Storytelling
Create
ai voice over
for videos and animations, bringing stories to life. Develop interactive audiobooks and podcasts with realistic and engaging narration. Use voice cloning software
to build unique personalized experiences.Customer Service and Chatbots
Enable voice-based customer service interactions, providing a more natural and human-like experience. Build chatbots that can respond to user queries using realistic speech.
Education and Training
Create interactive learning materials with audio narration. Develop virtual tutors that can provide personalized instruction using voice. The
openai api node.js
can be used to create interactive web-based learning experiences.Security and Ethical Considerations
It is crucial to consider the security and ethical implications of using AI-powered voice generation technology.
Custom voice generation
must be used responsibly.Preventing Misuse and Malicious Applications
Implement safeguards to prevent the creation of deepfakes and other malicious applications. Monitor API usage for suspicious activity. Consider the potential for misuse when designing your application.
Data Privacy and Security
Protect user data and privacy by implementing appropriate security measures. Comply with relevant data privacy regulations. Be transparent about how you are using user data.
Transparency and Disclosure
Disclose to users that the audio they are hearing is generated by AI. Avoid using AI-generated voices to impersonate real people without their consent.
Want to level-up your learning? Subscribe now
Subscribe to our newsletter for more tech based insights
FAQ